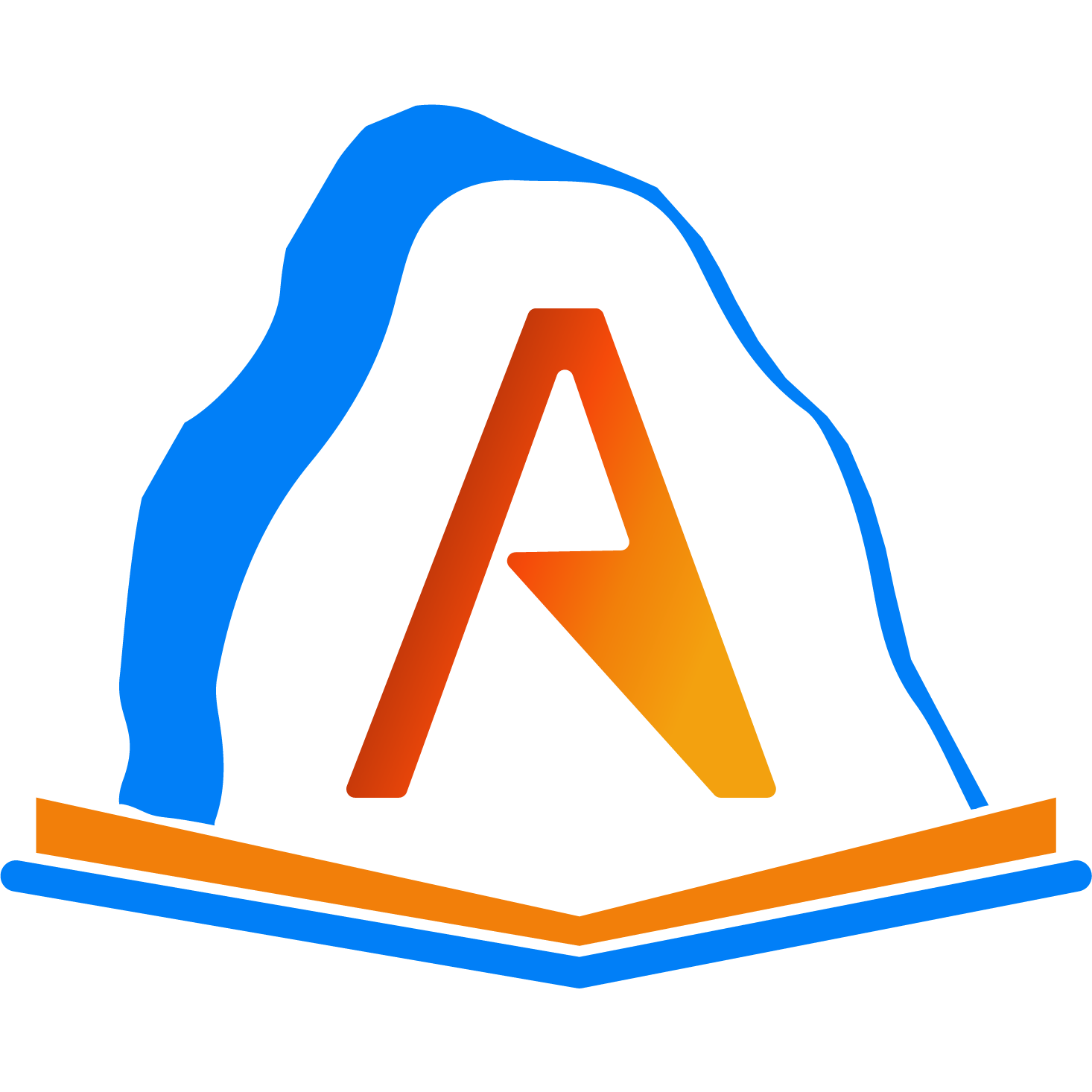
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 9.1 Objectives
- 9.2 Vocabulary and Definitions
- 9.3 The Graph Abstract Data Type
- 9.4 An Adjacency Matrix
- 9.5 An Adjacency List
- 9.6 Implementation
- 9.7 The Word Ladder Problem
- 9.8 Building the Word Ladder Graph
- 9.9 Implementing Breadth First Search
- 9.10 Breadth First Search Analysis
- 9.11 The Knight’s Tour Problem
- 9.12 Building the Knight’s Tour Graph
- 9.13 Implementing Knight’s Tour
- 9.14 Knight’s Tour Analysis
- 9.15 General Depth First Search
- 9.16 Depth First Search Analysis
- 9.17 Topological Sorting
- 9.18 Strongly Connected Components
- 9.19 Shortest Path Problems
- 9.20 Dijkstra’s Algorithm
- 9.21 Analysis of Dijkstra’s Algorithm
- 9.22 Prim’s Spanning Tree Algorithm
- 9.23 Summary
- 9.24 Discussion Questions
- 9.25 Programming Exercises
- 9.26 Glossary
- 9.2. Vocabulary and Definitions" data-toggle="tooltip">
- 9.4. An Adjacency Matrix' data-toggle="tooltip" >
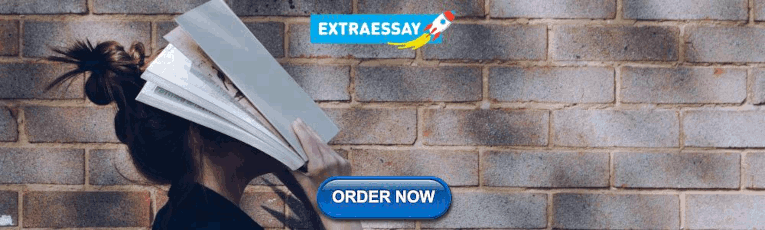
9.3. The Graph Abstract Data Type ¶
The graph abstract data type (ADT) is defined as follows:
Graph() creates a new, empty graph.
addVertex(vert) adds an instance of Vertex to the graph.
addEdge(fromVert, toVert) Adds a new, directed edge to the graph that connects two vertices.
addEdge(fromVert, toVert, weight) Adds a new, weighted, directed edge to the graph that connects two vertices.
getVertex(vertKey) finds the vertex in the graph named vertKey .
getVertices() returns the list of all vertices in the graph.
in returns True for a statement of the form vertex in graph , if the given vertex is in the graph, False otherwise.
Beginning with the formal definition for a graph there are several ways we can implement the graph ADT in Python. We will see that there are trade-offs in using different representations to implement the ADT described above. There are two well-known implementations of a graph, the adjacency matrix and the adjacency list . We will explain both of these options, and then implement one as a Python class.
- creates a new, empty graph.
- addVertex(vert)
- adds an instance of Vertex to the graph.
- addEdge(fromVert, toVert)
- Adds a new, directed edge to the graph that connects two vertices.
- addEdge(fromVert, toVert, weight)
- Adds a new, weighted, directed edge to the graph that connects two vertices.
- getVertex(vertKey)
- finds the vertex in the graph named vertKey.
- getVertices()
- returns the list of all vertices in the graph.
- returns True for a statement of the form vertex in graph, if the given vertex is in the graph, False otherwise.
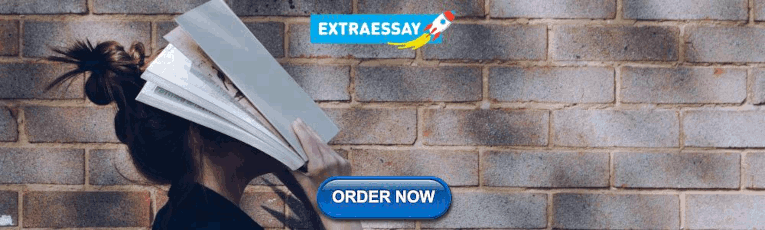
IMAGES
VIDEO
COMMENTS
It's easier to figure out tough problems faster using Chegg Study. Unlike static PDF Data Abstraction and Problem Solving with C++ solution manuals or printed answer keys, our experts show you how to solve each problem step-by-step. No need to wait for office hours or assignments to be graded to find out where you took a wrong turn.
With Expert Solutions for thousands of practice problems, you can take the guesswork out of studying and move forward with confidence. Science. Computer Science. Data Abstraction and Problem Solving with C++. 7th Edition. ISBN: 9780134463971. Alternate ISBNs. Frank Carrano, Timothy Henry. Textbook solutions.
Find step-by-step solutions and answers to Exercise 4 from Data Abstraction and Problem Solving with C++ - 9780134463971, as well as thousands of textbooks so you can move forward with confidence.
The text Explores problem solving and the efficient access and manipulation of data and is intended for readers who already have a basic understanding of C++. The "walls and mirrors" mentioned in the title represent problem-solving techniques that appear throughout the text. Data abstraction hides the details of a module from the rest of ...
This instructor's guide, which supplements Data Abstraction and Problem Solving with C++: Walls and Mirrors, is organized as follows: Three Possible Courses Based on Walls and Mirrors. We begin by offering suggestions for how to use Walls and Mirrors in your course. The book's flexibility will allow you to use it in a variety of
•To review the ideas of computer science, programming, and problem-solving. •To understand abstraction and the role it plays in the problem-solving process. •To understand and implement the notion of an abstract data type. •To review the Python programming language. 1.2Getting Started
The text explores problem solving and the efficient access and manipulation of data and is intended for students who already have a basic understanding of programming, preferably in C++. The "walls and mirrors" mentioned in the title represent problem-solving techniques that appear throughout the text. Data abstraction hides the details of ...
The Graph Abstract Data Type — Problem Solving with Algorithms and Data Structures using C++. 9.3. The Graph Abstract Data Type ¶. The graph abstract data type (ADT) is defined as follows: Graph() creates a new, empty graph. addVertex(vert) adds an instance of Vertex to the graph. addEdge(fromVert, toVert) Adds a new, directed edge to the ...
From the Publisher: Focusing on data abstraction and data structures, the second edition of this very successful book continues to emphasize the needs of both the instructor and the student. The book illustrates the role of classes and abstract data types (ADTs) in the problem-solving process as the foundation for an object-oriented approach.
Find step-by-step solutions and answers to Data Abstraction & Problem Solving with C++ - 9780134463971, as well as thousands of textbooks so you can move forward with confidence.
Data Abstraction & Problem Solving with C++: Walls and Mirrors. Published 2021. Paperback. $170.66. Price Reduced From: $213.32. Buy now. Free delivery. ISBN-13: 9780134463971. Data Abstraction & Problem Solving with C++: Walls and Mirrors. Published 2016. Need help? Get in touch
Now, with expert-verified solutions from Data Abstraction and Problem Solving with C 7th Edition, you'll learn how to solve your toughest homework problems. Our resource for Data Abstraction and Problem Solving with C includes answers to chapter exercises, as well as detailed information to walk you through the process step by step.
The overall goal of CSS 342 is to learn discrete mathematics concepts and computer programming. You solve mathematical problems, present formal mathematical arguments, and program solutions to problems. You review searching and sorting algorithms, object‐oriented programming, basic abstract data types, and study algorithm analysis.
Data Abstraction & Problem Solving With C++: Walls & Mirrors, Seventh Edition, Frank M. Carrano, Addison Wesley, 2017, ISBN-13: 9780134463971 An Active Introduction to Discrete Mathematics and Algorithms by Cusack, 2018 Grading Policy Course Work % Midterm exam 25 Final exam 25 Quizzes 10 In-Class Work 10 Assignments 30 Total 100
Data Abstraction & Problem Solving with C++: Walls and Mirrors. Published 2021. Paperback. $159.99. Price Reduced From: $199.99. Buy now. Free delivery. ISBN-13: 9780134463971. Data Abstraction & Problem Solving with C++: Walls and Mirrors. Published 2016. Need help?
Find step-by-step solutions and answers to Exercise 4 from Data Abstraction and Problem Solving with C++ - 9780134463971, as well as thousands of textbooks so you can move forward with confidence. ... For instance, if for a problem of size n n n, the algorithm needs time proportional with n 3 n^3 n 3, then the big O of the algorithm is O (n 3 ...
A person with low cohesion has "too many irons in the fire". Promotes self-documenting, easy-to-understand code. Easy to reuse in other software projects. Easy to revise or correct. Robust: less likely to be affected by change; performs well under unusual conditions. Promotes low coupling. Solutions.
With Expert Solutions for thousands of practice problems, you can take the guesswork out of studying and move forward with confidence. Science. Computer Science. Data Abstraction Problem Solving with C++. 6th Edition. ISBN: 9780132923729. D Henry, Frank Carrano, Timothy Henry. Textbook solutions. Verified.
It's easier to figure out tough problems faster using Chegg Study. Unlike static PDF Data Abstraction and Problem Solving with C++ solution manuals or printed answer keys, our experts show you how to solve each problem step-by-step. No need to wait for office hours or assignments to be graded to find out where you took a wrong turn.