cppreference.com
Copy assignment operator.
(C++20) | ||||
(C++20) | ||||
(C++11) | ||||
(C++11) | ||||
(C++11) | ||||
(C++17) | ||||
General | ||||
Members | ||||
pointer | ||||
(C++11) | ||||
specifier | ||||
specifier | ||||
Special member functions | ||||
(C++11) | ||||
(C++11) | ||||
Inheritance | ||||
(C++11) | ||||
(C++11) |
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . For a type to be CopyAssignable , it must have a public copy assignment operator.
Syntax Explanation Implicitly-declared copy assignment operator Deleted implicitly-declared copy assignment operator Trivial copy assignment operator Implicitly-defined copy assignment operator Notes Example Defect reports |
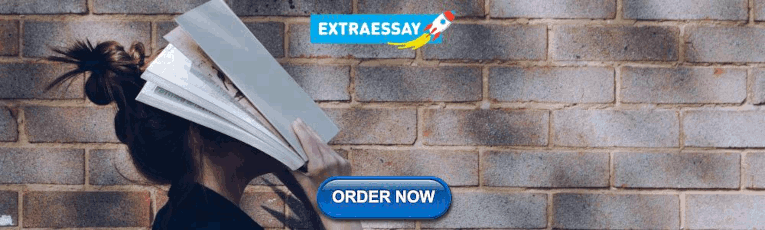
[ edit ] Syntax
class_name class_name ( class_name ) | (1) | ||||||||
class_name class_name ( const class_name ) | (2) | ||||||||
class_name class_name ( const class_name ) = default; | (3) | (since C++11) | |||||||
class_name class_name ( const class_name ) = delete; | (4) | (since C++11) | |||||||
[ edit ] Explanation
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used.
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used (non-swappable type or degraded performance).
- Forcing a copy assignment operator to be generated by the compiler.
- Avoiding implicit copy assignment.
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) exception specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
A implicitly-declared copy assignment operator for class T is defined as deleted if any of the following is true:
- T has a user-declared move constructor;
- T has a user-declared move assignment operator.
Otherwise, it is defined as defaulted.
A defaulted copy assignment operator for class T is defined as deleted if any of the following is true:
- T has a non-static data member of non-class type (or array thereof) that is const ;
- T has a non-static data member of a reference type;
- T has a non-static data member or a direct or virtual base class that cannot be copy-assigned (overload resolution for the copy assignment fails, or selects a deleted or inaccessible function);
- T is a union-like class , and has a variant member whose corresponding assignment operator is non-trivial.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted) , , and if it is defaulted, its signature is the same as implicitly-defined (until C++14) ;
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial;
has no non-static data members of -qualified type. | (since C++14) |
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
DR | Applied to | Behavior as published | Correct behavior |
---|---|---|---|
C++14 | operator=(X&) = default was non-trivial | made trivial |
- Pages with unreviewed CWG DR marker
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 9 January 2019, at 07:16.
- This page has been accessed 570,566 times.
- Privacy policy
- About cppreference.com
- Disclaimers
std::remove_copy_if() algorithm
- since C++20
- since C++17
- until C++17
(1) Ignores all elements for which predicate p returns true .
(2) Same as (1) , but executed according to policy .
These overloads participate in overload resolution only if std::is_execution_policy_v<std::decay_t<ExecutionPolicy>> ( until C++20 ) std::is_execution_policy_v<std::remove_cvref_t<ExecutionPolicy>> ( since C++20 ) is true .
- since C++11
- until C++11
Removing is done by shifting (by means of copy assignment ( until C++11 ) move assignment ( since C++11 ) ) the elements in the range in such a way that the elements that are not to be removed appear in the beginning of the range.
Relative order of the elements that remain is preserved and the physical size of the container is unchanged.
Iterators pointing to an element between the new logical end and the physical end of the range are still dereferenceable , but the elements themselves have unspecified values (as per MoveAssignable post-condition). ( since C++11 )
Parameters
| The range of elements to copy. |
The beginning of the destination range. | |
The execution policy to use. See for details. | |
Unary predicate which returns if the element should be . The expression must be convertible to for every argument of type (possibly const) , where is the value type of , regardless of value category, and must not modify . Thus, a parameter type of is not allowed , nor is unless for a move is equivalent to a copy. . |
Type requirements
| |
- until C++20
Must be to . |
Return value
Iterator to the element past the last element copied.
Complexity
Given N as std::distance(first, last) :
Exactly N applications of the predicate p .
Exceptions
The overloads with a template parameter named ExecutionPolicy report errors as follows:
- If the algorithm fails to allocate memory, std::bad_alloc is thrown.
Possible implementation
The following code outputs a string while erasing the hash characters '#' on the fly.
- Return value
- Possible implementation
cppreference.com

Copy assignment operator
(C++11) | ||||
(C++11) | ||||
(C++11) | ||||
General | ||||
Members | ||||
pointer | ||||
(C++11) | ||||
specifier | ||||
specifier | ||||
Special member functions | ||||
(C++11) | ||||
(C++11) | ||||
Inheritance | ||||
(C++11) | ||||
(C++11) | ||||
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . For a type to be CopyAssignable , it must have a public copy assignment operator.
Syntax Explanation Implicitly-declared copy assignment operator Deleted implicitly-declared copy assignment operator Trivial copy assignment operator Implicitly-defined copy assignment operator Notes Copy and swap Example |
[ edit ] Syntax
class_name class_name ( class_name ) | (1) | ||||||||
class_name class_name ( const class_name ) | (2) | ||||||||
class_name class_name ( const class_name ) = default; | (3) | (since C++11) | |||||||
class_name class_name ( const class_name ) = delete; | (4) | (since C++11) | |||||||
[ edit ] Explanation
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used (non-swappable type or degraded performance)
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
A implicitly-declared copy assignment operator for class T is defined as deleted if any of the following is true:
- T has a user-declared move constructor
- T has a user-declared move assignment operator
Otherwise, it is defined as defaulted.
A defaulted copy assignment operator for class T is defined as deleted if any of the following is true:
- T has a non-static data member of non-class type (or array thereof) that is const
- T has a non-static data member of a reference type.
- T has a non-static data member or a direct or virtual base class that cannot be copy-assigned (overload resolution for the copy assignment fails, or selects a deleted or inaccessible function)
- T is a union-like class , and has a variant member whose corresponding assignment operator is non-trivial.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- It is not user-provided (meaning, it is implicitly-defined or defaulted), and if it is defaulted, its signature is the same as implicitly-defined
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial
has no non-static data members of -qualified type | (since C++14) |
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
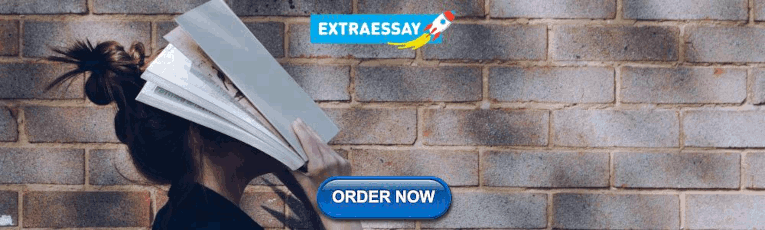
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std::move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move). However, this approach is not always advisable due to potentially significant overhead: see assignment operator overloading for details.
[ edit ] Example
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 30 November 2015, at 07:24.
- This page has been accessed 110,155 times.
- Privacy policy
- About cppreference.com
- Disclaimers

This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Copy constructors and copy assignment operators (C++)
- 8 contributors
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment . In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see Move Constructors and Move Assignment Operators (C++) .
Both the assignment operation and the initialization operation cause objects to be copied.
Assignment : When one object's value is assigned to another object, the first object is copied to the second object. So, this code copies the value of b into a :
Initialization : Initialization occurs when you declare a new object, when you pass function arguments by value, or when you return by value from a function.
You can define the semantics of "copy" for objects of class type. For example, consider this code:
The preceding code could mean "copy the contents of FILE1.DAT to FILE2.DAT" or it could mean "ignore FILE2.DAT and make b a second handle to FILE1.DAT." You must attach appropriate copying semantics to each class, as follows:
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x); .
Use the copy constructor.
If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you. Similarly, if you don't declare a copy assignment operator, the compiler generates a member-wise copy assignment operator for you. Declaring a copy constructor doesn't suppress the compiler-generated copy assignment operator, and vice-versa. If you implement either one, we recommend that you implement the other one, too. When you implement both, the meaning of the code is clear.
The copy constructor takes an argument of type ClassName& , where ClassName is the name of the class. For example:
Make the type of the copy constructor's argument const ClassName& whenever possible. This prevents the copy constructor from accidentally changing the copied object. It also lets you copy from const objects.
Compiler generated copy constructors
Compiler-generated copy constructors, like user-defined copy constructors, have a single argument of type "reference to class-name ." An exception is when all base classes and member classes have copy constructors declared as taking a single argument of type const class-name & . In such a case, the compiler-generated copy constructor's argument is also const .
When the argument type to the copy constructor isn't const , initialization by copying a const object generates an error. The reverse isn't true: If the argument is const , you can initialize by copying an object that's not const .
Compiler-generated assignment operators follow the same pattern for const . They take a single argument of type ClassName& unless the assignment operators in all base and member classes take arguments of type const ClassName& . In this case, the generated assignment operator for the class takes a const argument.
When virtual base classes are initialized by copy constructors, whether compiler-generated or user-defined, they're initialized only once: at the point when they are constructed.
The implications are similar to the copy constructor. When the argument type isn't const , assignment from a const object generates an error. The reverse isn't true: If a const value is assigned to a value that's not const , the assignment succeeds.
For more information about overloaded assignment operators, see Assignment .
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
21.12 — Overloading the assignment operator
- Graphics and multimedia
- Language Features
- Unix/Linux programming
- Source Code
- Standard Library
- Tips and Tricks
- Tools and Libraries
- Windows API
- Copy constructors, assignment operators,
Copy constructors, assignment operators, and exception safe assignment

MyClass& other ); MyClass( MyClass& other ); MyClass( MyClass& other ); MyClass( MyClass& other ); |
MyClass* other ); |
MyClass { x; c; std::string s; }; |
MyClass& other ) : x( other.x ), c( other.c ), s( other.s ) {} |
); |
print_me_bad( std::string& s ) { std::cout << s << std::endl; } print_me_good( std::string& s ) { std::cout << s << std::endl; } std::string hello( ); print_me_bad( hello ); print_me_bad( std::string( ) ); print_me_bad( ); print_me_good( hello ); print_me_good( std::string( ) ); print_me_good( ); |
, ); |
=( MyClass& other ) { x = other.x; c = other.c; s = other.s; * ; } |
< T > MyArray { size_t numElements; T* pElements; : size_t count() { numElements; } MyArray& =( MyArray& rhs ); }; |
<> MyArray<T>:: =( MyArray& rhs ) { ( != &rhs ) { [] pElements; pElements = T[ rhs.numElements ]; ( size_t i = 0; i < rhs.numElements; ++i ) pElements[ i ] = rhs.pElements[ i ]; numElements = rhs.numElements; } * ; } |
<> MyArray<T>:: =( MyArray& rhs ) { MyArray tmp( rhs ); std::swap( numElements, tmp.numElements ); std::swap( pElements, tmp.pElements ); * ; } |
< T > swap( T& one, T& two ) { T tmp( one ); one = two; two = tmp; } |
<> MyArray<T>:: =( MyArray tmp ) { std::swap( numElements, tmp.numElements ); std::swap( pElements, tmp.pElements ); * ; } |
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Copy Constructor vs Assignment Operator in C++
Copy constructor and Assignment operator are similar as they are both used to initialize one object using another object. But, there are some basic differences between them:
Copy constructor | Assignment operator |
---|---|
It is called when a new object is created from an existing object, as a copy of the existing object | This operator is called when an already initialized object is assigned a new value from another existing object. |
It creates a separate memory block for the new object. | It does not create a separate memory block or new memory space. |
It is an overloaded constructor. | It is a bitwise operator. |
C++ compiler implicitly provides a copy constructor, if no copy constructor is defined in the class. | A bitwise copy gets created, if the Assignment operator is not overloaded. |
className(const className &obj) { // body } |
className obj1, obj2; obj2 = obj1; |
Consider the following C++ program.
Explanation: Here, t2 = t1; calls the assignment operator , same as t2.operator=(t1); and Test t3 = t1; calls the copy constructor , same as Test t3(t1);
Must Read: When is a Copy Constructor Called in C++?
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
Get notified in your email when a new post is published to this blog
Writing a remove_all_pointers type trait, part 2
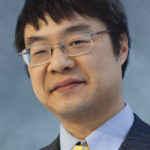
Raymond Chen
June 28th, 2024 2 0
Last time, we wrote a remove_ all_ pointers type trait , but I noted that even though we found a solution, we weren’t finished yet.
We can bring back the one-liner by using a different trick to delay the recursion: Don’t ask for the type until we know we really are recursing.
The sketch is
We first define a type_holder to be a type which has a type member type that holds our answer. If T is a pointer, then the type holder is the recursive call. Otherwise, the type holder is a dummy type whose sole purpose is to have a type member type that produces T again.
We can now pack up that if into a std:: conditional .
It turns out that we don’t need to define a dummy : The C++ standard library comes with one built in! It’s called std:: type_ identity<T> , available starting in C++20. ( We looked at std:: type_ identity<T> a little while ago .)
Now we can inline the type_holder .
Or even better, just derive from the type_holder !
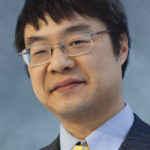
Leave a comment Cancel reply
Log in to join the discussion or edit/delete existing comments.
The Aha moment after comparing the initial attempt and the final approach: A manual design of short circuitry.
So basically moving the ::type outside the std::conditional_t fixes it, because this way you don’t construct the template unless it’s necessary. (But the last optimisation is nice.)
- remove_all_pointers type trait, part 2" title="Share on Twitter" aria-label="Share on Twitter" target="_blank" rel="noopener noreferrer nofollow" class="sublink twitter" tabindex="-1">

Insert/edit link
Enter the destination URL
Or link to existing content
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Deleted implicitly-declared copy assignment operator
According to the C++ reference on Copy assignment operator :
A defaulted copy assignment operator for class T is defined as deleted if any of the following is true T has a non-static data member of non-class type (or array thereof) that is const ...
I was hoping to create a case where I had a const class-type data member and a defaulted copy assignment operator not defined as deleted. In doing so, I found a discrepancy between clang and gcc. Consider the following code:
When I compile this with g++ -std=c++14 I get the following errors:
This does, comma, however, compile with clang , as the reference seems to indicate that it should. Am I in error? Which compiler is correct?
I'm using gcc version 7.3.0 (Ubuntu 7.3.0-27ubuntu1~18.04) and clang version 6.0.0-1ubuntu2 .
- assignment-operator
- In general you want to use C++ since that gives the question a much wider audience than C++ version specific tags. – Shafik Yaghmour Commented Nov 8, 2018 at 19:41
2 Answers 2
It seems that clang is right,
Although not yet confirmed, there is a report on the subject for gcc and as it was pointed out, the two rules relevant to this case don't apply
[class.copy.assign]/7
(7.2) a non-static data member of const non-class type (or array thereof), or [...] (7.4) a direct non-static data member of class type M (or array thereof) or a direct base class M that cannot be copied/moved because overload resolution ([over.match]), as applied to find M's corresponding assignment operator, results in an ambiguity or a function that is deleted or inaccessible from the defaulted assignment operator.
It sure looks like clang is correct, section [class.copy.assign]p7 says:
A defaulted copy/move assignment operator for class X is defined as deleted if X has: (7.1) a variant member with a non-trivial corresponding assignment operator and X is a union-like class, or (7.2) a non-static data member of const non-class type (or array thereof), or (7.3) a non-static data member of reference type, or (7.4) a direct non-static data member of class type M (or array thereof) or a direct base class M that cannot be copied/moved because overload resolution ([over.match]), as applied to find M's corresponding assignment operator, results in an ambiguity or a function that is deleted or inaccessible from the defaulted assignment operator. A defaulted move assignment operator that is defined as deleted is ignored by overload resolution ([over.match], [over.over]).
and none of those cases hold.
Although I have to say a const copy assignment operator that returns void feels novel, the wording in [class.copy.assign]p1 sure seems to allow.
There is an open gcc bug report for a similar case Const subobject with const assignment operator, but operator anyway deleted with code as follows:
The reporter points out the same section as I do.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c++ g++ c++14 clang++ assignment-operator or ask your own question .
- The Overflow Blog
- How to build open source apps in a highly regulated industry
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- PWM Dimming of a Low-Voltage DC Incandescent Filament (Thermal Shock?)
- Ideal diode in parallel with resistor and voltage source
- Unsorted Intersection
- Identify rear derailleur (Shimano 105 - medium or short)
- Is it possible to arrange the free n-minoes of orders 2, 3, 4 and 5 into a rectangle?
- mirrorlist.centos.org no longer resolve?
- Can someone explain the Trump immunity ruling?
- Con permiso to enter your own house?
- If a lambda is declared as a default argument, is it different for each call site?
- Can you help me to identify the aircraft in a 1920s photograph?
- How to handle a missing author on an ECCV paper submission after the deadline?
- I can't mount my external hard drive in Linux
- Is non-temperature related Symmetry Breaking possible?
- Book that I read around 1975, where the main character is a retired space pilot hired to steal an object from a lab called Menlo Park
- Remove duplicates in file (without sorting!) leaving the _last_ of the occurences
- A very basic autosegmental tree using forest
- Did the BBC censor a non-binary character in Transformers: EarthSpark?
- Do we know a lower bound or the exact number of 3-way races in France's 2nd round of elections in 2024?
- Powers of Gaussian primes are NOT collinear
- lme4 Inconsistency
- Geometry question about a six-pack of beer
- How to maintain dependencies shared among microservices?
- Does the Ogre-Faced Spider regenerate part of its eyes daily?
- I want to leave my current job during probation but I don't want to tell the next interviewer I am currently working
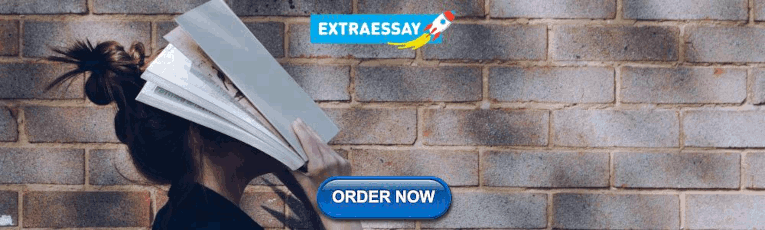
IMAGES
VIDEO
COMMENTS
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type. [] NoteIf both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move), and selects the copy assignment if the argument is an ...
std::remove_if requires that the objects obtained by dereferencing the iterator be MoveAssignable (§25.3.8/1). But because you've explicitly deleted the copy assignment operator, the move assignment operator is also implicitly deleted.. Assuming Session can support move semantics, you can get remove_if to work by defining a move assignment operator. For instance, simply adding a defaulted ...
For the overloads with an ExecutionPolicy, there may be a performance cost if ForwardIt1's value_type is not MoveConstructible. [] ExceptionThe overloads with a template parameter named ExecutionPolicy report errors as follows: . If execution of a function invoked as part of the algorithm throws an exception and ExecutionPolicy is one of the standard policies, std::terminate is called.
Explanation. Removing is done by shifting the elements in the range in such a way that the elements that are not to be removed appear in the beginning of the range. Shifting is done by copy assignment (until C++11) move assignment (since C++11) . The removing operation is stable: the relative order of the elements not to be removed stays the same.
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial; T has no non-static data members of volatile-qualified type. (since C++14) A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD ...
first last: The range of elements to copy. d_first: The beginning of the destination range. policy: The execution policy to use. See execution policy for details.. p: Unary predicate which returns true if the element should be ignored.. The expression p(v) must be convertible to bool for every argument v of type (possibly const) VT, where VT is the value type of InputIt, regardless of value ...
The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
The copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial T has no non-static data members of volatile-qualified type (since C++14) A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD ...
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
21.12 — Overloading the assignment operator. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
The first line runs the copy constructor of T, which can throw; the remaining lines are assignment operators which can also throw. HOWEVER, if you have a type T for which the default std::swap() may result in either T's copy constructor or assignment operator throwing, you are
The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial T has no non-static data members of volatile-qualified type (since C++14) A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD ...
assignment operator to assign two the value of the variable one. It can be tricky to differentiate between code using the assignment operator and code using the copy constructor. For example, if we rewrite the above code as MyClass one; MyClass two = one; We are now invoking the copy constructor rather than the assignment operator. Always ...
But, there are some basic differences between them: Copy constructor. Assignment operator. It is called when a new object is created from an existing object, as a copy of the existing object. This operator is called when an already initialized object is assigned a new value from another existing object. It creates a separate memory block for ...
1) Copy assignment operator. Replaces the contents with a copy of the contents of other . If std::allocator_traits<allocator_type>::propagate_on_container_copy_assignment::value is true, the allocator of *this is replaced by a copy of other. If the allocator of *this after assignment would compare unequal to its old value, the old allocator is ...
Copy assignment operators (C++ only) The copy assignment operator lets you create a new object from an existing one by initialization. A copy assignment operator of a class A is a nonstatic non-template member function that has one of the following forms: If you do not declare a copy assignment operator for a class A, the compiler will ...
Triviality of eligible move assignment operators determines whether the class is a trivially copyable type. [] NoteIf both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move), and selects the copy assignment if the argument is an ...
If you declare a copy constructor (even if you define it as deleted in the declaration), no move constructor will be declared implicitly. Cf. C++11 12.8/9: If the definition of a class X does not explicitly declare a move constructor, one will be implicitly declared as defaulted if and only if — X does not have a user-declared copy ...
Last time, we wrote a remove_ all_ pointers type trait, but I noted that even though we found a solution, we weren't finished yet.. We can bring back the one-liner by using a different trick to delay the recursion: Don't ask for the type until we know we really are recursing.. The sketch is. template<typename T> struct dummy { using type = T; }; template<typename T> struct remove_all ...
7. According to the C++ reference on Copy assignment operator: A defaulted copy assignment operator for class T is defined as deleted if any of the following is true. T has a non-static data member of non-class type (or array thereof) that is const ... I was hoping to create a case where I had a const class-type data member and a defaulted copy ...