- Python »
- 3.8.18 Documentation »
- The Python Tutorial »
- Theme Auto Light Dark |
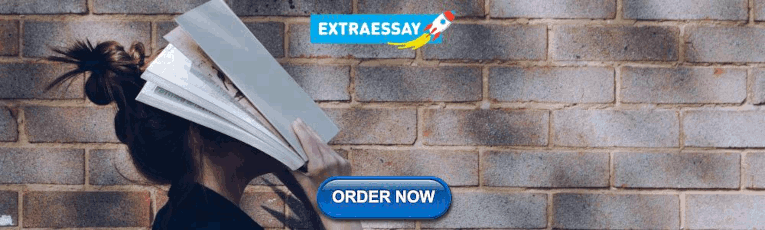
5. Data Structures ¶
This chapter describes some things you’ve learned about already in more detail, and adds some new things as well.
5.1. More on Lists ¶
The list data type has some more methods. Here are all of the methods of list objects:
Add an item to the end of the list. Equivalent to a[len(a):] = [x] .
Extend the list by appending all the items from the iterable. Equivalent to a[len(a):] = iterable .
Insert an item at a given position. The first argument is the index of the element before which to insert, so a.insert(0, x) inserts at the front of the list, and a.insert(len(a), x) is equivalent to a.append(x) .
Remove the first item from the list whose value is equal to x . It raises a ValueError if there is no such item.
Remove the item at the given position in the list, and return it. If no index is specified, a.pop() removes and returns the last item in the list. (The square brackets around the i in the method signature denote that the parameter is optional, not that you should type square brackets at that position. You will see this notation frequently in the Python Library Reference.)
Remove all items from the list. Equivalent to del a[:] .
Return zero-based index in the list of the first item whose value is equal to x . Raises a ValueError if there is no such item.
The optional arguments start and end are interpreted as in the slice notation and are used to limit the search to a particular subsequence of the list. The returned index is computed relative to the beginning of the full sequence rather than the start argument.
Return the number of times x appears in the list.
Sort the items of the list in place (the arguments can be used for sort customization, see sorted() for their explanation).
Reverse the elements of the list in place.
Return a shallow copy of the list. Equivalent to a[:] .
An example that uses most of the list methods:
You might have noticed that methods like insert , remove or sort that only modify the list have no return value printed – they return the default None . 1 This is a design principle for all mutable data structures in Python.
Another thing you might notice is that not all data can be sorted or compared. For instance, [None, 'hello', 10] doesn’t sort because integers can’t be compared to strings and None can’t be compared to other types. Also, there are some types that don’t have a defined ordering relation. For example, 3+4j < 5+7j isn’t a valid comparison.
5.1.1. Using Lists as Stacks ¶
The list methods make it very easy to use a list as a stack, where the last element added is the first element retrieved (“last-in, first-out”). To add an item to the top of the stack, use append() . To retrieve an item from the top of the stack, use pop() without an explicit index. For example:
5.1.2. Using Lists as Queues ¶
It is also possible to use a list as a queue, where the first element added is the first element retrieved (“first-in, first-out”); however, lists are not efficient for this purpose. While appends and pops from the end of list are fast, doing inserts or pops from the beginning of a list is slow (because all of the other elements have to be shifted by one).
To implement a queue, use collections.deque which was designed to have fast appends and pops from both ends. For example:
5.1.3. List Comprehensions ¶
List comprehensions provide a concise way to create lists. Common applications are to make new lists where each element is the result of some operations applied to each member of another sequence or iterable, or to create a subsequence of those elements that satisfy a certain condition.
For example, assume we want to create a list of squares, like:
Note that this creates (or overwrites) a variable named x that still exists after the loop completes. We can calculate the list of squares without any side effects using:
or, equivalently:
which is more concise and readable.
A list comprehension consists of brackets containing an expression followed by a for clause, then zero or more for or if clauses. The result will be a new list resulting from evaluating the expression in the context of the for and if clauses which follow it. For example, this listcomp combines the elements of two lists if they are not equal:
and it’s equivalent to:
Note how the order of the for and if statements is the same in both these snippets.
If the expression is a tuple (e.g. the (x, y) in the previous example), it must be parenthesized.
List comprehensions can contain complex expressions and nested functions:
5.1.4. Nested List Comprehensions ¶
The initial expression in a list comprehension can be any arbitrary expression, including another list comprehension.
Consider the following example of a 3x4 matrix implemented as a list of 3 lists of length 4:
The following list comprehension will transpose rows and columns:
As we saw in the previous section, the nested listcomp is evaluated in the context of the for that follows it, so this example is equivalent to:
which, in turn, is the same as:
In the real world, you should prefer built-in functions to complex flow statements. The zip() function would do a great job for this use case:
See Unpacking Argument Lists for details on the asterisk in this line.
5.2. The del statement ¶
There is a way to remove an item from a list given its index instead of its value: the del statement. This differs from the pop() method which returns a value. The del statement can also be used to remove slices from a list or clear the entire list (which we did earlier by assignment of an empty list to the slice). For example:
del can also be used to delete entire variables:
Referencing the name a hereafter is an error (at least until another value is assigned to it). We’ll find other uses for del later.
5.3. Tuples and Sequences ¶
We saw that lists and strings have many common properties, such as indexing and slicing operations. They are two examples of sequence data types (see Sequence Types — list, tuple, range ). Since Python is an evolving language, other sequence data types may be added. There is also another standard sequence data type: the tuple .
A tuple consists of a number of values separated by commas, for instance:
As you see, on output tuples are always enclosed in parentheses, so that nested tuples are interpreted correctly; they may be input with or without surrounding parentheses, although often parentheses are necessary anyway (if the tuple is part of a larger expression). It is not possible to assign to the individual items of a tuple, however it is possible to create tuples which contain mutable objects, such as lists.
Though tuples may seem similar to lists, they are often used in different situations and for different purposes. Tuples are immutable , and usually contain a heterogeneous sequence of elements that are accessed via unpacking (see later in this section) or indexing (or even by attribute in the case of namedtuples ). Lists are mutable , and their elements are usually homogeneous and are accessed by iterating over the list.
A special problem is the construction of tuples containing 0 or 1 items: the syntax has some extra quirks to accommodate these. Empty tuples are constructed by an empty pair of parentheses; a tuple with one item is constructed by following a value with a comma (it is not sufficient to enclose a single value in parentheses). Ugly, but effective. For example:
The statement t = 12345, 54321, 'hello!' is an example of tuple packing : the values 12345 , 54321 and 'hello!' are packed together in a tuple. The reverse operation is also possible:
This is called, appropriately enough, sequence unpacking and works for any sequence on the right-hand side. Sequence unpacking requires that there are as many variables on the left side of the equals sign as there are elements in the sequence. Note that multiple assignment is really just a combination of tuple packing and sequence unpacking.
5.4. Sets ¶
Python also includes a data type for sets . A set is an unordered collection with no duplicate elements. Basic uses include membership testing and eliminating duplicate entries. Set objects also support mathematical operations like union, intersection, difference, and symmetric difference.
Curly braces or the set() function can be used to create sets. Note: to create an empty set you have to use set() , not {} ; the latter creates an empty dictionary, a data structure that we discuss in the next section.
Here is a brief demonstration:
Similarly to list comprehensions , set comprehensions are also supported:
5.5. Dictionaries ¶
Another useful data type built into Python is the dictionary (see Mapping Types — dict ). Dictionaries are sometimes found in other languages as “associative memories” or “associative arrays”. Unlike sequences, which are indexed by a range of numbers, dictionaries are indexed by keys , which can be any immutable type; strings and numbers can always be keys. Tuples can be used as keys if they contain only strings, numbers, or tuples; if a tuple contains any mutable object either directly or indirectly, it cannot be used as a key. You can’t use lists as keys, since lists can be modified in place using index assignments, slice assignments, or methods like append() and extend() .
It is best to think of a dictionary as a set of key: value pairs, with the requirement that the keys are unique (within one dictionary). A pair of braces creates an empty dictionary: {} . Placing a comma-separated list of key:value pairs within the braces adds initial key:value pairs to the dictionary; this is also the way dictionaries are written on output.
The main operations on a dictionary are storing a value with some key and extracting the value given the key. It is also possible to delete a key:value pair with del . If you store using a key that is already in use, the old value associated with that key is forgotten. It is an error to extract a value using a non-existent key.
Performing list(d) on a dictionary returns a list of all the keys used in the dictionary, in insertion order (if you want it sorted, just use sorted(d) instead). To check whether a single key is in the dictionary, use the in keyword.
Here is a small example using a dictionary:
The dict() constructor builds dictionaries directly from sequences of key-value pairs:
In addition, dict comprehensions can be used to create dictionaries from arbitrary key and value expressions:
When the keys are simple strings, it is sometimes easier to specify pairs using keyword arguments:
5.6. Looping Techniques ¶
When looping through dictionaries, the key and corresponding value can be retrieved at the same time using the items() method.
When looping through a sequence, the position index and corresponding value can be retrieved at the same time using the enumerate() function.
To loop over two or more sequences at the same time, the entries can be paired with the zip() function.
To loop over a sequence in reverse, first specify the sequence in a forward direction and then call the reversed() function.
To loop over a sequence in sorted order, use the sorted() function which returns a new sorted list while leaving the source unaltered.
It is sometimes tempting to change a list while you are looping over it; however, it is often simpler and safer to create a new list instead.
5.7. More on Conditions ¶
The conditions used in while and if statements can contain any operators, not just comparisons.
The comparison operators in and not in check whether a value occurs (does not occur) in a sequence. The operators is and is not compare whether two objects are really the same object; this only matters for mutable objects like lists. All comparison operators have the same priority, which is lower than that of all numerical operators.
Comparisons can be chained. For example, a < b == c tests whether a is less than b and moreover b equals c .
Comparisons may be combined using the Boolean operators and and or , and the outcome of a comparison (or of any other Boolean expression) may be negated with not . These have lower priorities than comparison operators; between them, not has the highest priority and or the lowest, so that A and not B or C is equivalent to (A and (not B)) or C . As always, parentheses can be used to express the desired composition.
The Boolean operators and and or are so-called short-circuit operators: their arguments are evaluated from left to right, and evaluation stops as soon as the outcome is determined. For example, if A and C are true but B is false, A and B and C does not evaluate the expression C . When used as a general value and not as a Boolean, the return value of a short-circuit operator is the last evaluated argument.
It is possible to assign the result of a comparison or other Boolean expression to a variable. For example,
Note that in Python, unlike C, assignment inside expressions must be done explicitly with the walrus operator := . This avoids a common class of problems encountered in C programs: typing = in an expression when == was intended.
5.8. Comparing Sequences and Other Types ¶
Sequence objects typically may be compared to other objects with the same sequence type. The comparison uses lexicographical ordering: first the first two items are compared, and if they differ this determines the outcome of the comparison; if they are equal, the next two items are compared, and so on, until either sequence is exhausted. If two items to be compared are themselves sequences of the same type, the lexicographical comparison is carried out recursively. If all items of two sequences compare equal, the sequences are considered equal. If one sequence is an initial sub-sequence of the other, the shorter sequence is the smaller (lesser) one. Lexicographical ordering for strings uses the Unicode code point number to order individual characters. Some examples of comparisons between sequences of the same type:
Note that comparing objects of different types with < or > is legal provided that the objects have appropriate comparison methods. For example, mixed numeric types are compared according to their numeric value, so 0 equals 0.0, etc. Otherwise, rather than providing an arbitrary ordering, the interpreter will raise a TypeError exception.
Other languages may return the mutated object, which allows method chaining, such as d->insert("a")->remove("b")->sort(); .
Table of Contents
- 5.1.1. Using Lists as Stacks
- 5.1.2. Using Lists as Queues
- 5.1.3. List Comprehensions
- 5.1.4. Nested List Comprehensions
- 5.2. The del statement
- 5.3. Tuples and Sequences
- 5.5. Dictionaries
- 5.6. Looping Techniques
- 5.7. More on Conditions
- 5.8. Comparing Sequences and Other Types
Previous topic
4. More Control Flow Tools
- Report a Bug
- Show Source
Search This Blog
Coursera python data structures assignment answers.
This course will introduce the core data structure of the Python programming ... And, the answer is only for hint . it is helpful for those student who haven't submit Assignment and who confused ... These five lines are the lines to do this particular assignment where we are ...
chapter 7 week 3 Assignment 7.1

Post a Comment
if you have any doubt , please write comment
Popular posts from this blog
Chapter 9 week 5 assignment 9.4.

chapter 10 week 6 Assignment 10.2

chapter 6 week 1 Assignment 6.5

Learn Algorithms and Data Structures in Python
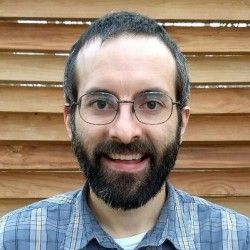
Algorithms and data structures are important for most programmers to understand.
We just released a course on the freeCodeCamp YouTube channel that is a beginner-friendly introduction to common data structures (linked lists, stacks, queues, graphs) and algorithms (search, sorting, recursion, dynamic programming) in Python.
This course will help you prepare for coding interviews and assessments. In this course, you will:
- Watch live hands-on coding-focused video tutorials
- Practice coding with cloud Jupyter notebooks
- Solve questions from real programming interviews
Aakash N S teaches this course. He is the co-founder and CEO of Jovian and has created many popular courses about machine learning and programming.
The course is broken up into a series of lessons, assignments, and projects. There are Jupyter Notebook files to go along with each section.
Here is what is covered in the course:
Lesson 1 - Binary Search, Linked Lists and Complexity
- Linear and Binary Search
- Complexity and Big O Notation
- Linked Lists using Python Classes
Assignment 1 - Binary Search Practice
- Understand and solve a problem systematically
- Implement linear search and analyze it
- Optimize the solution using binary search
Lesson 2 - Binary Search Trees, Traversals and Recursion
- Binary trees, traversals, and recursion
- Binary search trees & common operations
- Balanced binary trees and optimizations
Assignment 2 - Hash Tables and Python Dictionaries
- Hash tables from scratch in Python
- Handling collisions using linear probing
- Replicating Python dictionaries
Lesson 3 - Sorting Algorithms and Divide & Conquer
- Bubble sort and Insertion Sort
- Merge sort using Divide & Conquer
- Quicksort and average complexity
Assignment 3 - Divide and Conquer Practice
- Implement polynomial multiplication
- Optimize using divide and conquer
- Analyze time and space complexity
Lesson 4 - Recursion and Dynamic Programming
- Recursion and memoization
- Subsequence and knapsack problems
- Backtracking and pruning
Lesson 5 - Graph Algorithms (BFS, DFS & Shortest Paths)
- Graphs, trees, and adjacency lists
- Breadth-first and depth-first search
- Shortest paths and directed graphs
Project - Step-by-Step Solution to a Programming Problem
- Pick an interesting coding problem
- Solve the problem step-by-step
- Document and present the solution
Lesson 6 - Python Interview Questions, Tips & Advice
- Practice questions and solutions
- Tips for solving coding challenges
- Advice for cracking coding interviews
Watch the course below or on the freeCodeCamp.org YouTube channel (13-hour watch).
I'm a teacher and developer with freeCodeCamp.org. I run the freeCodeCamp.org YouTube channel.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started

- Top Courses

Python Data Structures
This course is part of Python for Everybody Specialization
Taught in English
Some content may not be translated

Instructor: Charles Russell Severance
Sponsored by Auroras Technological and Research Institute
1,028,675 already enrolled
(94,723 reviews)
What you'll learn
Explain the principles of data structures & how they are used
Create programs that are able to read and write data from files
Store data as key/value pairs using Python dictionaries
Accomplish multi-step tasks like sorting or looping using tuples
Skills you'll gain
- Computer Programming
- Critical Thinking
- Data Analysis
- Data Management
- Data Structures
- Problem Solving
- Python Programming
- Software Engineering
Details to know

Add to your LinkedIn profile
See how employees at top companies are mastering in-demand skills
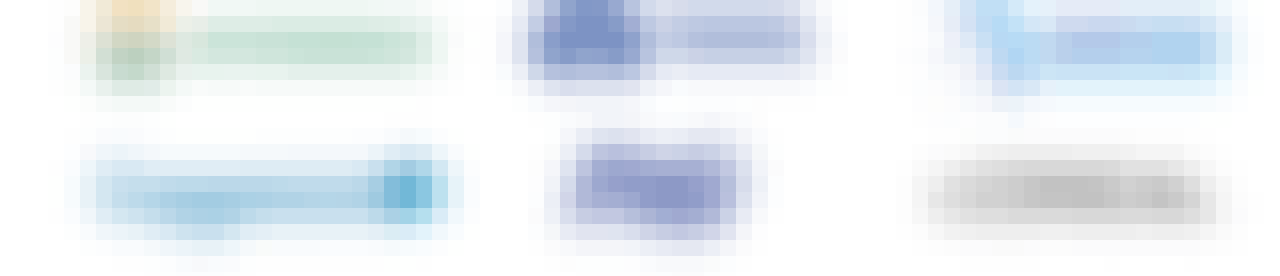
Build your subject-matter expertise
- Learn new concepts from industry experts
- Gain a foundational understanding of a subject or tool
- Develop job-relevant skills with hands-on projects
- Earn a shareable career certificate
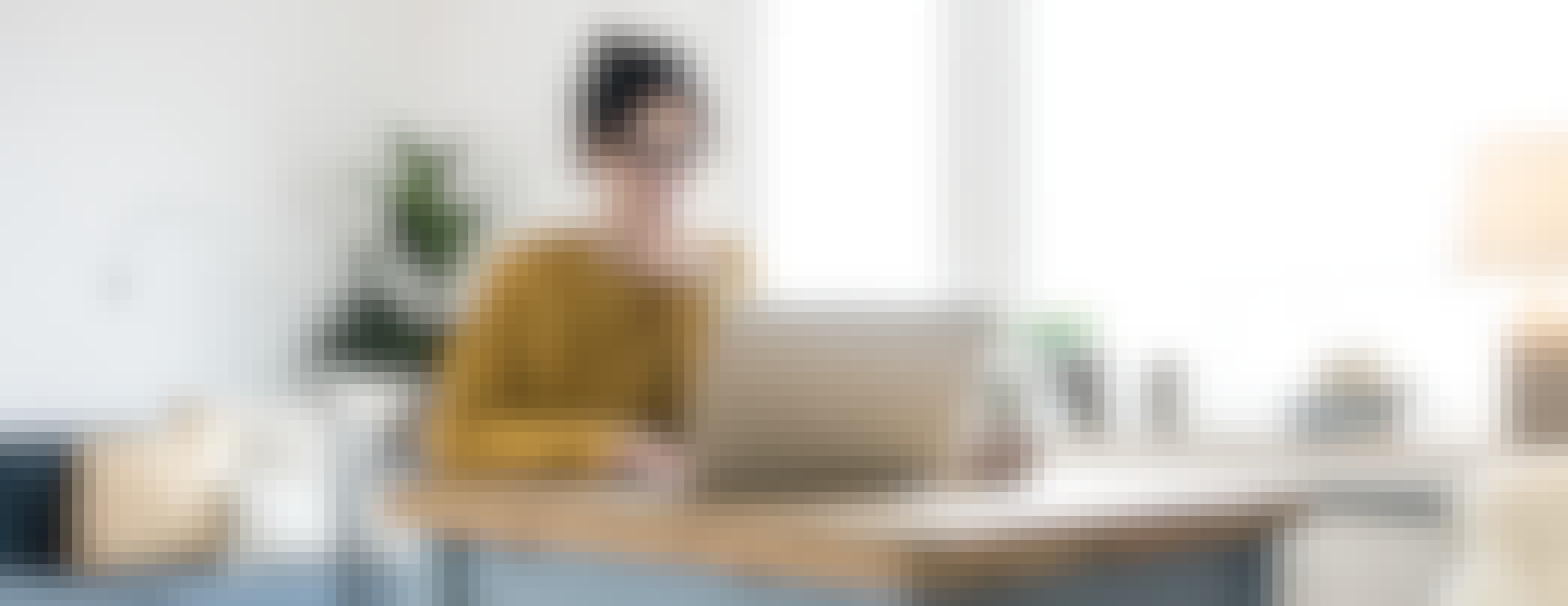
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV
Share it on social media and in your performance review
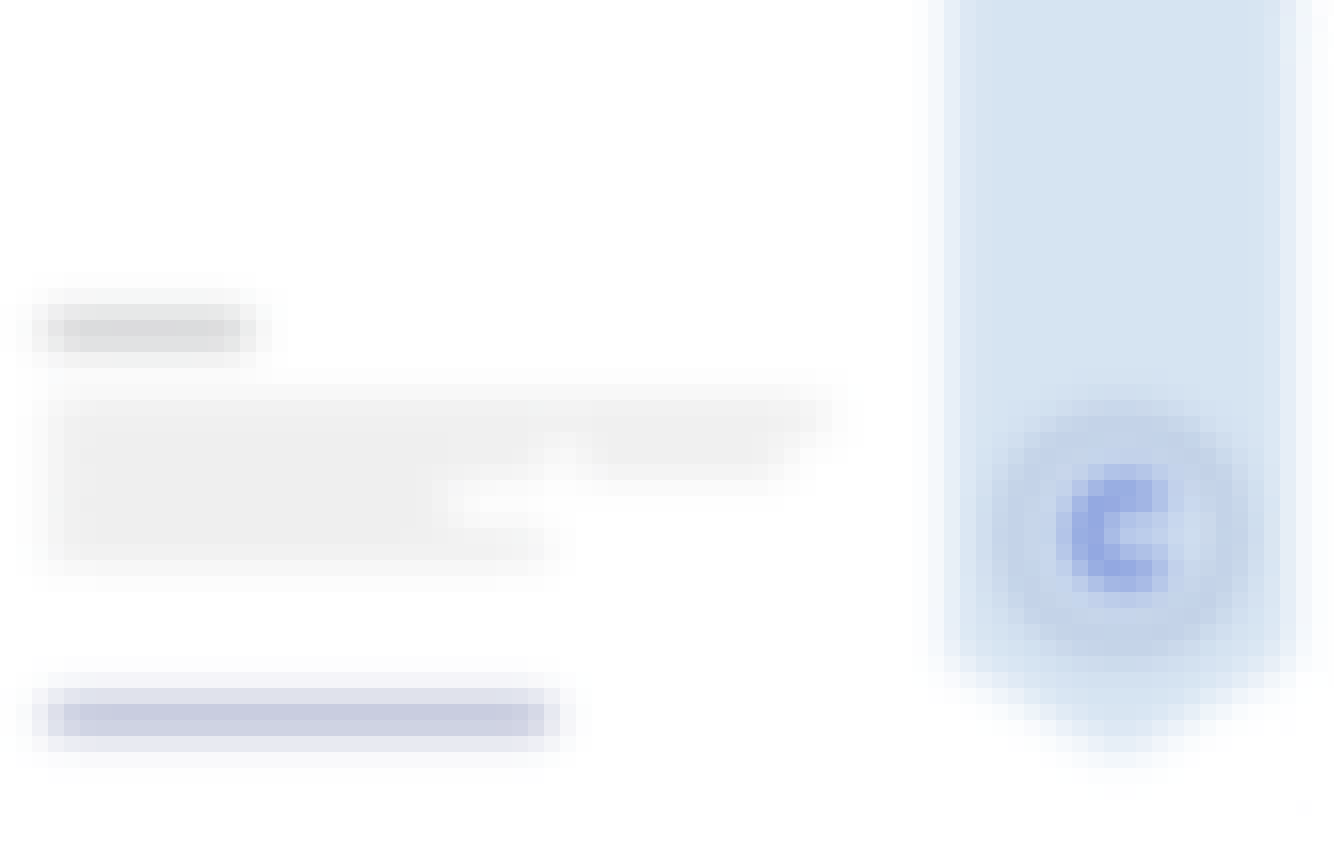
There are 7 modules in this course
This course will introduce the core data structures of the Python programming language. We will move past the basics of procedural programming and explore how we can use the Python built-in data structures such as lists, dictionaries, and tuples to perform increasingly complex data analysis. This course will cover Chapters 6-10 of the textbook “Python for Everybody”. This course covers Python 3.
Chapter Six: Strings
In this class, we pick up where we left off in the previous class, starting in Chapter 6 of the textbook and covering Strings and moving into data structures. The second week of this class is dedicated to getting Python installed if you want to actually run the applications on your desktop or laptop. If you choose not to install Python, you can just skip to the third week and get a head start.
What's included
7 videos 7 readings 1 quiz 1 app item
7 videos • Total 57 minutes
- Video Welcome - Dr. Chuck • 1 minute • Preview module
- 6.1 - Strings • 15 minutes
- 6.2 - Manipulating Strings • 17 minutes
- Worked Exercise: 6.5 • 8 minutes
- Bonus: Office Hours New York City • 2 minutes
- Bonus: Monash Museum of Computing History • 7 minutes
- Fun: The Textbook Authors Meet @PyCon • 3 minutes
7 readings • Total 70 minutes
- Reading: Welcome to Python Data Structures • 10 minutes
- Help Us Learn More About You! • 10 minutes
- Course Syllabus • 10 minutes
- Textbook • 10 minutes
- Submitting Assignments • 10 minutes
- Notice for Auditing Learners: Assignment Submission • 10 minutes
- Audio Versions of All Lectures • 10 minutes
1 quiz • Total 30 minutes
- Chapter 6 Quiz • 30 minutes
1 app item • Total 60 minutes
- Assignment 6.5 • 60 minutes
Unit: Installing and Using Python
In this module you will set things up so you can write Python programs. We do not require installation of Python for this class. You can write and test Python programs in the browser using the "Python Code Playground" in this lesson. Please read the "Using Python in this Class" material for details.
5 videos 2 readings 1 peer review 1 app item
5 videos • Total 26 minutes
- Demonstration: Using the Python Playground • 3 minutes • Preview module
- Windows 10: Installing Python and Writing A Program • 6 minutes
- Windows: Taking Screen Shots • 2 minutes
- Macintosh: Using Python and Writing A Program • 9 minutes
- Macintosh: Taking Screen Shots • 4 minutes
2 readings • Total 20 minutes
- Important Reading: Using Python in this Class • 10 minutes
- Notes on Choice of Text Editor • 10 minutes
1 peer review • Total 60 minutes
- Optional- Installing Python Screen Shots • 60 minutes
- Python Code Playground • 60 minutes
Chapter Seven: Files
Up to now, we have been working with data that is read from the user or data in constants. But real programs process much larger amounts of data by reading and writing files on the secondary storage on your computer. In this chapter we start to write our first programs that read, scan, and process real data.
5 videos 1 reading 1 quiz 2 app items
5 videos • Total 46 minutes
- 7.1 - Files • 8 minutes • Preview module
- 7.2 - Processing Files • 11 minutes
- Demonstration: Worked Exercise 7.1 • 9 minutes
- Bonus: Office Hours Barcelona • 1 minute
- Bonus: Gordon Bell - Building Blocks of Computing • 15 minutes
1 reading • Total 10 minutes
- Where is the 7.2 worked exercise? • 10 minutes
- Chapter 7 Quiz • 30 minutes
2 app items • Total 120 minutes
- Assignment 7.1 • 60 minutes
- Assignment 7.2 • 60 minutes
Chapter Eight: Lists
As we want to solve more complex problems in Python, we need more powerful variables. Up to now we have been using simple variables to store numbers or strings where we have a single value in a variable. Starting with lists we will store many values in a single variable using an indexing scheme to store, organize, and retrieve different values from within a single variable. We call these multi-valued variables "collections" or "data structures".
7 videos 1 quiz 2 app items
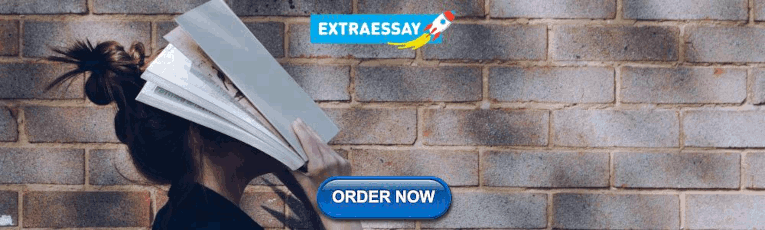
7 videos • Total 47 minutes
- 8.1 - Lists • 10 minutes • Preview module
- 8.2 - Manipulating Lists • 9 minutes
- 8.3 - Lists and Strings • 7 minutes
- Fun: Python Lists in Paris • 0 minutes
- Worked Exercise: Lists • 11 minutes
- Bonus: Office Hours - Chicago • 0 minutes
- Bonus: Rasmus Lerdorf - Inventing the PHP Language • 7 minutes
- Chapter 8 Quiz • 30 minutes
- Assignment 8.4 • 60 minutes
- Assignment 8.5 • 60 minutes
Chapter Nine: Dictionaries
The Python dictionary is one of its most powerful data structures. Instead of representing values in a linear list, dictionaries store data as key / value pairs. Using key / value pairs gives us a simple in-memory "database" in a single Python variable.
7 videos 1 quiz 1 app item
7 videos • Total 75 minutes
- 9.1 - Dictionaries • 9 minutes • Preview module
- 9.2 - Counting with Dictionaries • 8 minutes
- 9.3 - Dictionaries and Files • 13 minutes
- Worked Exercise: Dictionaries • 26 minutes
- Bonus: Office Hours - Amsterdam • 3 minutes
- Bonus: Brendan Eich - Inventing Javascript • 11 minutes
- Fun: Dr. Chuck Goes Motocross Racing • 2 minutes
- Chapter 9 Quiz • 30 minutes
- Assignment 9.4 • 60 minutes
Chapter Ten: Tuples
Tuples are our third and final basic Python data structure. Tuples are a simple version of lists. We often use tuples in conjunction with dictionaries to accomplish multi-step tasks like sorting or looping through all of the data in a dictionary.
6 videos 1 quiz 1 app item
6 videos • Total 51 minutes
- 10 - Tuples • 17 minutes • Preview module
- Worked Exercise: Tuples and Sorting • 12 minutes
- Bonus: Office Hours - Puebla, Mexico • 1 minute
- Bonus: John Resig - Inventing JQuery • 10 minutes
- Douglas Crockford: JavaScript Object Notation (JSON) • 7 minutes
- Fun: The Greatest Taco in the World • 2 minutes
- Chapter 10 Quiz • 30 minutes
- Assignment 10.2 • 60 minutes
To celebrate your making it to the halfway point in our Python for Everybody Specialization, we welcome you to attend our online graduation ceremony. It is not very long, and it features a Commencement speaker and very short commencement speech.
2 videos 2 readings
2 videos • Total 16 minutes
- Graduation Ceremony • 7 minutes • Preview module
- Dr.Chuck Wrap Up/What's Next • 8 minutes
- Please Rate this Course on Class-Central • 10 minutes
- Post-Course Survey • 10 minutes
Instructor ratings
We asked all learners to give feedback on our instructors based on the quality of their teaching style.

The mission of the University of Michigan is to serve the people of Michigan and the world through preeminence in creating, communicating, preserving and applying knowledge, art, and academic values, and in developing leaders and citizens who will challenge the present and enrich the future.
Why people choose Coursera for their career

Learner reviews
Showing 3 of 94723
94,723 reviews
Reviewed on Oct 8, 2017
assignment 9.4 auto grader not working .
LTI unable to launch. error message: This tool should be launched from a learning system using LTI. i am using chrome on mac book air 2 and python 3.6
Reviewed on Aug 18, 2017
i have learned what exact python is now on this stage i can program in python and i recommend everyone want to study about the python to take this course and experienced and really thanks to Dr. CHUCK
Reviewed on Jun 19, 2020
Great course for pyhton. Loved this course and enjoyed it. Thanks to Dr.Chuck. If anyone who want to take a course which is well explained and fun for python learning, then Hey!!! this is your course.
Recommended if you're interested in Computer Science
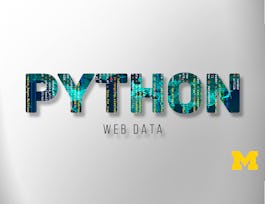
University of Michigan
Using Python to Access Web Data
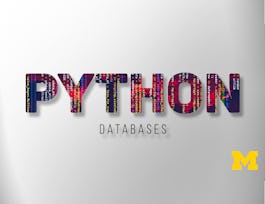
Using Databases with Python
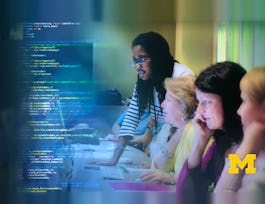
Capstone: Retrieving, Processing, and Visualizing Data with Python
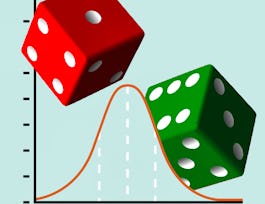
University of Zurich
An Intuitive Introduction to Probability

Open new doors with Coursera Plus
Unlimited access to 7,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Hash Map in Python
- Learn DSA with Python | Python Data Structures and Algorithms
- Insert and delete at the beginning in Doubly Linked List in Python
- Stack in Python
- Queue in Python
- Python Linked List
- Stack and Queues in Python
- Heapq with custom predicate in Python
- Heap queue (or heapq) in Python
- Linked list using dstructure library in Python
- Tree Traversal Techniques in Python
- What makes Python a slow language ?
- SymPy | Subset.rank_binary() in Python
- PyQt5 - Setting skin to editable combo box
- numpy.amin() in Python
- SymPy | Subset.prev_binary() in Python
- Python | Decimal compare_total() method
- Python | Decimal compare_total_mag() method
- numpy.amax() in Python
Python Data Structures
Data Structures are a way of organizing data so that it can be accessed more efficiently depending upon the situation. Data Structures are fundamentals of any programming language around which a program is built. Python helps to learn the fundamental of these data structures in a simpler way as compared to other programming languages.
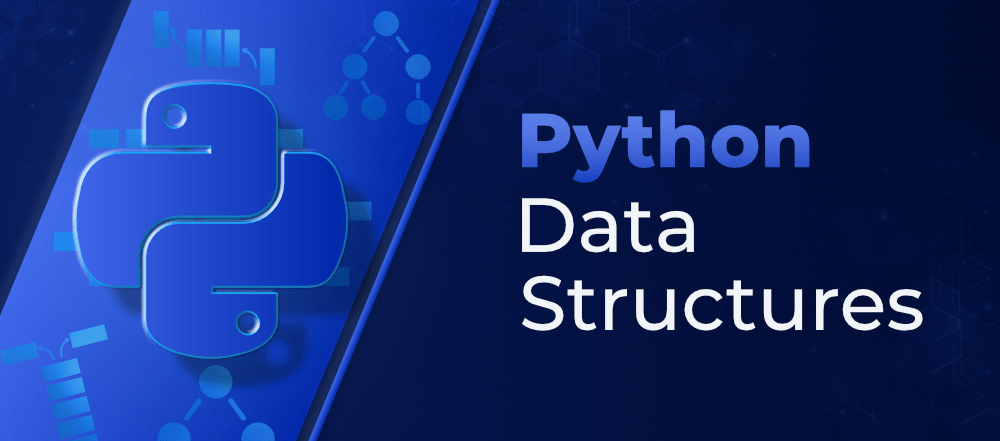
In this article, we will discuss the Data Structures in the Python Programming Language and how they are related to some specific Python Data Types . We will discuss all the in-built data structures like list tuples, dictionaries, etc. as well as some advanced data structures like trees, graphs, etc.
Python Lists are just like the arrays, declared in other languages which is an ordered collection of data. It is very flexible as the items in a list do not need to be of the same type.
The implementation of Python List is similar to Vectors in C++ or ArrayList in JAVA. The costly operation is inserting or deleting the element from the beginning of the List as all the elements are needed to be shifted. Insertion and deletion at the end of the list can also become costly in the case where the preallocated memory becomes full.
We can create a list in python as shown below.
Example: Creating Python List
List elements can be accessed by the assigned index. In python starting index of the list, sequence is 0 and the ending index is (if N elements are there) N-1.
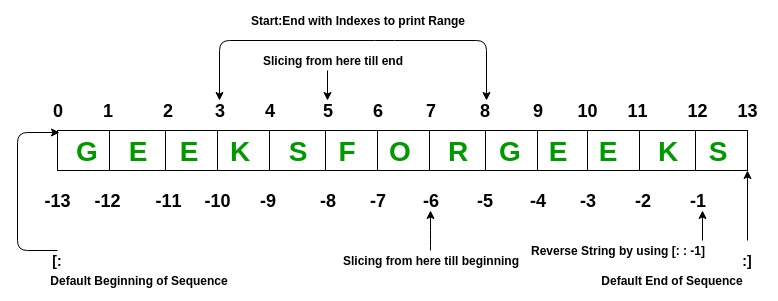
Example: Python List Operations
Python dictionary is like hash tables in any other language with the time complexity of O(1). It is an unordered collection of data values, used to store data values like a map, which, unlike other Data Types that hold only a single value as an element, Dictionary holds the key:value pair. Key-value is provided in the dictionary to make it more optimized.
Indexing of Python Dictionary is done with the help of keys. These are of any hashable type i.e. an object whose can never change like strings, numbers, tuples, etc. We can create a dictionary by using curly braces ({}) or dictionary comprehension .
Example: Python Dictionary Operations
Python Tuple is a collection of Python objects much like a list but Tuples are immutable in nature i.e. the elements in the tuple cannot be added or removed once created. Just like a List, a Tuple can also contain elements of various types.
In Python, tuples are created by placing a sequence of values separated by ‘comma’ with or without the use of parentheses for grouping of the data sequence.
Note: Tuples can also be created with a single element, but it is a bit tricky. Having one element in the parentheses is not sufficient, there must be a trailing ‘comma’ to make it a tuple.
Example: Python Tuple Operations
Python Set is an unordered collection of data that is mutable and does not allow any duplicate element. Sets are basically used to include membership testing and eliminating duplicate entries. The data structure used in this is Hashing, a popular technique to perform insertion, deletion, and traversal in O(1) on average.
If Multiple values are present at the same index position, then the value is appended to that index position, to form a Linked List. In, CPython Sets are implemented using a dictionary with dummy variables, where key beings the members set with greater optimizations to the time complexity.
Set Implementation:
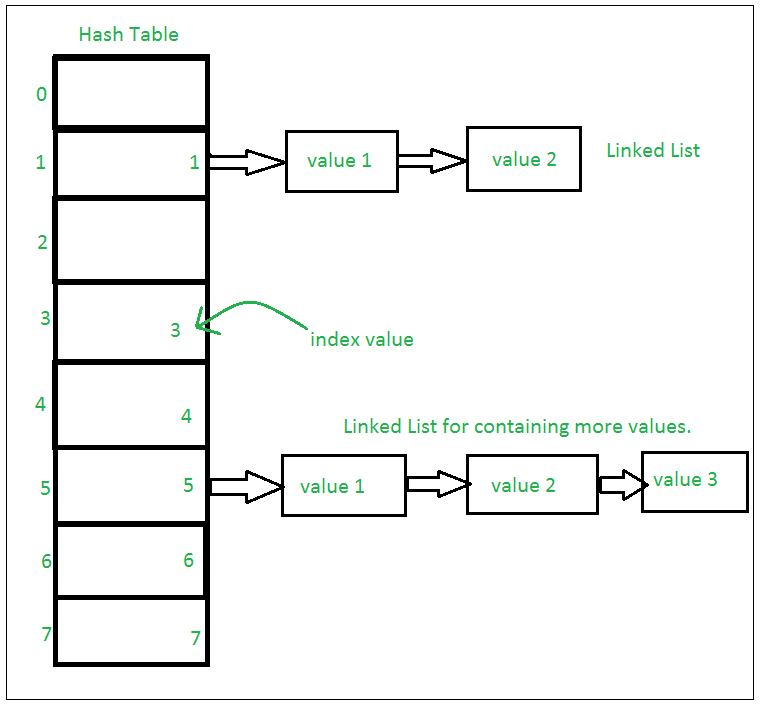
Sets with Numerous operations on a single HashTable:
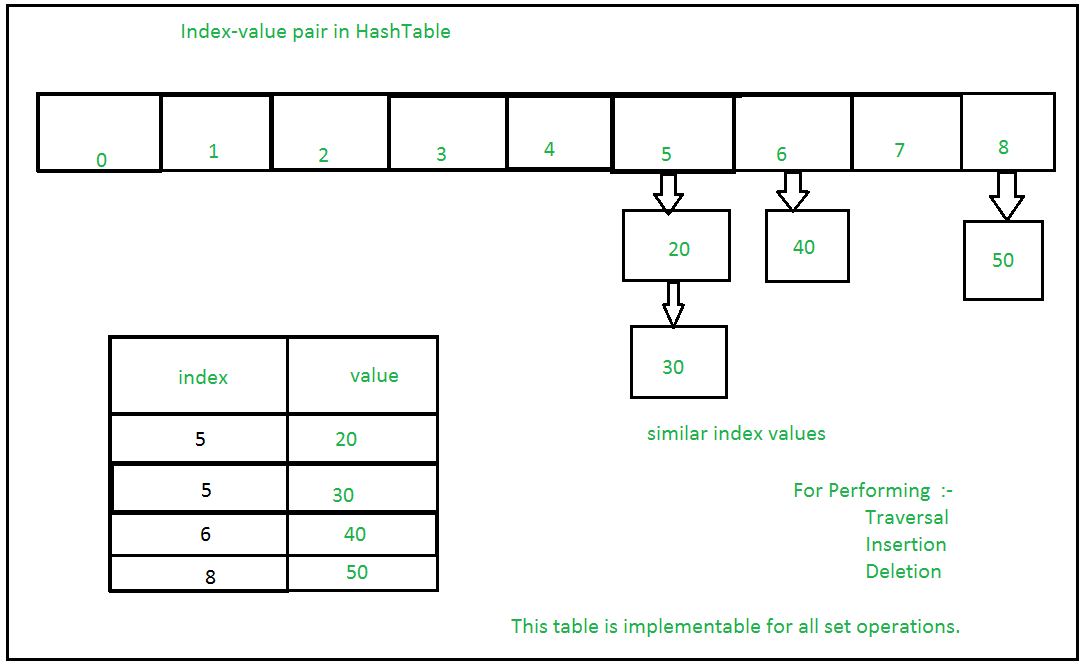
Example: Python Set Operations
Frozen sets.
Frozen sets in Python are immutable objects that only support methods and operators that produce a result without affecting the frozen set or sets to which they are applied. While elements of a set can be modified at any time, elements of the frozen set remain the same after creation.
If no parameters are passed, it returns an empty frozenset.
Python Strings are arrays of bytes representing Unicode characters. In simpler terms, a string is an immutable array of characters. Python does not have a character data type, a single character is simply a string with a length of 1.
Note: As strings are immutable, modifying a string will result in creating a new copy.
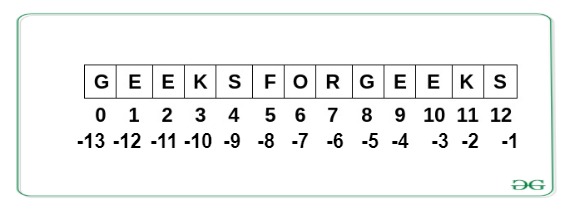
Example: Python Strings Operations
Python Bytearray gives a mutable sequence of integers in the range 0 <= x < 256.
Example: Python Bytearray Operations
Till now we have studied all the data structures that come built-in into core Python. Now let dive more deep into Python and see the collections module that provides some containers that are useful in many cases and provide more features than the above-defined functions.
Collections Module
Python collection module was introduced to improve the functionality of the built-in datatypes. It provides various containers let’s see each one of them in detail.
A counter is a sub-class of the dictionary. It is used to keep the count of the elements in an iterable in the form of an unordered dictionary where the key represents the element in the iterable and value represents the count of that element in the iterable. This is equivalent to a bag or multiset of other languages.
Example: Python Counter Operations
Ordereddict.
An OrderedDict is also a sub-class of dictionary but unlike a dictionary, it remembers the order in which the keys were inserted.
Example: Python OrderedDict Operations
Defaultdict.
DefaultDict is used to provide some default values for the key that does not exist and never raises a KeyError. Its objects can be initialized using DefaultDict() method by passing the data type as an argument.
Note: default_factory is a function that provides the default value for the dictionary created. If this parameter is absent then the KeyError is raised.
Example: Python DefaultDict Operations
A ChainMap encapsulates many dictionaries into a single unit and returns a list of dictionaries. When a key is needed to be found then all the dictionaries are searched one by one until the key is found.
Example: Python ChainMap Operations
A NamedTuple returns a tuple object with names for each position which the ordinary tuples lack. For example, consider a tuple names student where the first element represents fname, second represents lname and the third element represents the DOB. Suppose for calling fname instead of remembering the index position you can actually call the element by using the fname argument, then it will be really easy for accessing tuples element. This functionality is provided by the NamedTuple.
Example: Python NamedTuple Operations
Deque (Doubly Ended Queue) is the optimized list for quicker append and pop operations from both sides of the container. It provides O(1) time complexity for append and pop operations as compared to the list with O(n) time complexity.
Python Deque is implemented using doubly linked lists therefore the performance for randomly accessing the elements is O(n).
Example: Python Deque Operations
UserDict is a dictionary-like container that acts as a wrapper around the dictionary objects. This container is used when someone wants to create their own dictionary with some modified or new functionality.
Example: Python UserDict
UserList is a list-like container that acts as a wrapper around the list objects. This is useful when someone wants to create their own list with some modified or additional functionality.
Examples: Python UserList
UserString is a string-like container and just like UserDict and UserList, it acts as a wrapper around string objects. It is used when someone wants to create their own strings with some modified or additional functionality.
Example: Python UserString
Now after studying all the data structures let’s see some advanced data structures such as stack, queue, graph, linked list, etc. that can be used in Python Language.
Linked List
A linked list is a linear data structure, in which the elements are not stored at contiguous memory locations. The elements in a linked list are linked using pointers as shown in the below image:
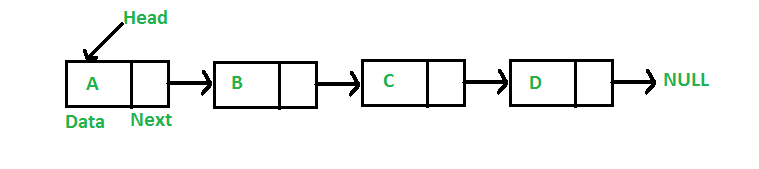
A linked list is represented by a pointer to the first node of the linked list. The first node is called the head. If the linked list is empty, then the value of the head is NULL. Each node in a list consists of at least two parts:
- Pointer (Or Reference) to the next node
Example: Defining Linked List in Python
Let us create a simple linked list with 3 nodes.
Linked List Traversal
In the previous program, we have created a simple linked list with three nodes. Let us traverse the created list and print the data of each node. For traversal, let us write a general-purpose function printList() that prints any given list.
A stack is a linear data structure that stores items in a Last-In/First-Out (LIFO) or First-In/Last-Out (FILO) manner. In stack, a new element is added at one end and an element is removed from that end only. The insert and delete operations are often called push and pop.
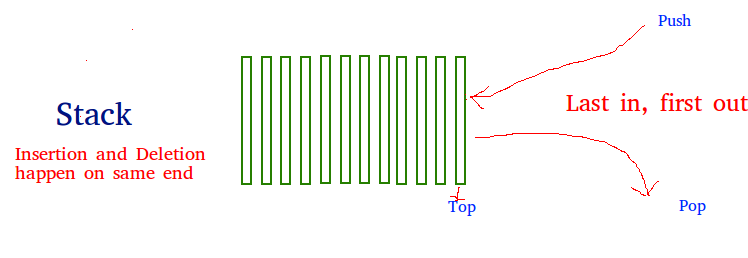
The functions associated with stack are:
- empty() – Returns whether the stack is empty – Time Complexity: O(1)
- size() – Returns the size of the stack – Time Complexity: O(1)
- top() – Returns a reference to the topmost element of the stack – Time Complexity: O(1)
- push(a) – Inserts the element ‘a’ at the top of the stack – Time Complexity: O(1)
- pop() – Deletes the topmost element of the stack – Time Complexity: O(1)
Python Stack Implementation
Stack in Python can be implemented using the following ways:
- Collections.deque
- queue.LifoQueue
Implementation using List
Python’s built-in data structure list can be used as a stack. Instead of push(), append() is used to add elements to the top of the stack while pop() removes the element in LIFO order.
Implementation using collections.deque:
Python stack can be implemented using the deque class from the collections module. Deque is preferred over the list in the cases where we need quicker append and pop operations from both the ends of the container, as deque provides an O(1) time complexity for append and pop operations as compared to list which provides O(n) time complexity.
Implementation using queue module
The queue module also has a LIFO Queue, which is basically a Stack. Data is inserted into Queue using the put() function and get() takes data out from the Queue.
As a stack, the queue is a linear data structure that stores items in a First In First Out (FIFO) manner. With a queue, the least recently added item is removed first. A good example of the queue is any queue of consumers for a resource where the consumer that came first is served first.
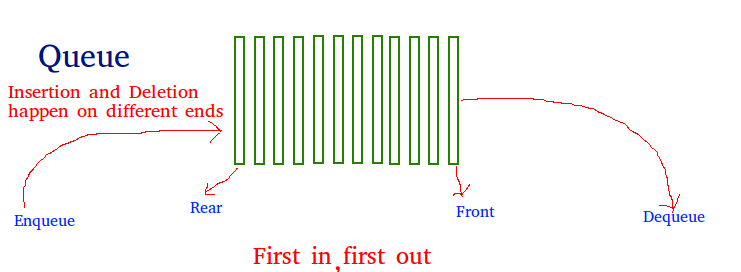
Operations associated with queue are:
- Enqueue: Adds an item to the queue. If the queue is full, then it is said to be an Overflow condition – Time Complexity: O(1)
- Dequeue: Removes an item from the queue. The items are popped in the same order in which they are pushed. If the queue is empty, then it is said to be an Underflow condition – Time Complexity: O(1)
- Front: Get the front item from queue – Time Complexity: O(1)
- Rear: Get the last item from queue – Time Complexity: O(1)
Python queue Implementation
Queue in Python can be implemented in the following ways:
- collections.deque
- queue.Queue
Implementation using list
Instead of enqueue() and dequeue(), append() and pop() function is used.
Implementation using collections.deque
Deque is preferred over the list in the cases where we need quicker append and pop operations from both the ends of the container, as deque provides an O(1) time complexity for append and pop operations as compared to list which provides O(n) time complexity.
Implementation using the queue.Queue
queue.Queue(maxsize) initializes a variable to a maximum size of maxsize. A maxsize of zero ‘0’ means an infinite queue. This Queue follows the FIFO rule.
Priority Queue
Priority Queues are abstract data structures where each data/value in the queue has a certain priority. For example, In airlines, baggage with the title “Business” or “First-class” arrives earlier than the rest. Priority Queue is an extension of the queue with the following properties.
- An element with high priority is dequeued before an element with low priority.
- If two elements have the same priority, they are served according to their order in the queue.
Heap queue (or heapq)
heapq module in Python provides the heap data structure that is mainly used to represent a priority queue. The property of this data structure in Python is that each time the smallest heap element is popped(min-heap). Whenever elements are pushed or popped, heap structure is maintained. The heap[0] element also returns the smallest element each time.
It supports the extraction and insertion of the smallest element in the O(log n) times.
Binary Tree
A tree is a hierarchical data structure that looks like the below figure –
The topmost node of the tree is called the root whereas the bottommost nodes or the nodes with no children are called the leaf nodes. The nodes that are directly under a node are called its children and the nodes that are directly above something are called its parent.
A binary tree is a tree whose elements can have almost two children. Since each element in a binary tree can have only 2 children, we typically name them the left and right children. A Binary Tree node contains the following parts.
- Pointer to left child
- Pointer to the right child
Example: Defining Node Class
Now let’s create a tree with 4 nodes in Python. Let’s assume the tree structure looks like below –
Example: Adding data to the tree
Tree traversal.
Trees can be traversed in different ways. Following are the generally used ways for traversing trees. Let us consider the below tree –
Depth First Traversals:
- Inorder (Left, Root, Right) : 4 2 5 1 3
- Preorder (Root, Left, Right) : 1 2 4 5 3
- Postorder (Left, Right, Root) : 4 5 2 3 1
Algorithm Inorder(tree)
- Traverse the left subtree, i.e., call Inorder(left-subtree)
- Visit the root.
- Traverse the right subtree, i.e., call Inorder(right-subtree)
Algorithm Preorder(tree)
- Traverse the left subtree, i.e., call Preorder(left-subtree)
- Traverse the right subtree, i.e., call Preorder(right-subtree)
Algorithm Postorder(tree)
- Traverse the left subtree, i.e., call Postorder(left-subtree)
- Traverse the right subtree, i.e., call Postorder(right-subtree)
Time Complexity – O(n)
Breadth-First or Level Order Traversal
Level order traversal of a tree is breadth-first traversal for the tree. The level order traversal of the above tree is 1 2 3 4 5.
For each node, first, the node is visited and then its child nodes are put in a FIFO queue. Below is the algorithm for the same –
- Create an empty queue q
- temp_node = root /*start from root*/
- print temp_node->data.
- Enqueue temp_node’s children (first left then right children) to q
- Dequeue a node from q
Time Complexity: O(n)
A graph is a nonlinear data structure consisting of nodes and edges. The nodes are sometimes also referred to as vertices and the edges are lines or arcs that connect any two nodes in the graph. More formally a Graph can be defined as a Graph consisting of a finite set of vertices(or nodes) and a set of edges that connect a pair of nodes.
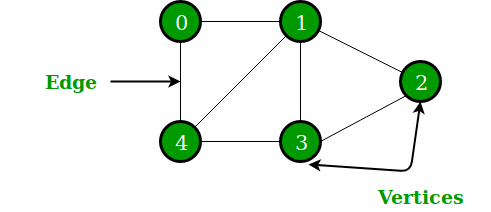
In the above Graph, the set of vertices V = {0,1,2,3,4} and the set of edges E = {01, 12, 23, 34, 04, 14, 13}.
The following two are the most commonly used representations of a graph.
Adjacency Matrix
Adjacency list.
Adjacency Matrix is a 2D array of size V x V where V is the number of vertices in a graph. Let the 2D array be adj[][], a slot adj[i][j] = 1 indicates that there is an edge from vertex i to vertex j. The adjacency matrix for an undirected graph is always symmetric. Adjacency Matrix is also used to represent weighted graphs. If adj[i][j] = w, then there is an edge from vertex i to vertex j with weight w.
Vertices of Graph [‘a’, ‘b’, ‘c’, ‘d’, ‘e’, ‘f’] Edges of Graph [(‘a’, ‘c’, 20), (‘a’, ‘e’, 10), (‘b’, ‘c’, 30), (‘b’, ‘e’, 40), (‘c’, ‘a’, 20), (‘c’, ‘b’, 30), (‘d’, ‘e’, 50), (‘e’, ‘a’, 10), (‘e’, ‘b’, 40), (‘e’, ‘d’, 50), (‘e’, ‘f’, 60), (‘f’, ‘e’, 60)] Adjacency Matrix of Graph [[-1, -1, 20, -1, 10, -1], [-1, -1, 30, -1, 40, -1], [20, 30, -1, -1, -1, -1], [-1, -1, -1, -1, 50, -1], [10, 40, -1, 50, -1, 60], [-1, -1, -1, -1, 60, -1]]
An array of lists is used. The size of the array is equal to the number of vertices. Let the array be an array[]. An entry array[i] represents the list of vertices adjacent to the ith vertex. This representation can also be used to represent a weighted graph. The weights of edges can be represented as lists of pairs. Following is the adjacency list representation of the above graph.

Graph Traversal
Breadth-First Search or BFS
Breadth-First Traversal for a graph is similar to Breadth-First Traversal of a tree. The only catch here is, unlike trees, graphs may contain cycles, so we may come to the same node again. To avoid processing a node more than once, we use a boolean visited array. For simplicity, it is assumed that all vertices are reachable from the starting vertex.
For example, in the following graph, we start traversal from vertex 2. When we come to vertex 0, we look for all adjacent vertices of it. 2 is also an adjacent vertex of 0. If we don’t mark visited vertices, then 2 will be processed again and it will become a non-terminating process. A Breadth-First Traversal of the following graph is 2, 0, 3, 1.
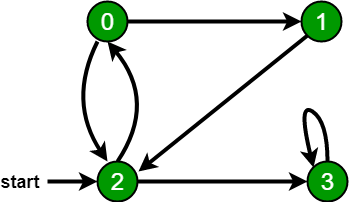
Time Complexity: O(V+E) where V is the number of vertices in the graph and E is the number of edges in the graph.
Depth First Search or DFS
Depth First Traversal for a graph is similar to Depth First Traversal of a tree. The only catch here is, unlike trees, graphs may contain cycles, a node may be visited twice. To avoid processing a node more than once, use a boolean visited array.
- Create a recursive function that takes the index of the node and a visited array.
- Mark the current node as visited and print the node.
- Traverse all the adjacent and unmarked nodes and call the recursive function with the index of the adjacent node.
Time complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Please Login to comment...
Similar reads.
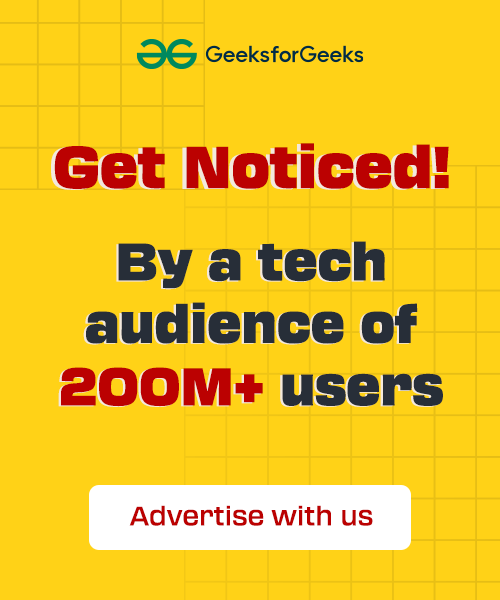
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

assignment 7.1 python data structures
- python repetition structures
- mac big sur and python3 problems
- python 0-1 kanpsack
- logical operators python
- Python For Data Science And Machine Learning Bootcamp
- python practice problems
- class item assignment python
- assignment 4.6 python for everybody
- assignment 6.5 python for everybody
- cors assignment 4.6 python
- python data structures
- advanced python course
- python fundamentals assignment solutions pdf
- python data structures 9.4
- python beginner practice problems
- learn python the hard way pdf
- data structures and algorithms in python
- python for data science
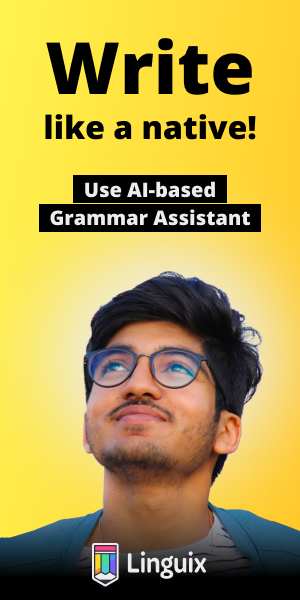
New to Communities?
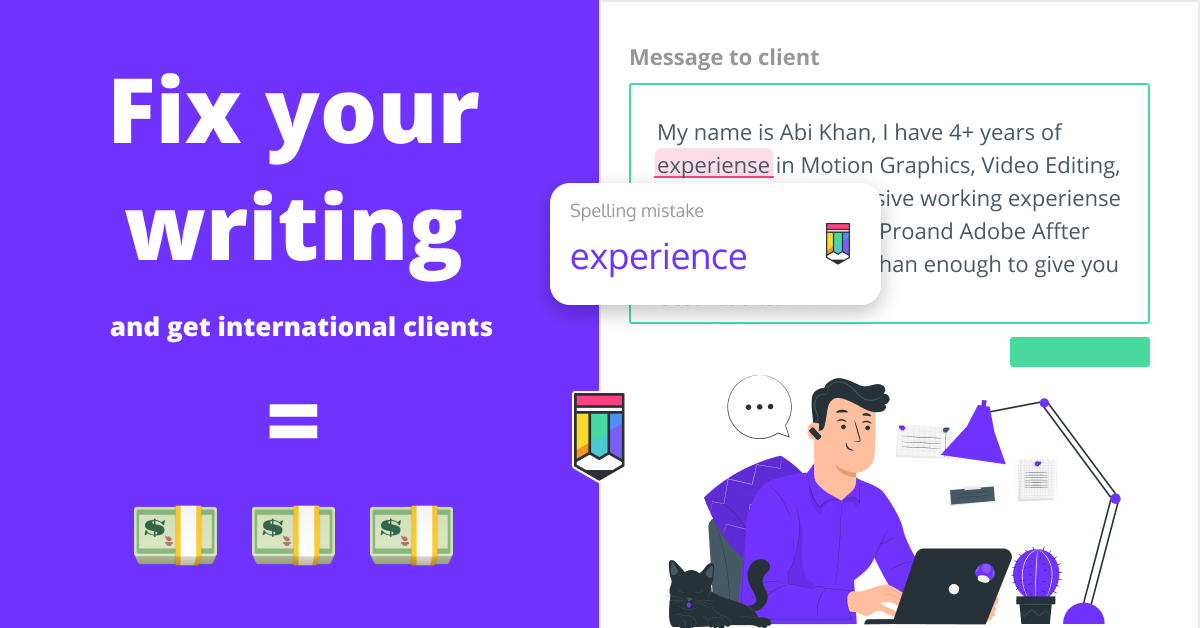
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
All my solved ASSIGNMENTS & QUIZZES in Python Data Structure course on COURSERA using Python 3.
shreyansh225/Coursera-Python-Data-Structures-University-of-Michigan-
Folders and files, repository files navigation, coursera-python-data-structures-university-of-michigan-.
- Python 100.0%
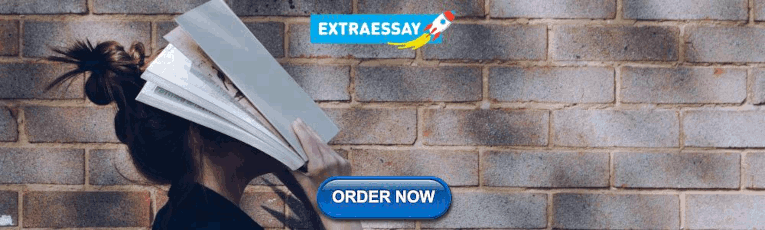
IMAGES
VIDEO
COMMENTS
My solutions to all quizzes and assignments for Python Data Structures on coursera by the University of Michigan - Coursera-Python-Data-Structures/Week 3 Assignment 7.1-solution.py at master · pavanayila/Coursera-Python-Data-Structures
Contribute to riya2905/Coursera-PY4E-Python-Data-Structures development by creating an account on GitHub.
Python Data Structures Assignment 7.1 Solution [Coursera] | Assignment 7.1 Python Data StructuresCoursera: Programming For Everybody Assignment 7.1 program s...
There are 7 modules in this course. This course will introduce the core data structures of the Python programming language. We will move past the basics of procedural programming and explore how we can use the Python built-in data structures such as lists, dictionaries, and tuples to perform increasingly complex data analysis.
In Python, dictionaries (or dicts for short) are a central data structure. Dicts store an arbitrary number of objects, each identified by a unique dictionary key. Dictionaries are also often called maps, hashmaps, lookup tables, or associative arrays. They allow for the efficient lookup, insertion, and deletion of any object associated with a ...
# Coursera :- #python data structures# Python👇👇program run code 👇👇https://1drv.ms/w/s!AspSlroH33QifAdPaMMP5CG9bwECHAPTER :- PYTHON DATA STRUCTURESASSIGN...
Python Data Structures Assignment 7.1 Solution [Coursera] | Assignment 7.1 Python Data StructuresPython Data Structures Assignment 7.1 Solution [Coursera] | ...
Data Structures in Python. In these tutorials, you'll learn about built-in data structures in Python. Python comes with a variety of versatile data structures in the core language, as well as in its large standard library. You'll also learn how you can implement abstract data structures, such as stacks, queues, hash tables, etc. in Python.
wk9 - quiz.py. python-for-everybody. /. wk7 - assignment 7.1.py. Cannot retrieve latest commit at this time. History. Code. 12 lines (9 loc) · 448 Bytes. 7.1 Write a program that prompts for a file name, then opens that file and reads through the file, and print the contents of the file in upper case.
Data Structures¶ This chapter describes some things you've learned about already in more detail, and adds some new things as well. 5.1. More on Lists¶ The list data type has some more methods. Here are all of the methods of list objects: list.append (x) Add an item to the end of the list. Equivalent to a[len(a):] = [x]. list.extend (iterable)
This tutorial is a beginner-friendly guide for learning data structures and algorithms using Python. In this article, we will discuss the in-built data structures such as lists, tuples, dictionaries, etc, and some user-defined data structures such as linked lists, trees, graphs, etc, and traversal as well as searching and sorting algorithms with the help of good and well-explained examples and ...
Data structure is a fundamental concept in programming, which is required for easily storing and retrieving data. Python has four main data structures split between mutable (lists, dictionaries, and sets) and immutable (tuples) types. Lists are useful to hold a heterogeneous collection of related objects.
7.1 Write a program that prompts for a file name, then opens that file and reads through the file, and print the contents of the file in upper case. Use the file words.txt to produce the output below.
Get started. Algorithms and data structures are important for most programmers to understand. We just released a course on the freeCodeCamp YouTube channel that is a beginner-friendly introduction to common data structures (linked lists, stacks, queues, graphs) and algorithms (search, sorting, recursion, dynamic programming) in Python.
There are 7 modules in this course. This course will introduce the core data structures of the Python programming language. We will move past the basics of procedural programming and explore how we can use the Python built-in data structures such as lists, dictionaries, and tuples to perform increasingly complex data analysis.
About Press Copyright Contact us Creators Advertise Developers Terms Privacy Policy & Safety How YouTube works Test new features NFL Sunday Ticket Press Copyright ...
Contribute to Ritik2703/Coursera---Python-Data-Structure-Answers development by creating an account on GitHub.
Tuple. Python Tuple is a collection of Python objects much like a list but Tuples are immutable in nature i.e. the elements in the tuple cannot be added or removed once created. Just like a List, a Tuple can also contain elements of various types. In Python, tuples are created by placing a sequence of values separated by 'comma' with or without the use of parentheses for grouping of the ...
This repository contains my solutions to the Data Structures and Algorithms assignments offered by the University of California, San Diego (UCSD) and the National Research University Higher School of Economics (HSE) on Coursera. All of the problems from courses 1 through 6 have been solved using Python. These solutions are intended to serve as a reference for those working on these assignments ...
..Welcome to #mythoughtsPYTHON DATA STRUCTURES Assignment 7.1 [coursera] | Assignment 7.1 in PYHTON DATA STRUCTURES |Assignment 7.1 in PY4E |
Get code examples like"assignment 7.1 python data structures". Write more code and save time using our ready-made code examples.
All my solved ASSIGNMENTS & QUIZZES in Python Data Structure course on COURSERA using Python 3. Topics python coursera python3 coursera-assignment coursera-python python-data-structures coursera-solutions
Text File of All 7 Assignment Coding :- https://ko-fi.com/s/bf5f9906dfFiles For Indian Payment Users:- Https://imojo.in/PythonC2Python Data Structures Course...