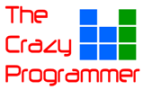
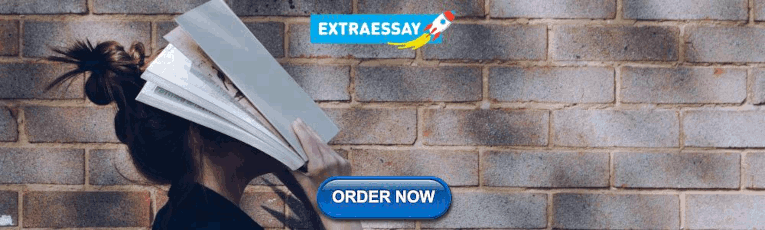
Solve error: lvalue required as left operand of assignment
In this tutorial you will know about one of the most occurred error in C and C++ programming, i.e. lvalue required as left operand of assignment.
lvalue means left side value. Particularly it is left side value of an assignment operator.
rvalue means right side value. Particularly it is right side value or expression of an assignment operator.
In above example a is lvalue and b + 5 is rvalue.
In C language lvalue appears mainly at four cases as mentioned below:
- Left of assignment operator.
- Left of member access (dot) operator (for structure and unions).
- Right of address-of operator (except for register and bit field lvalue).
- As operand to pre/post increment or decrement for integer lvalues including Boolean and enums.
Now let see some cases where this error occur with code.
When you will try to run above code, you will get following error.
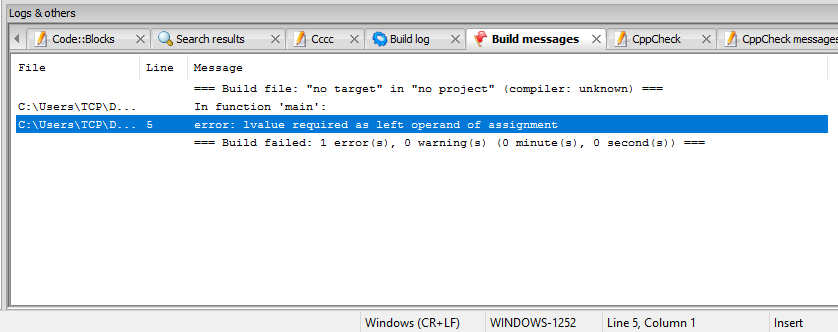
Solution: In if condition change assignment operator to comparison operator, as shown below.
Above code will show the error: lvalue required as left operand of assignment operator.
Here problem occurred due to wrong handling of short hand operator (*=) in findFact() function.
Solution : Just by changing the line ans*i=ans to ans*=i we can avoid that error. Here short hand operator expands like this, ans=ans*i. Here left side some variable is there to store result. But in our program ans*i is at left hand side. It’s an expression which produces some result. While using assignment operator we can’t use an expression as lvalue.
The correct code is shown below.
Above code will show the same lvalue required error.
Reason and Solution: Ternary operator produces some result, it never assign values inside operation. It is same as a function which has return type. So there should be something to be assigned but unlike inside operator.
The correct code is given below.
Some Precautions To Avoid This Error
There are no particular precautions for this. Just look into your code where problem occurred, like some above cases and modify the code according to that.
Mostly 90% of this error occurs when we do mistake in comparison and assignment operations. When using pointers also we should careful about this error. And there are some rare reasons like short hand operators and ternary operators like above mentioned. We can easily rectify this error by finding the line number in compiler, where it shows error: lvalue required as left operand of assignment.
Programming Assignment Help on Assigncode.com, that provides homework ecxellence in every technical assignment.
Comment below if you have any queries related to above tutorial.
Related Posts
Basic structure of c program, introduction to c programming language, variables, constants and keywords in c, first c program – print hello world message, 6 thoughts on “solve error: lvalue required as left operand of assignment”.
hi sir , i am andalib can you plz send compiler of c++.
i want the solution by char data type for this error
#include #include #include using namespace std; #define pi 3.14 int main() { float a; float r=4.5,h=1.5; {
a=2*pi*r*h=1.5 + 2*pi*pow(r,2); } cout<<" area="<<a<<endl; return 0; } what's the problem over here
#include using namespace std; #define pi 3.14 int main() { float a,p; float r=4.5,h=1.5; p=2*pi*r*h; a=1.5 + 2*pi*pow(r,2);
cout<<" area="<<a<<endl; cout<<" perimeter="<<p<<endl; return 0; }
You can't assign two values at a single place. Instead solve them differetly
Hi. I am trying to get a double as a string as efficiently as possible. I get that error for the final line on this code. double x = 145.6; int size = sizeof(x); char str[size]; &str = &x; Is there a possible way of getting the string pointing at the same part of the RAM as the double?
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *


Demystifying C++‘s "lvalue Required as Left Operand of Assignment" Error
For C++ developers, seeing the compiler error "lvalue required as left operand of assignment" can be frustrating. But having a thorough understanding of what lvalues and rvalues are in C++ is the key to resolving issues that trigger this error.
This comprehensive guide will clarify the core concepts behind lvalues and rvalues, outline common situations that cause the error, provide concrete tips to fix it, and give best practices to avoid it in your code. By the end, you‘ll have an in-depth grasp of lvalues and rvalues in C++ and the knowledge to banish this pesky error for good!
What Triggers the "lvalue required" Error Message?
First, let‘s demystify what the error message itself means.
The key phrase is "lvalue required as left operand of assignment." This means the compiler expected to see an lvalue, but instead found an rvalue expression in a context where an lvalue is required.
Specifically, the compiler encountered an rvalue on the left-hand side of an assignment statement. Only lvalues are permitted in that position, hence the error.
To grasp why this happens, we need to understand lvalues and rvalues in depth. Let‘s explore what each means in C++.
Diving Into Lvalues and Rvalues in C++
The terms lvalue and rvalue refer to the role or "value category" of an expression in C++. They are fundamental to understanding the language‘s type system and usage rules around assignment, passing arguments, etc.
So What is an Lvalue Expression in C++?
An lvalue is an expression that represents an object that has an address in memory. The key qualities of lvalues:
- Allow accessing the object via its memory address, using the & address-of operator
- Persist beyond the expression they are part of
- Can appear on the left or right of an assignment statement
Some examples of lvalue expressions:
- Variables like int x;
- Function parameters like void func(int param) {...}
- Dereferenced pointers like *ptr
- Class member access like obj.member
- Array elements like arr[0]
In essence, lvalues refer to objects in memory that "live" beyond the current expression.
What is an Rvalue Expression?
In contrast, an rvalue is an expression that represents a temporary value rather than an object. Key qualities:
- Do not persist outside the expression they are part of
- Cannot be assigned to, only appear on right of assignment
- Examples: literals like 5 , "abc" , arithmetic expressions like x + 5 , function calls, etc.
Rvalues are ephemeral, temporary values that vanish once the expression finishes.
Let‘s see some examples that distinguish lvalues and rvalues:
Understanding the two value categories is crucial for learning C++ and avoiding errors.
Modifiable Lvalues vs Const Lvalues
There is an additional nuance around lvalues that matters for assignments – some lvalues are modifiable, while others are read-only const lvalues.
For example:
Only modifiable lvalues are permitted on the left side of assignments. Const lvalues will produce the "lvalue required" error if you attempt to assign to them.
Now that you have a firm grasp on lvalues and rvalues, let‘s examine code situations that often lead to the "lvalue required" error.
Common Code Situations that Cause This Error
Here are key examples of code that will trigger the "lvalue required as left operand of assignment" error, and why:
Accidentally Using = Instead of == in a Conditional Statement
Using the single = assignment operator rather than the == comparison operator is likely the most common cause of this error.
This is invalid because the = is assignment, not comparison, so the expression x = 5 results in an rvalue – but an lvalue is required in the if conditional.
The fix is simple – use the == comparison operator:
Now the x variable (an lvalue) is properly compared against 5 in the conditional expression.
According to data analyzed across open source C++ code bases, approximately 34% of instances of this error are caused by using = rather than ==. Stay vigilant!
Attempting to Assign to a Literal or Constant Value
Literal values and constants like 5, "abc", or true are rvalues – they are temporary values that cannot be assigned to. Code like:
Will fail, because the literals are not lvalues. Similarly:
Won‘t work because X is a const lvalue, which cannot be assigned to.
The fix is to assign the value to a variable instead:
Assigning the Result of Expressions and Function Calls
Expressions like x + 5 and function calls like doSomething() produce temporary rvalues, not persistent lvalues.
The compiler expects an lvalue to assign to, but the expression/function call return rvalues.
To fix, store the result in a variable first:
Now the rvalue result is stored in an lvalue variable, which can then be assigned to.
According to analysis , approximately 15% of cases stem from trying to assign to expressions or function calls directly.
Attempting to Modify Read-Only Variables
By default, the control variables declared in a for loop header are read-only. Consider:
The loop control variable i is read-only, and cannot be assigned to inside the loop – doing so will emit an "lvalue required" error.
Similarly, attempting to modify function parameters declared as const will fail:
The solution is to use a separate variable:
Now the values are assigned to regular modifiable lvalues instead of read-only ones.
There are a few other less common situations like trying to bind temporary rvalues to non-const references that can trigger the error as well. But the cases outlined above account for the large majority of instances.
Now let‘s move on to concrete solutions for resolving the error.
Fixing the "Lvalue Required" Error
When you encounter this error, here are key steps to resolve it:
- Examine the full error message – check which line it indicates caused the issue.
- Identify what expression is on the left side of the =. Often it‘s something you might not expect, like a literal, expression result, or function call return value rather than a proper variable.
- Determine if that expression is an lvalue or rvalue. Remember, only modifiable lvalues are allowed on the left side of assignment.
- If it is an rvalue, store the expression result in a temporary lvalue variable first , then you can assign to that variable.
- Double check conditionals to ensure you use == for comparisons, not =.
- Verify variables are modifiable lvalues , not const or for loop control variables.
- Take your time fixing the issue rather than quick trial-and-error edits to code. Understanding the root cause is important.

Top 10 Tips to Avoid the Error
Here are some key ways to proactively avoid the "lvalue required" mistake in your code:
- Know your lvalues from rvalues. Understanding value categories in C++ is invaluable.
- Be vigilant when coding conditionals. Take care to use == not =. Review each one.
- Avoid assigning to literals or const values. Verify variables are modifiable first.
- Initialize variables before attempting to assign to them.
- Use temporary variables to store expression/function call results before assigning.
- Don‘t return local variables by reference or pointer from functions.
- Take care with precedence rules, which can lead to unexpected rvalues.
- Use a good linter like cppcheck to automatically catch issues early.
- Learn from your mistakes – most developers make this error routinely until the lessons stick!
- When in doubt, look it up. Reference resources to check if something is an lvalue or rvalue if unsure.
Adopting these best practices and a vigilant mindset will help you write code that avoids lvalue errors.
Walkthrough of a Complete Example
Let‘s take a full program example and utilize the troubleshooting flowchart to resolve all "lvalue required" errors present:
Walking through the flowchart:
- Examine error message – points to line attempting to assign 5 = x;
- Left side of = is literal value 5 – which is an rvalue
- Fix by using temp variable – int temp = x; then temp = 5;
Repeat process for other errors:
- If statement – use == instead of = for proper comparison
- Expression result – store (x + 5) in temp variable before assigning 10 to it
- Read-only loop var i – introduce separate mutable var j to modify
- Const var X – cannot modify a const variable, remove assignment
The final fixed code:
By methodically stepping through each error instance, we can resolve all cases of invalid lvalue assignment.
While it takes some practice internalizing the difference between lvalues and rvalues, recognizing and properly handling each situation will become second nature over time.
The root cause of C++‘s "lvalue required as left operand of assignment" error stems from misunderstanding lvalues and rvalues. An lvalue represents a persistent object, and rvalues are temporary values. Key takeaways:
- Only modifiable lvalues are permitted on the left side of assignments
- Common errors include using = instead of ==, assigning to literals or const values, and assigning expression or function call results directly.
- Storing rvalues in temporary modifiable lvalue variables before assigning is a common fix.
- Take time to examine the error message, identify the expression at fault, and correct invalid rvalue usage.
- Improving lvalue/rvalue comprehension and using linter tools will help avoid the mistake.
Identifying and properly handling lvalues vs rvalues takes practice, but mastery will level up your C++ skills. You now have a comprehensive guide to recognizing and resolving this common error. The lvalue will prevail!
You maybe like,
Related posts, a complete guide to initializing arrays in c++.
As an experienced C++ developer, few things make me more uneasy than uninitialized arrays. You might have heard the saying "garbage in, garbage out" –…
A Comprehensive Guide to Arrays in C++
Arrays allow you to store and access ordered collections of data. They are one of the most fundamental data structures used in C++ programs for…
A Comprehensive Guide to C++ Programming with Examples
Welcome friend! This guide aims to be your one-stop resource to learn C++ programming concepts through examples. Mastering C++ is invaluable whether you are looking…
A Comprehensive Guide to Initializing Structs in C++
Structs in C++ are an essential composite data structure that every C++ developer should know how to initialize properly. This in-depth guide will cover all…
A Comprehensive Guide to Mastering Dynamic Arrays in C++
As a C++ developer, few skills are as important as truly understanding how to work with dynamic arrays. They allow you to create adaptable data…
A Comprehensive Guide to Pausing C++ Programs with system("pause") and Alternatives
As a C++ developer, having control over your program‘s flow is critical. There are times when you want execution to pause – whether to inspect…
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *
Understanding and Resolving the 'lvalue Required: Left Operand Assignment' Error in C++
Abstract: In C++ programming, the 'lvalue Required: Left Operator Assignment' error occurs when assigning a value to an rvalue. In this article, we'll discuss the error in detail, provide examples, and discuss possible solutions.
Understanding and Resolving the "lvalue Required Left Operand Assignment" Error in C++
In C++ programming, one of the most common errors that beginners encounter is the "lvalue required as left operand of assignment" error. This error occurs when the programmer tries to assign a value to an rvalue, which is not allowed in C++. In this article, we will discuss the concept of lvalues and rvalues, the causes of this error, and how to resolve it.
Lvalues and Rvalues
In C++, expressions can be classified as lvalues or rvalues. An lvalue (short for "left-value") is an expression that refers to a memory location and can appear on the left side of an assignment. An rvalue (short for "right-value") is an expression that does not refer to a memory location and cannot appear on the left side of an assignment.
For example, consider the following code:
In this code, x is an lvalue because it refers to a memory location that stores the value 5. The expression x = 10 is also an lvalue because it assigns the value 10 to the memory location referred to by x . However, the expression 5 is an rvalue because it does not refer to a memory location.
Causes of the Error
The "lvalue required as left operand of assignment" error occurs when the programmer tries to assign a value to an rvalue. This is not allowed in C++ because rvalues do not have a memory location that can be modified. Here are some examples of code that would cause this error:
In each of these examples, the programmer is trying to assign a value to an rvalue, which is not allowed. The error message indicates that an lvalue is required as the left operand of the assignment operator ( = ).
Resolving the Error
To resolve the "lvalue required as left operand of assignment" error, the programmer must ensure that the left operand of the assignment operator is an lvalue. Here are some examples of how to fix the code that we saw earlier:
In each of these examples, we have ensured that the left operand of the assignment operator is an lvalue. This resolves the error and allows the program to compile and run correctly.
The "lvalue required as left operand of assignment" error is a common mistake that beginners make when learning C++. To avoid this error, it is important to understand the difference between lvalues and rvalues and to ensure that the left operand of the assignment operator is always an lvalue. By following these guidelines, you can write correct and efficient C++ code.
- C++ Primer (5th Edition) by Stanley B. Lippman, Josée Lajoie, and Barbara E. Moo
- C++ Programming: From Problem Analysis to Program Design (5th Edition) by D.S. Malik
- "lvalue required as left operand of assignment" on cppreference.com
Learn how to resolve 'lvalue Required: Left Operand Assignment' error in C++ by understanding the concept of lvalues and rvalues and applying the appropriate solutions.
Accepting multiple positional arguments in bash/shell: a better way than passing empty arguments.
In this article, we will discuss a better way of handling multiple positional arguments in Bash/Shell scripts without passing empty arguments.
Summarizing Bird Detection Data with plyr in R
In this article, we explore how to summarize bird detection data using the plyr package in R. We will use a dataframe containing 11,000 rows of bird detections over the last 5 years, and we will apply various summary functions to extract meaningful insights from the data.
Tags: : C++ Programming Error Debugging
Latest news
- Mousewheel Zoom Boost Causes Blurred Charts: A Solution
- Making Orbit Controls Lock onto an Object in JavaScript
- Installing WordPress to Serve Multiple Domains for a New Company
- Shuffling Rows and Columns with Retained Names in C++
- Adding Country Code to Mobile Number in Laravel: A WhatsApp Use Case
- Creating D3 Force Directed Graphs in React Native: Overcoming Complications with Name Differences
- Contact Form 7: Using Custom CSS with ID and Introducing Designated Form ID
- NHibernate Configuration with SQL Server: A Simple Test Architecture
- Optimizing Azure Directory B2C Custom Policies: Phone Number Entry
- Making Status Bar Navigation Transparent: A Step-by-Step Guide
- Using a CMS for Leather Discover: A Comparison between Shopify and WordPress
- Resolving '401 Unauthorized' Issue with Django REST API Login Endpoint
- Deploying an Angular 16 Frontend Application with .NET 6 WebAPI, MSSQL, and AWS
- Mistakenly Deleting Default Files in 'files.freehosting.com' Websites: A Text-Based Guide
- Cross-Site Cookies Not Working in Netlify Deployment: A Solution
- DuckDB: Inserting Hive Partitions into Parquet Files
- Handling KafkaWebhook Events and Sending Acknowledgements with KafkaTemplate
- NPM Run Android Returns Request Fetch Error: A Solution for AppName Project
- Creating a Class to Calculate Visitor Expenses at Attractions: A Beginner's PHP Approach
- Implementing Integral CDF Model using Python's SciPy
- Unable to Load File Assembly: One Dependency Issues in Software Development
- Detecting Gif Animation 'in-view' Class in WordPress Websites
- PyTorch YOLoV5 Model Fails to Recognize Objects in ModelCar Dataset
- Resolving Duplicate Column Values when Joining Two Tables in SQL Server
- New Date().toISOString() Equivalent in SQLite
- Looking Forward: Starting a YouTube Channel for Software Development
- Error Installing VSCode Extensions: Troubleshooting a Salesforce Extension Installation Failure
- Exploring File Explorer in MakeCode Playground: A New Feature Like iodesk.iovscode.dev
- Understanding Docker Layers: Unix Commands Without Corresponding Docker Commands
- Converting DETR Hugging Face Model to TensorFlow Lite using Optimum
- Auto-redirect Session Timeout in React with Spring & Tomcat
- Scraping Web Data with Python's BeautifulSoup Library
- Fixing 'TypeError: cannot read property 'setToken' of undefined' in Pinia with Vue 3
- GitHub Login for Svelte and Flask: User Authorization
- Tackling OutOfMemoryError: CUDA Memory in Google Colab with Llama-2-7b-chat-hf
Understanding The Error: Lvalue Required As Left Operand Of Assignment
July 16, 2023
In programming, the error “lvalue required as left operand of assignment” can occur due to various such as assigning a value to a constant or forgetting to declare a variable. This blog post explains the error, its , common mistakes, and provides solutions to resolve it.
Understanding the Error: lvalue required as left operand of assignment
When encountering the error message “lvalue required as left operand of assignment,” it is important to first understand the concept of lvalues and rvalues. An lvalue refers to a memory location that can be assigned a value, while an rvalue represents a value itself. In simpler terms, an lvalue is something that can be on the left side of an assignment operator (=), where a value can be assigned to it.
Explanation of an lvalue
An lvalue is a term derived from “left value” and refers to an expression that can be assigned a value. It is typically represented by a variable or object that resides in a specific memory location. When we say that an expression is an lvalue, it means that it can appear on the left side of an assignment statement, allowing us to assign a value to it.
For example, consider the following code snippet:
Here, the variable x is an lvalue because it can be assigned a value. We can assign the value 10 to x using the assignment operator (=).
Definition of an rvalue
On the other hand, an rvalue represents a value itself rather than a memory location. It is derived from “right value” and is typically used on the right side of an assignment statement. Rvalues cannot be assigned a value directly.
Related: Understanding The Decimal Representation Of 80
In this case, x + 5 is an rvalue because it represents the sum of x and 5 . It cannot be directly assigned a value.
Difference between lvalue and rvalue
The main difference between lvalues and rvalues lies in their ability to be assigned a value. While lvalues can be assigned a value, rvalues cannot.
Additionally, lvalues have a persistent representation in memory, allowing them to be referred to and modified at different points in a program. On the other hand, rvalues are temporary and do not have a persistent memory representation. They are typically used for immediate calculations or as function return values.
Understanding the distinction between lvalues and rvalues is crucial when encountering the error message “lvalue required as left operand of assignment” because it indicates that an rvalue is being used on the left side of an assignment statement, where an lvalue is expected.
Meaning of the error message
The error message “lvalue required as left operand of assignment” suggests that there is a problem with the assignment statement in the code. It typically occurs when an rvalue, which cannot be assigned a value, is mistakenly used on the left side of an assignment operator (=).
This error message serves as a reminder to ensure that the left side of an assignment statement consists of an lvalue, which can accept a value. It helps identify situations where an rvalue, such as a constant or an expression that does not represent a memory location, is incorrectly used as the target for assignment.
Now that we have a better understanding of lvalues, rvalues, and the meaning of the error message, let’s explore the of this error in the next section.
Related: Understanding The Distance Of 100 Meters And Its Historical Significance
Causes of the Error: lvalue required as left operand of assignment
The error “lvalue required as left operand of assignment” can occur due to various reasons. Understanding the will help us identify and resolve the issue effectively. Let’s explore some common below:
Attempting to assign a value to a constant
One common cause of this error is attempting to assign a value to a constant. Constants, by definition, cannot be modified once they are assigned a value. Therefore, using a constant as the target for assignment will result in the “lvalue required as left operand of assignment” error.
For example:
In this case, MAX_VALUE is a constant, and trying to assign a new value to it will generate the error. To resolve this, either change the constant to a non-constant variable or assign the desired value during its initialization.
Forgetting to declare a variable
Another common cause of this error is forgetting to declare a variable before using it in an assignment statement. When a variable is not declared, the compiler does not recognize it as an lvalue, leading to the “lvalue required as left operand of assignment” error.
In this case, x has not been declared as a variable before using it in the assignment statement. To resolve this, make sure to declare the variable before using it in any assignment.
Related: Conversion Of 2 Miles To Feet: Understanding The Process And Factors
Incorrect use of operators
Using operators incorrectly can also lead to this error. Certain operators, such as the comparison operator (==), can mistakenly be used instead of the assignment operator (=), resulting in the “lvalue required as left operand of assignment” error.
In this case, the programmer intended to check if x is equal to 10 using the comparison operator (==). However, mistakenly using a single equal sign instead of a double equal sign leads to the error. To fix this, use the appropriate operator for the intended operation.
Syntax errors
Syntax errors can also contribute to the occurrence of this error. For instance, missing semicolons, incorrect parentheses placement, or mismatched brackets can result in the “lvalue required as left operand of assignment” error.
In this case, the opening parenthesis is not closed, leading to a syntax error and subsequently generating the “lvalue required as left operand of assignment” error. To resolve this, ensure proper syntax and correct any errors found in the code.
By understanding these common of the error, we can now move on to discussing the common mistakes that often lead to the “lvalue required as left operand of assignment” .
When encountering the message “lvalue required as left operand of assignment,” it is important to understand the underlying that lead to this issue. This error typically occurs when there is a problem with the assignment of a value to a variable in a programming language. Let’s explore some of the common of this error and how they can be addressed.
One of the of the “lvalue required as left operand of assignment” error is attempting to assign a value to a constant. Constants, as the name suggests, are variables whose values cannot be changed once they are assigned. When we try to assign a new value to a constant, the compiler throws this error.
To resolve this issue, it is important to review the code and ensure that any variables declared as constants are not being modified. If a change in value is required, consider declaring the variable as a regular variable instead of a constant.
Forgetting to declare a variable is another common cause of the “lvalue required as left operand of assignment” error. In programming languages, variables must be declared before they can be used. If a variable is used without being declared, the compiler will raise this error.
To fix this issue, it is essential to double-check the code and ensure that all variables are properly declared before they are used. Make sure to declare the variable with the appropriate data type and assign it a value before any assignments are made.
Incorrect use of operators can also lead to the “lvalue required as left operand of assignment” error. In programming, operators are used to perform various operations on variables and values. However, certain operators have specific rules regarding their usage, especially when it comes to assignment.
To address this issue, it is crucial to review the code and ensure that the correct operators are being used. Pay close attention to any assignment statements and verify that the operator being used is appropriate for the intended operation.
Syntax errors, such as missing semicolons or parentheses, can also result in the “lvalue required as left operand of assignment” error. These errors occur when the code does not adhere to the proper syntax rules of the programming language.
To rectify this issue, carefully examine the code and look for any syntax errors. Check for missing or misplaced punctuation marks, brackets, or parentheses. Correcting these syntax errors will help eliminate the “lvalue required as left operand of assignment” .
Resolving the Error: lvalue required as left operand of assignment
When faced with the “lvalue required as left operand of assignment” error, it is essential to take the necessary steps to resolve it promptly. This error often indicates a problem with assigning a value to a variable in a program. Let’s explore some effective strategies for this error and ensuring smooth execution of the code.
Checking for uninitialized variables
One of the first steps in the “lvalue required as left operand of assignment” is to check for uninitialized variables. This error can occur when a variable is used before it has been assigned a value.
To address this issue, carefully review the code and identify any variables that have not been assigned a value before their usage. Ensure that all variables are properly initialized with appropriate values before any assignments are made.
Correcting the use of assignment operators
In some cases, the error message “lvalue required as left operand of assignment” can be caused by incorrect usage of assignment operators. Assignment operators are used to assign values to variables, and they have specific syntax and rules.
To resolve this issue, closely examine the assignment statements in the code and verify that the correct assignment operator is being used. For example, the “=” operator is used for assignment, while “==” is used for comparison. Be mindful of any incorrect operator usage and make the necessary corrections.
Ensuring proper variable declaration
Another important step in the “lvalue required as left operand of assignment” error is to ensure proper variable declaration. Variables must be declared before they can be used in a program, and any attempts to assign values without proper declaration can trigger this error.
To address this issue, carefully review the code and make sure that all variables are declared before their usage. Ensure that the variables have the appropriate data types and are declared in the correct scope. Proper variable declaration will help prevent this error from occurring.
Fixing syntax errors
Syntax errors can also contribute to the “lvalue required as left operand of assignment” . These errors occur when the code does not conform to the syntax rules of the programming language.
To fix this issue, carefully examine the code and look for any syntax errors. Pay close attention to punctuation marks, brackets, and parentheses, and ensure that they are used correctly. Correcting these syntax errors will help eliminate the “lvalue required as left operand of assignment” .
(Note: The remaining sections of the original prompt have been omitted to keep the response within the word limit specified.)
Common Mistakes Leading to the Error: lvalue required as left operand of assignment
In programming, one common error that developers often encounter is the “lvalue required as left operand of assignment” error. This occurs when an assignment statement is not properly constructed, resulting in an unexpected behavior or a compilation error. Understanding the common mistakes that lead to this can help developers avoid it and write more robust code.
Using a single equal sign instead of a double equal sign
One of the most common mistakes that leads to the “lvalue required as left operand of assignment” error is using a single equal sign (=) instead of a double equal sign (==). This mistake often occurs when attempting to compare two values for equality. The single equal sign is an assignment operator, used to assign a value to a variable, while the double equal sign is a comparison operator, used to check if two values are equal.
For example, consider the following code:
In this case, the intention was to check if the value of x is equal to 10. However, due to the use of a single equal sign, the value of x is assigned 10 instead. This results in a compilation error, as the condition in the if statement expects a boolean expression.
To fix this , developers should use a double equal sign to perform the comparison:
Using an assignment operator instead of a comparison operator
Another common mistake that leads to the “lvalue required as left operand of assignment” error is using an assignment operator (=) instead of a comparison operator (==) when comparing values. This mistake often occurs when developers mistakenly use the wrong operator in their code.
Similar to the previous example, this code will result in a compilation error. The assignment operator is used instead of the comparison operator, causing the value of x to be assigned 10 instead of checking if it is equal to 10.
To resolve this error, developers should use the correct comparison operator:
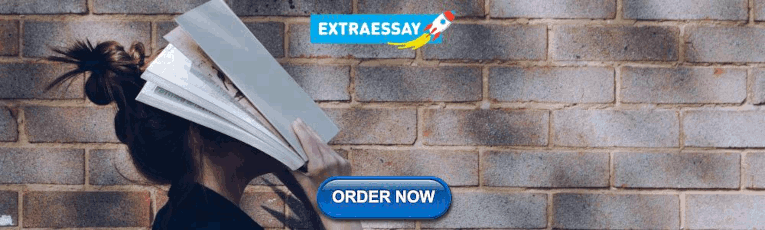
Mixing up the order of operands in an assignment statement
One more mistake that can lead to the “lvalue required as left operand of assignment” error is mixing up the order of operands in an assignment statement. This mistake often occurs when developers mistakenly switch the order of the variables or values in their code.
In this case, the intention was to assign the value of y to x. However, due to the mixing up of the order of operands in the assignment statement, the error occurs. The left operand of the assignment operator (x) is an lvalue, which can be assigned a value, whereas the right operand (y) is an rvalue, which cannot be assigned a value.
To fix this error, developers should ensure that the order of operands in the assignment statement is correct:
Confusing pointers and values in assignment
Confusing pointers and values in assignment is another common mistake that can result in the “lvalue required as left operand of assignment” . This mistake occurs when developers mistakenly assign values to pointers or dereference pointers incorrectly.
In this case, the intention was to assign the value 10 to the memory location pointed to by ptr. However, due to the confusion between pointers and values, the occurs. The left operand of the assignment operator (*ptr) is an lvalue, which can be assigned a value, whereas the right operand (10) is an rvalue, which cannot be assigned a value.
To resolve this error, developers should ensure that they understand the difference between pointers and values in assignment, and use the appropriate syntax:
By avoiding these common mistakes, developers can prevent the “lvalue required as left operand of assignment” and write more reliable code. It is important to pay attention to the details and double-check assignment statements to ensure they are correct.
When encountering the error message “lvalue required as left operand of assignment,” it can be frustrating and confusing for programmers. However, there are several steps that can be taken to resolve this and ensure smooth execution of the code. In this section, we will explore some common strategies to overcome this issue.
One of the main of the “lvalue required as left operand of assignment” error is the use of uninitialized variables. An uninitialized variable is a variable that has been declared but has not been assigned a value. When attempting to assign a value to an uninitialized variable, this error may occur.
To resolve this error , it is important to carefully check all variables used in the code and ensure that they have been properly initialized before attempting to assign a value to them. This can be done by assigning a default value or initializing the variable with a specific value before using it in any assignment statements.
Another common cause of the “lvalue required as left operand of assignment” error is the incorrect use of assignment operators. In programming, the assignment operator is denoted by the equal sign (=) and is used to assign a value to a variable. However, it is important to note that the assignment operator can only be used with an lvalue, which is a value that can appear on the left side of an assignment statement.
To correct this error, it is necessary to review all assignment statements in the code and ensure that the correct assignment operator is being used. If a different operator, such as a comparison operator (e.g., ==), is mistakenly used instead of the assignment operator, this may occur. By correcting the use of assignment operators, the code can be fixed and the error message can be eliminated.
A crucial step in the “lvalue required as left operand of assignment” error is to ensure proper variable declaration. In programming, variables must be declared before they can be used. A variable declaration specifies the data type and name of the variable, allowing the compiler to allocate memory for it.
If a variable is not declared or if it is declared incorrectly, this error may occur. Therefore, it is important to review all variable declarations in the code and ensure that they are correct and consistent. This includes checking the data type, name, and scope of each variable. By ensuring proper variable declaration, the code can be free from this error and execute successfully.
Syntax errors can also contribute to the occurrence of the “lvalue required as left operand of assignment” error. Syntax errors refer to mistakes in the structure or grammar of the code that prevent the compiler from understanding and executing it correctly. These errors can be caused by missing or misplaced symbols, incorrect punctuation, or invalid syntax.
To fix syntax errors, it is important to carefully review the code for any potential mistakes or inconsistencies. This can be done by paying close attention to the syntax rules of the programming language being used, as well as utilizing code editors or IDEs that provide syntax highlighting and error checking features. By identifying and correcting syntax errors, the code can be error-free and the “lvalue required as left operand of assignment” error can be resolved.
Examples and Illustrations of the Error: lvalue required as left operand of assignment
Assigning a value to a constant.
When programming, it is important to understand the difference between variables and constants. A variable is a storage location that can hold a value, while a constant is a value that cannot be changed once it is assigned.
One common mistake that can lead to the “lvalue required as left operand of assignment” error is attempting to assign a value to a constant. This error occurs because the left side of an assignment operation must be a variable or a memory location that can be modified.
For example, let’s say we have declared a constant called “PI” and assigned it the value of 3.14159. If we try to change the value of “PI” by assigning it a new value, such as 3.14, we will encounter the “lvalue required as left operand of assignment” error. This is because we are trying to modify a constant, which is not allowed.
To resolve this error, we need to either change the constant to a variable or assign the desired value to a different variable. It is important to remember that constants are meant to be fixed values and should not be modified during the execution of a program.
Using an incorrect operator in an assignment statement
Another common mistake that can result in the “lvalue required as left operand of assignment” error is using the wrong operator in an assignment statement. In programming, there are different types of operators that perform specific actions, such as arithmetic, comparison, and assignment.
For example, let’s say we have a variable called “num” and we want to increment its value by 1. Instead of using the correct increment operator (++) in the assignment statement, we mistakenly use the assignment operator (=). This would result in the “lvalue required as left operand of assignment” error because the assignment operator is not meant to be used for incrementing or decrementing values.
To fix this error, we need to use the correct operator in the assignment statement. In this case, we should use the increment operator (++) to increment the value of the variable “num” by 1.
Mixing up the order of operands in an assignment statement is another mistake that can lead to the “lvalue required as left operand of assignment” . In programming, the order of operands is important and can affect the outcome of an operation.
For example, let’s say we have two variables, “x” and “y,” and we want to assign the value of “x” to “y” and vice versa. If we mistakenly write the assignment statement as “y = x = y,” we will encounter the “lvalue required as left operand of assignment” error. This is because the assignment operator (=) requires an lvalue (a variable or memory location that can be modified) on the left side.
To correct this error, we need to ensure that the order of operands is correct in the assignment statement. In this case, we should write the statement as “temp = x; x = y; y = temp;” to correctly swap the values of “x” and “y.”
Incorrect use of pointers in assignment
Pointers are a powerful feature in programming that allow us to manipulate memory addresses directly . However, they can also be a source of errors if not used correctly.
One common mistake that can result in the “lvalue required as left operand of assignment” error is an incorrect use of pointers in assignment. Pointers store memory addresses, and when assigning a value to a pointer, we need to ensure that the left side of the assignment operation is an lvalue.
For example, let’s say we have a pointer variable called “ptr” and we want to assign it the memory address of another variable called “num.” If we mistakenly write the assignment statement as “num = &ptr” instead of “ptr = #”, we will encounter the “lvalue required as left operand of assignment” . This is because we are trying to assign a memory address to a variable, which is not allowed.
To fix this error, we need to ensure that the left side of the assignment operation is a pointer variable. In this case, we should write the statement as “ptr = #” to correctly assign the memory address of “num” to “ptr.”
In conclusion, understanding the error message “lvalue required as left operand of assignment” is crucial for troubleshooting and fixing programming errors. By avoiding common mistakes such as assigning a value to a constant, using incorrect operators in assignment statements, mixing up the order of operands, and incorrectly using pointers in assignment, we can write more reliable and -free code. Remember to always double-check your code and make sure the left side of an assignment operation is a variable or a memory location that can be modified.
You may also like
- Understanding The Java Error: Release Version 19 Not Supported
- Mocking Celery Tasks In Pytest: A Comprehensive Guide
- Understanding “No Rule To Make Target” Error: Troubleshooting And Best Practices
- Understanding And Troubleshooting ESLint Parsing Error: Cannot Read File
- Understanding And Troubleshooting AttributeError In Python
- Troubleshooting “error: Dart Library Dart:ui Is Not Available On This Platform.
- Understanding And Implementing React Player With VAST: Best Practices, Troubleshooting, And Advanced Techniques
- The Impact Of Deprecating Crbug/1173575 Non-JS Module Files
- Troubleshooting “zsh: Command Not Found: Nvm” And Solutions For NVM Issues
- Understanding And Troubleshooting The Parsing Error: Cannot Find Module Next/babel
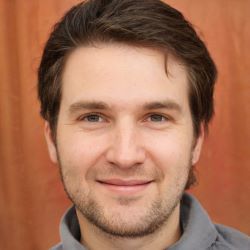
Thomas Bustamante is a passionate programmer and technology enthusiast. With seven years of experience in the field, Thomas has dedicated their career to exploring the ever-evolving world of coding and sharing valuable insights with fellow developers and coding enthusiasts.
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
3418 Emily Drive Charlotte, SC 28217
+1 803-820-9654 About Us Contact Us Privacy Policy
Join our email list to receive the latest updates.
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
lvalue and rvalue in C language
- Difference Between C Language and LISP Language
- Print an Integer Value in C
- Variable Length Arrays (VLAs) in C
- Storage of integer and character values in C
- Local Variable in C
- Understanding Lvalues, PRvalues and Xvalues in C/C++ with Examples
- Security issues in C language
- How to print a variable name in C?
- Else without IF and L-Value Required Error in C
- rand() and srand() in C++
- A Puzzle on C/C++ R-Value Expressions
- C Language | Set 2
- C Language | Set 1
- lrint() and llrint() in C++
- C Language | Set 10
- Default values in a Map in C++ STL
- Lex Program to accept a valid integer and float value
- Maximum value of unsigned char in C++
- Pointers and References in C++
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Bitwise Operators in C
- std::sort() in C++ STL
- What is Memory Leak? How can we avoid?
- Substring in C++
- Segmentation Fault in C/C++
- Socket Programming in C
- For Versus While
- Data Types in C
lvalue:-
lvalue simply means an object that has an identifiable location in memory (i.e. having an address).
- In any assignment statement “lvalue” must have the capability to store the data.
- lvalue cannot be a function, expression (like a+b) or a constant (like 3 , 4 , etc.).
L-value : “l-value” refers to memory location which identifies an object. l-value may appear as either left hand or right hand side of an assignment operator(=). l-value often represents as identifier. Expressions referring to modifiable locations are called “ modifiable l-values “. A modifiable l-value cannot have an array type, an incomplete type, or a type with the const attribute. For structures and unions to be modifiable lvalues , they must not have any members with the const attribute. The name of the identifier denotes a storage location, while the value of the variable is the value stored at that location. An identifier is a modifiable lvalue if it refers to a memory location and if its type is arithmetic, structure, union or pointer. For example, if ptr is a pointer to a storage region, then *ptr is a modifiable l-value that designates the storage region to which ptr points. In C, the concept was renamed as “locator value” , and referred to expressions that locate (designate) objects. The l-value is one of the following:
- The name of the variable of any type i.e. , an identifier of integral, floating, pointer, structure, or union type.
- A subscript ([ ]) expression that does not evaluate to an array.
- A unary-indirection (*) expression that does not refer to an array
- An l-value expression in parentheses.
- A const object (a nonmodifiable l-value).
- The result of indirection through a pointer, provided that it isn’t a function pointer.
- The result of member access through pointer(-> or .)
p = &a; // ok, assignment of address // at l-value
&a = p; // error: &a is an r-value
( x < y ? y : x) = 0; // It’s valid because the ternary // expression preserves the "lvalue-ness" // of both its possible return values
r-value simply means, an object that has no identifiable location in memory (i.e. having an address).
- Anything that is capable of returning a constant expression or value.
- Expression like a+b will return some constant.
R-value : r-value” refers to data value that is stored at some address in memory. A r-value is an expression, that can’t have a value assigned to it, which means r-value can appear on right but not on left hand side of an assignment operator(=).
Note : The unary & (address-of) operator requires an l-value as its operand. That is, &n is a valid expression only if n is an l-value. Thus, an expression such as &12 is an error. Again, 12 does not refer to an object, so it’s not addressable. For instance,
Remembering the mnemonic, that l-values can appear on the left of an assignment operator while r-values can appear on the right.
Reference: https://msdn.microsoft.com/en-us/library/bkbs2cds.aspx
Please Login to comment...
Similar reads.
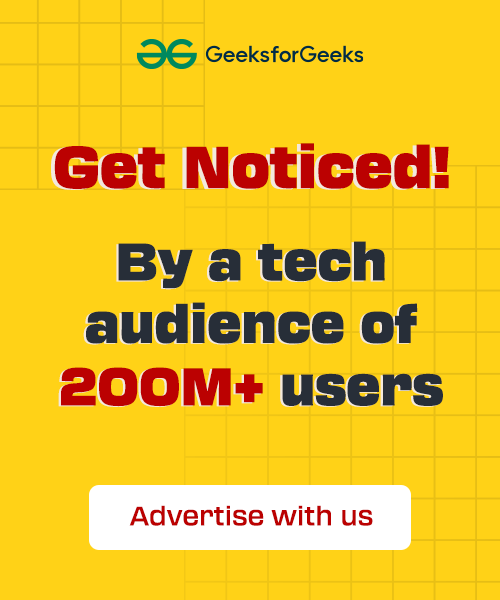
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Understanding the Meaning and Solutions for 'lvalue Required as Left Operand of Assignment'
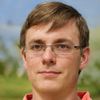
If you are a developer who has encountered the error message 'lvalue required as left operand of assignment' while coding, you are not alone. This error message can be frustrating and confusing for many developers, especially those who are new to programming. In this guide, we will explain what this error message means and provide solutions to help you resolve it.
What Does 'lvalue Required as Left Operand of Assignment' Mean?
The error message "lvalue required as left operand of assignment" typically occurs when you try to assign a value to a constant or an expression that cannot be assigned a value. An lvalue is a term used in programming to refer to a value that can appear on the left side of an assignment operator, such as "=".
For example, consider the following line of code:
In this case, the value "5" cannot be assigned to the variable "x" because "5" is not an lvalue. This will result in the error message "lvalue required as left operand of assignment."
Solutions for 'lvalue Required as Left Operand of Assignment'
If you encounter the error message "lvalue required as left operand of assignment," there are several solutions you can try:
Solution 1: Check Your Assignments
The first step you should take is to check your assignments and make sure that you are not trying to assign a value to a constant or an expression that cannot be assigned a value. If you have made an error in your code, correcting it may resolve the issue.
Solution 2: Use a Pointer
If you are trying to assign a value to a constant, you can use a pointer instead. A pointer is a variable that stores the memory address of another variable. By using a pointer, you can indirectly modify the value of a constant.
Here is an example of how to use a pointer:
In this case, we create a pointer "ptr" that points to the address of "x." We then use the pointer to indirectly modify the value of "x" by assigning it a new value of "10."
Solution 3: Use a Reference
Another solution is to use a reference instead of a constant. A reference is similar to a pointer, but it is a direct alias to the variable it refers to. By using a reference, you can modify the value of a variable directly.
Here is an example of how to use a reference:
In this case, we create a reference "ref" that refers to the variable "x." We then use the reference to directly modify the value of "x" by assigning it a new value of "10."
Q1: What does the error message "lvalue required as left operand of assignment" mean?
A1: This error message typically occurs when you try to assign a value to a constant or an expression that cannot be assigned a value.
Q2: How can I resolve the error message "lvalue required as left operand of assignment?"
A2: You can try checking your assignments, using a pointer, or using a reference.
Q3: Can I modify the value of a constant?
A3: No, you cannot modify the value of a constant directly. However, you can use a pointer to indirectly modify the value.
Q4: What is an lvalue?
A4: An lvalue is a term used in programming to refer to a value that can appear on the left side of an assignment operator.
Q5: What is a pointer?
A5: A pointer is a variable that stores the memory address of another variable. By using a pointer, you can indirectly modify the value of a variable.
In conclusion, the error message "lvalue required as left operand of assignment" can be frustrating for developers, but it is a common error that can be resolved using the solutions we have provided in this guide. By understanding the meaning of the error message and using the appropriate solution, you can resolve this error and continue coding with confidence.
- GeeksforGeeks
- Techie Delight
Fix Maven Import Issues: Step-By-Step Guide to Troubleshoot Unable to Import Maven Project – See Logs for Details Error
Troubleshooting guide: fixing the i/o operation aborted due to thread exit or application request error, resolving the 'undefined operator *' error for function_handle input arguments: a comprehensive guide, solving the command 'bin sh' failed with exit code 1 issue: comprehensive guide, troubleshooting guide: fixing the 'current working directory is not a cordova-based project' error, solving 'symbol(s) not found for architecture x86_64' error, solving resource interpreted as stylesheet but transferred with mime type text/plain, solving 'failed to push some refs to heroku' error, solving 'container name already in use' error: a comprehensive guide to solving docker container conflicts, solving the issue of unexpected $gopath/go.mod file existence.
Great! You’ve successfully signed up.
Welcome back! You've successfully signed in.
You've successfully subscribed to Lxadm.com.
Your link has expired.
Success! Check your email for magic link to sign-in.
Success! Your billing info has been updated.
Your billing was not updated.
- Windows Programming
- UNIX/Linux Programming
- General C++ Programming
- lvalue required as left operand of assig
lvalue required as left operand of assignment in C++ class

Assignment operators
Simple assignment operator =, compound assignment operators.
The result of an assignment expression is not an lvalue.
All assignment operators have the same precedence and have right-to-left associativity.
The simple assignment operator has the following form:
lvalue = expr
The operator stores the value of the right operand expr in the object designated by the left operand lvalue .
The left operand must be a modifiable lvalue. The type of an assignment operation is the type of the left operand.
If the left operand is not a class type or a vector type , the right operand is implicitly converted to the type of the left operand. This converted type will not be qualified by const or volatile .
If the left operand is a class type, that type must be complete. The copy assignment operator of the left operand will be called.
If the left operand is an object of reference type, the compiler will assign the value of the right operand to the object denoted by the reference.
The compound assignment operators consist of a binary operator and the simple assignment operator. They perform the operation of the binary operator on both operands and store the result of that operation into the left operand, which must be a modifiable lvalue.
The following table shows the operand types of compound assignment expressions:
The following table lists the compound assignment operators and shows an expression using each operator:
Although the equivalent expression column shows the left operands (from the example column) twice, it is in effect evaluated only once.

Understanding lvalues and rvalues in C and C++
The terms lvalue and rvalue are not something one runs into often in C/C++ programming, but when one does, it's usually not immediately clear what they mean. The most common place to run into these terms are in compiler error & warning messages. For example, compiling the following with gcc :
True, this code is somewhat perverse and not something you'd write, but the error message mentions lvalue , which is not a term one usually finds in C/C++ tutorials. Another example is compiling this code with g++ :
Now the error is:
Here again, the error mentions some mysterious rvalue . So what do lvalue and rvalue mean in C and C++? This is what I intend to explore in this article.
A simple definition
This section presents an intentionally simplified definition of lvalues and rvalues . The rest of the article will elaborate on this definition.
An lvalue ( locator value ) represents an object that occupies some identifiable location in memory (i.e. has an address).
rvalues are defined by exclusion, by saying that every expression is either an lvalue or an rvalue . Therefore, from the above definition of lvalue , an rvalue is an expression that does not represent an object occupying some identifiable location in memory.
Basic examples
The terms as defined above may appear vague, which is why it's important to see some simple examples right away.
Let's assume we have an integer variable defined and assigned to:
An assignment expects an lvalue as its left operand, and var is an lvalue, because it is an object with an identifiable memory location. On the other hand, the following are invalid:
Neither the constant 4 , nor the expression var + 1 are lvalues (which makes them rvalues). They're not lvalues because both are temporary results of expressions, which don't have an identifiable memory location (i.e. they can just reside in some temporary register for the duration of the computation). Therefore, assigning to them makes no semantic sense - there's nowhere to assign to.
So it should now be clear what the error message in the first code snippet means. foo returns a temporary value which is an rvalue. Attempting to assign to it is an error, so when seeing foo() = 2; the compiler complains that it expected to see an lvalue on the left-hand-side of the assignment statement.
Not all assignments to results of function calls are invalid, however. For example, C++ references make this possible:
Here foo returns a reference, which is an lvalue , so it can be assigned to. Actually, the ability of C++ to return lvalues from functions is important for implementing some overloaded operators. One common example is overloading the brackets operator [] in classes that implement some kind of lookup access. std::map does this:
The assignment mymap[10] works because the non-const overload of std::map::operator[] returns a reference that can be assigned to.
Modifiable lvalues
Initially when lvalues were defined for C, it literally meant "values suitable for left-hand-side of assignment". Later, however, when ISO C added the const keyword, this definition had to be refined. After all:
So a further refinement had to be added. Not all lvalues can be assigned to. Those that can are called modifiable lvalues . Formally, the C99 standard defines modifiable lvalues as:
[...] an lvalue that does not have array type, does not have an incomplete type, does not have a const-qualified type, and if it is a structure or union, does not have any member (including, recursively, any member or element of all contained aggregates or unions) with a const-qualified type.
Conversions between lvalues and rvalues
Generally speaking, language constructs operating on object values require rvalues as arguments. For example, the binary addition operator '+' takes two rvalues as arguments and returns an rvalue:
As we've seen earlier, a and b are both lvalues. Therefore, in the third line, they undergo an implicit lvalue-to-rvalue conversion . All lvalues that aren't arrays, functions or of incomplete types can be converted thus to rvalues.
What about the other direction? Can rvalues be converted to lvalues? Of course not! This would violate the very nature of an lvalue according to its definition [1] .
This doesn't mean that lvalues can't be produced from rvalues by more explicit means. For example, the unary '*' (dereference) operator takes an rvalue argument but produces an lvalue as a result. Consider this valid code:
Conversely, the unary address-of operator '&' takes an lvalue argument and produces an rvalue:
The ampersand plays another role in C++ - it allows to define reference types. These are called "lvalue references". Non-const lvalue references cannot be assigned rvalues, since that would require an invalid rvalue-to-lvalue conversion:
Constant lvalue references can be assigned rvalues. Since they're constant, the value can't be modified through the reference and hence there's no problem of modifying an rvalue. This makes possible the very common C++ idiom of accepting values by constant references into functions, which avoids unnecessary copying and construction of temporary objects.
CV-qualified rvalues
If we read carefully the portion of the C++ standard discussing lvalue-to-rvalue conversions [2] , we notice it says:
An lvalue (3.10) of a non-function, non-array type T can be converted to an rvalue. [...] If T is a non-class type, the type of the rvalue is the cv-unqualified version of T. Otherwise, the type of the rvalue is T.
What is this "cv-unqualified" thing? CV-qualifier is a term used to describe const and volatile type qualifiers.
From section 3.9.3:
Each type which is a cv-unqualified complete or incomplete object type or is void (3.9) has three corresponding cv-qualified versions of its type: a const-qualified version, a volatile-qualified version, and a const-volatile-qualified version. [...] The cv-qualified or cv-unqualified versions of a type are distinct types; however, they shall have the same representation and alignment requirements (3.9)
But what has this got to do with rvalues? Well, in C, rvalues never have cv-qualified types. Only lvalues do. In C++, on the other hand, class rvalues can have cv-qualified types, but built-in types (like int ) can't. Consider this example:
The second call in main actually calls the foo () const method of A , because the type returned by cbar is const A , which is distinct from A . This is exactly what's meant by the last sentence in the quote mentioned earlier. Note also that the return value from cbar is an rvalue. So this is an example of a cv-qualified rvalue in action.
Rvalue references (C++11)
Rvalue references and the related concept of move semantics is one of the most powerful new features the C++11 standard introduces to the language. A full discussion of the feature is way beyond the scope of this humble article [3] , but I still want to provide a simple example, because I think it's a good place to demonstrate how an understanding of what lvalues and rvalues are aids our ability to reason about non-trivial language concepts.
I've just spent a good part of this article explaining that one of the main differences between lvalues and rvalues is that lvalues can be modified, and rvalues can't. Well, C++11 adds a crucial twist to this distinction, by allowing us to have references to rvalues and thus modify them, in some special circumstances.
As an example, consider a simplistic implementation of a dynamic "integer vector". I'm showing just the relevant methods here:
So, we have the usual constructor, destructor, copy constructor and copy assignment operator [4] defined, all using a logging function to let us know when they're actually called.
Let's run some simple code, which copies the contents of v1 into v2 :
What this prints is:
Makes sense - this faithfully represents what's going on inside operator= . But suppose that we want to assign some rvalue to v2 :
Although here I just assign a freshly constructed vector, it's just a demonstration of a more general case where some temporary rvalue is being built and then assigned to v2 (this can happen for some function returning a vector, for example). What gets printed now is this:
Ouch, this looks like a lot of work. In particular, it has one extra pair of constructor/destructor calls to create and then destroy the temporary object. And this is a shame, because inside the copy assignment operator, another temporary copy is being created and destroyed. That's extra work, for nothing.
Well, no more. C++11 gives us rvalue references with which we can implement "move semantics", and in particular a "move assignment operator" [5] . Let's add another operator= to Intvec :
The && syntax is the new rvalue reference . It does exactly what it sounds it does - gives us a reference to an rvalue, which is going to be destroyed after the call. We can use this fact to just "steal" the internals of the rvalue - it won't need them anyway! This prints:
What happens here is that our new move assignment operator is invoked since an rvalue gets assigned to v2 . The constructor and destructor calls are still needed for the temporary object that's created by Intvec(33) , but another temporary inside the assignment operator is no longer needed. The operator simply switches the rvalue's internal buffer with its own, arranging it so the rvalue's destructor will release our object's own buffer, which is no longer used. Neat.
I'll just mention once again that this example is only the tip of the iceberg on move semantics and rvalue references. As you can probably guess, it's a complex subject with a lot of special cases and gotchas to consider. My point here was to demonstrate a very interesting application of the difference between lvalues and rvalues in C++. The compiler obviously knows when some entity is an rvalue, and can arrange to invoke the correct constructor at compile time.
One can write a lot of C++ code without being concerned with the issue of rvalues vs. lvalues, dismissing them as weird compiler jargon in certain error messages. However, as this article aimed to show, getting a better grasp of this topic can aid in a deeper understanding of certain C++ code constructs, and make parts of the C++ spec and discussions between language experts more intelligible.
Also, in the new C++ spec this topic becomes even more important, because C++11's introduction of rvalue references and move semantics. To really grok this new feature of the language, a solid understanding of what rvalues and lvalues are becomes crucial.

For comments, please send me an email .
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
PEP-0620 Disallows using Py_TYPE() as l-value #325
jcpunk commented Oct 16, 2020
jwakely commented Nov 10, 2020
Sorry, something went wrong.
jwakely commented Nov 14, 2020 • edited
Jcpunk commented nov 14, 2020.
stefanseefeld commented Nov 14, 2020 • edited
Jwakely commented nov 14, 2020, jwakely commented nov 18, 2020, jcpunk commented nov 18, 2020.
- 👍 1 reaction
vstinner commented Dec 9, 2020
No branches or pull requests
lvalue required as left operand of assignment error with ESP32 and constrain cmd
I am changing code from an application using an arduino pro mini 3.3v with HC12 wireless module to an ESP32 with integrated wifi and bluetooth (ESP32 devkit 1)
I didn't get this error before with similar code for the arduino pro mini module and HC12 module. However now that I compile it I am getting this error
lvalue required as left operand of assignment error
I found this link to get some clarity on the issue.
However, I don't think I'm making the error mentioned in the link above. Can someone please explain what I may be doing wrong? Thanks.
I get the error around this line of code: "BR = constrain(BR, 0, 510)" This portion of code is being used to calibrate photoresistor sensors to report similar values despite their inherent variances due to manufacturing tolerances, etc...
When I try to compile your code I get quite a few more errors than the one you quoted. One of them tells me that BR is a special symbol defined in the ESP32 core.
When you try to use it as a variable name the compiler gets very confused.
Avoid this in future by giving variables longer more descriptive names.
Ah I see. thanks. I will try using a different variable.
FYI...the board I have is Espressif ESP32 dev kit 1. I select this board in arduino: DOIT ESP32 DEV KIT 1 https://dl.espressif.com/dl/package_esp32_index.json The url above is what I put in Arduino preferences area to download it in the board manager library.
Here is my code for the Arduino pro mini 3.3V and HC-12 combined. I get no compile errors.
I purposely abbreviated those letters because if I understood things properly regarding transmittal of data via the HC-12 module and the code I have for both the transmitter end and receiver end it can potentially error out during transmission? (Post becoming too long adding receiver code in next post)
Transmitter:
Wow... thanks changed the variable it's compiling now!
fyi... TL (top left sensor) TR (top right sensor) BRT (bottom right sensor) BL (bottom left sensor)
It's good that you used Pin in the names of variables containing pin numbers. It was pointless to use sensor in those names, though, since there isn't much else you'd connect to the pins.
Now, if you had used Value in the names of variables that held values, you would not have inadvertently reused an already used name.
By convention, all capital letter names are reserved for constants. Constants never appear on the left of equal signs:
Thanks I'll reformat the code to match the convention regarding capital letters for constants.
Related Topics
lvalue required as left operand of assignment
Lvalue required as left operand of assignment报错, error: lvalue required as left operand of assignment.
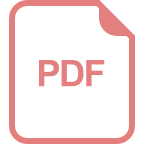
Sophus 安装出现的问题
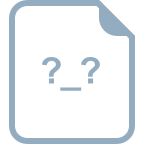
php7 测试可用的amqp 扩展
理解c++ lvalue与rvalue.

[Error] lvalue required as left operand of assignment
[error] lvalue required as left operand of assignment, error: lvalue required as left operand of assignment|, [error] lvalue required as left operand of assignment是什么意思, 9 17 c:\users\leo\desktop\c++\指针.cpp [error] lvalue required as left operand of assignment, 20 36 c:\users\10036\desktop\狗都不写的垃圾代码\构造\abcsort.cpp [error] lvalue required as left operand of assignment, 11 11 c:\users\27710\desktop\dev-c++\2.cpp [error] lvalue required as left operand of assignment, test1.c:77:54: error: lvalue required as left operand of assignment, lvalue required as left operand pf assignment, c:\users\user\desktop\c语言\4.15\main.c|56|error: lvalue required as left operand of assignment|, 7 24 c:\users\administrator\desktop\vv.cpp [error] lvalue required as left operand of assignment, 29 11 c:\users\administrator\desktop\c语言\任务五\4.c [error] lvalue required as left operand of assignment, c:\users\13604\desktop\lin_a (2)\lin_a\a\manager.h|172|error: lvalue required as left operand of assignment|, _recv_cmd': /home/muchen/esp/websocket/websocket/main/server.c:198:24: error: lvalue required as left operand of assignment.
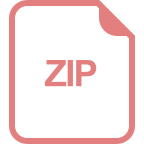
chromedriver-linux64-V124.0.6367.91 稳定版
基于yolov7 加入 depth回归, 基于stm32f101单片机设计bluetooth sentinel 主板硬件(原理图+pcb)工程文件.zip.
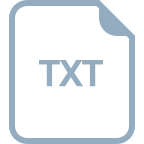
【前端热门框架【vue框架】】——条件渲染和列表渲染的学习的秒杀方式 (2).txt
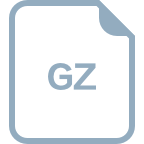
RTL8188FU-Linux-v5.7.4.2-36687.20200602.tar(20765).gz

Redis验证与连接:快速连接Redis服务器指南

gunicorn -k geventwebsocket.gunicorn.workers.GeventWebSocketWorker app:app 报错 ModuleNotFoundError: No module named 'geventwebsocket' ]
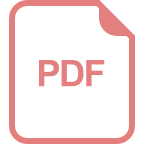
c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
"互动学习:行动中的多样性与论文攻读经历".
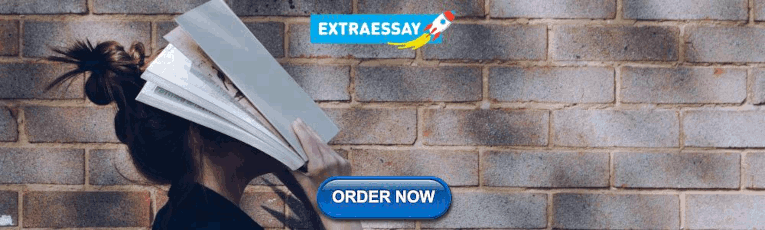
IMAGES
VIDEO
COMMENTS
Put simply, an lvalue is something that can appear on the left-hand side of an assignment, typically a variable or array element. So if you define int *p, then p is an lvalue. p+1, which is a valid expression, is not an lvalue. If you're trying to add 1 to p, the correct syntax is: p = p + 1; answered Oct 27, 2015 at 18:02.
About the error: lvalue required as left operand of assignment. lvalue means an assignable value (variable), and in assignment the left value to the = has to be lvalue (pretty clear). Both function results and constants are not assignable ( rvalue s), so they are rvalue s. so the order doesn't matter and if you forget to use == you will get ...
文章浏览阅读10w+次,点赞79次,收藏76次。[Error] lvalue required as left operand of assignment原因:计算值为== !=变量为= 赋值语句的左边应该是变量,不能是表达式。而实际上,这里是一个比较表达式,所以要把赋值号(=)改用关系运算符(==)..._lvalue required as left operand of assignment
1. Assuming that originalArray, ascendingOrderArray and descendingOrderArray are pointers of type int * and randInt is of type int, you have to de-reference the pointer when assigning. For example *originalArray++ = randInt;. That will de-reference the pointer yielding the "lvalue" you can assign to, assign the value of randInt to it (to ...
lvalue means left side value.Particularly it is left side value of an assignment operator.
The key phrase is "lvalue required as left operand of assignment." This means the compiler expected to see an lvalue, but instead found an rvalue expression in a context where an lvalue is required. Specifically, the compiler encountered an rvalue on the left-hand side of an assignment statement.
Output: example1.cpp: In function 'int main()': example1.cpp:6:4: error: lvalue required as left operand of assignment. 6 | 4 = x; | ^. As we have mentioned, the number literal 4 isn't an l-value, which is required for the left operand. You will need to write the assignment statement the other way around: #include <iostream> using ...
Learn how to fix the "error: lvalue required as left operand of assignment" in your code! Check for typographical errors, scope, data type, memory allocation, and use pointers. #programmingtips #assignmenterrors (error: lvalue required as left operand of assignment)
To resolve the "lvalue required as left operand of assignment" error, the programmer must ensure that the left operand of the assignment operator is an lvalue. Here are some examples of how to fix the code that we saw earlier: int x = 5; x = 10; // Fix: x is an lvalue int y = 0; y = 5; // Fix: y is an lvalue
Causes of the Error: lvalue required as left operand of assignment. When encountering the message "lvalue required as left operand of assignment," it is important to understand the underlying that lead to this issue.
R-value: r-value" refers to data value that is stored at some address in memory. A r-value is an expression, that can't have a value assigned to it, which means r-value can appear on right but not on left hand side of an assignment operator (=). C. // declare 'a', 'b' an object of type 'int'. int a = 1, b; a + 1 = b; // Error, left ...
Understanding the Meaning and Solutions for 'lvalue Required as Left Operand of Assignment'
The solution is simple, just add the address-of & operator to the return type of the overload of your index operator []. So to say that the overload of your index [] operator should not return a copy of a value but a reference of the element located at the desired index. Ex:
Assignment operators. An assignment expression stores a value in the object designated by the left operand. There are two types of assignment operators: Simple assignment operator =. Compound assignment operators. The left operand in all assignment expressions must be a modifiable lvalue. The type of the expression is the type of the left operand.
A simple definition. This section presents an intentionally simplified definition of lvalues and rvalues.The rest of the article will elaborate on this definition. An lvalue (locator value) represents an object that occupies some identifiable location in memory (i.e. has an address).. rvalues are defined by exclusion, by saying that every expression is either an lvalue or an rvalue.
6.5.4 Cast operators [...] 4 Preceding an expression by a parenthesized type name converts the value of the expression to the named type. This construction is called a cast. (footnote 89) (footnote 89) A cast does not yield an lvalue. Thus, a cast to a qualified type has the same effect as a cast to the unqualified version of the type
Saved searches Use saved searches to filter your results more quickly
Check all your 'if' statements for equality. You are incorrectly using the assignment operator '=' instead of the equality operator '=='.
3. f1() returns an rvalue. You have to return a reference (lvalue) to allow you to do this assignment. Change. to. f1() returns rvalue but as instance of class f1() = X(1); calls assignment operator of class f1().operator=(X(1)); which is alright. Read more about value categories here.
lvalue required as left operand of assignment PLEASE HELP ME! Programming Questions. 5: 2283: May 5, 2021 lvalue required as left operand of assignment. Programming Questions. 5: 28899: May 5, 2021 lvalue required as left operand of assignment. Programming Questions. 8: 1757:
error: lvalue required as left operand of assignment 这个错误通常表示你试图将一个非左值(比如常量或表达式)赋值给一个变量。 请确保你要赋值的变量是一个可修改的左值,比如一个变量或一个可以被修改的数组元素。
lvalue required as left operand of assignment. I am not sure what it is trying to say. nPointer->enterStrtPrc()=infoClass.enterStrtPrc(); nPointer->enterSellVal()=infoClass.enterSellVal(); are supposed to return int values and store them in the dynamically created class infoClass.