- DSA Tutorial
- Data Structures
- Linked List
- Dynamic Programming
- Binary Tree
- Binary Search Tree
- Divide & Conquer
- Mathematical
- Backtracking
- Branch and Bound
- Pattern Searching
- Advanced Data Structures
- Generic Linked List in C
- Memory efficient doubly linked list
- XOR Linked List - A Memory Efficient Doubly Linked List | Set 1
- XOR Linked List – A Memory Efficient Doubly Linked List | Set 2
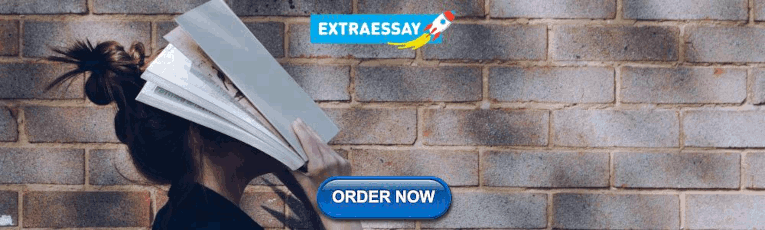
Skip List | Set 1 (Introduction)
- Self Organizing List | Set 1 (Introduction)
- Unrolled Linked List | Set 1 (Introduction)
- Searching in Splay Tree
- Insertion in Splay Tree
- Trie Data Structure Tutorial
- Segment Tree
Scape Goat Tree and Treap
- ScapeGoat Tree | Set 1 (Introduction and Insertion)
- Treap (A Randomized Binary Search Tree)
- Implementation of Search, Insert and Delete in Treap
INTRODUCTION:
A skip list is a data structure that allows for efficient search, insertion and deletion of elements in a sorted list. It is a probabilistic data structure, meaning that its average time complexity is determined through a probabilistic analysis.
In a skip list, elements are organized in layers, with each layer having a smaller number of elements than the one below it. The bottom layer is a regular linked list, while the layers above it contain “skipping” links that allow for fast navigation to elements that are far apart in the bottom layer. The idea behind this is to allow for quick traversal to the desired element, reducing the average number of steps needed to reach it.
Skip lists are implemented using a technique called “coin flipping.” In this technique, a random number is generated for each insertion to determine the number of layers the new element will occupy. This means that, on average, each element will be in log(n) layers, where n is the number of elements in the bottom layer.
Skip lists have an average time complexity of O(log n) for search, insertion and deletion, which is similar to that of balanced trees, such as AVL trees and red-black trees, but with the advantage of simpler implementation and lower overhead.
In summary, skip lists provide a simple and efficient alternative to balanced trees for certain use cases, particularly when the average number of elements in the list is large.

Advantages of Skip List:
- The skip list is solid and trustworthy.
- To add a new node to it, it will be inserted extremely quickly.
- Easy to implement compared to the hash table and binary search tree
- The number of nodes in the skip list increases, and the possibility of the worst-case decreases
- Requires only ?(logn) time in the average case for all operations.
- Finding a node in the list is relatively straightforward.
Disadvantages of Skip List:
- It needs a greater amount of memory than the balanced tree.
- Reverse search is not permitted.
- Searching is slower than a linked list
- Skip lists are not cache-friendly because they don’t optimize the locality of reference
Can we do better? The time complexity of skip lists can be reduced further by adding more layers. The time complexity of the search, the insert, and delete can become O(Logn) in an average cases with O(n) extra space. We will soon be publishing more posts on Skip Lists. References MIT Video Lecture on Skip Lists http://en.wikipedia.org/wiki/Skip_list
Sure, here are some related subtopics with details:
- Coin flipping technique: The coin flipping technique is used to randomly determine the number of layers an element will occupy in a skip list. When a new element is inserted, a random number is generated and compared to a predetermined threshold. If the random number is less than the threshold, the element is inserted into the next layer. This process is repeated until the random number is greater than the threshold, at which point the element is inserted into the bottom layer. The coin flipping technique is what gives skip lists their probabilistic nature and allows for their efficient average time complexity.
- Search operation: The search operation in a skip list involves traversing the layers from the top to bottom, skipping over elements that are not of interest, until the desired element is found or it is determined that it does not exist in the list. The skipping links in each layer allow for quick navigation to the desired element, reducing the average number of steps needed to reach it. The average time complexity of search in a skip list is O(log n), where n is the number of elements in the bottom layer.
- Insertion operation: The insertion operation in a skip list involves generating a random number to determine the number of layers the new element will occupy, as described in the coin flipping technique, and then inserting the element into the appropriate layers. The insertion operation preserves the sorted order of the elements in the list. The average time complexity of insertion in a skip list is O(log n), where n is the number of elements in the bottom layer.
- Deletion operation: The deletion operation in a skip list involves finding the element to be deleted and then removing it from all layers in which it appears. The deletion operation preserves the sorted order of the elements in the list. The average time complexity of deletion in a skip list is O(log n), where n is the number of elements in the bottom layer.
- Complexity analysis: The average time complexity of search, insertion, and deletion in a skip list is O(log n), where n is the number of elements in the bottom layer. This is due to the use of the coin flipping technique, which allows for quick navigation to the desired element, and the probabilistic nature of skip lists, which ensures that the average number of steps needed to reach an element is logarithmic in the number of elements in the bottom layer. However, the worst-case time complexity of these operations can be O(n), which occurs in the rare case where all elements are inserted into only the bottom layer.
Please Login to comment...
Similar reads.
- Advanced Data Structure
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
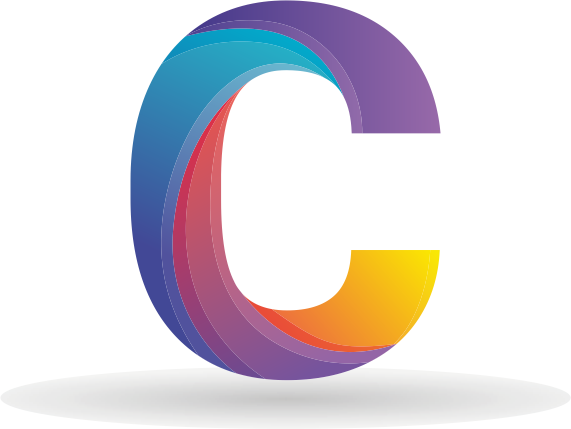
- Introduction
- Abstract data types
- Linked List Introduction
- Single Linked List
- Stacks using Array
- Stacks using linkedlist
- Queues using array
- Queues using linkedlist
- Stack Applications
- Infix to Postfic Converstion
- Postfix Evaluation
- Introduction to Dictionaries
- Linear list representation
Skip list representation
- Introduction Hash Table
- Hash functions
- Collision Resolution
- Separate chaining
- Open addressing
- Extendible hashing
- Introduction to Tree
- Binary trees Representation
- Binary tree traversals
- Binary Search Tree
- Red Black Tree
- Comparison of Trees
- Introduction to Graph
- Graph Representation
- Breadth-First Search
- Depth-First Search
- Introduction to pattern Matching
- Brute force Pattern Matching
- Boyer–Moore Pattern Matching
- KMP Pattern Matching
- Introduction to Tries
- Standard Trie
- Compressed tries
- Suffix Trie
- Control Structures
- Form Handing
- File Uploading
- Database Programming
- File Handling
A Skip List is an advanced data structure that allows for fast search within an ordered sequence of elements, providing a clever alternative to balanced trees. It is effectively a linked-list that adds multiple layers on top of the standard list to enable faster search times.
Here's how a Skip List is typically represented:
- Base Layer: The bottom layer is a standard linear linked list that contains all the elements in the set, sorted by key.
- Express Layers: Above the base layer are one or more layers of "express lanes." Each layer is a linked list that skips over some elements from the list below it. The topmost layer has the fewest elements, and each layer doubles (or varies by some factor) the number of elements it skips compared to the layer directly beneath it.
A Skip List is an efficient, probabilistic data structure that enables fast search, insertion, and deletion operations, akin to balanced trees like AVL or Red-Black trees. Here's a step-by-step explanation of how a Skip List is used to represent a dictionary:
Step 1: Understanding the Structure
A Skip List is composed of several layers of linked lists, where each higher layer provides a "shortcut" through the lower layers. The bottom layer (Layer 0) contains all the elements, and each successive layer contains a subset of these elements, chosen randomly.
Step 2: Layered Links
Each node in the Skip List contains pointers to the next node in the same layer and down to the same node in the layer immediately below it.
Step 3: Initialization
When initializing a Skip List:
- Create a "head" node with pointers set to NULL for each layer.
- Set a maximum level for the list, which determines how many layers the list can have.
- Optionally, set a "tail" node with maximum possible key value to mark the end of each level.
Step 4: Insertion
To insert a key-value pair:
- Find the Position: Start from the topmost layer and move forward until you find a node with a greater key or reach the end of the layer. Then, move down one layer and continue. Record the nodes where you move down a level.
- Choose a Random Level: For the new node, randomly decide the number of layers (level) it will participate in (usually done with a coin flip algorithm).
- Rearrange Pointers: For each level up to the chosen level, update the pointers of the recorded nodes to include the new node.
Step 5: Search
To search for a key:
- Start from the topmost layer of the head node.
- Move forward in the current layer until you find a node with a greater key or reach the end.
- If you find a greater key, move down one layer.
- Repeat until you reach the bottom layer. If the key matches, return the value; otherwise, the key is not in the list.
Step 6: Deletion
To delete a key-value pair:
- Perform a search for the key, keeping track of the predecessors at each level.
- If the key is found, update the pointers of these predecessor nodes to skip the node being deleted.
- Remove the node and deallocate its memory.
Step 7: Random Level Generation
The level for a new node is typically determined using a random process. A common method is a coin flip algorithm: a random level is generated, and as long as a coin flip results in heads (or a random value meets a certain condition), you increase the level.
Step 8: Traversal
To traverse the Skip List, simply follow the bottom layer from the head node to the end, processing or printing the values.
Algorithm for Implementing Skip List
Advantages skip list representation of a dictionary:.
- Simpler to Implement: Compared to balanced trees, skip lists are easier to implement while still providing efficient search operations.
- Amortized Costs: Operations have good amortized time costs, making them suitable for applications with a mix of frequent reads and occasional writes.
- Lock-Free Concurrency: Skip lists can be more easily implemented in a lock-free manner, which is useful for concurrent algorithms.
Disadvantages Skip List representation of a dictionary:
- Randomization Overhead: The randomization needed for maintaining balance can introduce overhead.
- Space Consumption: Skip lists use more memory than a simple linked list due to the additional pointers for the express lanes.
Suggestion/Feedback Form :
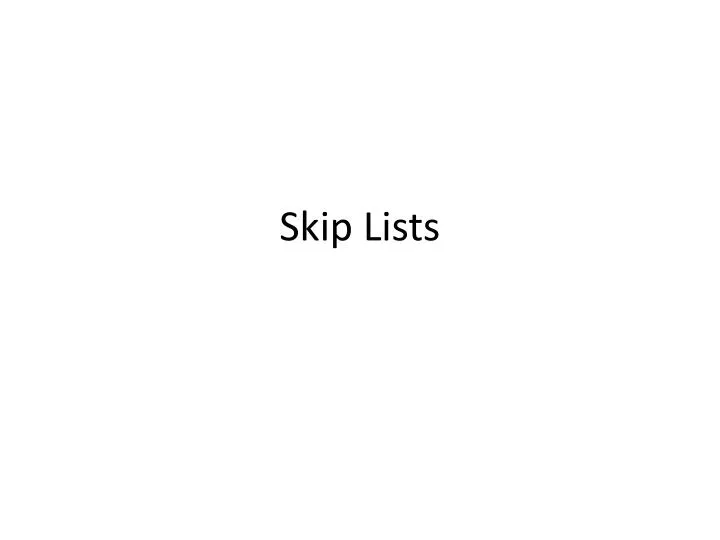
Oct 02, 2014
170 likes | 351 Views
Skip Lists. Outline and Reading. What is a skip list ( §9.4 ) Operations ( §9.4.1 ) Search Insertion Deletion Implementation Analysis ( §9.4.2 ) Space usage Search and update times.
Share Presentation
- running time
- coin tosses
- skip list 9
- coin tosses give heads
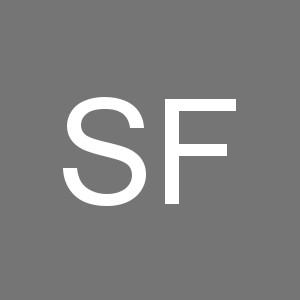
Presentation Transcript
Outline and Reading • What is a skip list (§9.4) • Operations (§9.4.1) • Search • Insertion • Deletion • Implementation • Analysis (§9.4.2) • Space usage • Search and update times
A skip list for a set S of distinct (key, element) items is a series of lists S0, S1 , … , Sh such that Each list Si contains the special keys + and - List S0 contains the keys of S in non-decreasing order Each list is a subsequence of the previous one, i.e.,S0 S1 … Sh List Sh contains only the two special keys Skip lists are one way to implement the dictionary ADT Java applet - + - 12 23 26 31 34 44 56 64 78 + What is a Skip List S3 S2 - 31 + S1 - 23 31 34 64 + S0
We can implement a skip list with quad-nodes A quad-node stores: item link to the node before link to the node after link to the node below Also, we define special keys PLUS_INF and MINUS_INF, and we modify the key comparator to handle them Implementation quad-node x
We search for a key x in a a skip list as follows: We start at the first position of the top list At the current position p, we compare x with y key(after(p)) x = y: we return element(after(p)) x > y: we “scan forward” x < y: we “drop down” If we try to drop down past the bottom list, we return NO_SUCH_KEY Example: search for 78 Search S3 - + S2 - 31 + S1 - 23 31 34 64 + S0 - 12 23 26 31 34 44 56 64 78 +
We search for a key x in a a skip list as follows: We start at the first position of the top list At the current position p, we compare x with y key(after(p)) x = y: we return element(after(p)) x > y: we “scan forward” x < y: we “drop down” If we try to drop down past the bottom list, we return NO_SUCH_KEY Ex 1: search for 64: list the (Si, node) pairs visited and the return value Ex 2: search for 27: list the (Si, node) pairs visited and the return value Exercise: Search S3 - + S2 - 31 + S1 - 23 31 34 64 + S0 - 12 23 26 31 34 44 56 64 78 +
- + - 15 + - 15 23 + - 10 23 36 + 15 Insertion • To insert an item (x, o) into a skip list, we use a randomized algorithm: • We repeatedly toss a coin until we get tails, and we denote with i the number of times the coin came up heads • If i h, we add to the skip list new lists Sh+1, … , Si +1, each containing only the two special keys • We search for x in the skip list and find the positions p0, p1 , …, pi of the items with largest key less than x in each list S0, S1, … , Si • For j 0, …, i, we insert item (x, o) into list Sj after position pj • Example: insert key 15, with i= 2 S3 p2 S2 S2 - + p1 S1 S1 - + 23 p0 S0 S0 - 10 23 36 +
Deletion • To remove an item with key xfrom a skip list, we proceed as follows: • We search for x in the skip list and find the positions p0, p1 , …, pi of the items with key x, where position pj is in list Sj • We remove positions p0, p1 , …, pi from the lists S0, S1, … , Si • We remove all but one list containing only the two special keys • Example: remove key 34 S3 - + p2 S2 S2 - + - 34 + p1 S1 S1 - + - 23 34 + 23 p0 S0 S0 - 12 23 45 + - 12 23 45 + 34
A randomized algorithm controls its execution through random selection (e.g., coin tosses) It contains statements like: b randomBit() if b= 0 do A … else { b= 1} do B … Its running time depends on the outcomes of the coin tosses Through probabilistic analysis we can derive the expected running time of a randomized algorithm We make the following assumptions in the analysis: the coins are unbiased the coin tosses are independent The worst-case running time of a randomized algorithm is often large but has very low probability (e.g., it occurs when all the coin tosses give “heads”) We use a randomized algorithm to insert items into a skip list to insert in expected O(log n)-time When randomization is used in data structures they are referred to as probabilistic data structures Randomized Algorithms
The space used by a skip list depends on the random bits used by each invocation of the insertion algorithm We use the following two basic probabilistic facts: Fact 1: The probability of getting i consecutive heads when flipping a coin is 1/2i Fact 2: If each of n items is present in a set with probability p, the expected size of the set is np Consider a skip list with n items By Fact 1, we insert an item in list Si with probability 1/2i By Fact 2, the expected size of list Si is n/2i The expected number of nodes used by the skip list is Space Usage Thus, the expected space usage of a skip list with n items is O(n)
The running time of the search an insertion algorithms is affected by the height h of the skip list We show that with high probability, a skip list with n items has height O(log n) We use the following additional probabilistic fact: Fact 3: If each of n events has probability p, the probability that at least one event occurs is at most np Consider a skip list with n items By Fact 1, we insert an item in list Si with probability 1/2i By Fact 3, the probability that list Si has at least one item is at most n/2i By picking i= 3log n, we have that the probability that S3log n has at least one item isat mostn/23log n= n/n3= 1/n2 Thus a skip list with n items has height at most 3log n with probability at least 1 - 1/n2 Height
The search time in a skip list is proportional to the number of drop-down steps, plus the number of scan-forward steps The drop-down steps are bounded by the height of the skip list and thus are O(log n) with high probability To analyze the scan-forward steps, we use yet another probabilistic fact: Fact 4:The expected number of coin tosses required in order to get tails is 2 When we scan forward in a list, the destination key does not belong to a higher list A scan-forward step is associated with a former coin toss that gave tails By Fact 4, in each list the expected number of scan-forward steps is 2 Thus, the expected number of scan-forward steps is O(log n) We conclude that a search in a skip list takes O(log n) expected time The analysis of insertion and deletion gives similar results Search and Update Times
Exercise • You are working for ObscureDictionaries.com a new online start-up which specializes in sci-fi languages. The CEO wants your team to describe a data structure which will efficiently allow for searching, inserting, and deleting new entries. You believe a skip list is a good idea, but need to convince the CEO. Perform the following: • Illustrate insertion of “X-wing” into this skip list. Randomly generated (1, 1, 1, 0). • Illustrate deletion of an incorrect entry “Enterprise” S2 - + S1 Enterprise + - S0 BobaFett Yoda + - Enterprise
A skip list is a data structure for dictionaries that uses a randomized insertion algorithm In a skip list with n items The expected space used is O(n) The expected search, insertion and deletion time is O(log n) Using a more complex probabilistic analysis, one can show that these performance bounds also hold with high probability Skip lists are fast and simple to implement in practice Summary
- More by User
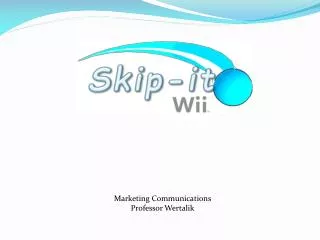
Skip-it. Marketing Communications Professor Wertalik. Table of Contents. Executive Summary pg. 3 Industry Background pg. 4 Company Snapshot pg. 5 Brand Review pg. 6 Competitive Review pg. 7 Buyer Analysis pg. 8 SWOT Analysis pg. 9 Marketing Goals pg. 10
483 views • 30 slides
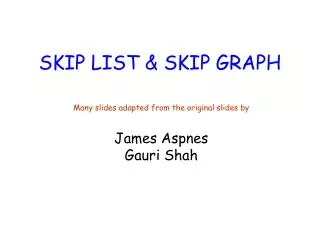
SKIP LIST & SKIP GRAPH
SKIP LIST & SKIP GRAPH. Many slides adapted from the original slides by James Aspnes Gauri Shah. Definition of Skip List. A skip list for a set L of distinct (key, element) items is a series of linked lists L 0 , L 1 , … , L h such that
768 views • 40 slides
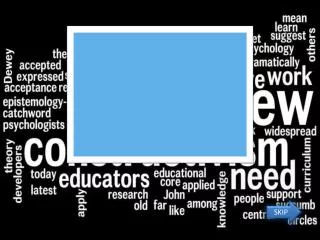
SKIP. KMR Enterprises Software License Agreement for Conditions of Learning and Instructional Design
344 views • 16 slides
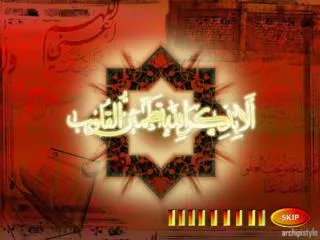
SKIP. ǽ Я α™. Registration Number. 1. 4. D. K. A. 0. 8. F. 2. 0. 1. 9. Presented by. Password. Aliyul Adzim bin Abdul Ghapar. ●. ●. ●. ●. ●. ●. ●. ●. Mohd Saifullizam bin Norhman. Sign In. Rudihartono bin Hasnan. Forgot the password? Click here or sign up.
257 views • 13 slides
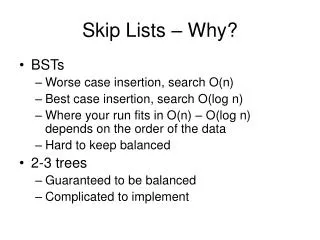
Skip Lists – Why?
Skip Lists – Why?. BSTs Worse case insertion, search O(n) Best case insertion, search O(log n) Where your run fits in O(n) – O(log n) depends on the order of the data Hard to keep balanced 2-3 trees Guaranteed to be balanced Complicated to implement. Skip List.
193 views • 7 slides
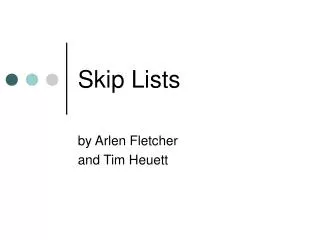
Skip Lists. by Arlen Fletcher and Tim Heuett. What are skip lists?. Developed around 1989 by William Pugh as an alternative to balanced trees A probabilistic data structure based on parallel linked lists (we’ll get to this…). Skip Lists – “Express Lanes”!. Express Lanes. Highway. Roads.
746 views • 12 slides
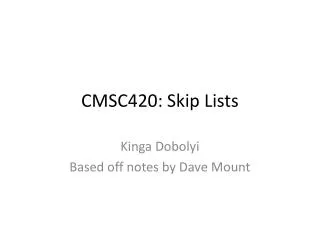
CMSC420: Skip Lists
CMSC420: Skip Lists. Kinga Dobolyi Based off notes by Dave Mount. What is the purpose of this class?. Data storage Speed Trees Binary search tree Could degenerate into a linked list AVL tree Better performance, difficult to implement Splay tree Good overall performance -- amortized.
247 views • 12 slides
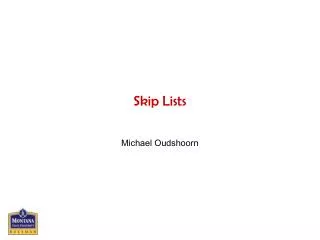
Skip Lists. Michael Oudshoorn. Skip Lists. Binary Search Trees: O(log n) If, and only if, the tree is “balanced” Insertion of partially sorted data is optimally bad for binary search trees. Skip Lists make use of some randomization to maintain O(log n) search and update times
315 views • 12 slides
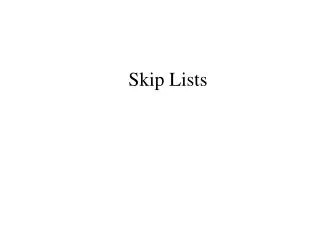
Skip Lists. 12. 23. 26. 31. 34. 44. 56. 64. 78. Skip List. Question: Can we create a structure that adds the best properties of Array and Linked list Data Structure? Query: O(log n) in sorted Arrays Insert/Removal: O(1) in Linked List.
333 views • 16 slides
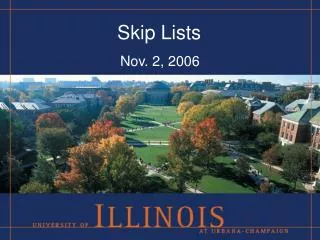
Skip Lists. Nov. 2, 2006. Midterm #2. Nov. 9 Thu 7pm. Pseudorandom number generator. Middle-square method (John von Neumann, 1946) Take any number, square it, remove the middle digits of the resulting number as your "random number", then use that number as the seed for the next iteration
153 views • 9 slides
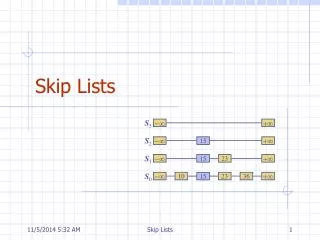
- . + . - . 15. + . - . 15. 23. + . - . 10. 23. 36. + . 15. Skip Lists. S 3. S 2. S 1. S 0. Outline and Reading. What is a skip list ( §8.4 ) Operations Search ( §8.4.1 ) Insertion ( §8.4.2 ) Deletion ( §8.4.2 ) Implementation Analysis ( §8.4.3 ) Space usage
306 views • 16 slides
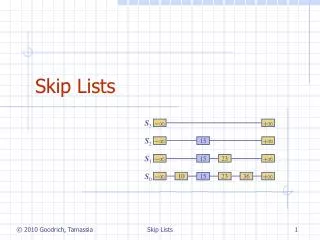
- . + . - . 15. + . - . 15. 23. + . - . 10. 23. 36. + . 15. Skip Lists. S 3. S 2. S 1. S 0. A skip list for a set S of distinct (key, element) items is a series of lists S 0 , S 1 , … , S h such that Each list S i contains the special keys + and -
186 views • 11 slides
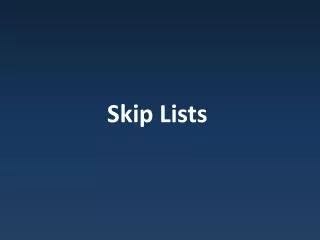
Skip Lists. Linked Lists. Fast modifications given a pointer Slow traversals to random point. Linked Lists. Fast modifications given a pointer Slow traversals to random point What if we add an express lane?. Linked Lists.
482 views • 40 slides
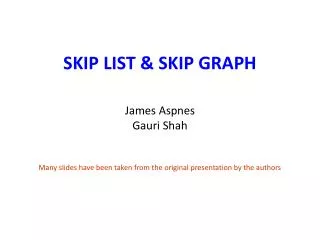
SKIP LIST & SKIP GRAPH. James Aspnes Gauri Shah Many slides have been taken from the original presentation by the authors. Definition of Skip List. A skip list for a set L of distinct (key, element) items is a series of linked lists L 0 , L 1 , … , L h such that
599 views • 40 slides
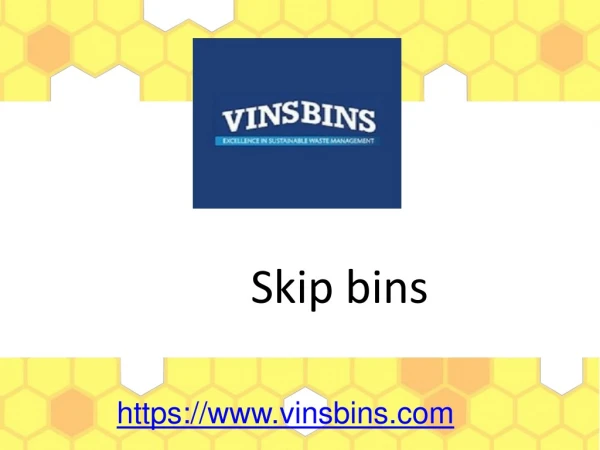
VinsBins are your Hastings Skip Hire experts. VinsBins provide bin hire and waste management services to Hastings and across the Mornington Peninsula. We have close to 500 skip bins for hire, with many size options available. These include the very popular 3 cubic meter bin that's used for household clean-ups or as a site bin. More- https://www.vinsbins.com/
60 views • 5 slides
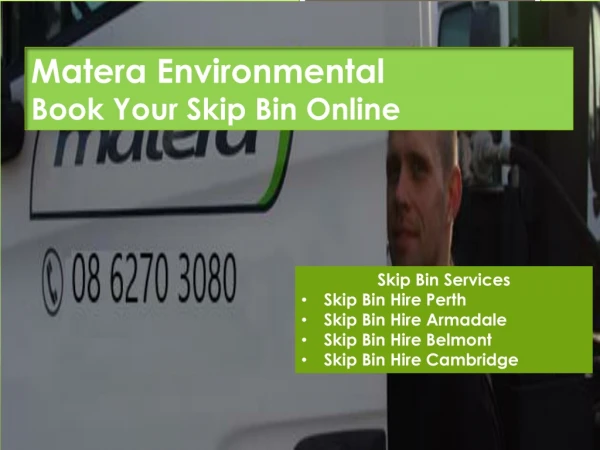
Skip Bin Hire|Hire Skip Bins|Skip Bins|Skip Bins Perth|Perth Skip Bin Hire|Skip Hire Perth
Contact Matera Environmental today for Skip Bins Hire in Perth .We provide Online,Cost-Effective and reliable Skip Bin Hire services in Perth,South West of WA.
77 views • 6 slides
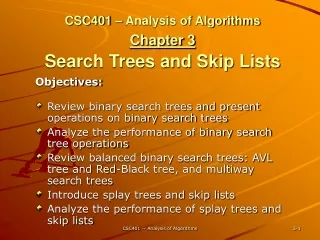
CSC401 – Analysis of Algorithms Chapter 3 Search Trees and Skip Lists
CSC401 – Analysis of Algorithms Chapter 3 Search Trees and Skip Lists. Objectives: Review binary search trees and present operations on binary search trees Analyze the performance of binary search tree operations
621 views • 61 slides
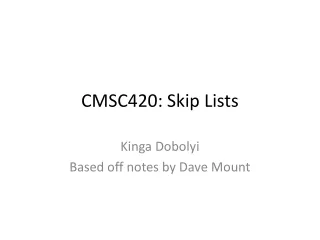
141 views • 12 slides
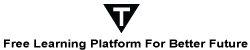
- Data Structure
- Design Pattern
DS Tutorial
Ds linked list, ds searching, differences.
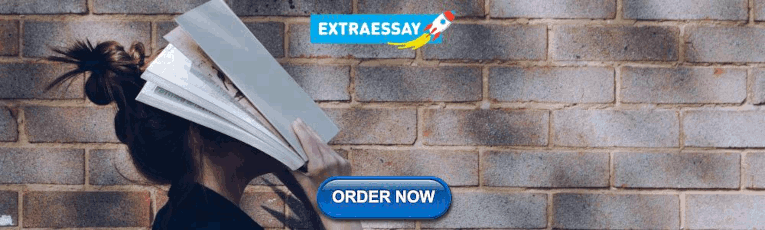
Working of the Skip list
Let's take an example to understand the working of the skip list. In this example, we have 14 nodes, such that these nodes are divided into two layers, as shown in the diagram.
The lower layer is a common line that links all nodes, and the top layer is an express line that links only the main nodes, as you can see in the diagram.
Suppose you want to find 47 in this example. You will start the search from the first node of the express line and continue running on the express line until you find a node that is equal a 47 or more than 47.
You can see in the example that 47 does not exist in the express line, so you search for a node of less than 47, which is 40. Now, you go to the normal line with the help of 40, and search the 47, as shown in the diagram.
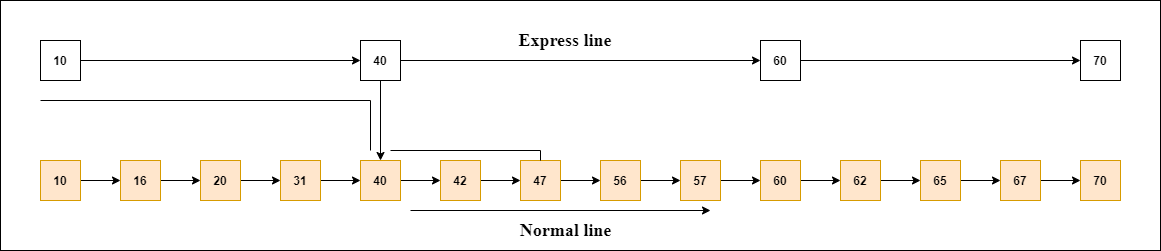
Note: Once you find a node like this on the "express line", you go from this node to a "normal lane" using a pointer, and when you search for the node in the normal line.
Skip list basic operations.
There are the following types of operations in the skip list.
- Insertion operation: It is used to add a new node to a particular location in a specific situation.
- Deletion operation: It is used to delete a node in a specific situation.
- Search Operation: The search operation is used to search a particular node in a skip list.
Algorithm of the insertion operation
Algorithm of deletion operation
Algorithm of searching operation
Example 1: Create a skip list, we want to insert these following keys in the empty skip list.
- 6 with level 1.
- 29 with level 1.
- 22 with level 4.
- 9 with level 3.
- 17 with level 1.
- 4 with level 2.
Step 1: Insert 6 with level 1
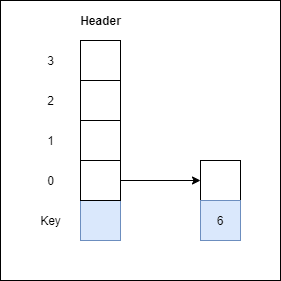
Step 2: Insert 29 with level 1
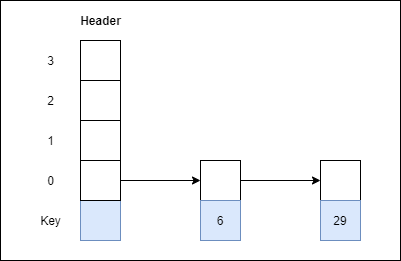
Step 3: Insert 22 with level 4
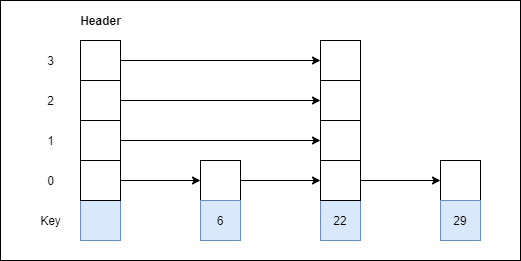
Step 4: Insert 9 with level 3
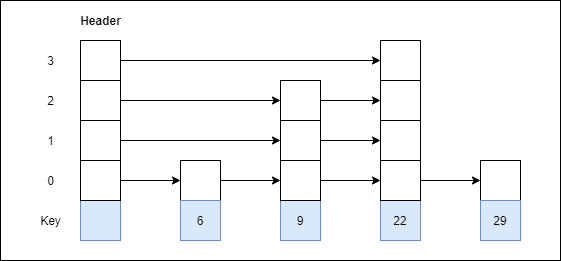
Step 5: Insert 17 with level 1
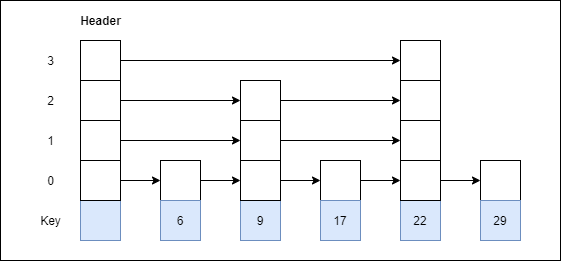
Step 6: Insert 4 with level 2
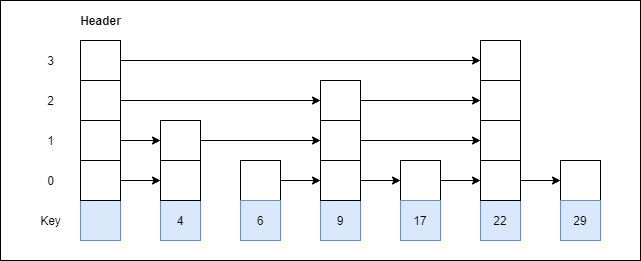
Example 2: Consider this example where we want to search for key 17.
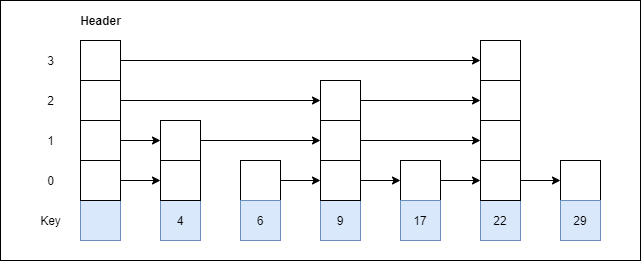
Advantages of the Skip list
- If you want to insert a new node in the skip list, then it will insert the node very fast because there are no rotations in the skip list.
- The skip list is simple to implement as compared to the hash table and the binary search tree.
- It is very simple to find a node in the list because it stores the nodes in sorted form.
- The skip list algorithm can be modified very easily in a more specific structure, such as indexable skip lists, trees, or priority queues.
- The skip list is a robust and reliable list.
Disadvantages of the Skip list
- It requires more memory than the balanced tree.
- Reverse searching is not allowed.
- The skip list searches the node much slower than the linked list.
Applications of the Skip list
- It is used in distributed applications, and it represents the pointers and system in the distributed applications.
- It is used to implement a dynamic elastic concurrent queue with low lock contention.
- It is also used with the QMap template class.
- The indexing of the skip list is used in running median problems.
- The skip list is used for the delta-encoding posting in the Lucene search.

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse


- Preferences
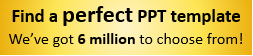
Skip Lists - PowerPoint PPT Presentation

Something went wrong! Please try again and reload the page.
What is a Skip List. A skip list for a set S of distinct (key, element) items is a series of lists S0, ... We start at the first position of the top list ... – PowerPoint PPT presentation
- Can we create a structure that adds the best properties of Array and Linked list Data Structure?
- Query O(log n) in sorted Arrays
- Insert/Removal O(1) in Linked List
- A skip list for a set S of distinct (key, element) items is a series of lists S0, S1 , , Sh such that
- Each list Si contains the special keys ? and -?
- List S0 contains the keys of S in nondecreasing order
- Each list is a subsequence of the previous one, i.e., S0 ? S1 ? ? Sh
- List Sh contains only the two special keys
- We show how to use a skip list to implement the dictionary ADT
- We search for a key x in a skip list as follows
- We start at the first position of the top list
- At the current position p, we compare x with y ? key(after(p))
- x y we return element(after(p))
- x gt y we scan forward
- x lt y we drop down
- If we try to drop down past the bottom list, we return NO_SUCH_KEY
- Example search for 78
- To insert an item (x, o) into a skip list, we use a randomized algorithm
- We repeatedly toss a coin until we get tails, and we denote with i the number of times the coin came up heads
- If i ? h, we add to the skip list new lists Sh1, , Si 1, each containing only the two special keys
- We search for x in the skip list and find the positions p0, p1 , , pi of the items with largest key less than x in each list S0, S1, , Si
- For j ? 0, , i, we insert item (x, o) into list Sj after position pj
- Example insert key 15, with i 2
- To remove an item with key x from a skip list, we proceed as follows
- We search for x in the skip list and find the positions p0, p1 , , pi of the items with key x, where position pj is in list Sj
- We remove positions p0, p1 , , pi from the lists S0, S1, , Si
- We remove all but one list containing only the two special keys
- Example remove key 34
- We can implement a skip list with quad-nodes
- A quad-node stores
- link to the node before
- link to the node after
- link to the node below
- Also, we define special keys PLUS_INF and MINUS_INF, and we modify the key comparator to handle them
- Consider a skip list with n items
- By Fact 1, we insert an item in list Si with probability 1/2i
- By Fact 2, the expected size of list Si is n/2i
- The expected number of nodes used by the skip list is
- The space used by a skip list depends on the random bits used by each invocation of the insertion algorithm
- We use the following two basic probabilistic facts
- Fact 1 The probability of getting i consecutive heads when flipping a coin is 1/2i
- Fact 2 If each of n items is present in a set with probability p, the expected size of the set is np
- Thus, the expected space usage of a skip list with n items is O(n)
- The running time of the search an insertion algorithms is affected by the height h of the skip list
- We show that with high probability, a skip list with n items has height O(log n)
- We use the following additional probabilistic fact
- Fact 3 If each of n events has probability p, the probability that at least one event occurs is at most np
- By Fact 3, the probability that list Si has at least one item is at most n/2i
- By picking i 3log n, we have the probability that S3log n has at least one item is at most n/23log n n/n3 1/n2
- Thus a skip list with n items has height at most 3log n with probability at least 1 - 1/n2
- The search time in a skip list is proportional to
- the number of drop-down steps, plus
- the number of scan-forward steps
- The drop-down steps are bounded by the height of the skip list and thus are O(log n) with high probability
- To analyze the scan-forward steps, we use yet another probabilistic fact
- Fact 4 The expected number of coin tosses required in order to get tails is 2
- When we scan forward in a list, the destination key does not belong to a higher list
- A scan-forward step is associated with a former coin toss that gave tails
- By Fact 4, in each list the expected number of scan-forward steps is 2
- Thus, the expected number of scan-forward steps is O(log n)
- We conclude that a search in a skip list takes O(log n) expected time
- The analysis of insertion and deletion gives similar results
- A skip list is a data structure for dictionaries that uses a randomized insertion algorithm
- In a skip list with n items
- The expected space used is O(n)
- The expected search, insertion and deletion time is O(log n)
- Using a more complex probabilistic analysis, one can show that these performance bounds also hold with high probability
- Skip lists are fast and simple to implement in practice
- Many sorting algorithms are comparison based.
- They sort by making comparisons between pairs of objects
- Examples bubble-sort, selection-sort, insertion-sort, heap-sort, merge-sort, quick-sort, ...
- Let us therefore derive a lower bound on the running time of any algorithm that uses comparisons to sort n elements, x1, x2, , xn.
- Let us just count comparisons then.
- Each possible run of the algorithm corresponds to a root-to-leaf path in a decision tree
- Example xa, xb, xc
- The height of this decision tree is a lower bound on the running time
- Every possible input permutation must lead to a separate leaf output.
- If not, some input 45 would have same output ordering as 54, which would be wrong.
- Since there are n!12n leaves, the height is at least log (n!)
- Any comparison-based sorting algorithms takes at least log (n!) time
- Therefore, any such algorithm takes time at least
- That is, any comparison-based sorting algorithm must run in O(n log n) time.
PowerShow.com is a leading presentation sharing website. It has millions of presentations already uploaded and available with 1,000s more being uploaded by its users every day. Whatever your area of interest, here you’ll be able to find and view presentations you’ll love and possibly download. And, best of all, it is completely free and easy to use.
You might even have a presentation you’d like to share with others. If so, just upload it to PowerShow.com. We’ll convert it to an HTML5 slideshow that includes all the media types you’ve already added: audio, video, music, pictures, animations and transition effects. Then you can share it with your target audience as well as PowerShow.com’s millions of monthly visitors. And, again, it’s all free.
About the Developers
PowerShow.com is brought to you by CrystalGraphics , the award-winning developer and market-leading publisher of rich-media enhancement products for presentations. Our product offerings include millions of PowerPoint templates, diagrams, animated 3D characters and more.

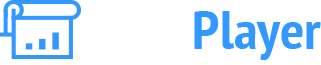
- My presentations
Auth with social network:
Download presentation
We think you have liked this presentation. If you wish to download it, please recommend it to your friends in any social system. Share buttons are a little bit lower. Thank you!
Presentation is loading. Please wait.
Skip Lists.
Published by Yenny Jayadi Modified over 5 years ago
Similar presentations
Presentation on theme: "Skip Lists."— Presentation transcript:
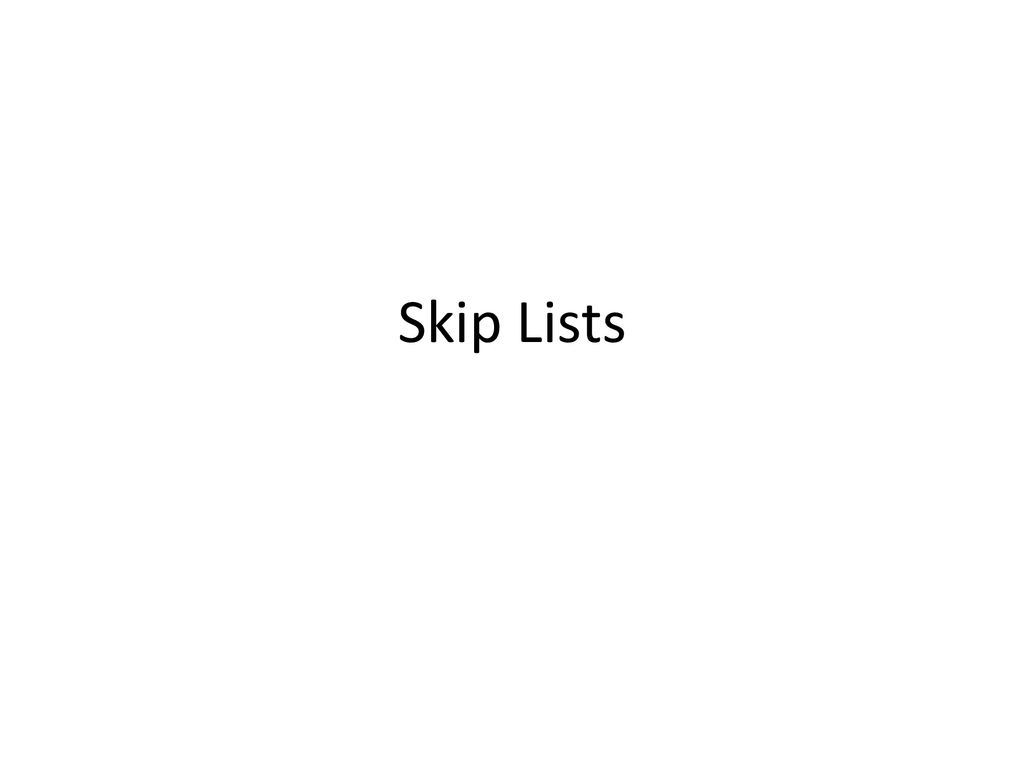
© 2004 Goodrich, Tamassia Skip Lists1 S0S0 S1S1 S2S2 S3S
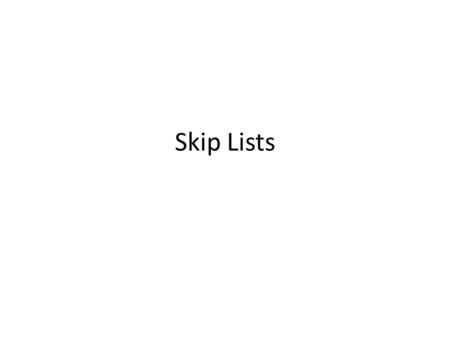
Skip Lists. Outline and Reading What is a skip list (§9.4) – Operations (§9.4.1) – Search – Insertion – Deletion Implementation Analysis (§9.4.2) – Space.
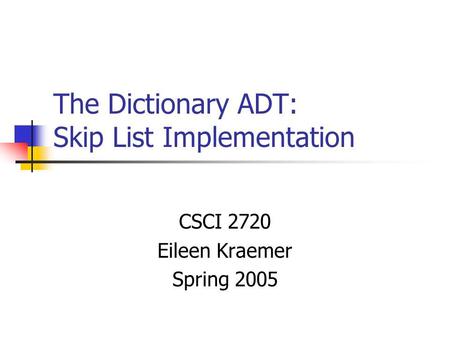
The Dictionary ADT: Skip List Implementation

SKIP LISTS Amihood Amir Incorporationg the slides of Goodrich and Tamassia (2004)
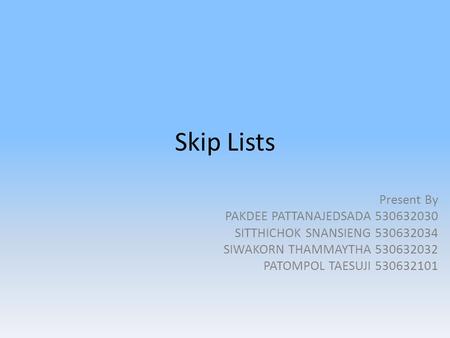
Skip Lists Present By PAKDEE PATTANAJEDSADA SITTHICHOK SNANSIENG SIWAKORN THAMMAYTHA PATOMPOL TAESUJI
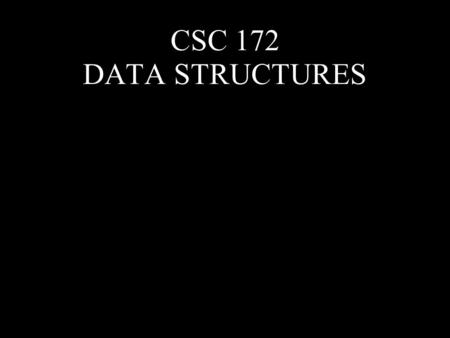
CSC 172 DATA STRUCTURES. SKIP LISTS Read Weiss
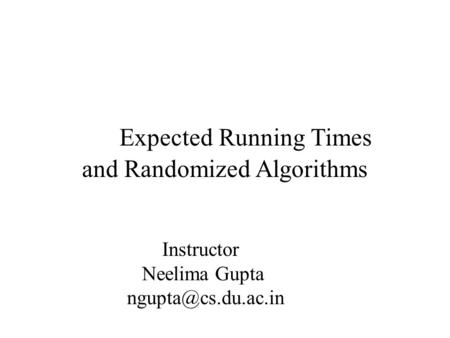
Expected Running Times and Randomized Algorithms Instructor Neelima Gupta
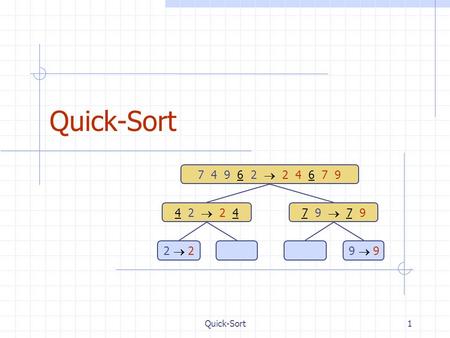
Quick-Sort 29 9.
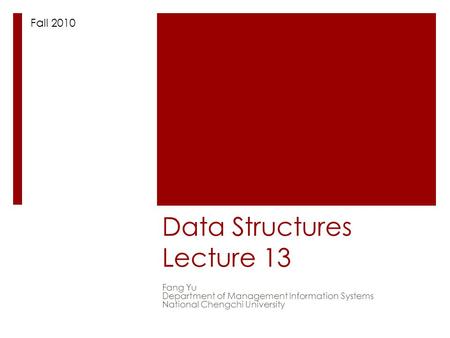
Data Structures Lecture 13 Fang Yu Department of Management Information Systems National Chengchi University Fall 2010.
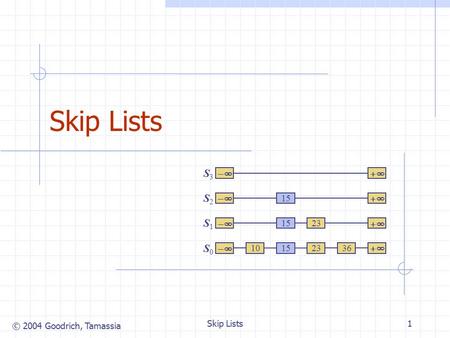
© 2004 Goodrich, Tamassia Skip Lists1 S0S0 S1S1 S2S2 S3S3 2315.
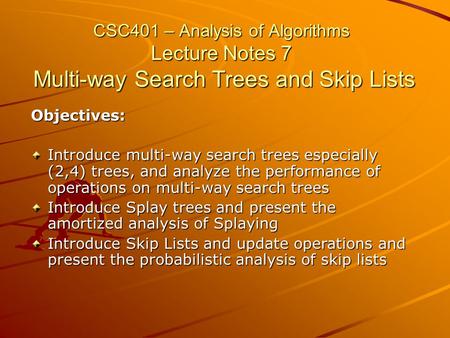
CSC401 – Analysis of Algorithms Lecture Notes 7 Multi-way Search Trees and Skip Lists Objectives: Introduce multi-way search trees especially (2,4) trees,
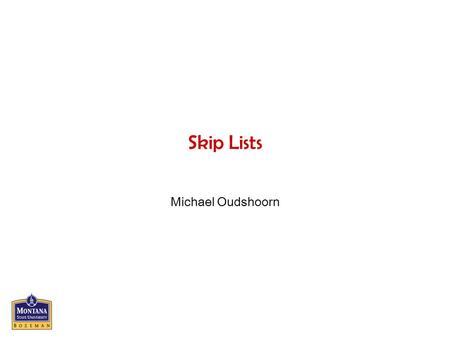
Skip Lists Michael Oudshoorn. CS351 Software Engineering (AY05)2 Skip Lists Binary Search Trees: O(log n) –If, and only if, the tree is “balanced” Insertion.
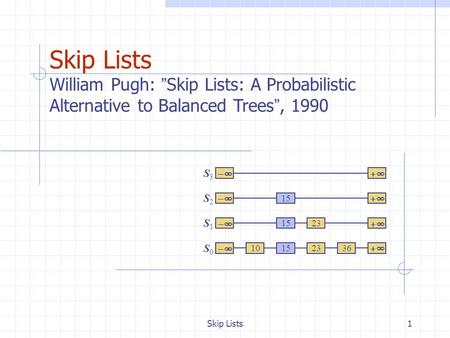
Skip Lists1 Skip Lists William Pugh: ” Skip Lists: A Probabilistic Alternative to Balanced Trees ”, 1990 S0S0 S1S1 S2S2 S3S3
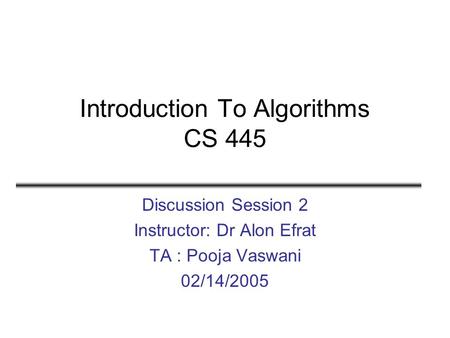
Introduction To Algorithms CS 445 Discussion Session 2 Instructor: Dr Alon Efrat TA : Pooja Vaswani 02/14/2005.
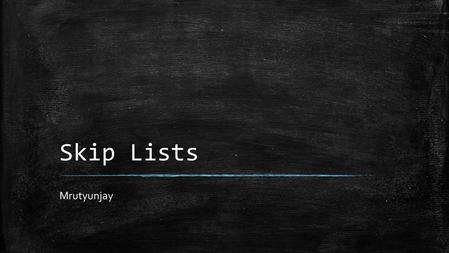
Skip Lists Mrutyunjay. Introduction ▪ Linked Lists Benefits & Drawbacks: – Benefits: – Easy Insert and Deletes, implementations. – Drawbacks: – Hard to.
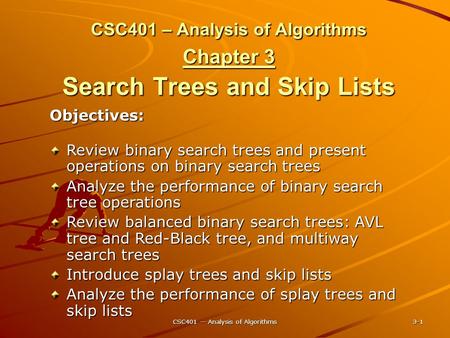
CSC Analysis of Algorithms 3-1 CSC401 – Analysis of Algorithms Chapter 3 Search Trees and Skip Lists Objectives: Review binary search trees and present.
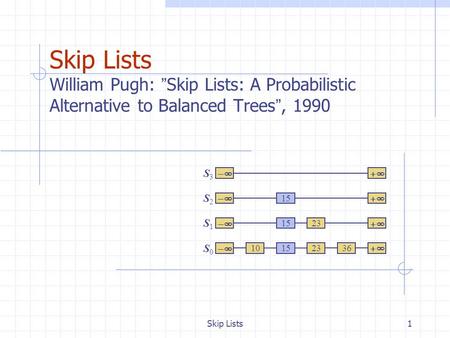
Skip Lists 二○一七年四月二十五日
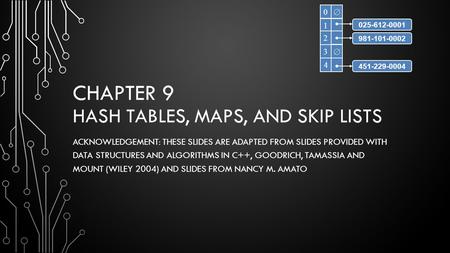
CHAPTER 9 HASH TABLES, MAPS, AND SKIP LISTS ACKNOWLEDGEMENT: THESE SLIDES ARE ADAPTED FROM SLIDES PROVIDED WITH DATA STRUCTURES AND ALGORITHMS IN C++,
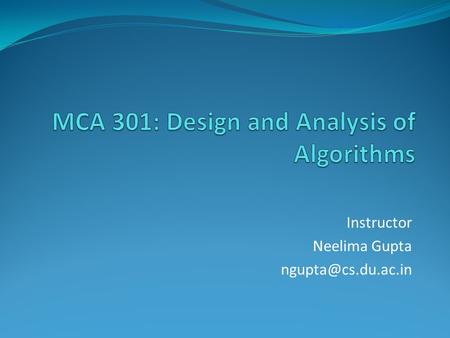
Instructor Neelima Gupta Expected Running Times and Randomized Algorithms Instructor Neelima Gupta
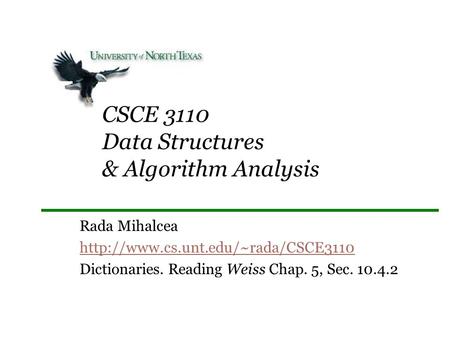
CSCE 3110 Data Structures & Algorithm Analysis Rada Mihalcea Dictionaries. Reading Weiss Chap. 5, Sec
About project
© 2024 SlidePlayer.com Inc. All rights reserved.
Here Are The Major Allegations Against Sean ‘Diddy’ Combs—As Cassie Ventura Breaks Silence On Attack Video
- Share to Facebook
- Share to Twitter
- Share to Linkedin
Singer Cassie Ventura said Thursday domestic violence “broke” her in a statement posted to Instagram , her first public remarks since CNN published footage last week depicting her ex-boyfriend Sean “Diddy” Combs attacking her in 2016—the latest controversy involving the rapper, who has faced a search of his home by federal agents and multiple civil lawsuits accusing him of abuse, all of which he has denied.
Combs has denied wrongdoing across all of the lawsuits and not been criminally charged. (Photo by ... [+] Paras Griffin/Getty Images)
Singer Cassie Ventura, Combs’ ex-girlfriend, spoke out after CNN obtained a 2016 video of Combs attacking her in a hotel hallway, stating on Instagram Thursday morning that domestic violence “broke” her and she will “always be recovering” from her past.
The video—which Combs later apologized for —came after Ventura sued Combs in November, alleging Combs raped her in 2018 and subjected her to a years-long abusive relationship that included physical abuse and his assertion of “complete control” over her personal and professional life.
Ventura’s $30 million suit was settled the day after it was filed for an undisclosed amount, with Ventura telling CNN she had chosen to “resolve this matter amicably,” while Combs’ attorney said the settlement was “in no way an admission of wrongdoing” and didn’t change his denial of the allegations.
Ventura’s lawsuit was the first in a litany of civil suits against Combs: Most recently, former model Crystal McKinney filed a lawsuit in Manhattan federal court on Tuesday accusing the rapper of drugging and sexually assaulting her at his New York recording studio in 2003.
In November, a woman named Joie Dickerson-Neal alleged in a lawsuit Combs drugged her, sexually assaulted her and secretly recorded the assault while she was a college student in 1991.
Separately that same month, an anonymous plaintiff accused Combs and singer-songwriter Aaron Hall of raping her and a friend in 1990 or 1991 after meeting at an MCA Records event in New York—a suit that, like the Dickerson-Neal complaint, was filed shortly before the expiration of a New York law temporarily allowing lawsuits for older assault allegations that would ordinarily be past the statute of limitations.
Combs was hit with another sexual assault suit in December, accusing the rapper of drugging and participating in a gang rape of the unnamed woman in 2003, when the accuser was 17 years old.
Producer Rodney “Lil Rod” Jones sued the rapper in New York in February and alleged he was “subjected to unwanted advances by associates of Diddy at his direction” and was forced to engage in relations with sex workers he hired.
In a set of widely covered allegations, Jones said in the lawsuit that Combs regularly hosted “sex-trafficking parties” with underage women and illegal drugs, and implies record label executives who looked the other way financially benefited from access to celebrities and dignitaries like the British royal Prince Harry, who is not accused of any wrongdoing or of attending parties himself (Combs’ attorney told the Los Angeles Times the suit includes “reckless name-dropping about events that are pure fiction.”)
Get Forbes Breaking News Text Alerts: We’re launching text message alerts so you'll always know the biggest stories shaping the day’s headlines. Text “Alerts” to (201) 335-0739 or sign up here : joinsubtext.com/forbes.
What We Don’t Know
Why Combs’ homes in Los Angeles and Miami were raided by federal Homeland Security Investigations agents in March. The agency did not elaborate on the investigation that prompted the searches, though NBC News and the Associated Press reported the searches stem from a sex trafficking probe. Combs has not been charged or accused by federal prosecutors of a crime, and it’s unclear whether charges against anybody are forthcoming.
Combs, 54, has denied all of the allegations against him, with his attorneys characterizing some of the lawsuits and their accusations to Forbes as money grabs , “ baseless ” or “ sickening .” Combs has not been criminally charged.
What To Watch For
An upcoming docuseries. Producer Curtis “50 Cent” Jackson said Tuesday that Netflix had won a “bidding war” for a docuseries about the string of recent abuse, rape and sex trafficking allegations against Combs. Jackson confirmed in a tweet Tuesday that the streamer bought the G-Unit Film & Television series about Combs he first started teasing in December, adding that, “if more victims keep coming out, I’m gonna need more episodes.” Proceeds from the film go to victims of sexual assault, Jackson said in November.
On Sunday, Combs posted to Instagram to apologize for his “disgusting” behavior in the surveillance video that showed him grabbing, dragging and kicking Cassie in 2016. The video seemed to back up much of the claims Ventura made in her November lawsuit, which an attorney for Combs called “offensive and outrageous” at the time. Los Angeles District Attorney’s Office called the video “extremely disturbing” and “difficult to watch” but said no charges would be filed because the apparent assault took place beyond the statue of limitation in California. In his apology video, Combs said the events occurred in "one of the darkest times” of his life and said he was “truly sorry” for his behavior. Meredith Firetog, one of Ventura's lawyers, later slammed the apology as disingenuous in a statement and said it was “more about himself than the many people he has hurt.” In Ventura’s November lawsuit, she accused Combs of paying the hotel in which the surveillance video was captured $50,000 for the footage.
Surprising Fact
Combs’ former personal chef, Cindy Rueda, accused Combs in a since-settled 2017 sexual harassment lawsuit of having her prepare and serve food to the rapper and his guests while they were engaged in sexual activity or right after they had done so.
Key Background
The allegations contained in lawsuits against Combs date as far back as the 1990s, when he founded his own record label, Bad Boy Records, which Rolling Stone has called “one of the most influential hip-hop labels of all time.” The label has signed major artists like The Notorious B.I.G., Janelle Monáe and Cassie, and has put out several of Combs' own albums, including "Press Play" and "Last Train To Paris.” Combs sold a 50% stake in Bad Boy to Warner Music Group in a reported $30 million deal in 2005. Combs has built a fortune through Bad Boy Records, several liquor brands, a fashion label and other ventures. He sold his share in the DeLeón tequila brand for $200 million earlier this year. He was ranked No. 14 on Forbes' list of the highest-paid entertainers in 2022, making an estimated $90 million that year. One of the rapper’s raided homes is located in Holmby Hills, an affluent neighborhood where Combs purchased a home for $40 million ten years ago.
Further Reading
Feds Search Sean ‘Diddy’ Combs’ L.A. And Miami Homes (Forbes)
Sean Combs Accused Of Sexually Assaulting 17-Year-Old In Latest Lawsuit (Forbes)
Rapper Sean ‘Diddy’ Combs Accused Of Rape And Sexual Assault In Two New Separate Lawsuits (Forbes)
Rapper Sean ‘Diddy’ Combs Accused Of Rape And Sex Trafficking By Singer And Former Partner (Forbes)
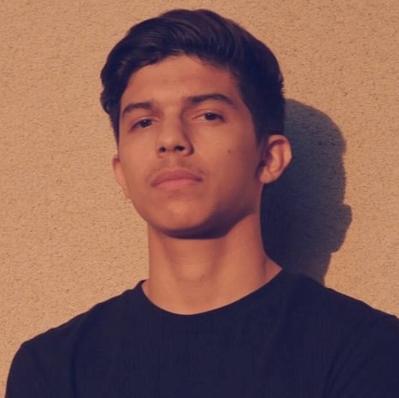
- Editorial Standards
- Reprints & Permissions
Join The Conversation
One Community. Many Voices. Create a free account to share your thoughts.
Forbes Community Guidelines
Our community is about connecting people through open and thoughtful conversations. We want our readers to share their views and exchange ideas and facts in a safe space.
In order to do so, please follow the posting rules in our site's Terms of Service. We've summarized some of those key rules below. Simply put, keep it civil.
Your post will be rejected if we notice that it seems to contain:
- False or intentionally out-of-context or misleading information
- Insults, profanity, incoherent, obscene or inflammatory language or threats of any kind
- Attacks on the identity of other commenters or the article's author
- Content that otherwise violates our site's terms.
User accounts will be blocked if we notice or believe that users are engaged in:
- Continuous attempts to re-post comments that have been previously moderated/rejected
- Racist, sexist, homophobic or other discriminatory comments
- Attempts or tactics that put the site security at risk
- Actions that otherwise violate our site's terms.
So, how can you be a power user?
- Stay on topic and share your insights
- Feel free to be clear and thoughtful to get your point across
- ‘Like’ or ‘Dislike’ to show your point of view.
- Protect your community.
- Use the report tool to alert us when someone breaks the rules.
Thanks for reading our community guidelines. Please read the full list of posting rules found in our site's Terms of Service.
- Category: Xbox Game Pass
Coming to Game Pass: Senua’s Saga: Hellblade II, Immortals of Aveum, Lords of the Fallen, and More
Grab your controllers and keyboards, because we have another round of games coming soon! Most importantly though, grab your headphones – Senua’s Saga: Hellblade II launches next week. While sharing is caring, this is one you’re going to want to listen to yourself for the full experience. Now let’s get to all the games!
Available Today
Brothers: A Tale of Two Sons (Cloud, Console, and PC) The critically acclaimed and award-winning classic is returning to the Game Pass library! Guide two brothers on an epic fairy tale journey from visionary Swedish film director Josef Fares and developer Starbreeze Studios. Solve puzzles, explore the varied locations, and fight boss battles while controlling one brother with each thumb stick.
Coming Soon
Chants of Sennaar (Cloud, Console, and PC) – May 15 In this award-winning puzzle adventure game, play as the Traveler whose quest is to reunite the Peoples of the Tower. Observe, listen, and decipher ancient languages in a fascinating universe inspired by the Myth of Babel.
EA Sports NHL 24 (Cloud) EA Play – May 16 EA Sports NHL 24 will be available with Xbox Cloud Gaming soon via EA Play! Feel the intensity of hockey with all new gameplay features that dial up the pressure, physicality, and control of authentic on-ice action.
Immortals of Aveum (Cloud, PC, and Xbox Series X|S) EA Play – May 16 Immortals of Aveum is coming to The Play List! Summon your power with PC Game Pass and Ultimate via EA Play in this single-player, first-person magic shooter. Unleash an arsenal of spells as Jak, who joins an elite order of battlemages to save a world on the edge of abyss.
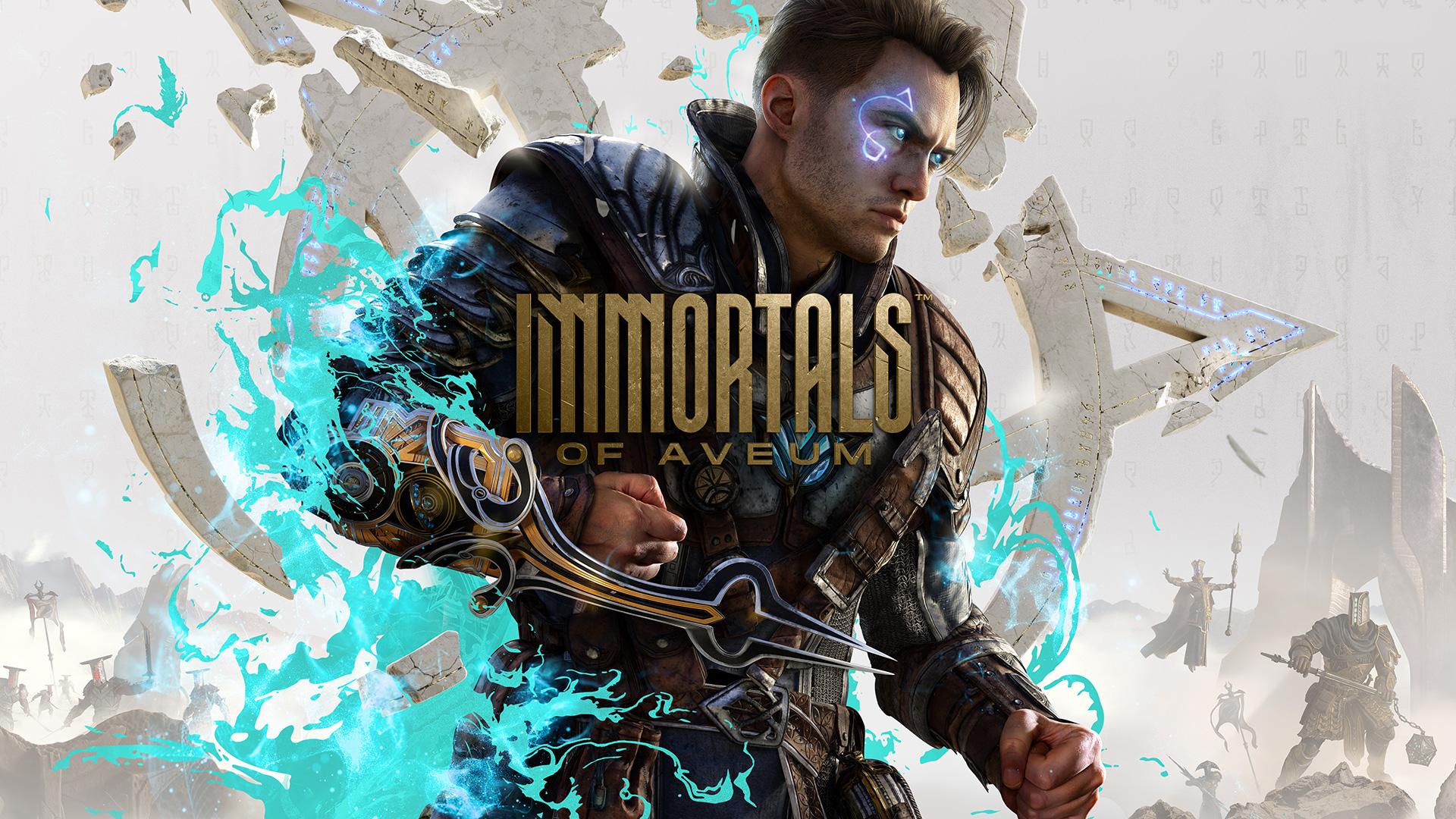
Senua’s Saga: Hellblade II (Cloud, PC, and Xbox Series X|S) – May 21 Available on day one with Game Pass! The sequel to the award winning Hellblade: Senua’s Sacrifice , Senua returns in a brutal journey of survival through the myth and torment of Viking Iceland. Intent on saving those who have fallen victim to the horrors of tyranny, Senua faces a battle of overcoming the darkness within and without. Pre-install now to get ready to play on day one.
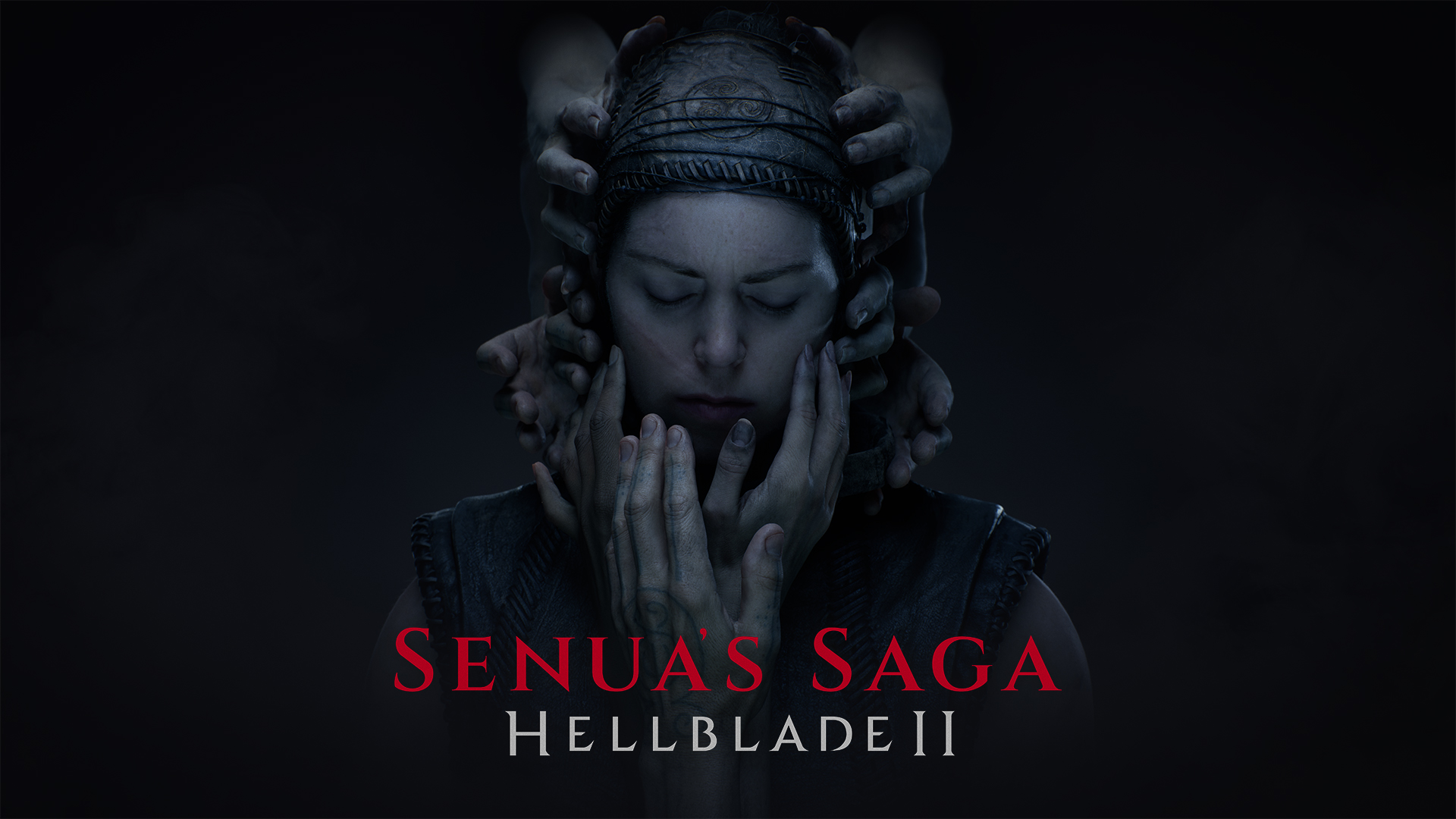
Galacticare (Cloud, PC, Xbox Series X|S) – May 23 Available on day one with Game Pass! You are the Director of Galacticare, an interstellar healthcare company and quasi-voluntary savior of the Galaxy (for cash). Build hospitals and recruit staff to satisfy the whims of various alien species and cure their bizarre illnesses. Save the (literal) Galaxy in story mode, or head into sandbox to design the hospital of your dreams.
Hauntii (Cloud, Console, and PC) – May 23 Available on day one with Game Pass! Play as a brave yet naive little ghost, Hauntii, and set off on a quest guided by enigmatic Eternians. Possess, solve puzzles, and shape your fate in this captivating adventure. The game’s hand-crafted art style blends line art and animation with a striking palette, creating a visually captivating experience. Coupled with the dynamic soundtrack, it enhances the immersive exploration of mysterious landscapes.
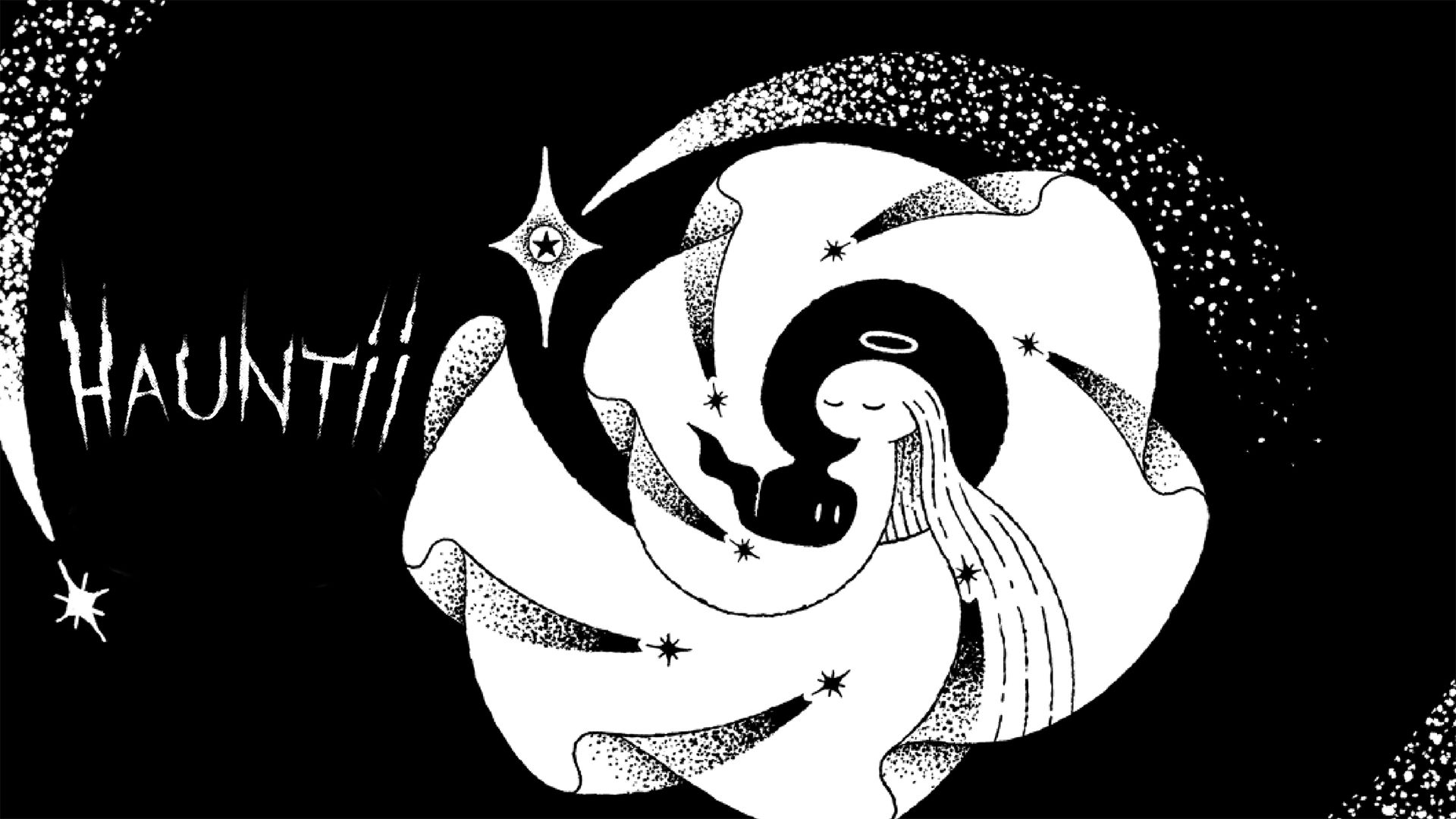
Moving Out 2 (Cloud, Console, and PC) – May 28 Are you ready to become an all-star F.A.R.T? That’s a Furniture Arrangement & Relocation Technician in the world of Moving Out 2 . Working solo, or with up to three friends, slip into your uniform and help the residents of Packmore, and beyond, to pack up and ship out!
Humanity (Cloud, Console, and PC) – May 30 Become a Shiba Inu and help reconstruct humankind in the acclaimed action-puzzle game Humanity . Drop commands to guide a mindless human horde to the goal through 90 increasingly challenging handcrafted story mode stages. Then, choose from thousands of user-made puzzles expanding on the breadth of mechanics, or design your own and share it with the community.
Lords of the Fallen (Cloud, PC, and Xbox Series X|S) – May 30 A vast world awaits in the all-new, dark fantasy action-RPG, Lords of the Fallen . As one of the fabled Dark Crusaders, embark on an epic quest to overthrow Adyr, the demon God. Learn more about how Lords of the Fallen goes rogue(like) with its recent ‘Master of Fate’ update on Xbox Wire .
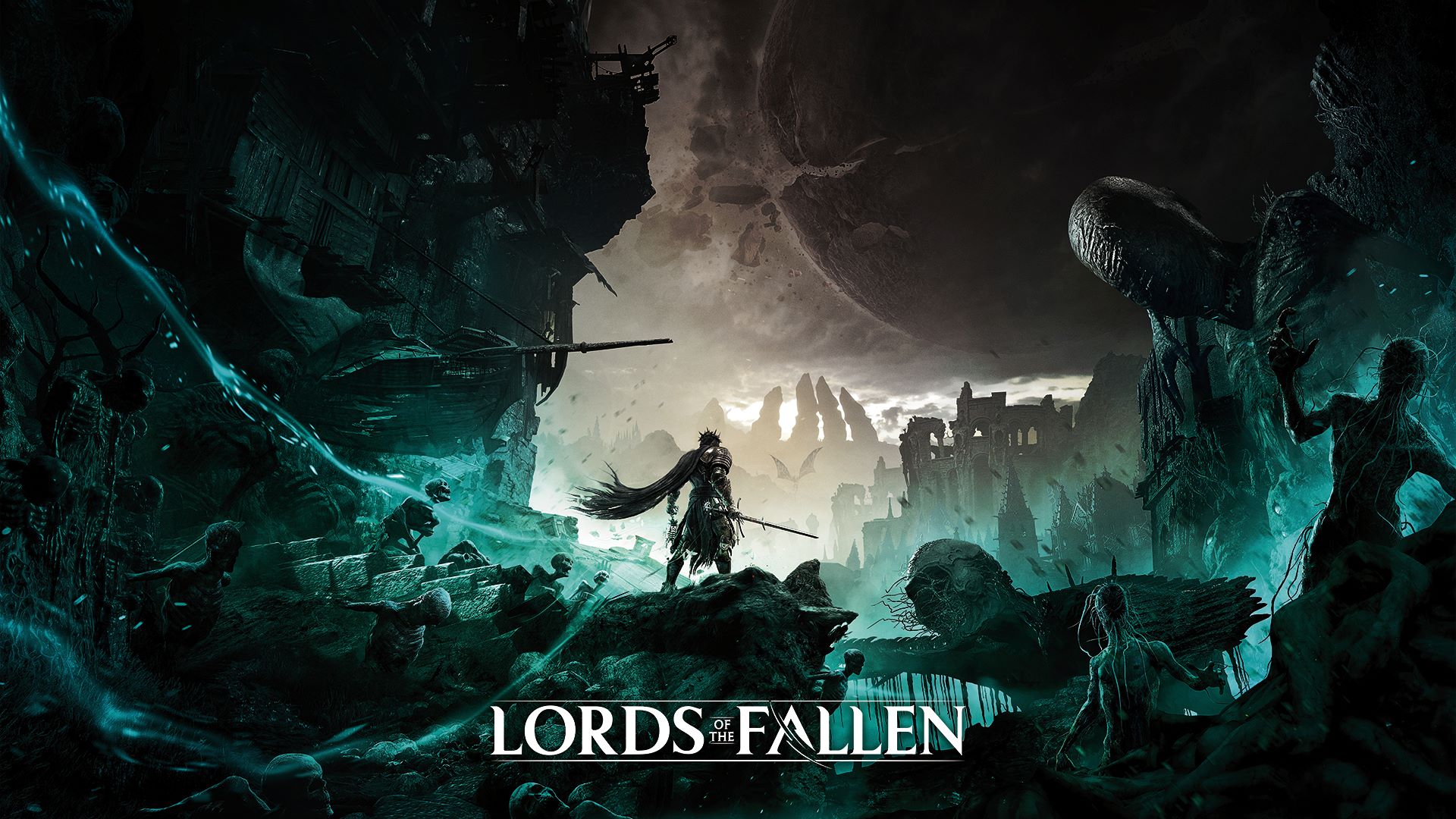
Firework (PC) – June 4 An accidental fire at a funeral forces the police to re-investigate the closed case of a massacre. You will play as a rookie police officer who participates in the re-investigation by chance. As the investigation goes deeper, the past of the victims gradually emerges and the case becomes more bewildering.
Rolling Hills (Cloud, Console, and PC) – June 4 Available on day one with Game Pass! Serve up sushi as a robot chef in Rolling Hills , a life sim about running your own restaurant in a cozy village. Make new friends, purchase ingredients, enhance your shop, and improve the lives of your neighbors as you perfect your craft!
DLC / Game Updates
Vampire Survivors : Operation Guns – Available now Game Pass members save 10% off their purchase! Vampire Survivors : Operation Guns sees the smash hit roguelike join forces with Konami’s iconic Contra series, adding tons of guns and other weapons (we’re talking 22, including evolutions!), 11 new characters, a huge map, and a soundtrack that combines classic Contra tracks with Vampire Survivors covers.
Minecraft 15 Year Anniversary – Starting May 15 Minecraft is celebrating 15 years of gripping adventures and mind-blowing creations! Whether you’ve been crafting since alpha or joined somewhere along the way, you’ve left your mark on every block. Watch out for upcoming announcements from Minecraft!
Starfield Update – May 15 Starfield’s largest update since launch is coming on May 15! The May Update includes more detailed surface maps; new gameplay difficulty options and display settings; new features for ship customization and more! Learn more on Bethesda.net .
Xbox Game Pass Ultimate
Minecraft : 500 Minecoins – Starting May 15 Claim 500 Minecoins! Spend them on epic adventure maps, skins, add-ons, and more imaginative content, all crafted by creators and available on Minecraft Marketplace. What will your next adventure be?
Naraka: Bladepoint – Available Now Claim exclusive Xbox headgear, new season treasures, legendary skin trial bundle and more in the new Perks Bundle! Get a kick start in the new season with the help of experience boost cards! This Perk content requires Naraka: Bladepoint to use.
Leaving May 31
The following games are leaving soon, so be sure to jump back in and tie up and loose ends or grab some extra achievements before they leave! You can also save up to 20% off your purchase to keep them in your library and keep the fun going.
- Chicory: A Colorful Tale (Cloud, Console, and PC)
- Farworld Pioneers (Cloud, Console, and PC)
- JoJo’s Bizarre Adventure: All-Star Battle (Cloud, Console, and PC)
- Pac-man Museum Plus (Cloud, Console, and PC)
- Little Witch in the Woods (Cloud, Console, and PC)
- Railway Empire II (Cloud, Console, and PC)
That’s the list for now, but you can always keep an eye on Xbox , Game Pass , and PC Game Pas s for reminders on when all of these are available to play. Also, don’t forget to block your calendar for the Xbox Games Showcase followed by [REDACTED] Direct June 9 – we’ll have even more news for you then. Talk soon!
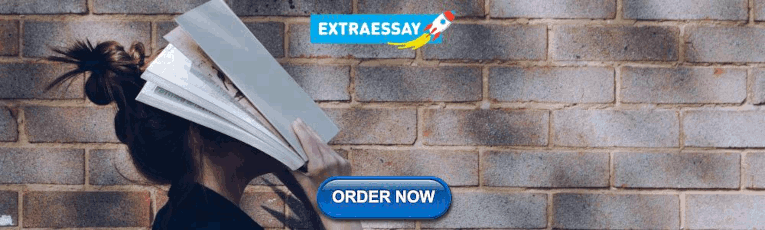
IMAGES
VIDEO
COMMENTS
Skip list is data structure that possesses the concept of the expressway in terms of basic operation like insertion, deletion and searching. ... This presentation covers the essential parameters of Unit 2 Operations Processes of the subject Operations & Supply Chain Management. Topics Covered: Volume Variety and Flow. ...
INTRODUCTION: A skip list is a data structure that allows for efficient search, insertion and deletion of elements in a sorted list. It is a probabilistic data structure, meaning that its average time complexity is determined through a probabilistic analysis. In a skip list, elements are organized in layers, with each layer having a smaller ...
Data Structures and Algorithms Skip List. Department of Computer Science. Data Structures and Algorithms. CS 225 GCarlEvans April 26, 2024. Skip List. Slides by Brad Solomon. Learning Objectives. Conceptualize and code core functions Discuss efficiency of skip list Motivate and introduce the skip list ADT. Linked List with 'Checkpoints'.
Similarly, we'll assume that the list is a data structure with two attributes: - the current maximal level of the list. - the array of pointers to the heads at the levels. 4. How to Insert Into a Skip List. To insert a node with value into a skip list, we have to do three things: find where to place it.
Skip Lists Outline and Reading What is a skip list (§9.4) Operations (§9.4.1) Search Insertion Deletion Implementation Analysis (§9.4.2) Space usage Search and update times What is a Skip List A skip list for a set S of distinct (key, element) items is a series of lists S0, S1 , … , Sh such that Each list Si contains the special keys + and - List S0 contains the keys of S in non ...
Presentation for use with the textbook, Algorithm Design and Applications, by M. T. Goodrich and R. Tamassia, Wiley, 2015 . Skip Lists 2 What is a Skip List q A skip list for a set S of distinct (key, element) items is a series of lists S 0, S 1
Handout 17: Lecture Notes on Skip Lists 5 Analysis: Proof of Theorem • Cool idea: Analyze search backwards—from leaf to root - Search starts at leaf (element in bottommost level) - At each node visited: If node wasn't promoted higher (got TAILS here), then we go [came from] left If node was promoted higher (got HEADS here), then we go [came from] up
In computer science, a skip list (or skiplist) is a probabilistic data structure that allows () average complexity for search as well as () average complexity for insertion within an ordered sequence of elements. Thus it can get the best features of a sorted array (for searching) while maintaining a linked list-like structure that allows insertion, which is not possible with a static array.
Insert & Delete • Insert & delete might need to rearrange the entire list • Like Perfect Binary Search Trees, Perfect Skip Lists are too structured to support efficient updates. • Idea:-Relax the requirement that each level have exactly half the items of the previous level-Instead: design structure so that we expect 1/2 the items to be carried up to the next level
In this video, we will talk about an advanced data structure called Skip List. It is a very useful data structure and often interview questions revolve appli...
Where your run fits in O(n) - O(log n) depends on the order of the data. Hard to keep balanced. 2-3 trees. Guaranteed to be balanced. Complicated to implement. Skip List Still no guarantee to be O(log n) for insertion, search, deletion, in every situation. Will give O(log n) performance with high probability (in most runs).
Presentation on theme: "Skip List: formally A skip list for a set S of distinct (key, element) items is a series of lists S0, S1 , … , Sh such that Each list Si contains the special."— ... 4 Skip List: Search We search for a key x as follows: We start at the first position of the top list At the current position p, we compare x with y key ...
Skip Lists ( Part 2 ) : https://www.youtube.com/watch?v=RigE4QjdNks In this video, we will learn many things about Skip Lists:i) Why do we need Skip Lists?...
Presentation on theme: "Skip List: Implementation"— Presentation transcript: ... 6 Performance of Skip Lists In a skip list with n items The expected space used is proportional to n. The expected search, insertion and deletion time is proportional to log n. Skip lists are fast and simple to implement in practice ...
SKIP LIST & SKIP GRAPH. SKIP LIST & SKIP GRAPH. James Aspnes Gauri Shah Many slides have been taken from the original presentation by the authors. Definition of Skip List. A skip list for a set L of distinct (key, element) items is a series of linked lists L 0 , L 1 , … , L h such that. 598 views • 40 slides
Advantages Skip List representation of a dictionary: Simpler to Implement: Compared to balanced trees, skip lists are easier to implement while still providing efficient search operations. Amortized Costs: Operations have good amortized time costs, making them suitable for applications with a mix of frequent reads and occasional writes. Lock-Free Concurrency: Skip lists can be more easily ...
A skip list is a data structure for dictionaries that uses a randomized insertion algorithm In a skip list with n items The expected space used is O (n) The expected search, insertion and deletion time is O (log n) Using a more complex probabilistic analysis, one can show that these performance bounds also hold with high probability Skip lists ...
What is a Skip List A skip list for a set S of distinct (key, element) items is a series of lists S0, S1 , … , Sh such that Each list Si contains the special keys + and - List S0 contains the keys of S in nondecreasing order Each list is a subsequence of the previous one, i.e., S0 S1 … Sh List Sh contains only the two special keys We show how to use a skip list to implement the dictionary ...
A skip list is a probabilistic data structure. The skip list is used to store a sorted list of elements or data with a linked list. It allows the process of the elements or data to view efficiently. In one single step, it skips several elements of the entire list, which is why it is known as a skip list. The skip list is an extended version of ...
Consider a skip list with n items. By Fact 1, we insert an item in list Si with. probability 1/2i. By Fact 2, the expected size of list Si is n/2i. The expected number of nodes used by the skip. list is. The space used by a skip list depends on the. random bits used by each invocation of the.
Here's what to know about the lawsuit and what landed Live Nation in hot water. What is Live Nation accused of? The lawsuit alleges Live Nation "engaged in numerous forms of anticompetitive ...
The content included in this presentation is intended for informational purposes only relating to the Preliminary List of Potential National Interest Electric Transmission Corridors (Preliminary List). Any content within this presentation that appears discrepant from the Preliminary List is superseded by the Preliminary List language.
Here, we have made a list of the top five business model powerpoint templates of 2024 that are reshaping the way businesses conceptualize, plan, and execute their ventures. 1. Business Model Canvas Template. One of the top business model presentation templates 2024 is Business Model Canvas powerpoint template.
Here are some features that make Visme stand out from other Canva alternatives: Advanced Data Visualization Tools. Whether you're looking to visualize complex data or effectively communicate insights, Visme's data visualization tool has everything you need. The tool is packed with over 40 charts and graphs, customizable flowcharts, an interactive map builder, an easy-to-use table maker and ...
We're excited to introduce the ability for anyone to create custom copilots from SharePoint.In just a few clicks, you— whether an admin or business user— can create and share a copilot from SharePoint that's grounded in the curated, authoritative content you choose.
10 Space Usage Consider a skip list with n items By Fact 1, we insert an item in list Si with probability 1/2i By Fact 2, the expected size of list Si is n/2i The expected number of nodes used by the skip list is The space used by a skip list depends on the random bits used by each invocation of the insertion algorithm We use the following two basic probabilistic facts: Fact 1: The probability ...
In a set of widely covered allegations, Jones said in the lawsuit that Combs regularly hosted "sex-trafficking parties" with underage women and illegal drugs, and implies record label ...
These colleges offer students a top-notch education and they come at a steep discount compared to universities like Harvard and Penn, Forbes reports.
Immortals of Aveum is coming to The Play List! Summon your power with PC Game Pass and Ultimate via EA Play in this single-player, first-person magic shooter. Unleash an arsenal of spells as Jak, who joins an elite order of battlemages to save a world on the edge of abyss.