Computersciencementor | Hardware, Software, Networking and programming
Algorithm and flowchart explained with examples, what is algorithm and flowchart.
Algorithm and flowchart are programming tools. A Programmer uses various programming languages to create programs. But before actually writing a program in a programming language, a programmer first needs to find a procedure for solving the problem which is known as planning the program. The program written without proper pre-planning have higher chances of errors. The tools that are used to plan or design the problem are known as programming tools. Algorithm and flowchart are widely used programming tools.
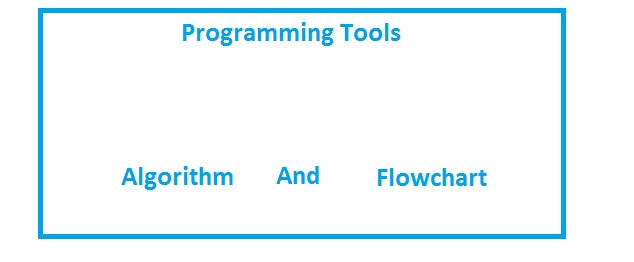
The word “algorithm” relates to the name of the mathematician Al- khowarizmi , which means a procedure or a technique. Programmer commonly uses an algorithm for planning and solving the problems.
An algorithm is a specific set of meaningful instructions written in a specific order for carrying out or solving a specific problem.
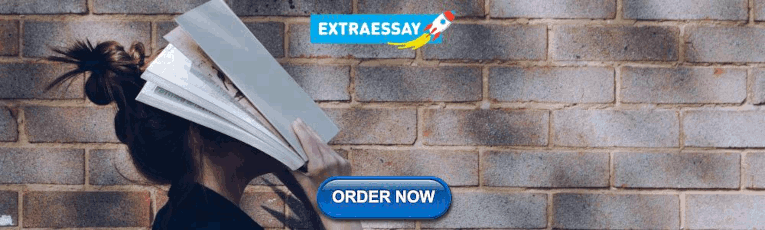
Types of Algorithm:
The algorithm and flowchart are classified into three types of control structures.
- Branching(Selection)
- Loop(Repetition)
According to the condition and requirement, these three control structures can be used.
In the sequence structure, statements are placed one after the other and the execution takes place starting from up to down.
Whereas in branch control, there is a condition and according to a condition, a decision of either TRUE or FALSE is achieved. In the case of TRUE, one of the two branches is explored; but in the case of FALSE condition, the other alternative is taken. Generally, the ‘IF-THEN’ is used to represent branch control.
Write an algorithm to find the smallest number between two numbers
Write an algorithm to check odd or even number.
The Loop or Repetition allows a statements or block of statements to be executed repeatedly based on certain loop condition. ‘While’ and ‘for’ construct are used to represent the loop structure in most programming languages. Loops are of two types: Bounded and Unbounded loop. In bounded loop, the number of iterations is fixed while in unbounded loops the condition has to satisfy to end the loop.
An algorithm to calculate even numbers between 20 and 40
Write an algorithm to input a natural number, n, and calculate the odd numbers equal or less than n.
Characteristics of a good algorithm.
- The Finite number of steps:
After starting an algorithm for any problem, it has to terminate at some point.
- Easy Modification.
There can be numbers of steps in an algorithm depending on the type of problem. It supports easy modification of Steps.
- Easy and simple to understand
A Simple English language is used while writing an algorithm. It is not dependent on any particular programming language. People without the knowledge of programming can read and understand the steps in the algorithm.
An algorithm is just a design of a program. Every program needs to display certain output after processing the input data. So one always expects the result as an output from an algorithm. It can give output at different stages. The result obtained at the end of an algorithm is known as an end result and if the result is obtained at an intermediate stage of process or operation then the result is known as an intermediate result. Also, the output has to be as expected having some relation to the inputs.
The first design of flowchart goes back to 1945 which was designed by John Von Neumann . Unlike an algorithm, Flowchart uses different symbols to design a solution to a problem. It is another commonly used programming tool.
In general, a flowchart is a diagram that uses different symbols to visually present the flow of data. By looking at a flow chart one can understand the operations and sequence of operations performed in a system. This is why flowchart is often considered as a blueprint of a design used for solving a specific problem.
A flowchart is defined as a symbolic or a graphical representation of an algorithm that uses different standard symbols.
Flowchart Symbols:
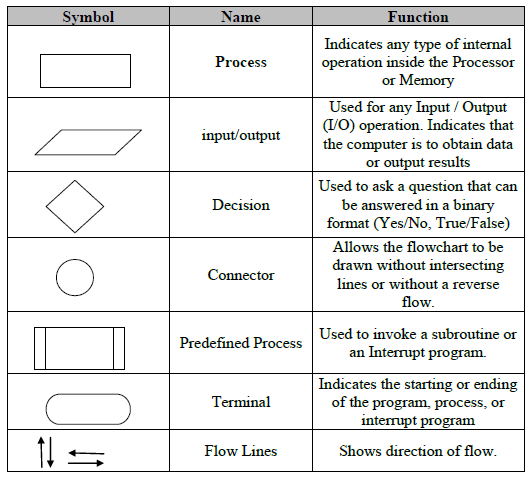
Guidelines for drawing a flowchart.
- The Title for every flowchart is compulsory.
- There must be START and END point for every flowchart.
- The symbols used in flowchart should have only one entry point on the top. The exit point for symbols (except for decision/diamond symbol) is on the button.
- There should be two exit points for decision symbol; exit points can be on the bottom and one side or on the sides.
- The flow of flowchart is generally from top to bottom. But in some cases, it can also flow to upward direction
- The direction of the flow of control should be indicated by arrowheads.
- The operations for every step should be written inside the symbol.
- The language used in flowchart should be simple so that it can be easily understood.
- The flowlines that show the direction of flow of flowchart must not cross each other.
- While connecting different pages of the same flowchart, Connectors must be used.
Some examples of algorithm and flowchart.
Example1: To calculate the area of a circle
Step1: Start
Step2: Input radius of the circle say r
Step3: Use the formula πr 2 and store result in a variable AREA
Step4: Print AREA
Step5: Stop Flowchart:
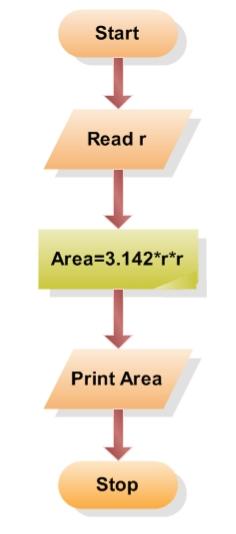
Related Posts
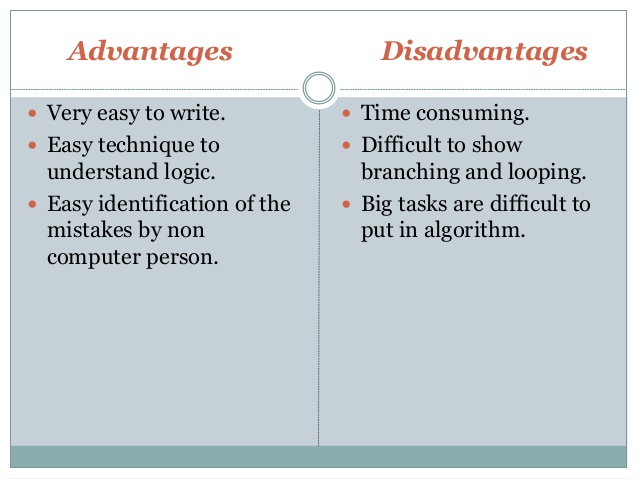
Sampurna shrestha
Save my name, email, and website in this browser for the next time I comment.
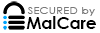
Algorithms, flowcharts, and pseudocode. ¶
Overview, objectives, and key terms ¶.
In this lesson, we’ll dive right into the basic logic needed to plan one’s program, significantly extending the process identified in Lesson 2 . We’ll examine algorithms for several applications and illustrate solutions using flowcharts and pseudocode . Along the way, we’ll see for the first time the three principal structures in programming logic: sequence , selection , and iteration . Throughout, specialized syntax (Python or otherwise) is avoided (with the exception of the handy slicing syntax introduced in Lesson 3 ), but variables and simple operators are used.
Objectives ¶
By the end of this lesson, you should be able to
- define what an algorithm is
- decompose problems into a sequence of simple steps
- illustrate those steps graphically using flowcharts
- describe those steps in words using pseudocode
Key Terms ¶
- counter variable
- index variable
- conditional statement
Algorithms ¶
An algorithm is procedure by which a problem (computational or otherwise) is solved following a certain set of rules. In many computer science departments, entire courses are dedicated to the study of algorithms important for solving a wide variety of problems. Some of the most important algorithms (including a few that we’ll be studying in part throughout the semester) are
- Gaussian elimination (for solving \(\mathbf{Ax}=\mathbf{b}\) )
- Bubble sort (for sorting an array of values)
- Quicksort (a better way to sort those same values)
- Sieve of Eratosthenes (for finding prime numbers)
- Babylonian method (for finding square roots)
- Linear search (for finding a value in an array)
- Binary search (a better way for finding that value)
- Dijkstra’s algorithm (for finding, e.g., the shortest path between two cities)
- RSA algorithm (for encrypting and decrypting messages)
Many more such algorithms are listed elsewhere .
It turns out, however, that we apply algorithms all the time in every-day life, often without realizing it. Such occasions might be as boring as choosing one’s outfit for the day based on planned activities, the weather, the general status of laundry (and how many times you think you can wear that pair of jeans), etc. Other occasions of somewhat more importance might include choosing a baby’s name (which might mean making a list, whittling it down, and then convincing your wife that Linus is the one not just because that’s the name of the Finnish-born inventor of Linux). The list goes on and on: life presents problems, and we apply logic to solve those problems.
Sequence by Example: Computing Grades ¶
Let’s consider something with which you are all familiar: a course grading scheme. As a concrete example, suppose the course in question has graded tasks in four categories, each with its own weight:
- homework - 20%
- laboratories - 10%
- quizzes - 10%
- examinations - 60%
Such a scheme is probably familiar, and you’ve probably used your intuition, knowledge of your grades, and knowledge of this sort of weighting scheme to compute a final percentage. Here, we’ll formalize that process using flowcharts and pseudocode.
To simplify matters somewhat (for now), assume that there are a fixed number of tasks in each category, and each task within a category is worth the same amount. For example, the course might have 5 homework assignments, and each of these assignments might be assessed out of ten points. Take those scores to be 10, 7, 9, 10, and 10.
You might immediately see how the computation of a homework percentage can be broken into several independent steps. For instance, we can first compute the homework score. How? Think carefully. If you were using a calculator, what would you enter? On mine, I can enter 10 followed by a + and then 7 and then another + . After every addition + , it prints out the running total. In other words, it’s automatically storing the running total.
We can be more explicit, though. Before we add any of the scores at all, we can set the total score to zero, and thereafter, we can add each of the homework scores to that total in sequence . When that’s done, we need to compute the total possible score, which is the number of tasks multiplied by the point value per task. Finally, we need to compute the percentage earned on these homework assignments, which is equal to the total homework score divided by the total number of points possible (and then multiplied by 100).
This entire process can be described using pseudocode , which is any informal, high-level (few details) language used to describe a program, algorithm, procedure, or anything else with well-defined steps. There is no official syntax for pseudocode, but usually it is much closer to regular English than computer programming languages like Python. Here is one possible pseudocode representation of the procedure just outlined for computing the homework score:
Of course, this might seem long-winded, but it is explicit . As long as the input and output processes are specified (e.g., providing a printout of grades and asking for a final value to be written on that printout), this procedure could be given to most reasonable people and executed without difficulty. For reference, the total homework score (in percent) that one should get from the individual scores above is 92.
Exercise : For each line of the pseudocode above, write the value of total_score , total_possible , and hw_percent . If a variable does not have a value at a particular line, then say it is undefined .
A flowchart of the same procedure is provided below.
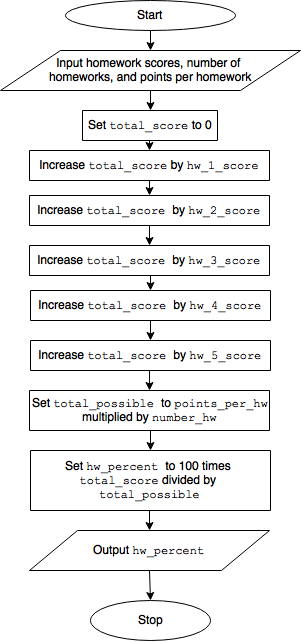
Flowchart for Computing Homework Percentage
Now, revisit the bolded word above: sequence . This particular process for computing the homework percentage is a sequence , which is the simplest of the logical structures used in programming. A sequence is nothing more than a set of instructions executed one after another. The number of these instructions and the order in which they are executed is known from the start, just like our example here (and the example seen in Lesson 2 ).
Selection ¶
The same sort of procedure can be used for all of the grading categories, and hence, let us assume now that we have available the final percentages for homework, laboratories, quizzes, and homeworks. The question now is how to assign a final letter grade based, for example, on the traditional 90/80/70/60 scheme for A/B/C/D. For this grading scheme, anything 90% or higher earns an A, anything less than 90% but greater than or equal to 80% earns a B, and so forth.
Of course, this requires that we first compute the final grade, for which the final percentages and the corresponding category weights are required. In pseudocode, that process can be done via
What we need next is to define the grade, and that’s where selection enters the game. Selection refers to a structure that allows certain statements to be executed preferentially based on some condition . Hence, selection is often implemented using conditional statements . In English, a basic conditional statement takes the form If some condition is satisfied, then do something . More complicated statements can be formed, but all conditional statements can be rewritten using one or more of these simple statements.
A basic conditional statement can be represented graphically as shown below.
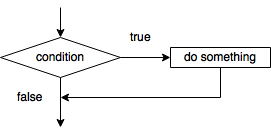
Flowchart fragment for a simple conditional statement
Armed with conditional statements for selection, we can tackle the final grade. Given the final percentage, we check whether that value is greater than 90. If so, the grade assigned is an A. If not, we can further check whether that percentage is greater than or equal to 80 and less than 90. If so, a B. If not, we check against 70 and then 60 for a C and D, respectively. If the percentage has not earned a D by the time we’ve checked, our poor student’s grade, of course, is an F.
This procedure, much like those described previously, can be described compactly in pseudocode:
As a flowchart, this same procedure is shown below.
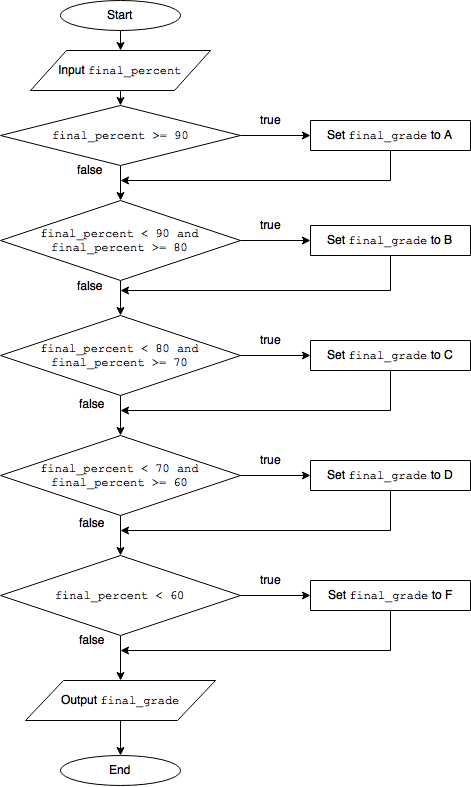
Flowchart for computing the final grade
Iteration ¶
Suppose that the homework grades analyzed above are subjected to a slight policy change: the lowest score is dropped. Such a policy is pretty common. How do we tackle this problem? It requires that we make a decision when adding a particular homework score to the total. In particularly, we skip adding the lowest score.
Exercise : Think back to the scores (i.e., 10, 7, 9, 10, and 10). Now, pick out the lowest score and, importantly , write down how you came to that conclusion. Seriously—think about what you needed to do to choose the lowest number and get it down in words.
When I first glance at the numbers, I see that there are some 10’s and other numbers, and scanning left to right, I pick out 7 as being the lowest of them. Then, keeping track of 7 (subconsciously, in this case), I scan the list and confirm that 7 is, in fact, the lowest score. I’ll bet you do something similar, but computers don’t have eyes (or a subconscience), and we therefore need to define this a bit more carefully.
The Basic Idea ¶
To start, let’s assume that the individual homework grades are stored in an array (e.g., a NumPy ndarray ) rather than as separate values. Call this array hw_scores so that we use, e.g., hw_scores[0] and hw_scores[1] instead of hw_1_score and hw_2_score . From this array, we need to pick out which element represents the score to be dropped. Therefore, we need language to describe the process of iterating through the array elements, i.e., iteration . In English, we might say something like “for each score in the array, do something.” More generically, iteration consists of doing that something until some condition is no longer satisfied, as illustrated in the flowchart fragment below:
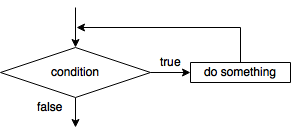
Flowchart fragment for a simple loop
Notice that this flowchart bears a striking similarity to the one shown above for the conditional statement. The difference, however, is that the flow of the program is redirected to before the condition block. In other words, a repeating loop is produced that is broken only if the condition is no longer because of changes made inside the “do something” block. The pseudocode fragment for this same loop is
Let’s apply this to a simple but illustrative problem before moving on to our homework application. Suppose we want to print every value in an array a of length n . A canonical approach to this sort of problem starts by defining an index or counter variable (often named i ) that is used to access the i th element of the array during the i th time through the loop. After the i th element has been used, the counter is increased by one, and the loop begins again. Once the counter is equal to the number of elements in the array, the loop is terminated. Here’s the complete algorithm in pseudocode
and as a flowchart
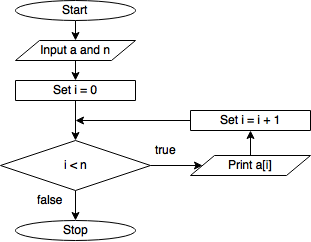
Flowchart for printing the elements of an array
Back to Homework ¶
For our homework example, the condition is “have all the scores been considered?”, while the “something” to be done is checking whether or not a given score is lower than the others. The counter-based approach to iteration developed above can be used to go through each element of the homework score array, thereby ensuring that all the elements are considered. We could compare the i th grade to every other grade (implying an iteration within an iteration), but there is a simpler way. First, we can set the lowest score equal to the first grade (i.e., hw_grades[0] ). Then, we can go through all the other scores and compare each to the lowest score. If a score is lower than the current value of lowest score, that score becomes the lowest score. Along the way, we can also keep track of the index (location) of the lowest score within the array. Here’s the idea in pseudocode:
Here it is also as a flow chart:
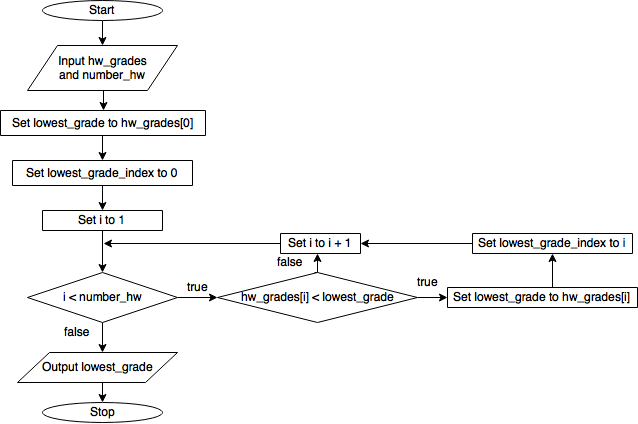
Flowchart for finding the lowest grade
Putting It All Together ¶
By now, we have all the pieces needed to compute the total homework percentage when the lowest score is dropped. Here it is, all together, in pseudocode:
Practice Problems ¶
Planning out the logic needed to solve problems can be tricky, and most students require lots of practice. Each of the topics discussed above will be the focus of the next several lessons as we dive into their implementation in Python. However, now is the time to start applying the sequence, selection, and iteration constructs, and listed below are several great practice problems to help get you started.
Develop an algorithm for each of the following tasks using both pseudocode and a flowchart . Note, many of these tasks represent great practice problems for Python programs, too. Of course, if you plan out the logic first using the tools employed in this lesson, the implementation of your solution in Python later on should be much easier!
- Prepare a pot of coffee.
- Prepare a cup of coffee for your friend. (Cream or sugar?)
- String a guitar.
- Choose your outfit (making sure to account for the day of the week and the weather).
- Determine the largest number in an array.
- Determine the second largest number in an array.
- Determine whether an integer is even.
- Determine whether an integer is prime .
- Determine whether a given year is a leap year .
- Determine the next leap year after a given year.
- Divide one number by another number using long division
- Determine the sum of an array of numbers.
- Determine the sum of the elements of an array of numbers that are divisible by 3 and 5.
- Given any two integers n and d , determine whether the quotient n/d leads to a finite decimal number (e.g., \(5/4 = 1.25\) ) or an infinite decimal number (e.g., \(1/3 = 0.3333\ldots = 0.\bar{3}\) ).
- Extend the homework grade algorithm to drop the two lowest scores.
- Determine a final grade in ME 400 following the course grading policies (including drops).
Further Reading ¶
Here is a nice example of flow chart construction for the game of hangman.
Table Of Contents
- Sequence by Example: Computing Grades
- The Basic Idea
- Back to Homework
- Putting It All Together
- Practice Problems
- Further Reading
Related Topics
- Previous: More on NumPy Arrays: Slicing and np.linalg
- Next: Lecture 6 - Conditional Statements and the Structure of Python Code
- Show Source
Quick search
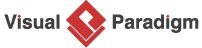
- Demo Videos
- Interactive Product Tours
- Request Demo
Flowchart Tutorial (with Symbols, Guide and Examples)
A flowchart is simply a graphical representation of steps. It shows steps in sequential order and is widely used in presenting the flow of algorithms, workflow or processes. Typically, a flowchart shows the steps as boxes of various kinds, and their order by connecting them with arrows.
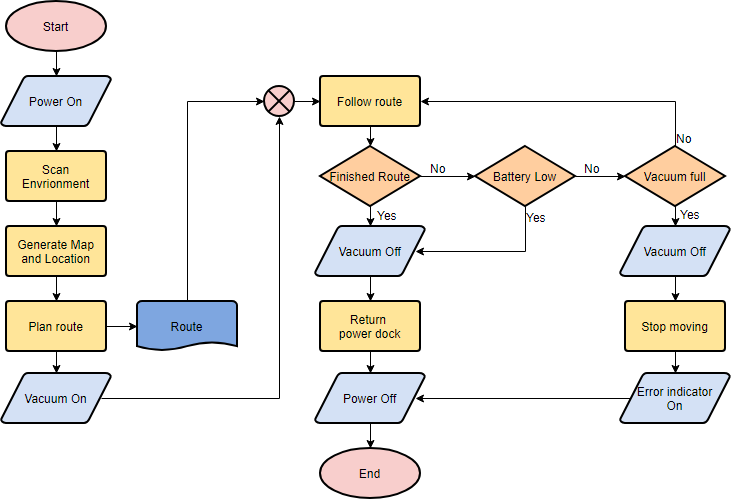
What is a Flowchart?
A flowchart is a graphical representations of steps. It was originated from computer science as a tool for representing algorithms and programming logic but had extended to use in all other kinds of processes. Nowadays, flowcharts play an extremely important role in displaying information and assisting reasoning. They help us visualize complex processes, or make explicit the structure of problems and tasks. A flowchart can also be used to define a process or project to be implemented.
Flowchart Symbols
Different flowchart shapes have different conventional meanings. The meanings of some of the more common shapes are as follows:
The terminator symbol represents the starting or ending point of the system.
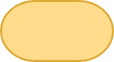
A box indicates some particular operation.
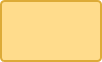
This represents a printout, such as a document or a report.
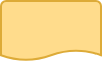
A diamond represents a decision or branching point. Lines coming out from the diamond indicates different possible situations, leading to different sub-processes.

It represents information entering or leaving the system. An input might be an order from a customer. Output can be a product to be delivered.
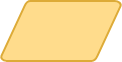
On-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on the same page.

Off-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on a different page.

Delay or Bottleneck
Identifies a delay or a bottleneck.
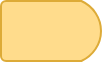
Lines represent the flow of the sequence and direction of a process.

When to Draw Flowchart?
Using a flowchart has a variety of benefits:
- It helps to clarify complex processes.
- It identifies steps that do not add value to the internal or external customer, including delays; needless storage and transportation; unnecessary work, duplication, and added expense; breakdowns in communication.
- It helps team members gain a shared understanding of the process and use this knowledge to collect data, identify problems, focus discussions, and identify resources.
- It serves as a basis for designing new processes.
Flowchart examples
Here are several flowchart examples. See how you can apply a flowchart practically.
Flowchart Example – Medical Service
This is a hospital flowchart example that shows how clinical cases shall be processed. This flowchart uses decision shapes intensively in representing alternative flows.
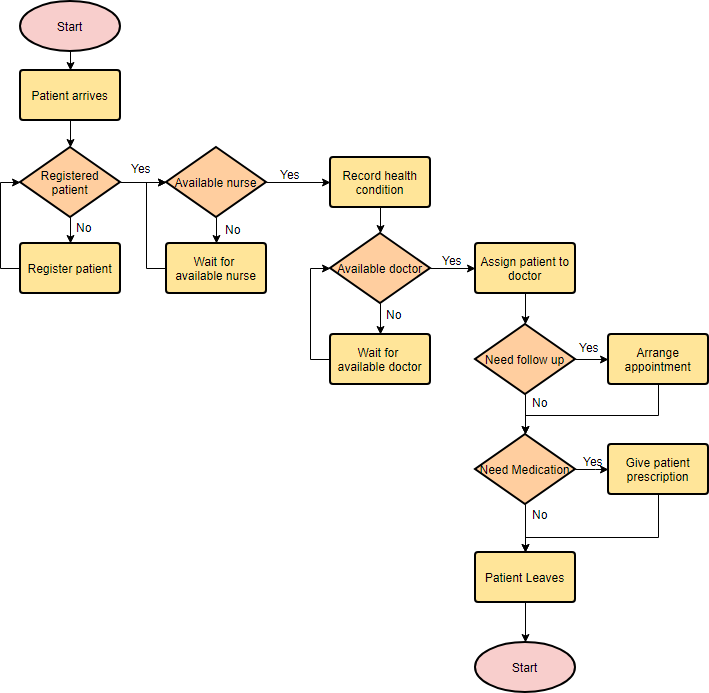
Flowchart Example – Simple Algorithms
A flowchart can also be used in visualizing algorithms, regardless of its complexity. Here is an example that shows how flowchart can be used in showing a simple summation process.
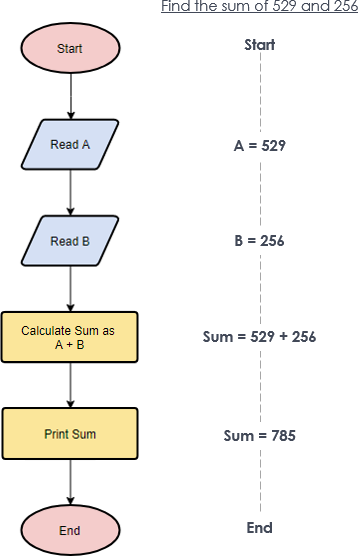
Flowchart Example – Calculate Profit and Loss
The flowchart example below shows how profit and loss can be calculated.
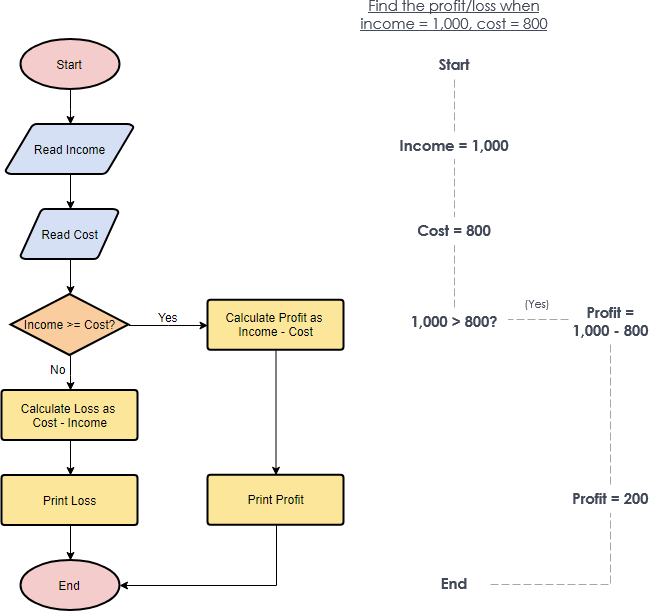
Creating a Flowchart in Visual Paradigm
Let’s see how to draw a flowchart in Visual Paradigm. We will use a very simple flowchart example here. You may expand the example when finished this tutorial.
- Select Diagram > New from the main menu.
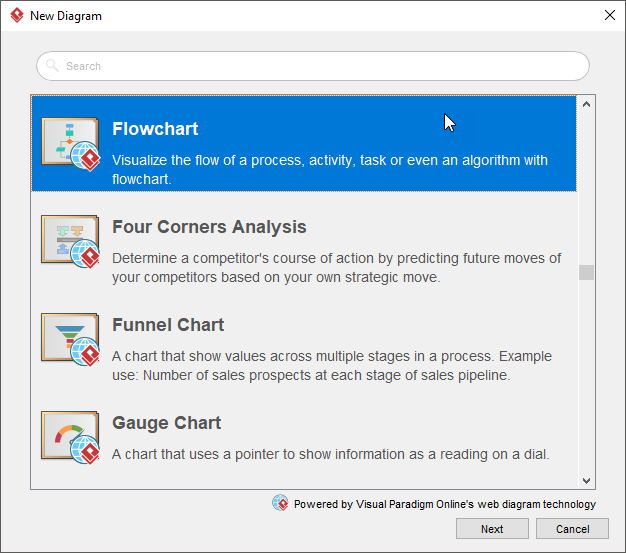
- Enter the name of the flowchart and click OK .
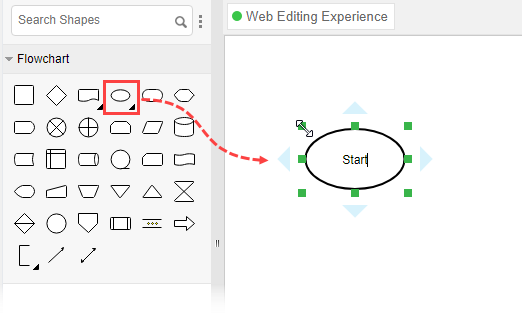
- Enter Add items to Cart as the name of the process.

Turn every software project into a successful one.
We use cookies to offer you a better experience. By visiting our website, you agree to the use of cookies as described in our Cookie Policy .
© 2024 by Visual Paradigm. All rights reserved.
- Privacy statement
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center
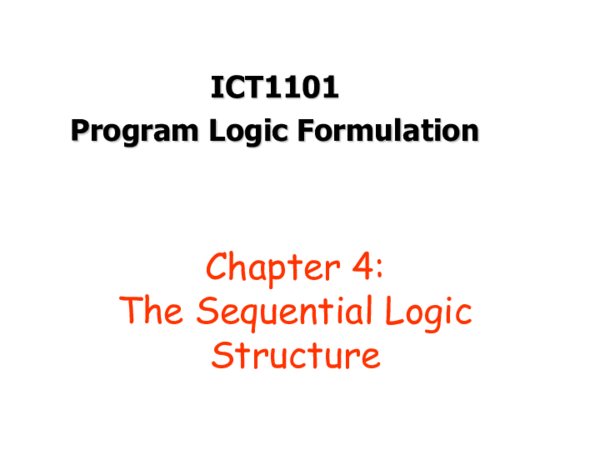
CHAPTER 4 sequential logic structure

ICT Looping structure notes
Related Papers
Clara Szalai
In spite of the fact that we made great progress toward understanding complexity, logic – whether first order, second order, or other – is still a mechanistic, linear, reductionistic attempt at describing natural language, and it cannot really deal with self-reference or paradoxes. Although aware of meta-language, it focuses on object-language (true/false), and a logician is needed to infer. A paradigm shift is needed to not only describe, but create language and meaning, a dynamic logic that is emergent, adaptive, in harmony with the holistic principles of the zeitgeist, a logic that can be the fundament of true artificial intelligence through creating its own meta-language and inference, and in fact, a logic that can be the fundament of all natural phenomena. In this paper I am proposing the first steps to such a logic that on the one hand corresponds with the science of complexity, and on the other, is indicative of a greater understanding of complexity, its navigability and control.

Tem Journal
There are three possible behavioral patterns for the WHILE loop: it does not terminate, it potentially terminates and its termination is guaranteed. Based on that, to describe the behavior of the WHILE loop we introduce appropriate formulas of the first-order predicate logic defined on the abstract state space (briefly S-formulas). This paper presents our approach to analyzing the WHILE loop semantics that is solely based on the first order predicate logic.
Marlene Eynn
Muhammad Asad
Theory and Practice of Logic Programming
Martin Gebser
suranjith rajapaksha
harish chottu
Ricardo Menotti
ABSTRACT Sequences of loops or sets of nested loops exist in many applications. This paper shows a scheme to pipeline those sequences of loops in such a way that subsequent loops can start execution before the end of the previous ones. It uses a hardware scheme with decoupled and concurrent datapath and control units that start execution at the same time. The communication of data items between two loops in sequence is conducted by memories.
Journal of Logic, Language and Information
Alexander Bochman
OBAHOPO TEMITOPE OLORUNTOBI
OBAHOPO T E M I T O P E OLORUNTOBI
The basic mathematical logic and its application to digital circuit.
Loading Preview
Sorry, preview is currently unavailable. You can download the paper by clicking the button above.
RELATED PAPERS
Obat Alami Buah Zakar Bengkak & Sakit Sebelah Di Apotik Terbukti Paling Ampuh
Varikokel Zakar Bengkak
Journal of Ayub Medical College (JAMC)
Arshalooz Rahman
Florian Mussgnug , Irina Marchesini
Schizophrenia Research
Salvador Sarró
Nihon Rinsho Geka Gakkai Zasshi (Journal of Japan Surgical Association)
Yoshihisa Saida
Journal of Quaternary Science
isabel vilanova
Analytical biochemistry
Magaji Ladan
Journal of Fungi
Naeem Sattar
Angel Falcones
Prática Contabilística e Implicações Fiscais
Genial Maths Learning to BsP
Iranian Journal of Pharmaceutical Research
Valiollah Mehrzad
Cambridge Scholars Publishing
The Journal of Immunology
Michael LePage
Journal of Polymer Science Part A: Polymer Chemistry
MUHAMMED BEŞİR AYDIN
Cuadernos Filosóficos / Segunda Época
Marcela Coria
Rosario García Cruz
Bulgarian Journal of Agricultural Science
Haris Kriswantoro
Historia (Santiago)
CARLOS DANIEL CAYARI CHOQUE
Adriana Aparecida da silva
Abdul Hakim Hissobri Khalip
David Imhof
Biotechnology and Bioprocess Engineering
Postharvest Biology and Technology
Juan Del Aguila
Jasminka Persec
2021 International Conference on Software, Telecommunications and Computer Networks (SoftCOM)
Adrian Kliks
See More Documents Like This
RELATED TOPICS
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024
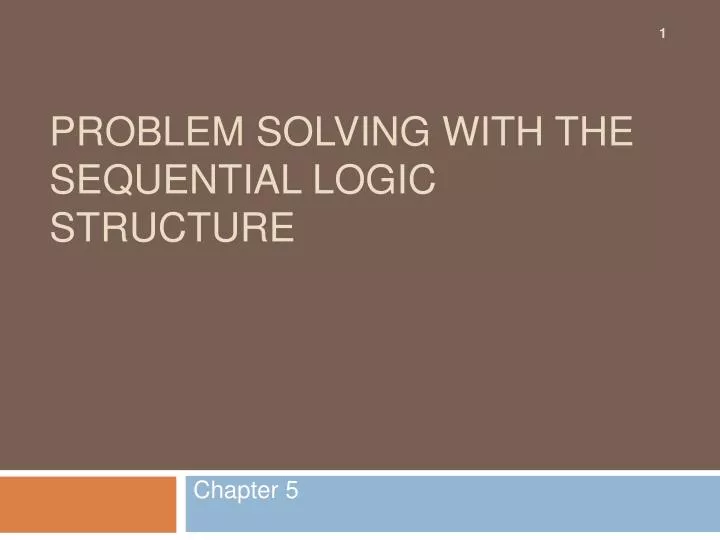
Problem Solving with the Sequential Logic Structure
Sep 15, 2012
390 likes | 1.98k Views
Problem Solving with the Sequential Logic Structure. Chapter 5. Review. Recall that the: Algorithm: set of instructions telling the computer how to process a module in a solution. A flowchart: visual illustration of an algorithm. In this chapter you will learn how to:
Share Presentation
- seventh step
- sequential logic structure
- simplest logic structure
- data dictionary
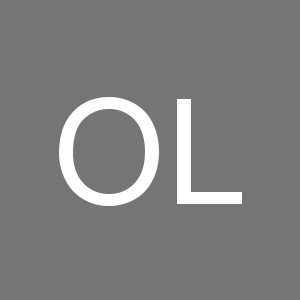
Presentation Transcript
Problem Solving with the Sequential Logic Structure Chapter 5
Review • Recall that the: • Algorithm: set of instructions telling the computer how to process a module in a solution. • A flowchart: visual illustration of an algorithm. • In this chapter you will learn how to: • Write an algorithm for each module in a program. • Draw a flowchart to accompany in each algorithm.
Algorithm Instructions and Flowchart Symbols
Exit is used to end a subordinate module if there is no return value . • Return is used when the module is to be processed within an expression . average = sum ( num1 , num2) / 2
Sequential Logic Structure • The most commonly used and the simplest logic structure . • all problem use the sequential structure . • most problem problems use it in conjunction with one or more of the other logic structure .
Sequential Logic Structure • The form of the algorithm looks like this : module name ( list of parameters) 1. instruction 2. instruction 3. ….. … x x.End,Exit,or Return (variable )
Flowchart Diagram for the Sequential Structure
Example: The algorithm and flowchart to enter a name and age into computer and print it on the screen. ( ) Notice that the algorithm instructions are numbered starting with the first instruction after the name of module
solution development • The problem analysis chart. • The interactivity chart . • The IPO chart . • The coupling diagram and the data dictionary . • The algorithms . • The flowcharts . • The seventh step in solving the problem is to test the solution to catch any errors in logic or calculation .
Let’s work a problem…
The Problem • Marry smith is looking for the bank that will give the most return on her money over the next five years. She has $2,000 to put into a savings account. • The standard equation to calculate principal plus interest at the end of a period of time is: Amount = p * (1+I/M)^(N*M) 12
The Problem • Amount = p * (1+I/M)^(N*M) • p= principal ( amount of money to invest) • I = interest ( percentage rate the bank pays to the invested) • N = number of years ( time for which the principle is invested ) • M = compound interval ( the number of times per year the interest is calculated and added to the principal )
The Interactivity (Structure) Chart
Coupling Diagram
Data Dictionary • in this problem all variables are local . • All coupling will be done through parameters .
Algorithm and Flowchart for InterestControl Module
Algorithm and Flowchart for Read Module
Algorithm and Flowchart for Calc Module
Algorithm and Flowchart for Print Module
- More by User
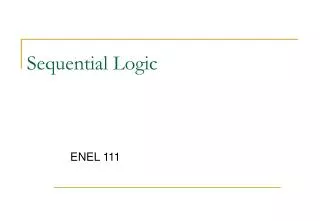
Sequential Logic
Sequential Logic ENEL 111 1 7 3 Sequential Logic Circuits So far we have only considered circuits where the output is purely a function of the inputs With sequential circuits the output is a function of the values of past and present inputs This particular example is not very useful
1.38k views • 37 slides
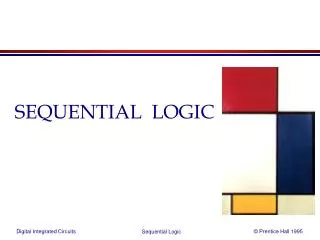
SEQUENTIAL LOGIC
SEQUENTIAL LOGIC. Sequential Logic. Positive Feedback: Bi-Stability. Meta-Stability. Gain should be larger than 1 in the transition region. R. S. Q. Q. S. 0. Q. 0. Q. S. Q. 0. 0. 1. 1. Q. R. 0. 1. 0. 1. R. 1. 0. 1. 0. S. Q. Q. R. SR-Flip Flop. Q. Q. R. S. Q.
596 views • 29 slides
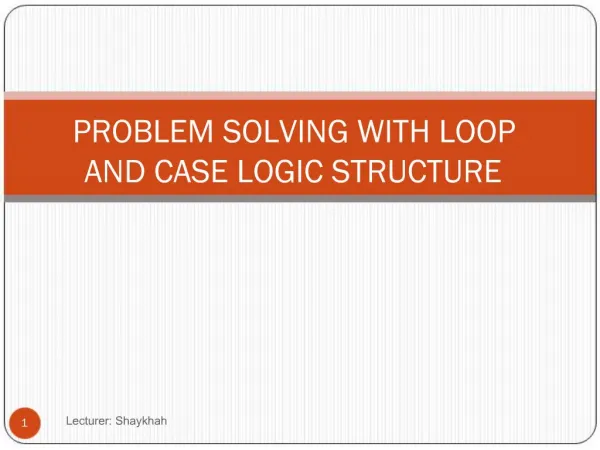
PROBLEM SOLVING WITH LOOP AND CASE LOGIC STRUCTURE
Shaykhah. PROBLEM SOLVING WITH LOOP AND CASE LOGIC STRUCTURE. Lecturer: Shaykhah. 2. Objective:Develop problems using the loop logic structure Using nested loop constructsDistinguish the different uses of three loop constructs.. Shaykhah. Pre-requisites. Lecturer: Shaykhah. 3. Short hand Assi
567 views • 23 slides
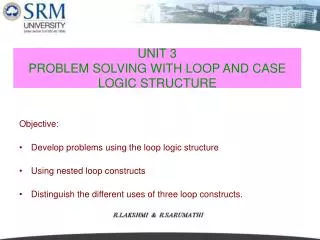
UNIT 3 PROBLEM SOLVING WITH LOOP AND CASE LOGIC STRUCTURE
UNIT 3 PROBLEM SOLVING WITH LOOP AND CASE LOGIC STRUCTURE. Objective: Develop problems using the loop logic structure Using nested loop constructs Distinguish the different uses of three loop constructs. Pre-requisites. Short hand Assignment Operators
915 views • 22 slides
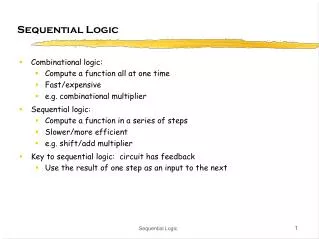
Sequential Logic. Combinational logic: Compute a function all at one time Fast/expensive e.g. combinational multiplier Sequential logic: Compute a function in a series of steps Slower/more efficient e.g. shift/add multiplier Key to sequential logic: circuit has feedback
319 views • 15 slides
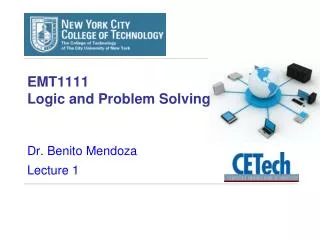
EMT1111 Logic and Problem Solving
Dr. Benito Mendoza Lecture 1. EMT1111 Logic and Problem Solving. Outline. The professor Class objectives Text book and references In class conduct Homework, assignments, and evaluation Blackboard Basic computer skills you should know Create your portfolio. The Professor.
297 views • 20 slides
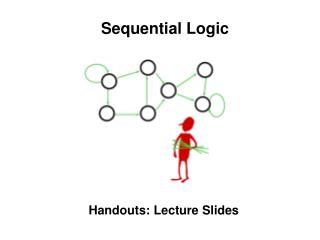
Sequential Logic. Handouts: Lecture Slides. 6.004: Progress so far…. PHYSICS: Continuous variables, Memory, Noise, f(RC) = 1 - e-t/R. COMBINATIONAL: Discrete, memoryless, noise-free, lookup table functions. What other building blocks do we need in order to compute?.
516 views • 41 slides
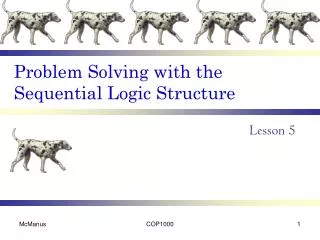
Problem Solving with the Sequential Logic Structure. Lesson 5. Overview. Algorithm Instructions Sequential Logic Structure Solution Development. Flowchart Symbols. Terminal Starts, Stops, Ends Input/Output Input data, Output information Assign Apply values to variables Process
403 views • 27 slides
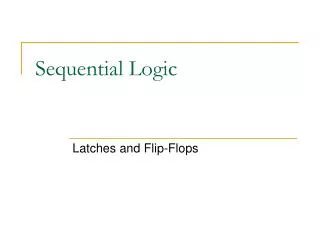
Sequential Logic. Latches and Flip-Flops. Sequential Logic Circuits. The output of sequential logic circuits depends on the past history of the state of the outputs. Therefore, it incorporate a memory element (circuits must remember the past states of their outputs).
490 views • 31 slides
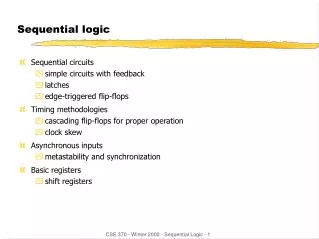
Sequential logic
Sequential logic. Sequential circuits simple circuits with feedback latches edge-triggered flip-flops Timing methodologies cascading flip-flops for proper operation clock skew Asynchronous inputs metastability and synchronization Basic registers shift registers. Sequential circuits.
622 views • 46 slides
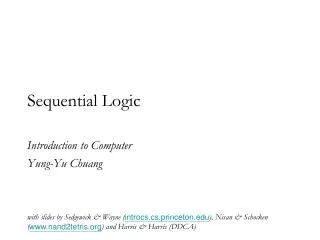
Sequential Logic. Introduction to Computer Yung-Yu Chuang. with slides by Sedgewick & Wayne ( introcs.cs.princeton.edu ), Nisan & Schocken ( www.nand2tetris.org ) and Harris & Harris (DDCA). ALU combinational. Memory state. Review of Combinational Circuits. Combinational circuits.
681 views • 51 slides
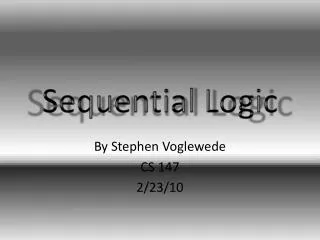
Sequential Logic. By Stephen Voglewede CS 147 2/23/10. What is Sequential Logic?. Combinational Logic. Combinational Logic. The output of a combinational circuit is a function of it’s input. Consequently, it doesn’t matter what the previous output was. Or what the future output will be.
332 views • 20 slides
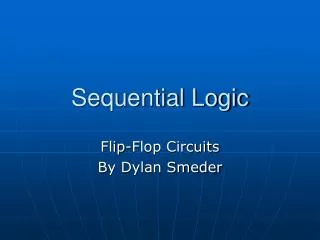
Sequential Logic. Flip-Flop Circuits By Dylan Smeder. What Is A Sequential Circuit?. Circuit that has memory Sequence of inputs creates memory At the simplest a Flip-Flop circuit “Sequential Circuits along with combinational circuits are the building blocks of computers”. Simple memory.
532 views • 16 slides
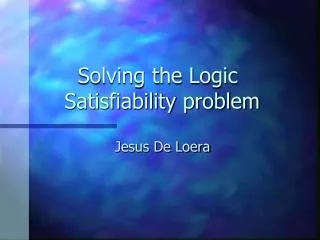
Solving the Logic Satisfiability problem
Solving the Logic Satisfiability problem. Jesus De Loera. Overview. The Satisfiability Problem in its most general form is determining whether it is possible to satisfy a given set of logical constraints Could take many forms: Boolean expressions & logic circuits
294 views • 12 slides
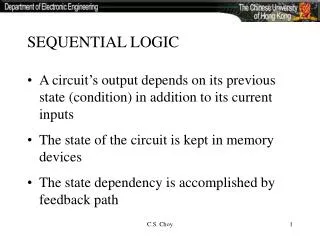
SEQUENTIAL LOGIC. A circuit’s output depends on its previous state (condition) in addition to its current inputs The state of the circuit is kept in memory devices The state dependency is accomplished by feedback path. LATCH. Active Low. LATCH. Active High. LATCH APPLICATION.
526 views • 40 slides
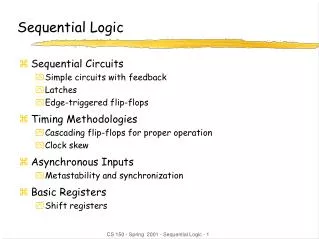
Sequential Logic. Sequential Circuits Simple circuits with feedback Latches Edge-triggered flip-flops Timing Methodologies Cascading flip-flops for proper operation Clock skew Asynchronous Inputs Metastability and synchronization Basic Registers Shift registers. Sequential Circuits.
613 views • 46 slides
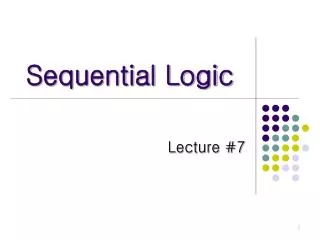
Sequential Logic. Lecture #7. 강의순서. Latch FlipFlop Active-high Clock & asynchronous Clear Active-low Clock & asynchronous Clear Active-high Clock & asynchronous Preset Active-high Clock & asynchronous Clear & Preset Shift Register Counter 4 bits Universal Counter : 74161
751 views • 49 slides
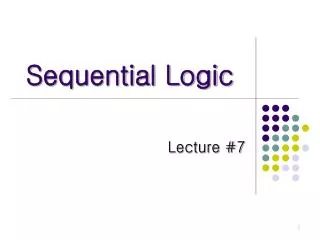
Sequential Logic. Lecture #7. 강의순서. Latch FlipFlop Shift Register Counter. SR Latch. Most simple “ storage element ” . Q next = (R + Q ’ current ) ’ Q ’ next = (S + Q current ) ’. NOR. In1 In2 Out 0 0 1 0 1 0 1 0 0 1 1 0. Function Table. Storing. SR latches are sequential.
950 views • 64 slides
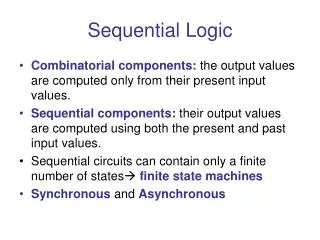
Sequential Logic. Combinatorial components: the output values are computed only from their present input values. Sequential components: their output values are computed using both the present and past input values.
366 views • 26 slides
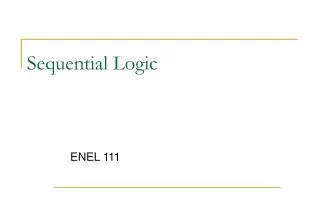
Sequential Logic. ENEL 111. 1. 7. 3. Sequential Logic Circuits. So far we have only considered circuits where the output is purely a function of the inputs With sequential circuits the output is a function of the values of past and present inputs This particular example is not very useful.
466 views • 37 slides
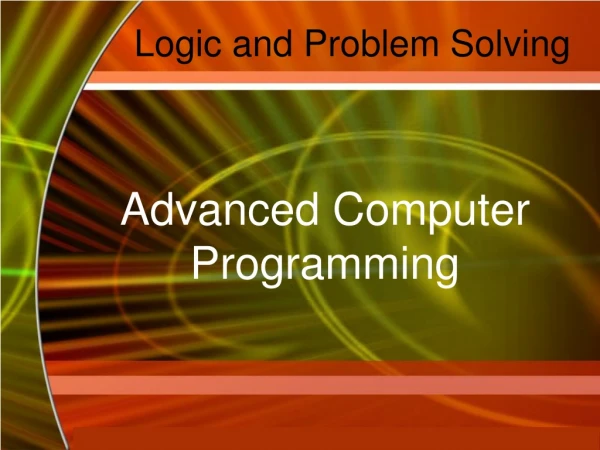
Logic and Problem Solving
Logic and Problem Solving. Advanced Computer Programming. Lecture Objective. After completing this Lecture: We will be able to apply the six-step approach to problem-solving. Enabling Objectives. Identify the six steps in problem solving Describe the purpose of each step in problem solving
299 views • 28 slides
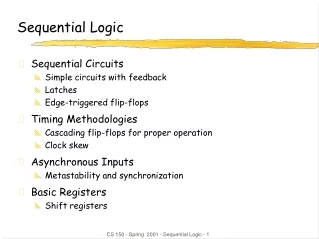
519 views • 46 slides

Decoding Algorithm Flowchart: Your Comprehensive Guide
3 minutes read
In the world of computational logic and programming, the concept of algorithms holds supreme significance. Algorithms, like the C language , provide a set of instructions to accomplish a specific task. To make these complex sets of instructions more comprehensible, professionals use an illustrative tool known as an algorithm flowchart.
In this article, you’ll learn all aspects of the algorithm flowchart and the tutorial on how to draw one based on your needs. Let’s get started!
What Is an Algorithm Flowchart
An algorithm flowchart is a diagrammatic representation that depicts the step-by-step procedure of an algorithm. Each step is symbolized by a specific shape, with arrows guiding the order of operations. These flowcharts simplify complex algorithms into visually understandable forms, enabling programmers, project managers, and even non-technical stakeholders to grasp the functionality of an algorithm quickly and efficiently.
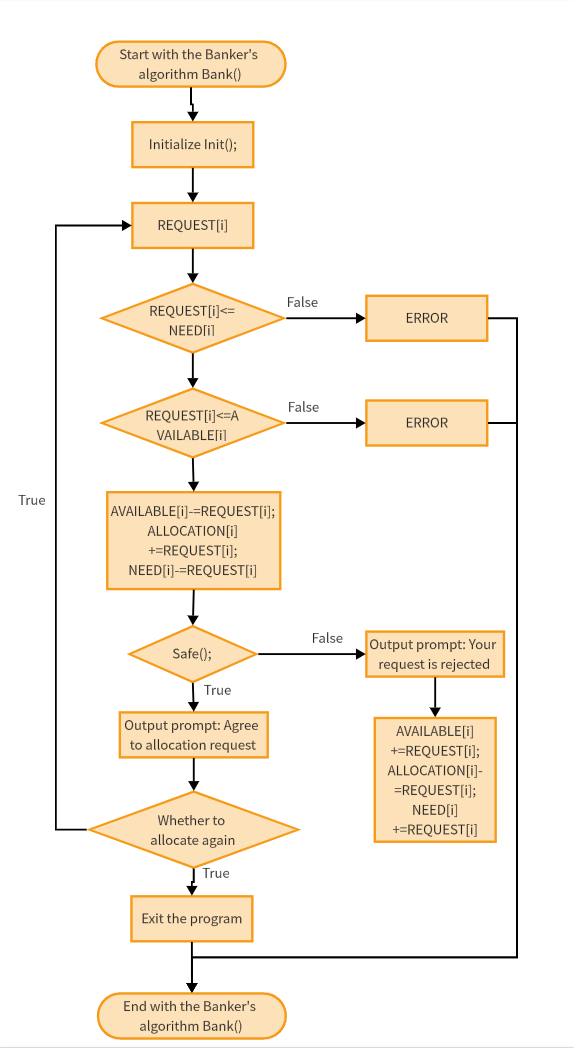
Why Use Flowcharts for Algorithm
Flowcharts serve as the bridge between technical complexity and human understanding, which is very important for the m odern computer science . Here's why they are particularly beneficial for representing algorithms:
Universal Comprehension
Regardless of linguistic or technical expertise, flowcharts are universally comprehensible. They replace abstract concepts and intricate codes with basic symbols and diagrams, ensuring everyone on the team understands the algorithm's functionality.
Error Detection
With a clear view of the entire algorithm process, identifying potential errors becomes considerably easier. Inconsistent steps, logic errors, or redundant procedures can be readily spotted and rectified.
Efficient Communication
Flowcharts serve as an effective communication tool, reducing misunderstandings and promoting clear, concise discussions about the algorithm among team members.
Benefits of an Algorithm Flowchart
Flowcharts offer numerous benefits for algorithm representation:
- Simplification of Complex Processes: Through their visual nature, flowcharts simplify complicated algorithmic processes into understandable sequences.
- Promotion of Logical Thinking: Flowcharts require the creator to think logically about the sequence of steps involved in executing the algorithm, promoting problem-solving and analytical skills.
- Efficient Documentation: Flowcharts serve as efficient documentation for future reference. They can be easily updated or modified as necessary without the need to delve into lines of code.
Different Types of Algorithm Flowcharts
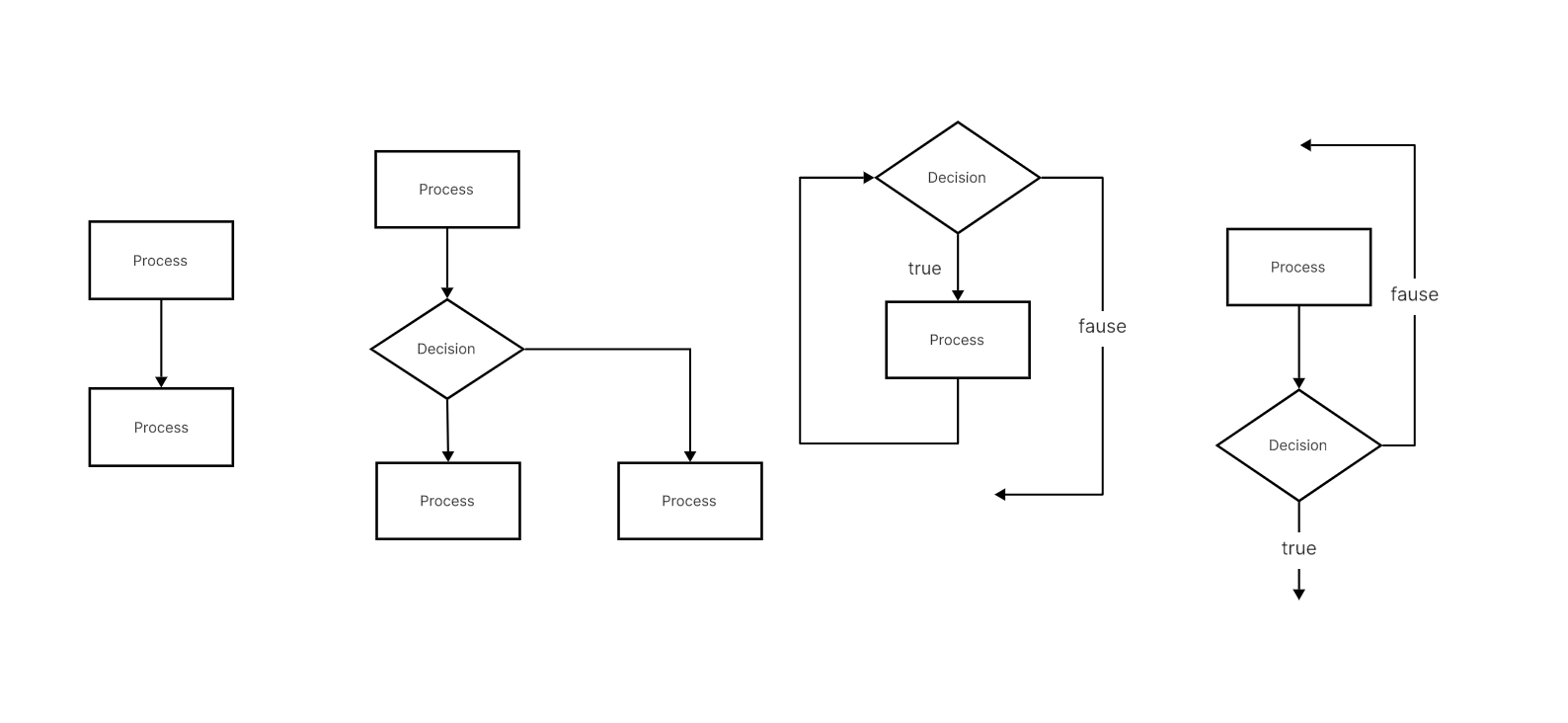
Flowchart for Linear Algorithms
Linear algorithms are sequential in nature, proceeding from one step to the next with no loops or decision points. The flowcharts for these algorithms are typically simple, with an easy-to-follow start-to-finish structure.
Flowchart for Branching Algorithms
These flowcharts are used for algorithms involving decision-making processes, usually represented by a diamond-shaped box in the chart. Each branch stemming from this box represents a possible decision or condition, leading to different paths through the algorithm.
Flowchart for Looping Algorithms
Looping algorithm flowcharts represent algorithms that involve iterative processes. This type is defined by the presence of a circular pathway within the flowchart, symbolizing the loop in the algorithm where certain operations are repeated until a specific condition is met.
Flowchart for Nested Algorithms
Nested algorithms have one or more algorithms residing within another algorithm. The flowcharts for nested algorithms contain layers of rectangles and decision points, illustrating the internal structures and interactions of the nested algorithms.
Flowchart for Recursive Algorithms
Recursive algorithm flowcharts are used to depict algorithms where a function calls itself within its definition. These flowcharts often use curved arrows looping back to illustrate recursion.
Flowchart for Parallel Algorithms
Parallel algorithm flowcharts represent algorithms designed for concurrent processing. These flowcharts contain simultaneous pathways to indicate multiple operations happening at once, generally running parallel to each other.
Flowchart for Distributed Algorithms
These charts are used for algorithms that run on multiple processors that might be distributed across a network or even geographically separated. Distributed algorithm flowcharts often depict a centralized controller node that delegates tasks to other nodes.
How to Draw an Algorithm Flowchart with Boardmix
Creating algorithm flowcharts has never been easier with Boardmix , a top-rated algorithm flowchart maker. Here's a simple guide on how to draw an algorithm flowchart using this intuitive tool:
Step 1: Define Your Algorithm
Before embarking on the flowchart creation process, it's crucial to define your algorithm. Understand the task, identify the steps required, and the sequence they should follow.
Step 2: Open Boardmix and Create a New Chart
With your algorithm set, open Boardmix. Navigate to the main dashboard and create a new board.
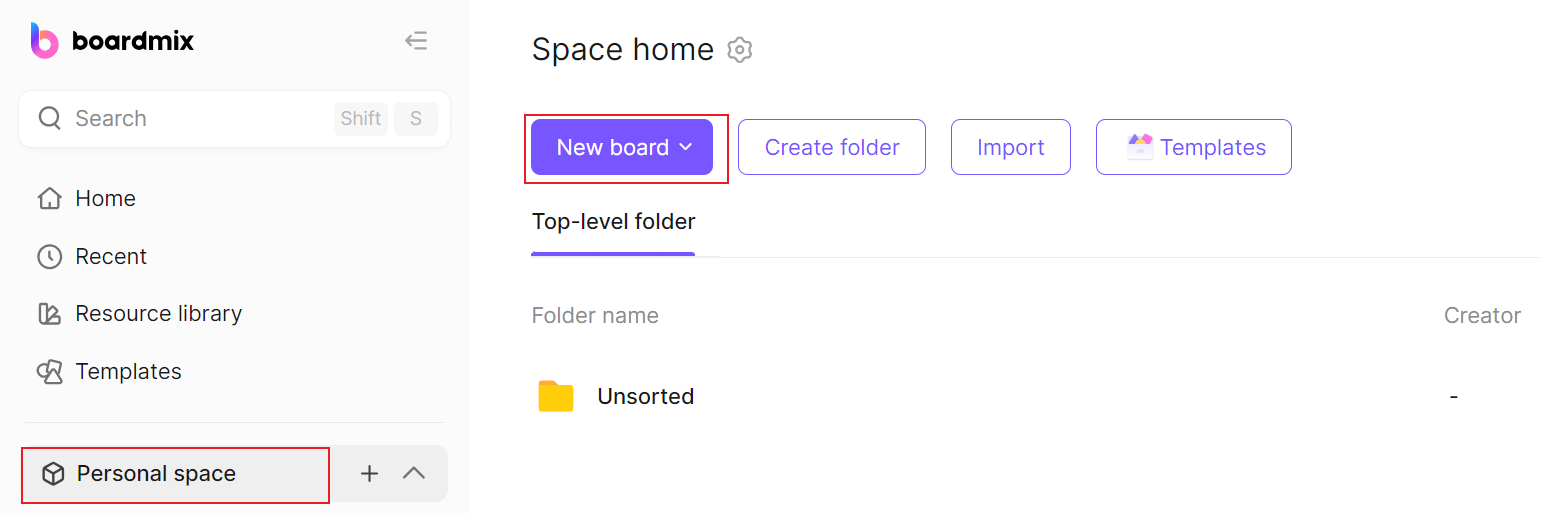
Step 3: Drag and Drop Shapes
From the shape library on the left of the screen, select the appropriate shape for each step of your algorithm and drag it onto the canvas. You can also choose a ready-made flowchart template from its library and customize it.
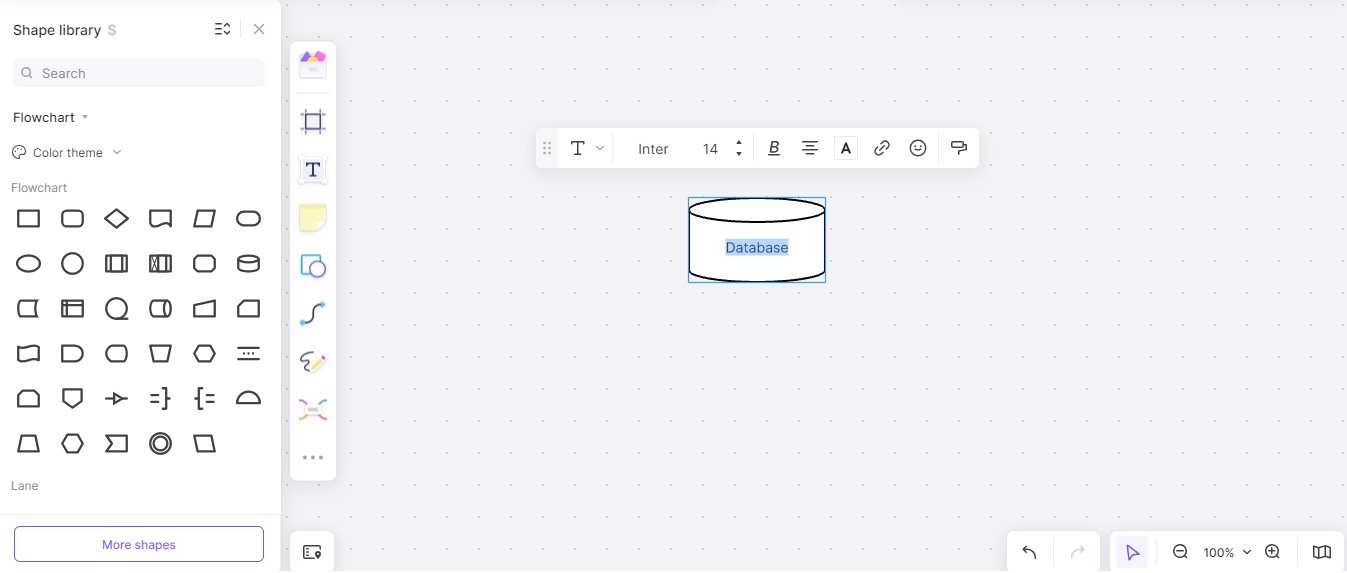
Step 4: Add Step Details
Click on each shape to add text detailing what each step in the algorithm does.
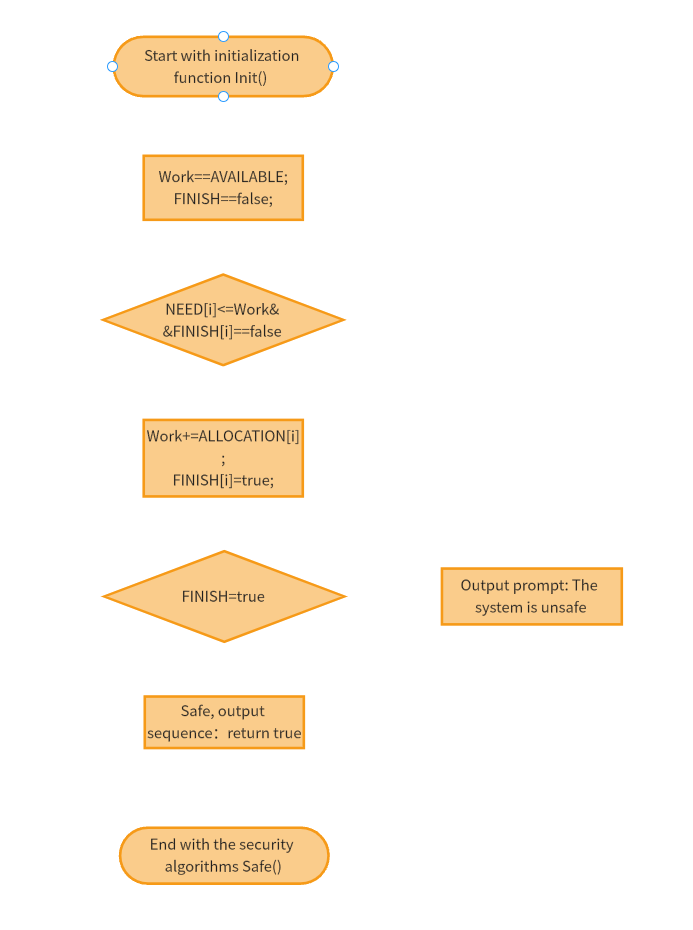
Step 5: Connect the Shapes
Use arrows from the shape library to connect the shapes in the order that the steps should follow.
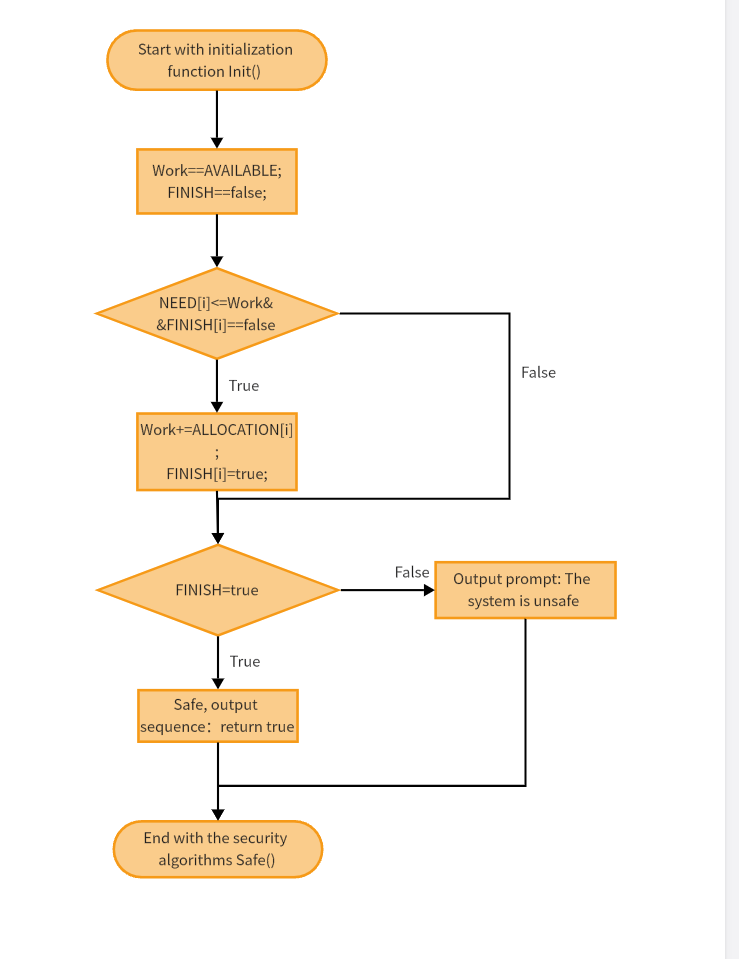
Step 6: Validate and Save
Ensure that your flowchart accurately represents your algorithm. Make necessary adjustments, then save your flowchart.
Mistakes to Avoid When Creating Algorithm Flowcharts
While flowcharts are incredibly useful tools, their efficacy can be compromised by some common mistakes. Here's what you should avoid when creating algorithm flowcharts:
Oversimplification or Overcomplication
Striking a balance between simplicity and detail is key. While oversimplifying can omit important steps or decisions, overcomplicating can make the flowchart difficult to understand.
Lack of Clear Start and End Points
Every flowchart should have defined start and end points. Without these, it can be hard to follow the flowchart's sequence.
Improper Use of Symbols
Each symbol in a flowchart has a specific meaning. Using them incorrectly can lead to confusion and misinterpretation of the algorithm.
Failure to Validate
Before finalizing your flowchart, validate it against your defined algorithm. Ensure every step is included, and the sequence is correct.
In conclusion, creating an algorithm flowchart with Boardmix is as straightforward as it is rewarding. Avoiding common mistakes guarantees a flowchart that is accurate, clear, and valuable for understanding the underlying algorithm.
FAQ: What is the difference between algorithm and flowchart?
The difference between an algorithm and a flowchart lies in their nature and purpose.
An algorithm is a step-by-step procedure or a set of rules designed for solving a specific problem or completing a specific task.
It is expressed in natural language, pseudocode, or a programming language and focuses on the logic and sequence of steps required to achieve a result. Algorithms are independent of any specific programming language and are more abstract in nature.
A flowchart is a visual representation of an algorithm using different shapes and arrows to illustrate the flow of control.It provides a graphical representation of the steps or processes involved in an algorithm, making it easier to understand and analyze.
Flowcharts are helpful for visualizing the decision-making process and the overall structure of an algorithm.
In summary, an algorithm is a logical and sequential set of instructions, while a flowchart is a visual representation that helps illustrate the steps of an algorithm. The algorithm itself can be written in various forms, including natural language or programming code, while the flowchart serves as a visual aid to understand the algorithmic process.
Join Boardmix to collaborate with your team.
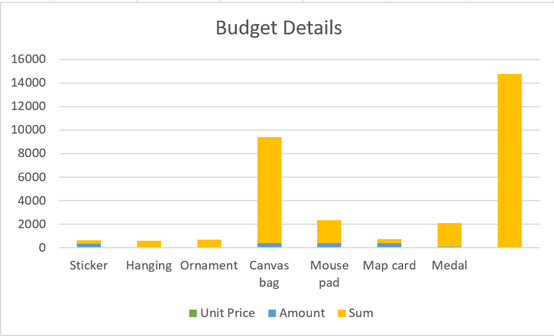
How to Make a Stacked Column Chart in Excel
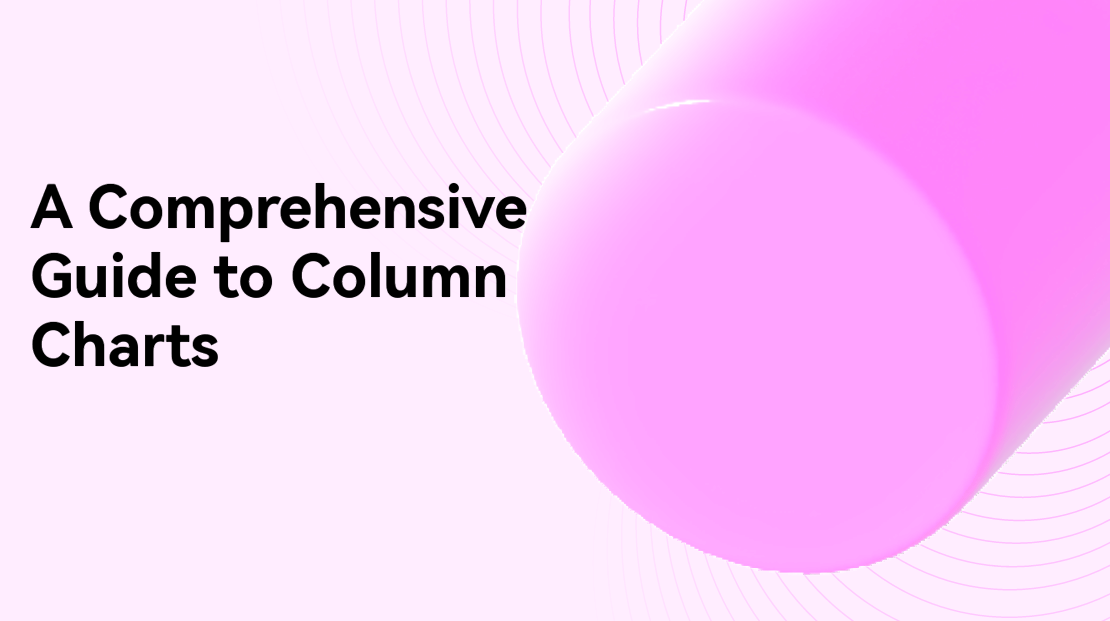
Mastering Data Visualization: A Comprehensive Guide to Column Charts
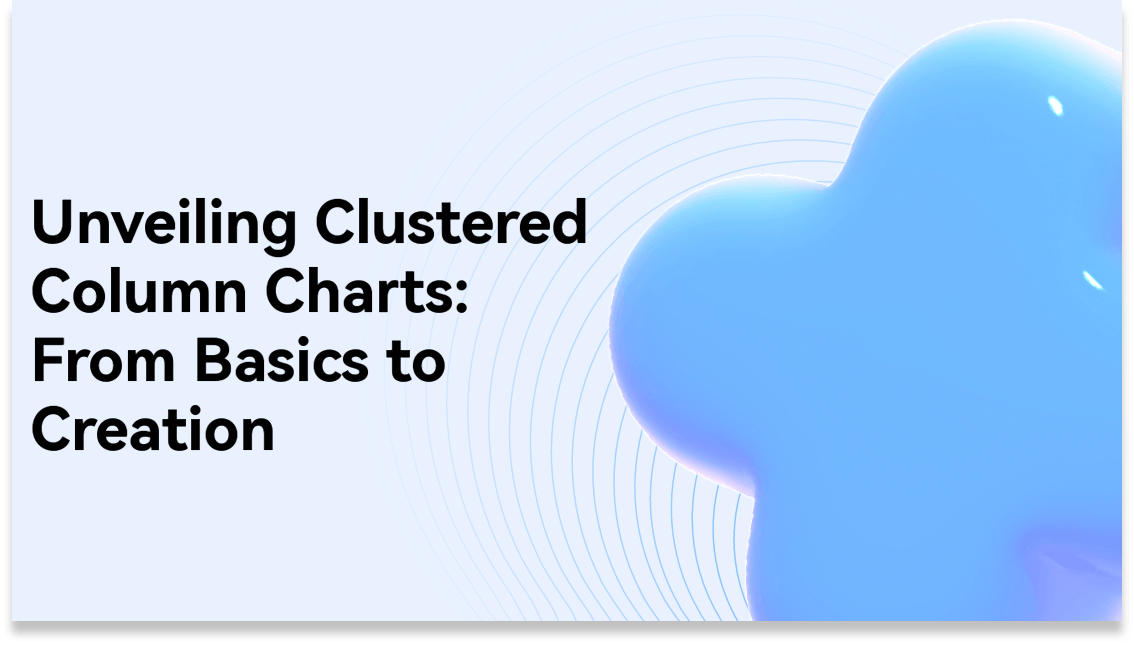
Unveiling Clustered Column Charts: From Basics to Creation
Popular Tutorials
Popular examples, learn python interactively, related articles.
- self in Python, Demystified
- Increment ++ and Decrement -- Operator as Prefix and Postfix
- Interpreter Vs Compiler : Differences Between Interpreter and Compiler
- Algorithm in Programming
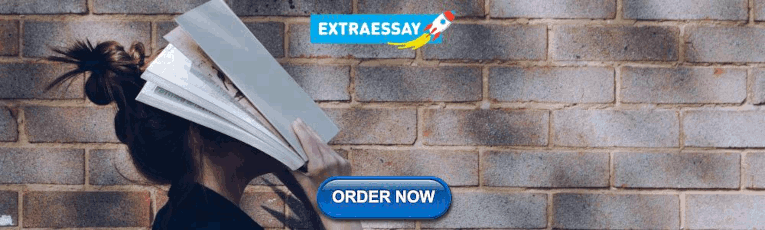
Flowchart In Programming
A flowchart is a diagrammatic representation of an algorithm. A flowchart can be helpful for both writing programs and explaining the program to others.
Symbols Used In Flowchart
Examples of flowcharts in programming.
1. Add two numbers entered by the user.
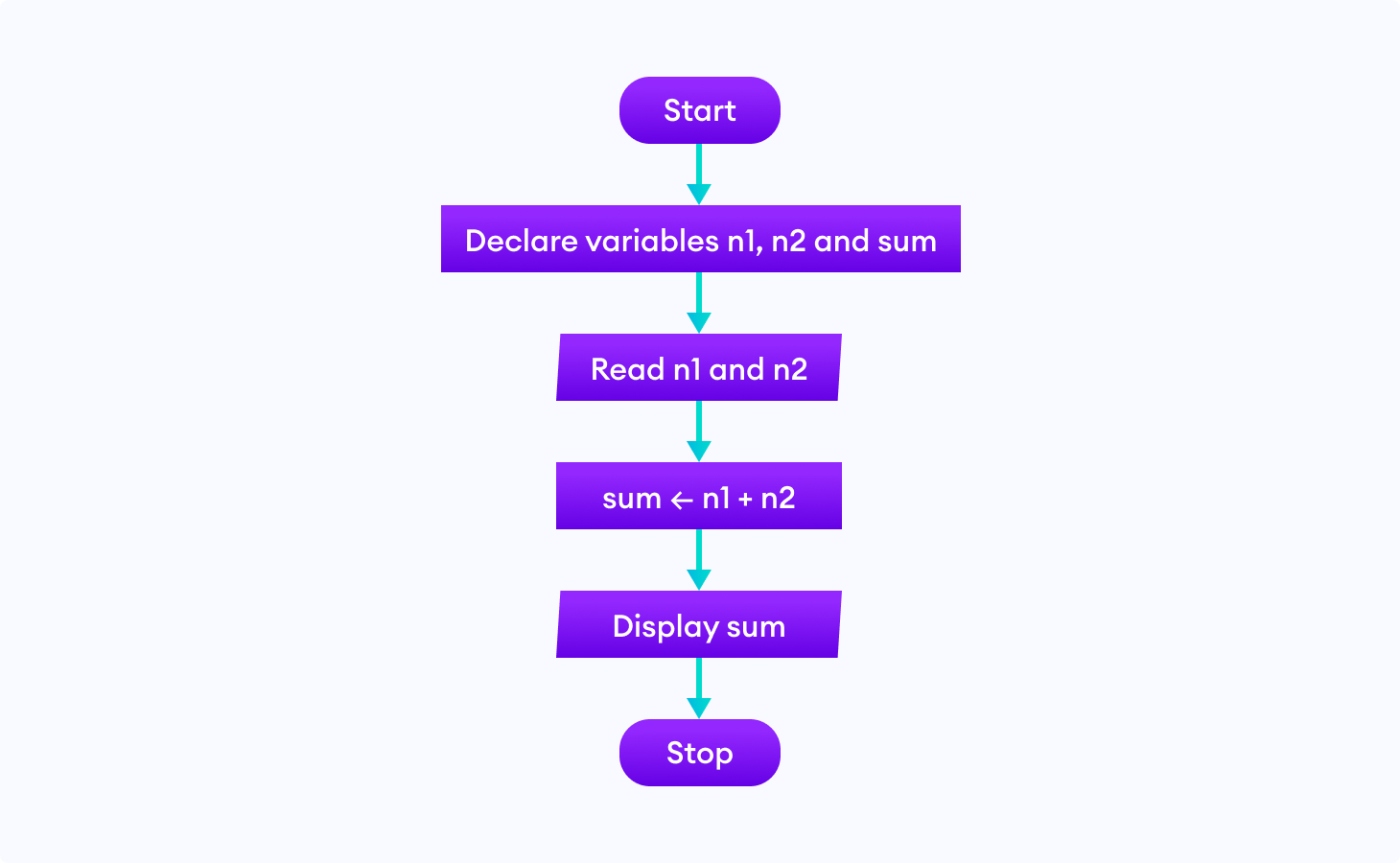
2. Find the largest among three different numbers entered by the user.
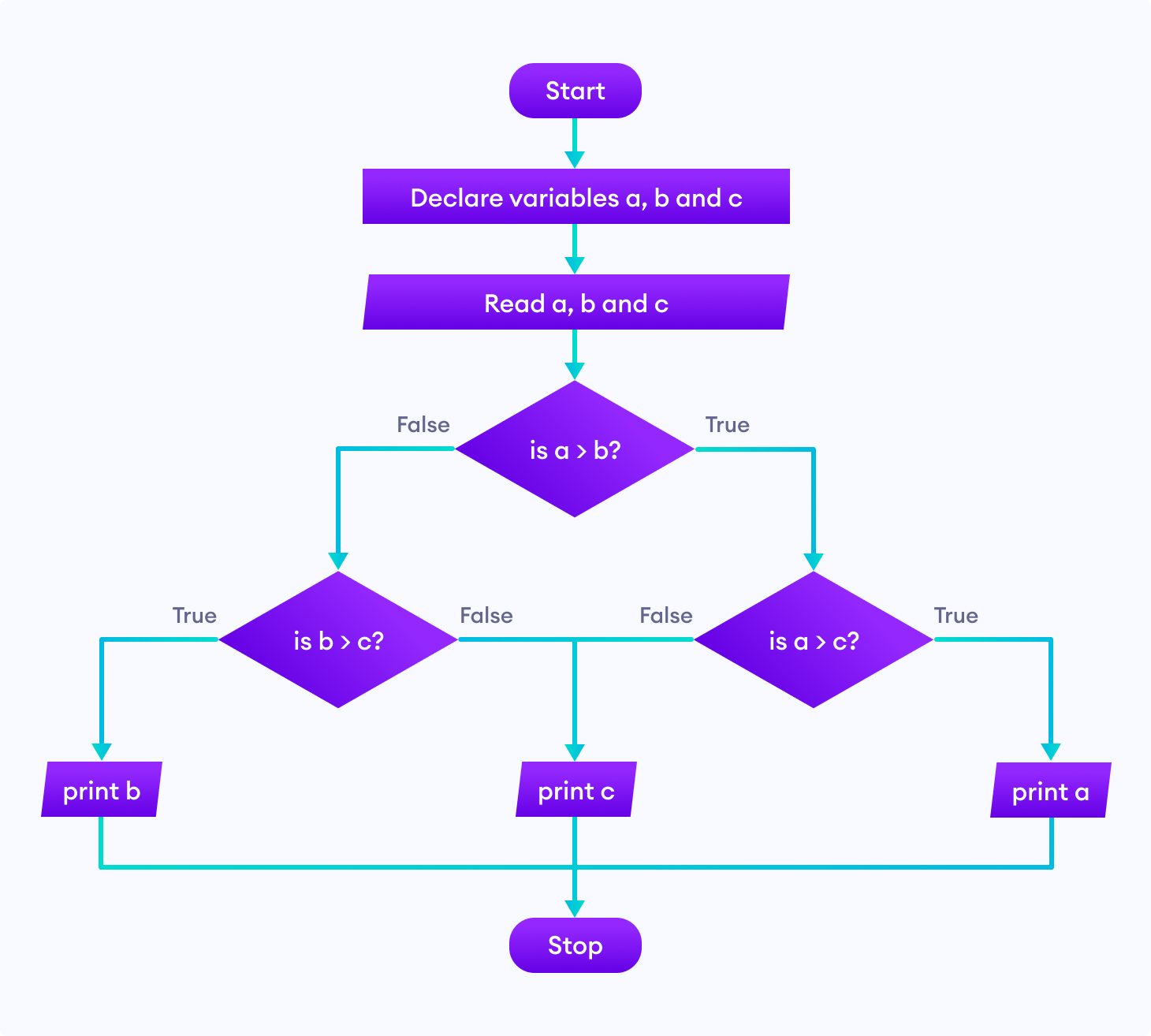
3. Find all the roots of a quadratic equation ax 2 +bx+c=0
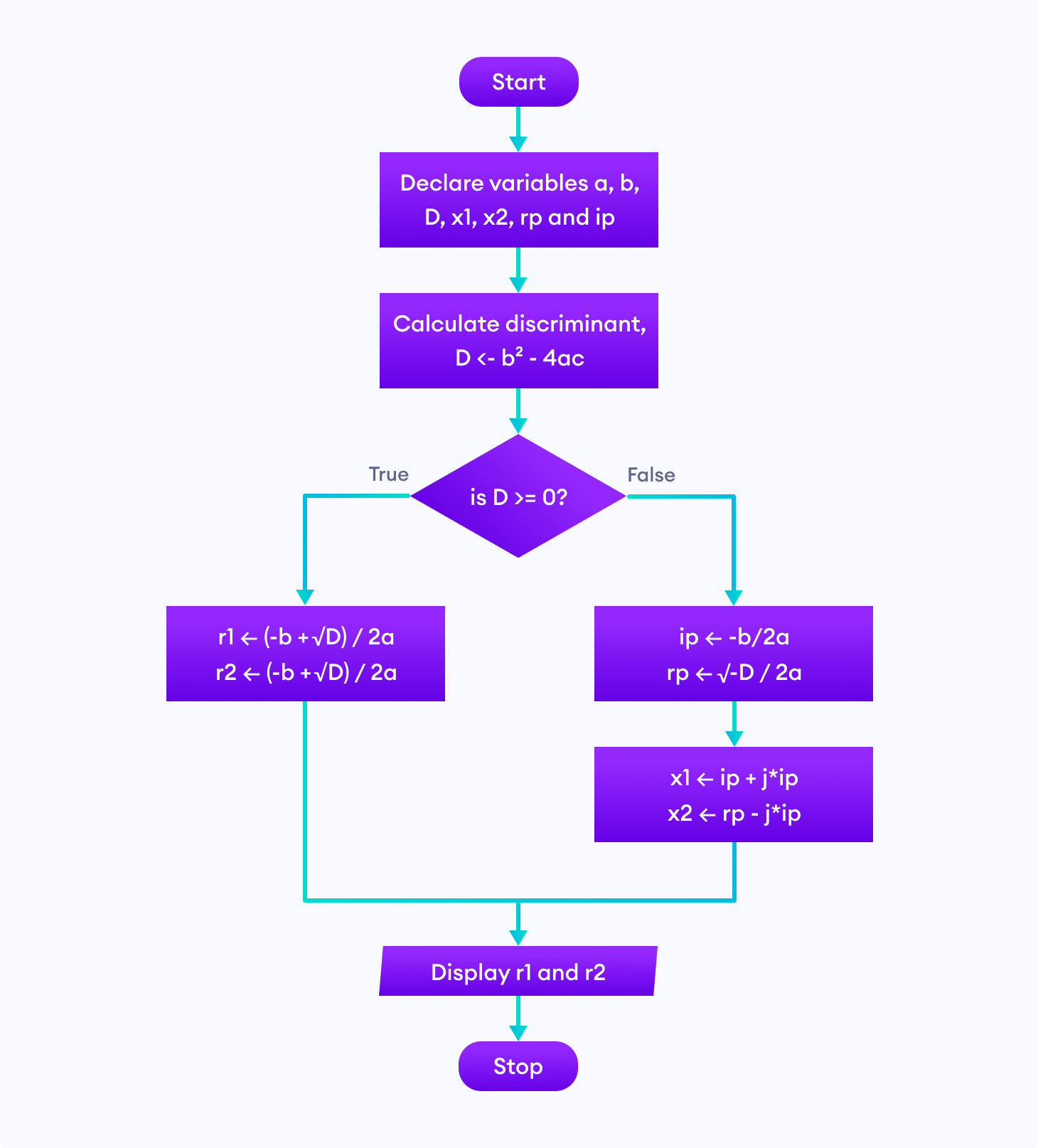
4. Find the Fibonacci series till term≤1000.
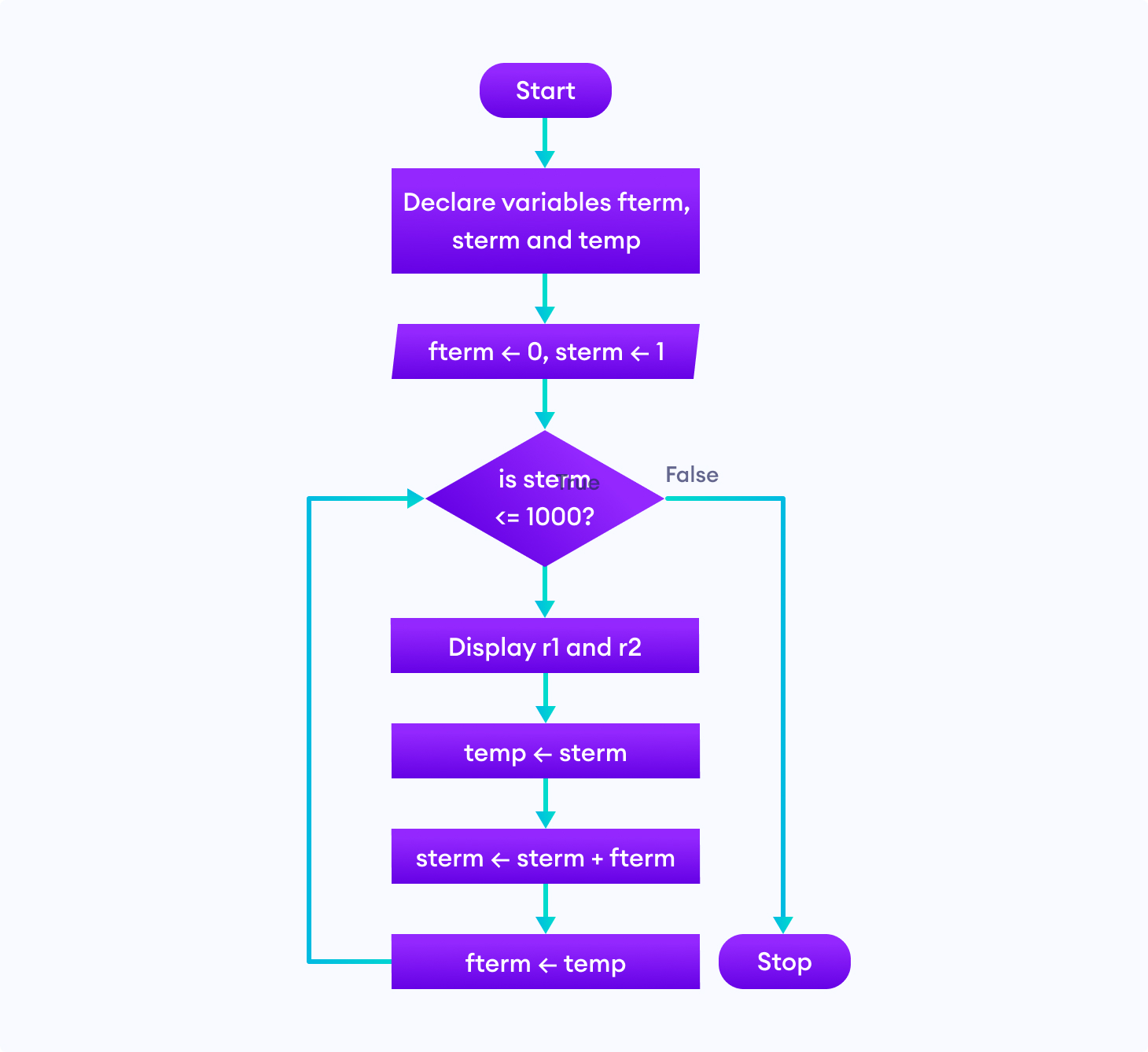
Note: Though flowcharts can be useful for writing and analyzing a program, drawing a flowchart for complex programs can be more complicated than writing the program itself. Hence, creating flowcharts for complex programs is often ignored.
Sorry about that.
- System Design Tutorial
- What is System Design
- System Design Life Cycle
- High Level Design HLD
- Low Level Design LLD
- Design Patterns
- UML Diagrams
- System Design Interview Guide
- Crack System Design Round
- System Design Bootcamp
- System Design Interview Questions
- Microservices
- Scalability
- Program to print diamond pattern using numbers and stars
- CBSE Class 11 | Computer Science - C++ Syllabus
- Office Tools and Domain Specific Tools
- CBSE Class 11 | Mobile Operating Systems - Symbian, Android and iOS
- Conceptual Model of the Unified Modeling Language (UML)
- CBSE Class 11 C++ Sample Paper-3
- CBSE Class 11 | Concepts of Programming Methodology
- CBSE Class 11 C++ | Sample Paper -2
- Open Source, Freeware and Shareware Softwares
- Domain Specific Tools
- Classification of Computers
- System Software
- Introduction to UCD - User centered design
- CBSE Class 11 | Problem Solving Methodologies
- Design Principles and Usability Heuristics
- Concept of Comments in Computer Programming
- Modular Approach in Programming
- Types of Operating Systems
An introduction to Flowcharts
What is a Flowchart? Flowchart is a graphical representation of an algorithm. Programmers often use it as a program-planning tool to solve a problem. It makes use of symbols which are connected among them to indicate the flow of information and processing. The process of drawing a flowchart for an algorithm is known as “flowcharting”.
Basic Symbols used in Flowchart Designs
- Terminal: The oval symbol indicates Start, Stop and Halt in a program’s logic flow. A pause/halt is generally used in a program logic under some error conditions. Terminal is the first and last symbols in the flowchart.
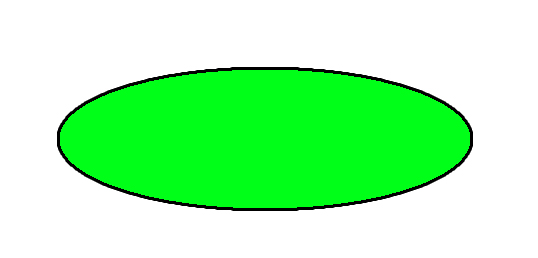
- Input/Output: A parallelogram denotes any function of input/output type. Program instructions that take input from input devices and display output on output devices are indicated with parallelogram in a flowchart.
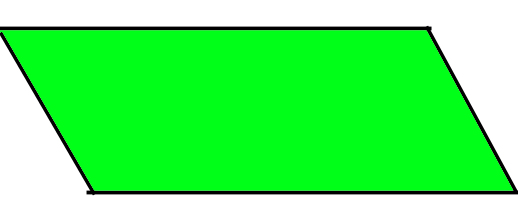
- Processing: A box represents arithmetic instructions. All arithmetic processes such as adding, subtracting, multiplication and division are indicated by action or process symbol.
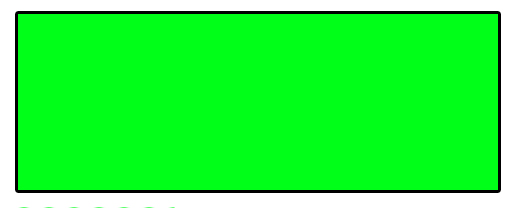
- Decision Diamond symbol represents a decision point. Decision based operations such as yes/no question or true/false are indicated by diamond in flowchart.
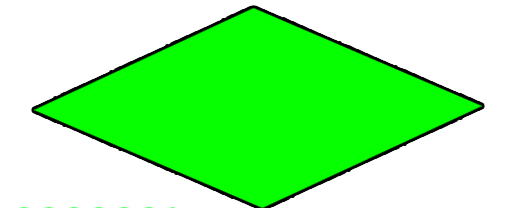
- Connectors: Whenever flowchart becomes complex or it spreads over more than one page, it is useful to use connectors to avoid any confusions. It is represented by a circle.
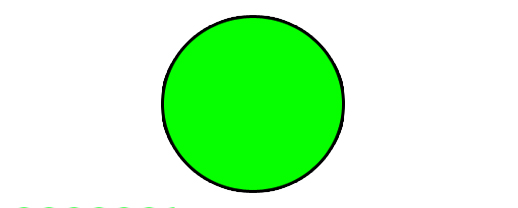
- Flow lines: Flow lines indicate the exact sequence in which instructions are executed. Arrows represent the direction of flow of control and relationship among different symbols of flowchart.
Rules For Creating Flowchart :
A flowchart is a graphical representation of an algorithm.it should follow some rules while creating a flowchart Rule 1: Flowchart opening statement must be ‘start’ keyword. Rule 2: Flowchart ending statement must be ‘end’ keyword. Rule 3: All symbols in the flowchart must be connected with an arrow line. Rule 4: The decision symbol in the flowchart is associated with the arrow line.
Advantages of Flowchart:
- Flowcharts are a better way of communicating the logic of the system.
- Flowcharts act as a guide for blueprint during program designed.
- Flowcharts help in debugging process.
- With the help of flowcharts programs can be easily analyzed.
- It provides better documentation.
- Flowcharts serve as a good proper documentation.
- Easy to trace errors in the software.
- Easy to understand.
- The flowchart can be reused for inconvenience in the future.
- It helps to provide correct logic.
Disadvantages of Flowchart:
- It is difficult to draw flowcharts for large and complex programs.
- There is no standard to determine the amount of detail.
- Difficult to reproduce the flowcharts.
- It is very difficult to modify the Flowchart.
- Making a flowchart is costly.
- Some developer thinks that it is waste of time.
- It makes software processes low.
- If changes are done in software, then the flowchart must be redrawn
Example : Draw a flowchart to input two numbers from the user and display the largest of two numbers
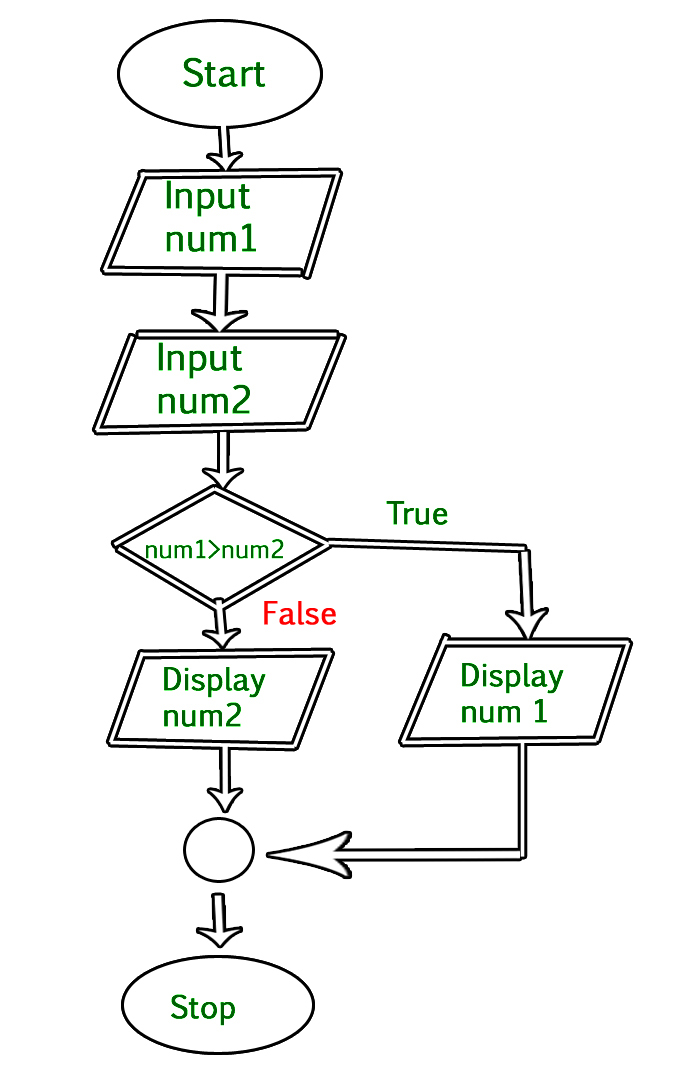
References: Computer Fundamentals by Pradeep K. Sinha and Priti Sinha
Please Login to comment...
- CBSE - Class 11
- school-programming
- Design Pattern
- School Programming
- System Design
- Technical Scripter
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
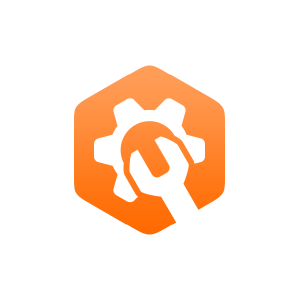
1.1: Activity 1 - Introduction to Algorithms and Problem Solving
- Last updated
- Save as PDF
- Page ID 48194
Introduction
In this learning activity section, the learner will be introduced to algorithms and how to write algorithms to solve tasks faced by learners or everyday problems. Examples of the algorithm are also provided with a specific application to everyday problems that the learner is familiar with. The learners will particularly learn what is an algorithm, the process of developing a solution for a given task, and finally examples of application of the algorithms are given.
An algorithm is a finite sequence of steps for accomplishing some computational task. It must
- Have steps that are simple and definite enough to be done by a computer, and
- Terminate after finitely many steps.
An algorithm can be considered as a computational procedure that consists of a set of instructions, that takes some value or set of values, as input, and produces some value or set of values, as output, as illustrated in Figure 1.3.1 . It can also be described as a procedure that accepts data, manipulate them following the prescribed steps, so as to eventually fill the required unknown with the desired value(s). The concept of an algorithm is best illustrated by the example of a recipe, although many algorithms are much more complex; algorithms often have steps that repeat (iterate) or require decisions (such as logic or comparison) until the task is completed. Correctly performing an algorithm will not solve a problem if the algorithm is flawed or not appropriate to the problem. A recipe is a set of instructions that show how to prepare or make something, especially a culinary dish.
Different algorithms may complete the same task with a different set of instructions in more or less time, space, or effort than others. Algorithms are essential to the way computers process information, because a computer program is essentially an algorithm that tells the computer what specific steps to perform (in what specific order) in order to carry out a specified task.
Algorithmic problem solving comes in two phases. These include:
- derivation of an algorithm that solves the problem, and
- conversion of the algorithm into code.
It is worth noting that:
- an algorithm is a sequence of steps, not a program.
- same algorithm can be used in different programs, or the same algorithm can be expressed in different languages, because an algorithm is an entity that is abstracted from implementation details.
An algorithm can be expressed in the following ways:
- human language
- pseudo code
Example \(\PageIndex{1}\)
Problem: Given a list of positive numbers, return the largest number on the list.
Inputs: A list L of positive numbers. This list must contain at least one number.
Outputs: A number n, which will be the largest number of the list.
- Set max to 0.
- For each number x in the list L, compare it to max. If x is larger, set max to x.
- max is now set to the largest number in the list.
The learner was introduced to the concept of algorithms and the various ways he/she can develop a solution to a task. In particular, the learner was introduced to the definition/s of an algorithm, the three main ways of developing or expressing an algorithm which are the human language, Pseudo code and the flow chart. An example was also given to reinforce the concept of the algorithm.
- Outline the algorithmic steps that can be used to add two given numbers.
- By using an example, describe how the concept of algorithms can be well presented to a group of students being introduced to it.
Problem-Solving Flowchart: A Visual Method to Find Perfect Solutions
Lucid Content
Reading time: about 7 min
“People ask me questions Lost in confusion Well, I tell them there's no problem Only solutions” —John Lennon, “Watching the Wheels”
Despite John Lennon’s lyrics, nobody is free from problems, and that’s especially true in business. Chances are that you encounter some kind of problem at work nearly every day, and maybe you’ve had to “put out a fire” before lunchtime once or twice in your career.
But perhaps what Lennon’s saying is that, no matter what comes our way, we can find solutions. How do you approach problems? Do you have a process in place to ensure that you and your co-workers come to the right solution?
In this article, we will give you some tips on how to find solutions visually through a problem-solving flowchart and other methods.
What is visual problem-solving?
If you are a literal thinker, you may think that visual problem-solving is something that your ophthalmologist does when your vision is blurry. For the rest of us, visual problem-solving involves executing the following steps in a visual way:
- Define the problem.
- Brainstorm solutions.
- Pick a solution.
- Implement solutions.
- Review the results.
How to make your problem-solving process more visual
Words pack a lot of power and are very important to how we communicate on a daily basis. Using words alone, you can brainstorm, organize data, identify problems, and come up with possible solutions. The way you write your ideas may make sense to you, but it may not be as easy for other team members to follow.
When you use flowcharts, diagrams, mind maps, and other visuals, the information is easier to digest. Your eyes dart around the page quickly gathering information, more fully engaging your brain to find patterns and make sense of the data.
Identify the problem with mind maps
So you know there is a problem that needs to be solved. Do you know what that problem is? Is there only one problem? Is the problem sum total of a bunch of smaller problems?
You need to ask these kinds of questions to be sure that you are working on the root of the issue. You don’t want to spend too much time and energy solving the wrong problem.
To help you identify the problem, use a mind map. Mind maps can help you visually brainstorm and collect ideas without a strict organization or structure. A mind map more closely aligns with the way a lot of our brains work—participants can bounce from one thought to the next defining the relationships as they go.

Mind mapping to solve a problem includes, but is not limited to, these relatively easy steps:
- In the center of the page, add your main idea or concept (in this case, the problem).
- Branch out from the center with possible root causes of the issue. Connect each cause to the central idea.
- Branch out from each of the subtopics with examples or additional details about the possible cause. As you add more information, make sure you are keeping the most important ideas closer to the main idea in the center.
- Use different colors, diagrams, and shapes to organize the different levels of thought.
Alternatively, you could use mind maps to brainstorm solutions once you discover the root cause. Search through Lucidchart’s mind maps template library or add the mind map shape library to quickly start your own mind map.
Create a problem-solving flowchart
A mind map is generally a good tool for non-linear thinkers. However, if you are a linear thinker—a person who thinks in terms of step-by-step progression making a flowchart may work better for your problem-solving strategy. A flowchart is a graphical representation of a workflow or process with various shapes connected by arrows representing each step.
Whether you are trying to solve a simple or complex problem, the steps you take to solve that problem with a flowchart are easy and straightforward. Using boxes and other shapes to represent steps, you connect the shapes with arrows that will take you down different paths until you find the logical solution at the end.

Flowcharts or decision trees are best used to solve problems or answer questions that are likely to come up multiple times. For example, Yoder Lumber , a family-owned hardwood manufacturer, built decision trees in Lucidchart to demonstrate what employees should do in the case of an injury.
To start your problem-solving flowchart, follow these steps:
- Draw a starting shape to state your problem.
- Draw a decision shape where you can ask questions that will give you yes-or-no answers.
- Based on the yes-or-no answers, draw arrows connecting the possible paths you can take to work through the steps and individual processes.
- Continue following paths and asking questions until you reach a logical solution to the stated problem.
- Try the solution. If it works, you’re done. If it doesn’t work, review the flowchart to analyze what may have gone wrong and rework the flowchart until you find the solution that works.
If your problem involves a process or workflow , you can also use flowcharts to visualize the current state of your process to find the bottleneck or problem that’s costing your company time and money.

Lucidchart has a large library of flowchart templates to help you analyze, design, and document problem-solving processes or any other type of procedure you can think of.
Draw a cause-and-effect diagram
A cause-and-effect diagram is used to analyze the relationship between an event or problem and the reason it happened. There is not always just one underlying cause of a problem, so this visual method can help you think through different potential causes and pinpoint the actual cause of a stated problem.
Cause-and-effect diagrams, created by Kaoru Ishikawa, are also known as Ishikawa diagrams, fishbone diagrams , or herringbone diagrams (because they resemble a fishbone when completed). By organizing causes and effects into smaller categories, these diagrams can be used to examine why things went wrong or might go wrong.

To perform a cause-and-effect analysis, follow these steps.
1. Start with a problem statement.
The problem statement is usually placed in a box or another shape at the far right of your page. Draw a horizontal line, called a “spine” or “backbone,” along the center of the page pointing to your problem statement.
2. Add the categories that represent possible causes.
For example, the category “Materials” may contain causes such as “poor quality,” “too expensive,” and “low inventory.” Draw angled lines (or “bones”) that branch out from the spine to these categories.
3. Add causes to each category.
Draw as many branches as you need to brainstorm the causes that belong in each category.
Like all visuals and diagrams, a cause-and-effect diagram can be as simple or as complex as you need it to be to help you analyze operations and other factors to identify causes related to undesired effects.
Collaborate with Lucidchart
You may have superior problem-solving skills, but that does not mean that you have to solve problems alone. The visual strategies above can help you engage the rest of your team. The more involved the team is in the creation of your visual problem-solving narrative, the more willing they will be to take ownership of the process and the more invested they will be in its outcome.
In Lucidchart, you can simply share the documents with the team members you want to be involved in the problem-solving process. It doesn’t matter where these people are located because Lucidchart documents can be accessed at any time from anywhere in the world.
Whatever method you decide to use to solve problems, work with Lucidchart to create the documents you need. Sign up for a free account today and start diagramming in minutes.
Lucidchart, a cloud-based intelligent diagramming application, is a core component of Lucid Software's Visual Collaboration Suite. This intuitive, cloud-based solution empowers teams to collaborate in real-time to build flowcharts, mockups, UML diagrams, customer journey maps, and more. Lucidchart propels teams forward to build the future faster. Lucid is proud to serve top businesses around the world, including customers such as Google, GE, and NBC Universal, and 99% of the Fortune 500. Lucid partners with industry leaders, including Google, Atlassian, and Microsoft. Since its founding, Lucid has received numerous awards for its products, business, and workplace culture. For more information, visit lucidchart.com.
Related articles

Sometimes you're faced with challenges that traditional problem solving can't fix. Creative problem solving encourages you to find new, creative ways of thinking that can help you overcome the issue at hand more quickly.

Dialogue mapping is a facilitation technique used to visualize critical thinking as a group. Learn how you and your team can start dialogue mapping today to solve problems and bridge gaps in knowledge and understanding (plus get a free template!).
Bring your bright ideas to life.
or continue with
- Create more than 280 types of diagrams
- Supply more than 1500 built-in templates and 26,000 symbols
- Share your work and collaborate with your team in any file format
- What is a Flowchart
- How to Draw a FlowChart
- Flowchart Symbol and Their Usage
- Flowchart Examples & Templates
- Free Flowchart Maker
- What is a Floor Plan
- How to Draw Your Own Floor Plan
- Floor Plan Symbols
- Floor Plan Examples & Templates
- Floor Plan Maker
- What is a UML Diagram
- How to Create A UML Class Diagram
- UML Diagram Symbol
- UML Diagram Examples & Templates
- UML Diagram Tool
- Wiring Diagram
- Circuit Diagram
- How to Create a Basic Electrical Diagram
- Basic Electrical Symbols and Their Usage
- Electrical Drawing Software
- What is a Mind Map
- How to Create A Mind Map on Microsoft Word
- Mind Map Examples & Templates
- Free Mind Map Software
- What is an Organizational Chart
- How to Create an Org Chart
- Basic Organizational Chart Symbols
- Free Org Chart Templates
- Organizational Chart Software
- What is a P&ID
- How to Read P&ID
- How to Create a P&ID Drawing
- P&ID Symbol Legend
- P&ID Software
- What is a Gantt Chart
- What is a Milestone in a Gantt Chart
- How to Make a Gantt Chart
- Gantt Chart Examples
- Gantt Chart Symbols
- Gantt Chart Maker
- What is an Infographic
- 50+ Editable Infographic Templates
- How to Make an Infographic
- 7 Easy Tips to Make Your Infographics Stand Out
- Infographic Maker
- Diagram Center
Explain Algorithm and Flowchart with Examples
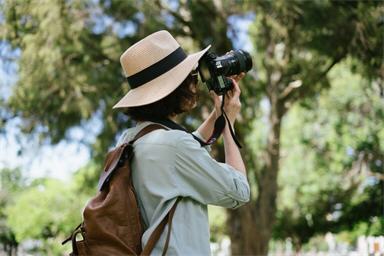
The algorithm and flowchart are two types of tools to explain the process of a program. In this page, we discuss the differences between an algorithm and a flowchart and how to create a flowchart to illustrate the algorithm visually. Algorithms and flowcharts are two different tools that are helpful for creating new programs, especially in computer programming. An algorithm is a step-by-step analysis of the process, while a flowchart explains the steps of a program in a graphical way.
In this article
Definition of algorithm, definition of flowchart, difference between algorithm and flowchart.
- Recursive Algorithm
- Divide and Conquer Algorithm
- Dynamic Programming Algorithm
- Greedy Algorithm
- Brute Force Algorithm
- Backtracking Algorithm
Use Flowcharts to Represent Algorithms
Writing a logical step-by-step method to solve the problem is called the algorithm . In other words, an algorithm is a procedure for solving problems. In order to solve a mathematical or computer problem, this is the first step in the process.
An algorithm includes calculations, reasoning, and data processing. Algorithms can be presented by natural languages, pseudocode, and flowcharts, etc.
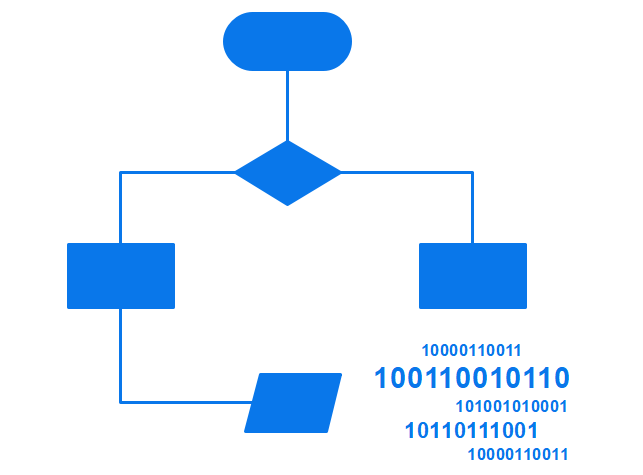
A flowchart is the graphical or pictorial representation of an algorithm with the help of different symbols, shapes, and arrows to demonstrate a process or a program. With algorithms, we can easily understand a program. The main purpose of using a flowchart is to analyze different methods. Several standard symbols are applied in a flowchart:
The symbols above represent different parts of a flowchart. The process in a flowchart can be expressed through boxes and arrows with different sizes and colors. In a flowchart, we can easily highlight certain elements and the relationships between each part.
During the reading of this article, if you find an icon type picture that you are interested in, you can download EdrawMax products to discover more or experience creating a free diagram of your own. All drawings are from EdrawMax .
If you compare a flowchart to a movie, then an algorithm is the story of that movie. In other words, an algorithm is the core of a flowchart . Actually, in the field of computer programming, there are many differences between algorithm and flowchart regarding various aspects, such as the accuracy, the way they display, and the way people feel about them. Below is a table illustrating the differences between them in detail.
- It is a procedure for solving problems.
- The process is shown in step-by-step instruction.
- It is complex and difficult to understand.
- It is convenient to debug errors.
- The solution is showcased in natural language.
- It is somewhat easier to solve complex problem.
- It costs more time to create an algorithm.
- It is a graphic representation of a process.
- The process is shown in block-by-block information diagram.
- It is intuitive and easy to understand.
- It is hard to debug errors.
- The solution is showcased in pictorial format.
- It is hard to solve complex problem.
- It costs less time to create a flowchart.

EdrawMax provides beginners and pros the cutting-edge functionalities to build professional-looking diagrams easier, faster, and cheaper! It allows you to create more than 280 types of diagrams and should be an excellent Visio alternative.
Types of Algorithm
It is not surprising that algorithms are widely used in computer programming. However, it can be applied to solving mathematical problems and even in everyday life. Here comes a question: how many types of algorithms? According to Dr. Christoph Koutschan, a computer scientist working at the Research Institute for Symbolic Computation (RISC) in Austria, he has surveyed voting for the important types of algorithms. As a result, he has listed 32 crucial algorithms in computer science. Despite the complexity of algorithms, we can generally divide algorithms into six fundamental types based on their function.
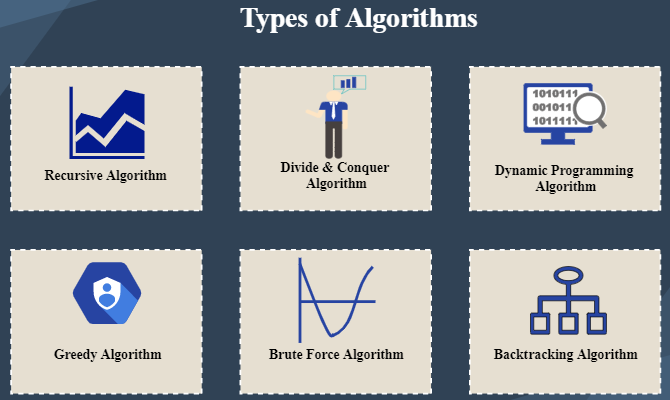
#1 Recursive Algorithm
It refers to a way to solve problems by repeatedly breaking down the problem into sub-problems of the same kind. The classic example of using a recursive algorithm to solve problems is the Tower of Hanoi.
#2 Divide and Conquer Algorithm
Traditionally, the divide and conquer algorithm consists of two parts: 1. breaking down a problem into some smaller independent sub-problems of the same type; 2. finding the final solution of the original issues after solving these more minor problems separately. The key points of the divide and conquer algorithm are:
- If you can find the repeated sub-problems and the loop substructure of the original problem, you may quickly turn the original problem into a small, simple issue.
- Try to break down the whole solution into various steps (different steps need different solutions) to make the process easier.
- Are sub-problems easy to solve? If not, the original problem may cost lots of time.
#3 Dynamic Programming Algorithm
Developed by Richard Bellman in the 1950s, the dynamic programming algorithm is generally used for optimization problems. In this type of algorithm, past results are collected for future use. Like the divide and conquer algorithm, a dynamic programming algorithm simplifies a complex problem by breaking it down into some simple sub-problems. However, the most significant difference between them is that the latter requires overlapping sub-problems, while the former doesn’t need to.
#4 Greedy Algorithm
This is another way of solving optimization problems – greedy algorithm. It refers to always finding the best solution in every step instead of considering the overall optimality. That is to say, what he has done is just at a local optimum. Due to the limitations of the greedy algorithm, it has to be noted that the key to choosing a greedy algorithm is whether to consider any consequences in the future.
#5 Brute Force Algorithm
The brute force algorithm is a simple and straightforward solution to the problem, generally based on the description of the problem and the definition of the concept involved. You can also use "just do it!" to describe the strategy of brute force. In short, a brute force algorithm is considered as one of the simplest algorithms, which iterates all possibilities and ends up with a satisfactory solution.
#6 Backtracking Algorithm
Based on a depth-first recursive search, the backtracking algorithm focusing on finding the solution to the problem during the enumeration-like searching process. When it cannot satisfy the condition, it will return "backtracking" and tries another path. It is suitable for solving large and complicated problems, which gains the reputation of the "general solution method". One of the most famous backtracking algorithm example it the eight queens puzzle.
Now that we have learned the definitions of algorithm and flowchart, how can we use a flowchart to represent an algorithm? To create an algorithm flowchart, we need to use a handy diagramming tool like EdrawMax to finish the work.
Algorithms are mainly used for mathematical and computer programs, whilst flowcharts can be used to describe all sorts of processes: business, educational, personal, and algorithms. So flowcharts are often used as a program planning tool to organize the program's step-by-step process visually. Here are some examples:
Example 1: Print 1 to 20:
- Step 1: Initialize X as 0,
- Step 2: Increment X by 1,
- Step 3: Print X,
- Step 4: If X is less than 20 then go back to step 2.
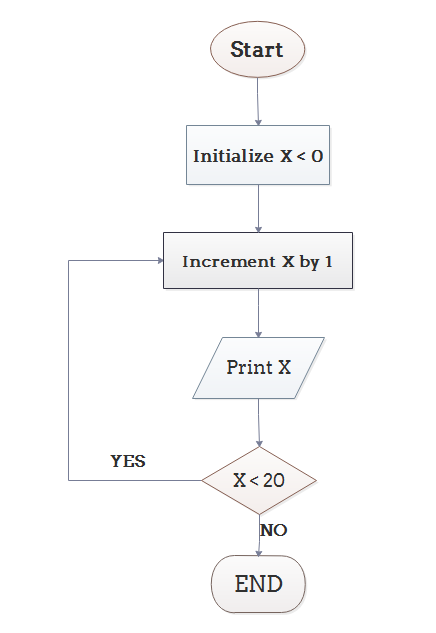
Example 2: Convert Temperature from Fahrenheit (℉) to Celsius (℃)
- Step 1: Read temperature in Fahrenheit,
- Step 2: Calculate temperature with formula C=5/9*(F-32),
- Step 3: Print C.
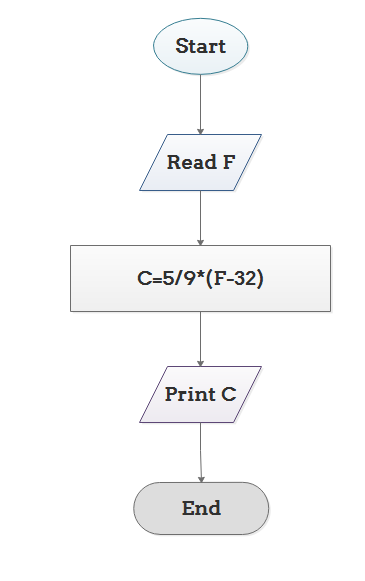
Example 3: Determine Whether A Student Passed the Exam or Not:
- Step 1: Input grades of 4 courses M1, M2, M3 and M4,
- Step 2: Calculate the average grade with formula "Grade=(M1+M2+M3+M4)/4"
- Step 3: If the average grade is less than 60, print "FAIL", else print "PASS".

From the above, we can come to the conclusion that a flowchart is a pictorial representation of an algorithm, an algorithm can be expressed and analyzed through a flowchart. An algorithm shows you every step of reaching the final solution, while a flowchart shows you how to carry out the process by connecting each step. An algorithm uses mainly words to describe the steps while you can create a flowchart with flowchart symbols to make the process more logical.
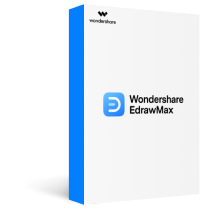
You May Also Like
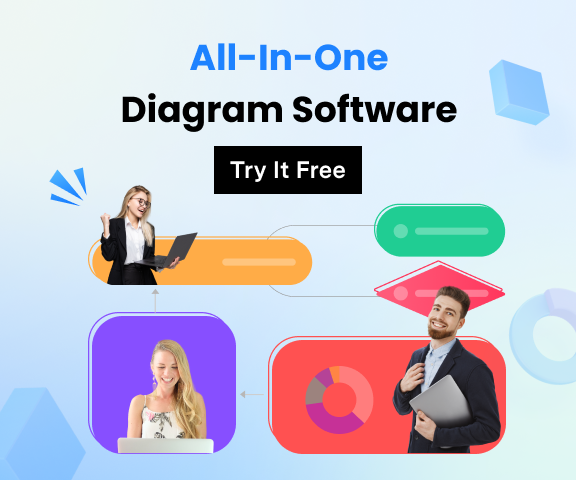
Related articles
Ada Computer Science
You need to enable JavaScript to access Ada Computer Science.
We use essential cookies to make Venngage work. By clicking “Accept All Cookies”, you agree to the storing of cookies on your device to enhance site navigation, analyze site usage, and assist in our marketing efforts.
Manage Cookies
Cookies and similar technologies collect certain information about how you’re using our website. Some of them are essential, and without them you wouldn’t be able to use Venngage. But others are optional, and you get to choose whether we use them or not.
Strictly Necessary Cookies
These cookies are always on, as they’re essential for making Venngage work, and making it safe. Without these cookies, services you’ve asked for can’t be provided.
Show cookie providers
- Google Login
Functionality Cookies
These cookies help us provide enhanced functionality and personalisation, and remember your settings. They may be set by us or by third party providers.
Performance Cookies
These cookies help us analyze how many people are using Venngage, where they come from and how they're using it. If you opt out of these cookies, we can’t get feedback to make Venngage better for you and all our users.
- Google Analytics
Targeting Cookies
These cookies are set by our advertising partners to track your activity and show you relevant Venngage ads on other sites as you browse the internet.
- Google Tag Manager
- Infographics
- Daily Infographics
- Graphic Design
- Graphs and Charts
- Data Visualization
- Human Resources
- Training and Development
- Beginner Guides
Blog Business
What is a Problem-Solving Flowchart & How to Make One
By Danesh Ramuthi , Aug 10, 2023
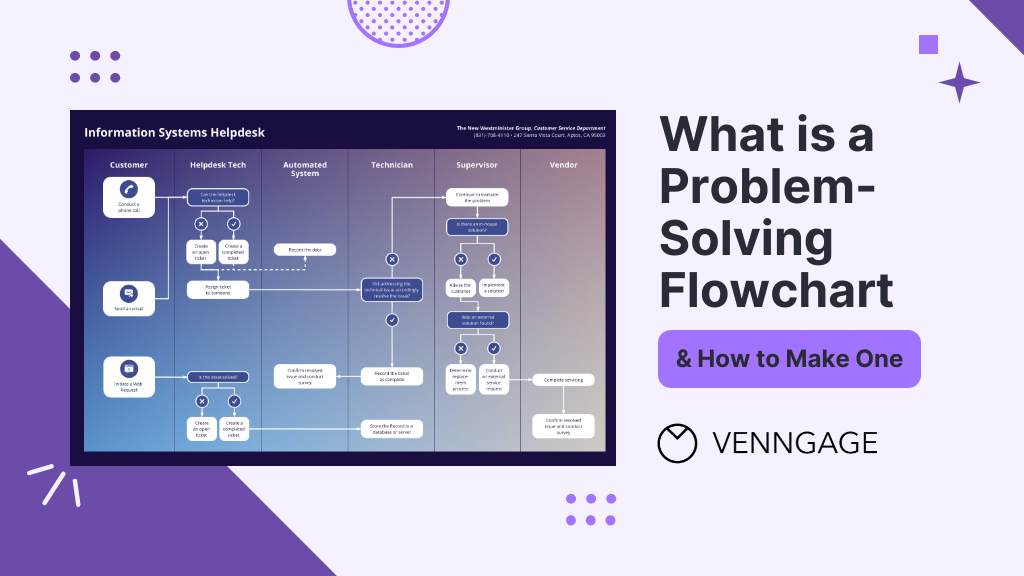
Problem-Solving Flowcharts, contrary to what many believe aren’t just aesthetic wonders — they’re almost like magical blueprints for troubleshooting those pesky problems that many of us face.
Flowcharts take business challenges and turn them into a navigable pathway. In this post, I will guide you on key aspects of problem-solving flowcharts such as what it is, the advantages of problem-solving flowcharts, how to create one and more.
Besides, you’ll also discover how to create problem-solving flowcharts with the help of Venngage’s Flowchart Maker.
And for those of you thinking, “I’m no designer, how can I create one?” worry not! I’ve got you covered. Just hop on Venggage’s Flowchart Templates and you’ll be charting your way to problem-solving glory in no time.
Click to jump ahead:
What are problem-solving flowcharts?
When to use problem-solving flowcharts, what are the advantages of flowcharts in problem-solving, what are the 7 steps of problem-solving flowcharts.
- 5 different types of problem-solving flowcharts
Best practices for designing effective problem-solving flowcharts
How to make a flowchart using venngage , problem-solving flowcharts faqs.
- Final Thoughts
Problem-Solving Flowcharts is a graphical representation used to break down problem or process into smaller, manageable parts, identify the root causes and outline a step-by-step solution.
It helps in visually organizing information and showing the relationships between various parts of the problem.
This type of flowcharts consists of different symbols and arrows, each representing different components or steps in the problem-solving process.
By following the flow of the chart, individuals or teams can methodically approach problem, analyze different aspects of it and come to a well-informed solution.
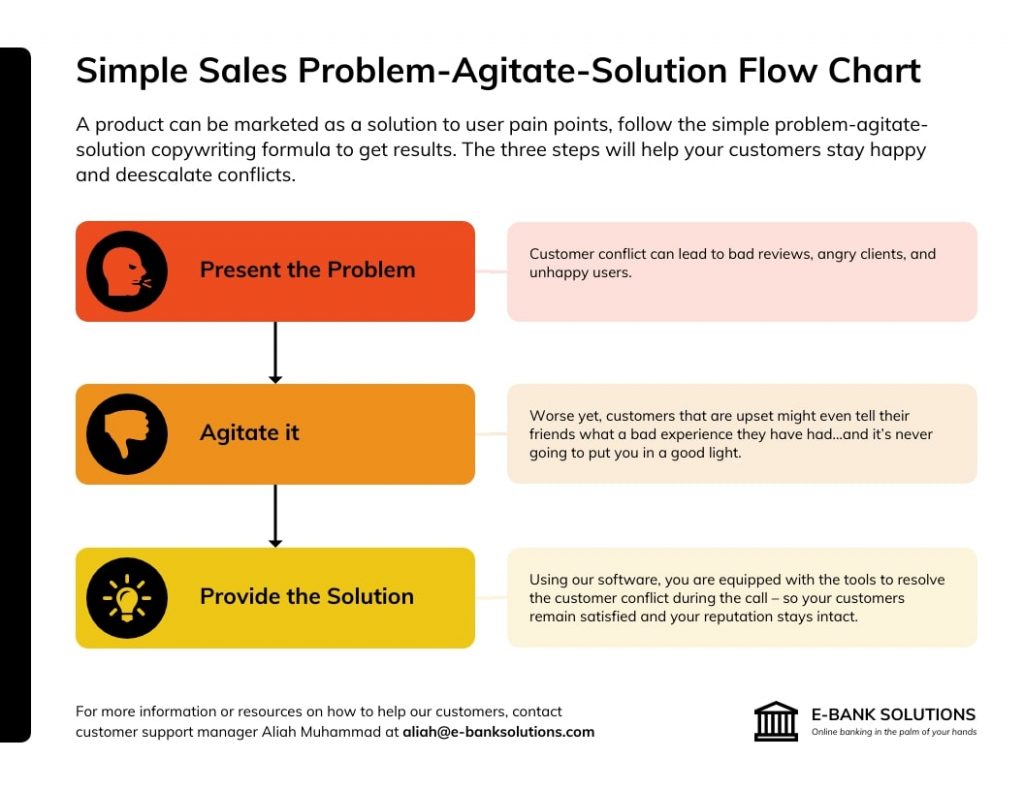
Problem-Solving Flowcharts is a versatile tool that can be used in various scenarios. Here’s when to consider utilizing one:
- Complex Problems: When faced with a multifaceted issue that involves multiple steps or variables, flowcharts can help break down the complexity into digestible parts.
- Team Collaboration: If you’re working with a team and need a common understanding of problem and its potential solutions then a flowchart provides a visual that everyone can refer to.
- Analyzing Processes: In a situation where you need to understand a particular process, whether it’s within a project or a part of regular operations then mapping it out in a flowchart can offer clarity.
- Decision Making: When various paths or decisions might be taken, a flowchart can outline the potential outcomes of each aiding in making an informed choice.
- Training and Onboarding: Flowcharts can be used in training materials to help new employees understand complex processes or procedures which makes the learning curve smoother.
- Identifying Root Causes: If you’re looking to identify the underlying causes of problem then a flowchart can facilitate a systematic approach to reaching the root of the issue.
Related: How to Use Fishbone Diagrams to Solve Complex Problems
Problem-solving flowcharts can offer several benefits to the users who are looking to solve a particular problem. Few advantages of flowcharts in problem solving are:
Visual Clarity
When you’re dealing with multifaceted problems or processes, words alone can make the situation seem even more tangled. Flowcharts distill these complexities into easily understandable visual elements.
By mapping out each phase or component of problem, flowcharts offer a bird’s eye view enabling individuals to grasp the bigger picture and the finer details simultaneously.
Sequential Representation
Flowcharts excel in laying out the sequence of events or actions. By indicating a clear starting point and illustrating each subsequent step, they guide users through a process or solution path methodically.
This linear representation ensures that no step is overlooked and each is executed in the right order.
Collaboration
Problem-solving often requires team effort and flowcharts are instrumental in fostering collaborative environments.
When a team is discussing potential solutions or trying to understand problem’s intricacies, a flowchart serves as a collective reference point.
It aids in synchronizing everyone’s understanding, minimizing miscommunications and promoting constructive discussions.
Read more about: Flowcharts Symbols and Meaning
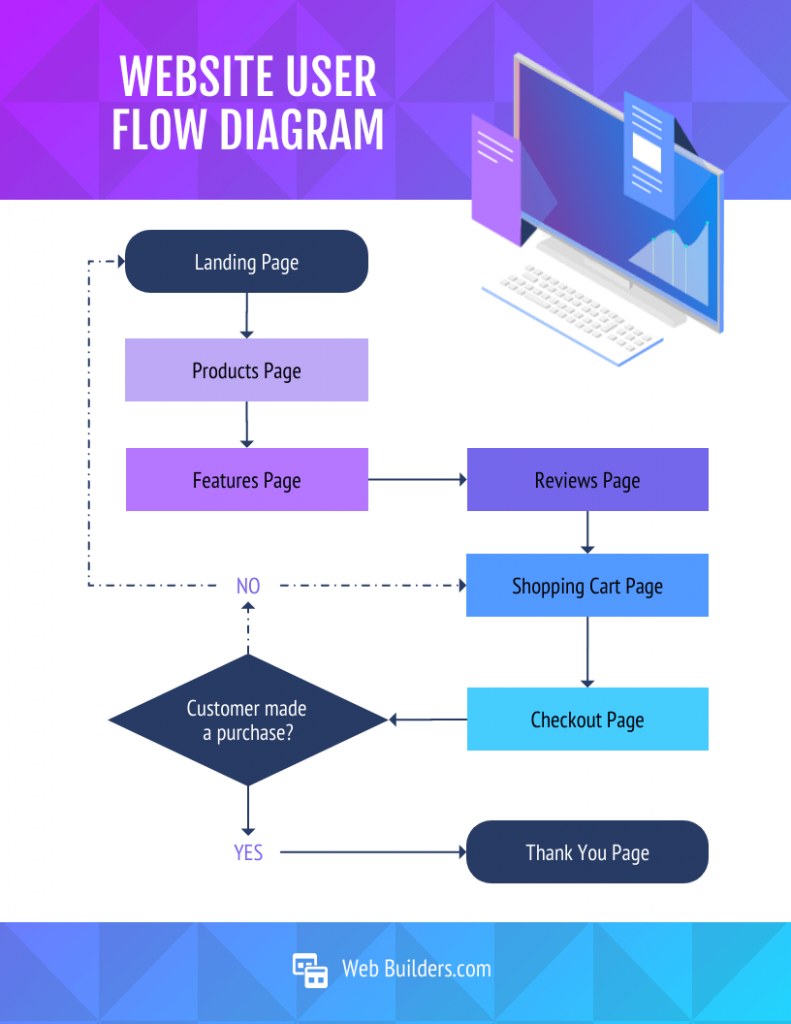
1. Define the Problem
Before anything else, it’s essential to articulate the problem or task you want to solve clearly and accurately. By understanding exactly what needs to be addressed you can ensure that subsequent steps align with the core issue.
2. Identify the Inputs and Outputs
Determine what inputs (such as data, information or resources) will be required to solve the problem and what the desired outputs or outcomes are. Identifying these factors will guide you in structuring the steps needed to reach the end goal and ensure that all necessary resources are at hand.
3. Identify the Main Steps
Break down the problem-solving process into its main steps or subtasks. This involves pinpointing the essential actions or stages necessary to reach the solution. Create a roadmap that helps in understanding how to approach the problem methodically.
4. Use Decision Symbols
In problem-solving, decisions often lead to different paths or outcomes. Using standard symbols to represent these decision points in the flowcharts allows for a clear understanding of these critical junctures. It helps visually present various scenarios and their consequences.
5. Add Descriptions and Details
A well-designed flowcharts is concise but clear in its labeling. Using arrows and short, descriptive phrases to explain what happens at each step or decision point ensures that the flowcharts communicates the process without unnecessary complexity.
6. Revise and Refine
Creating a flowcharts is not always a one-and-done process. It may require revisions to improve its clarity, accuracy or comprehensiveness. Necessary refinement ensures that the flowcharts precisely reflects the problem-solving process and is free from errors or ambiguities.
7. Use Flowchart Tool
While it’s possible to draw a flowcharts manually, using a flowcharts tool like Venngage’s Flowchart Maker and Venngage’s Flowchart Templates can make the process more efficient and flexible. These tools come with pre-designed templates and intuitive interfaces that make it easy to create, modify and share flowcharts.
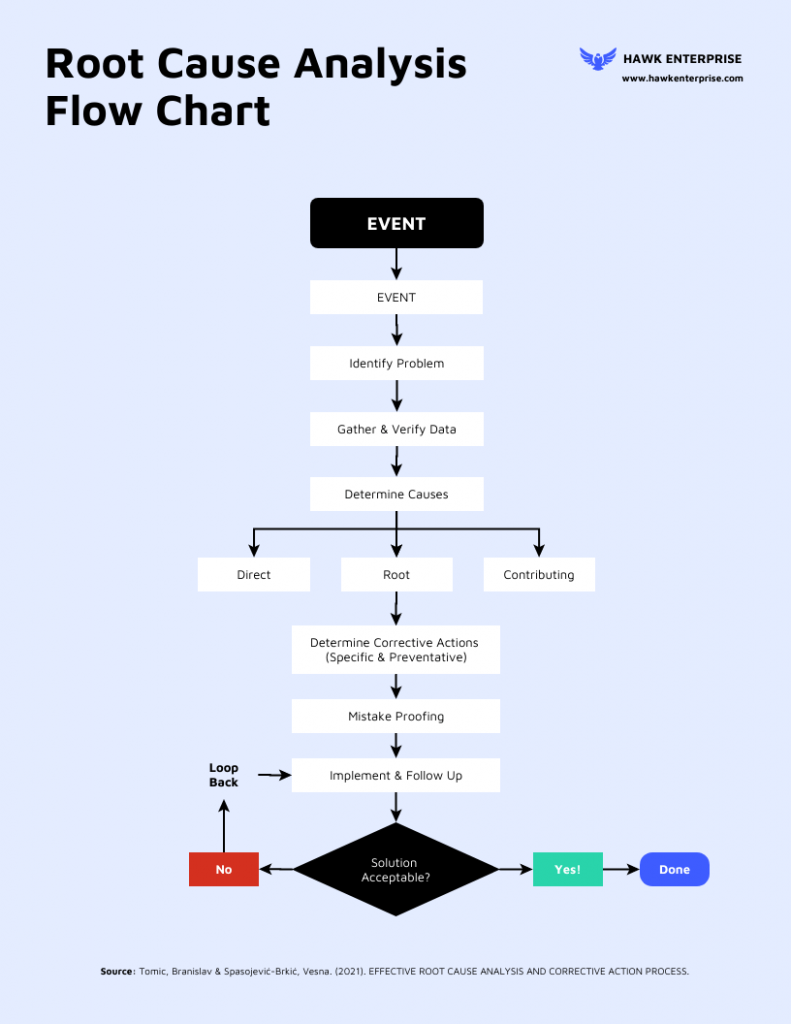
5 different types of problem-solving flowcharts
Let’s have a look at 5 most common types of flowcharts that individuals and organizations often use.
1. Process Flowchart s
A process flowcharts is a visual representation of the sequence of steps and decisions involved in executing a particular process or procedure.
It serves as a blueprint that showcases how different stages or functions are interconnected in a systematic flow and it highlights the direction of the process from its beginning to its end.
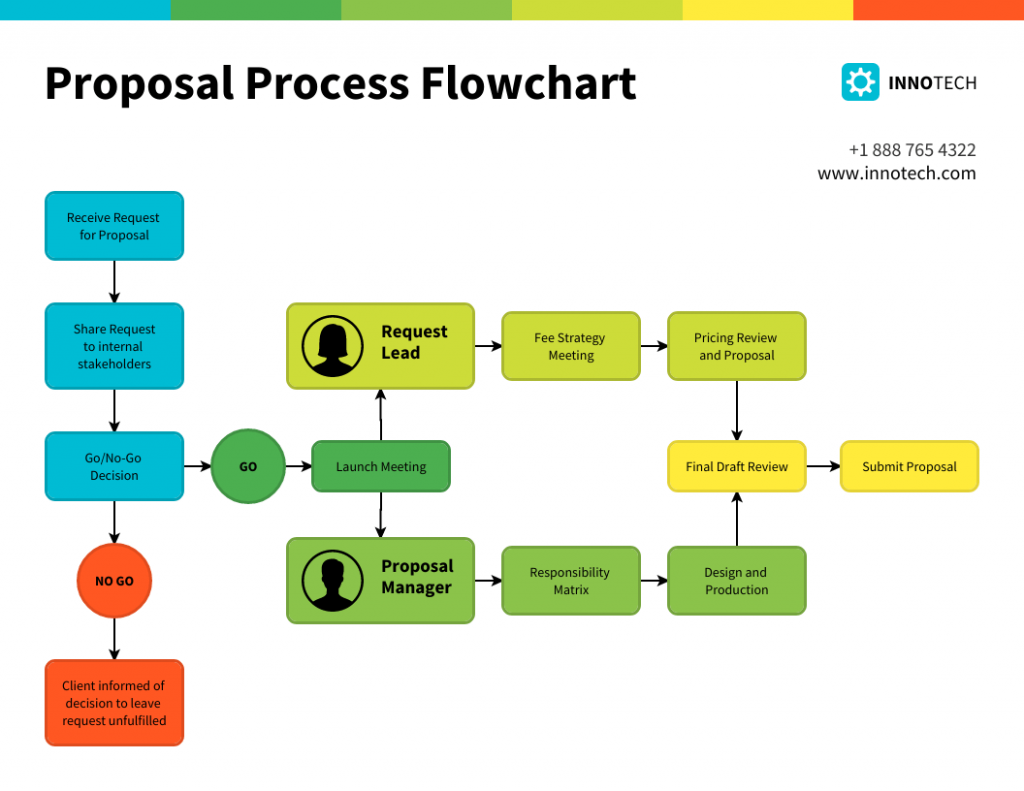
Process flowcharts are instrumental in training and onboarding, sales process , process optimization, documentation, recruitment and in any scenario where clear communication of a process is crucial.
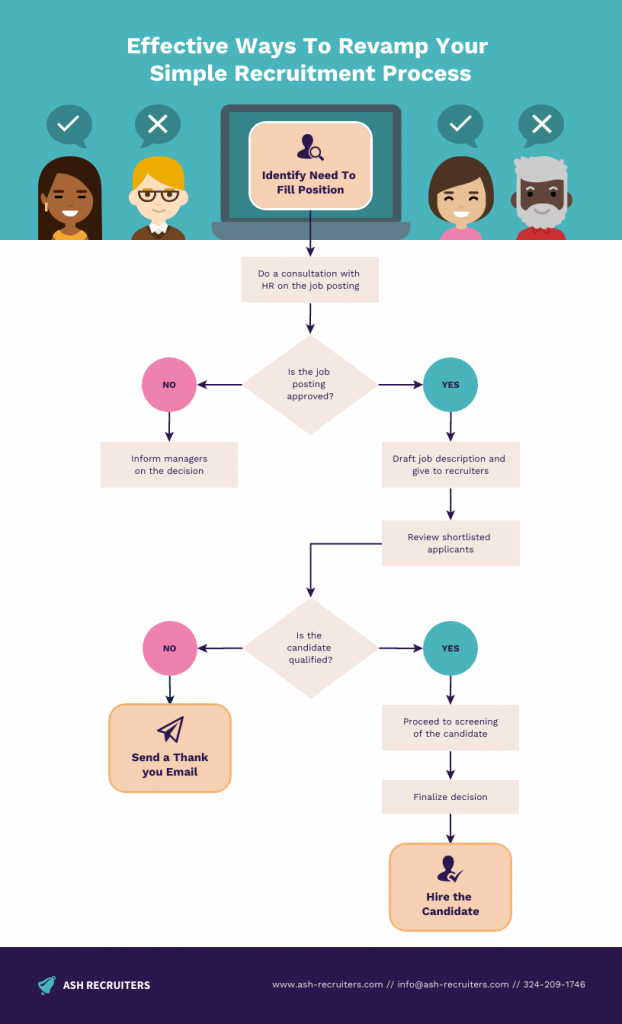
2. Flowcharts Infographic
A flowcharts infographic is a great way to showcase the process or a series of steps using a combination of graphics, icons, symbols and concise text. It aims to communicate complex information in a clear and easy-to-understand manner, making it a popular tool for conveying information, data and instructions in a visually engaging way.
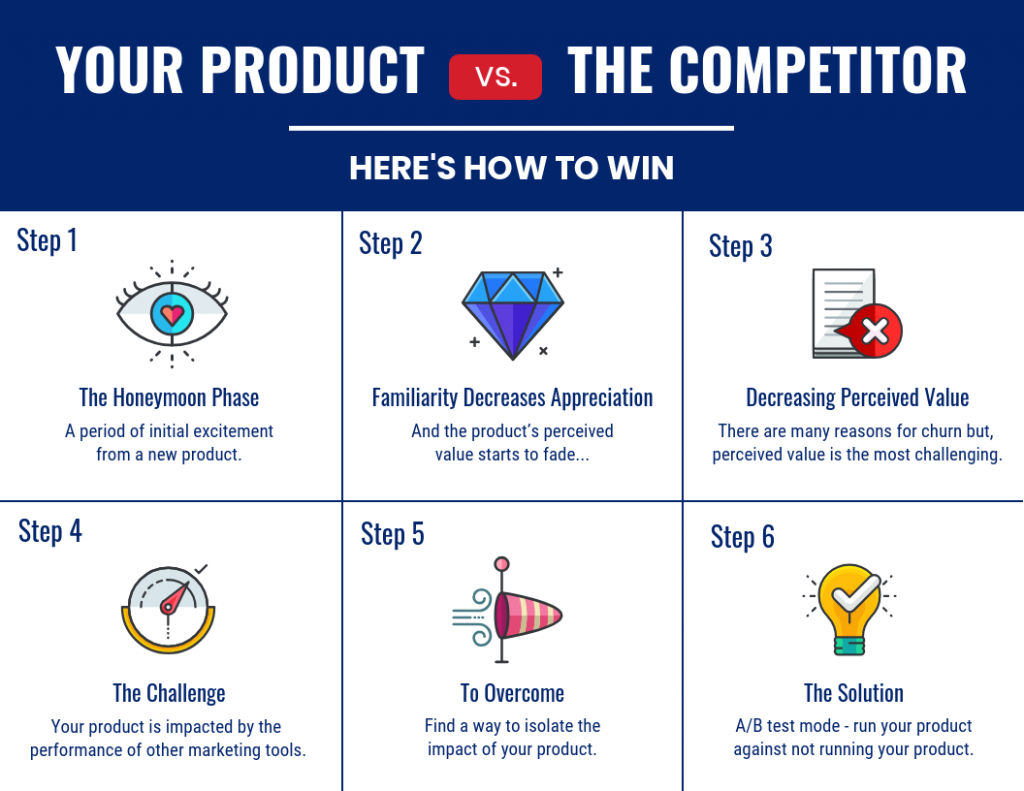
For example, you can use this flowchart to illustrate a health insurance process that visually explains the steps involved from finding a provider to paying for your healthcare provider.
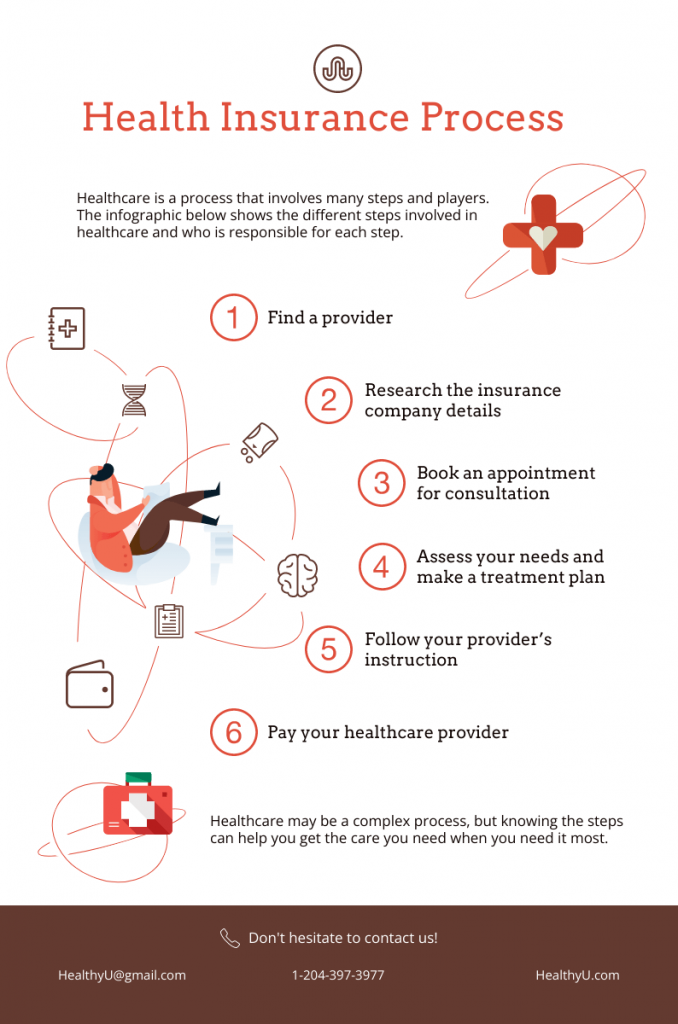
3. Circular Flowcharts
A circular flowcharts is used to illustrate the flow of information, goods, services or money within a closed system or process. It gets its name from its circular shape, which emphasizes the continuous and cyclical nature of the flow.
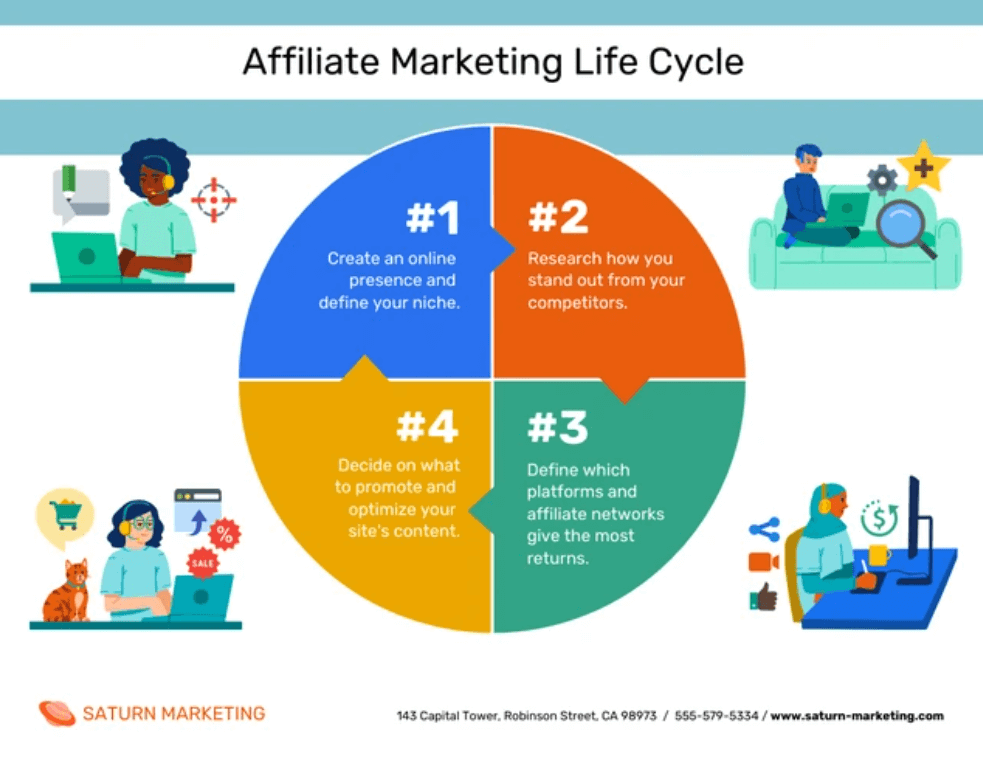
Circular flowcharts are widely used in various fields such as economics, business, engineering and process management to help visualize and understand complex systems.
In a circular flowcharts , elements are represented using various shapes and connected with arrows to indicate the direction of flow. The circular arrangement indicates that the process is ongoing and repeats itself over time.
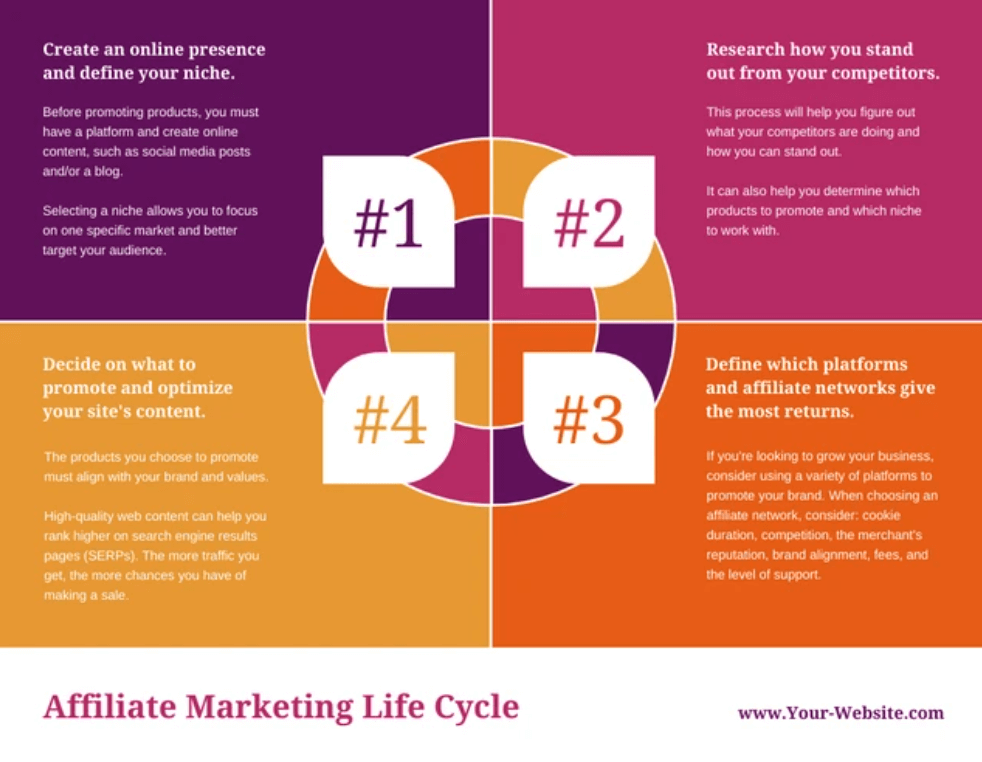
4. Swimlane flowcharts
Swimlane flowcharts , also known as cross-functional flowcharts are a specific type of flowchart that organizes the process flow into lanes or “swimlanes.”
Each lane represents a different participant or functional area involved in the process and the flowchart shows how activities or information move between these participants.
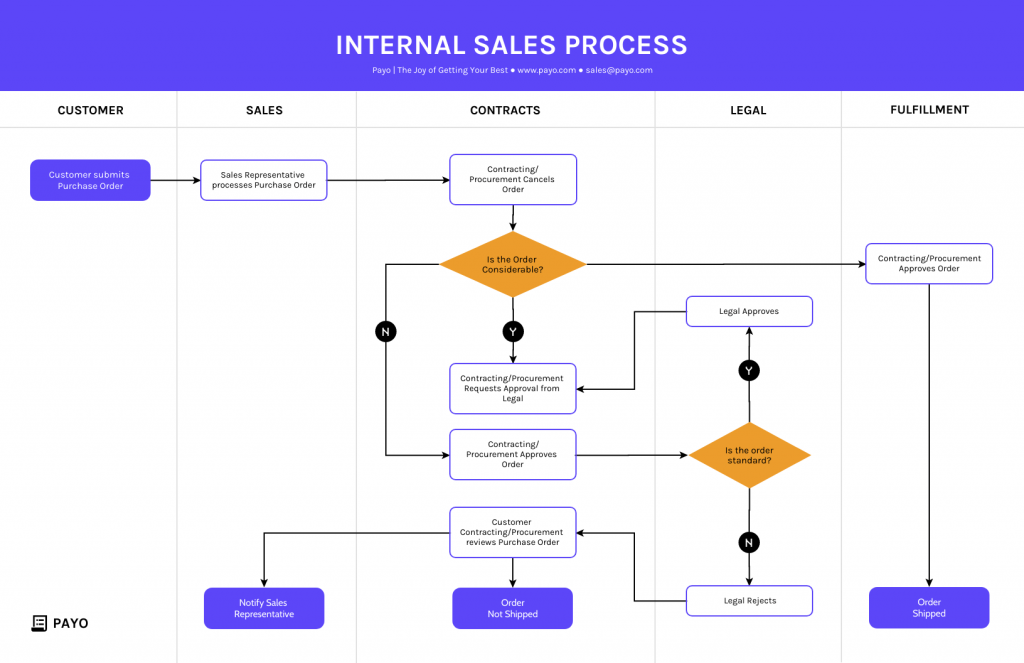
Swimlane flowcharts are particularly useful for illustrating complex processes that involve multiple stakeholders or departments.
In a swimlane flowcharts, the process is divided horizontally into lanes and each lane is labeled with the name of the department, person or role responsible for that part of the process. Vertically, the flowchart displays the sequence of steps or actions taken in the process.
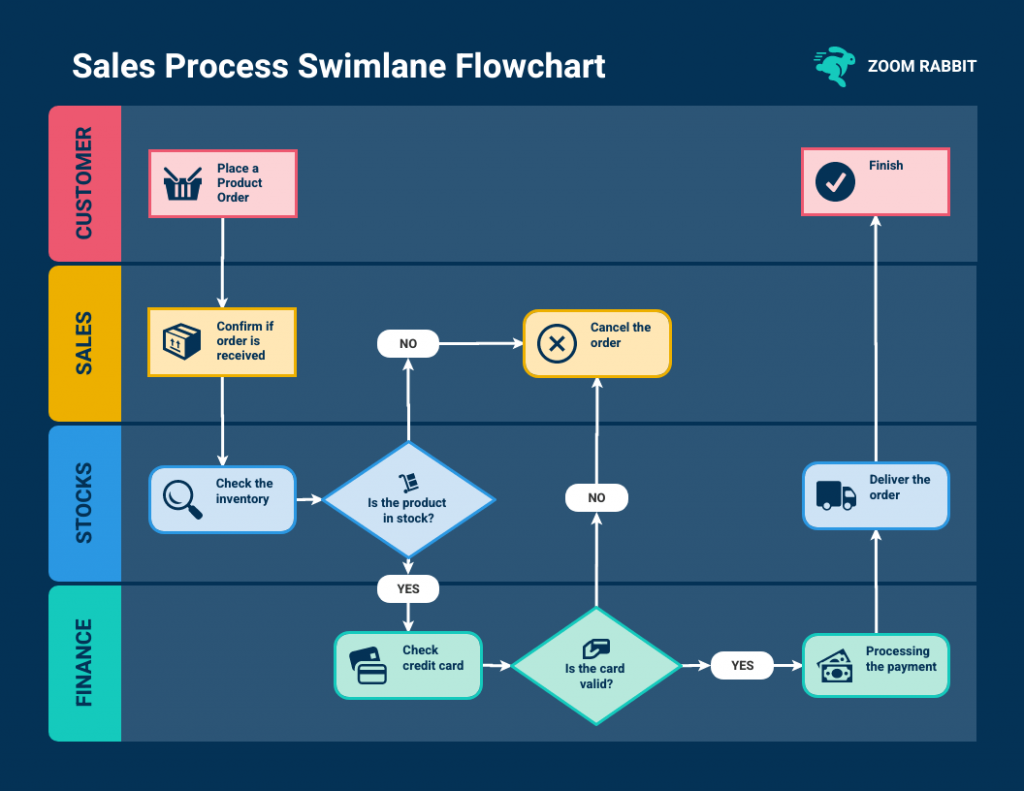
5. Decision Flowchart s
Decision flowcharts, also known as decision trees or flow diagrams are graphical representations that illustrate the process of making decisions or solving problems.
They are widely used in various fields such as computer science, business mapping , engineering and problem-solving scenarios.
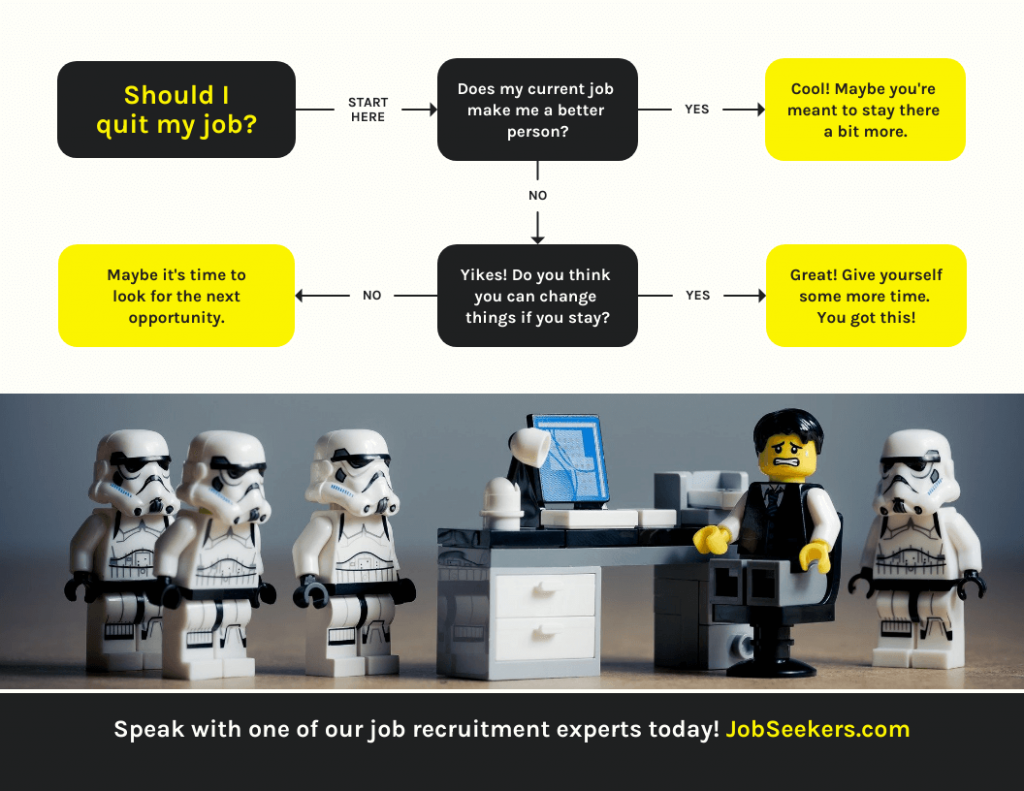
Decision flowcharts help break down complex decision-making processes into simple, sequential steps, making it easier to understand and follow.
A decision tree is a specialized flowchart used to visually represent the process of decision-making.
Businesses and other individuals can employ a decision tree analysis as a tool to aid in evaluating different options and the possible consequences associated with each choice.
Decision trees Infographics can be used to create a more nuanced type of flowchart that is more informative and visually appealing by combining a decision flowchart and the flowchart infographic.
Decision flowcharts are valuable tools for visualizing decision-making processes, analyzing complex problems and communicating them effectively to others.
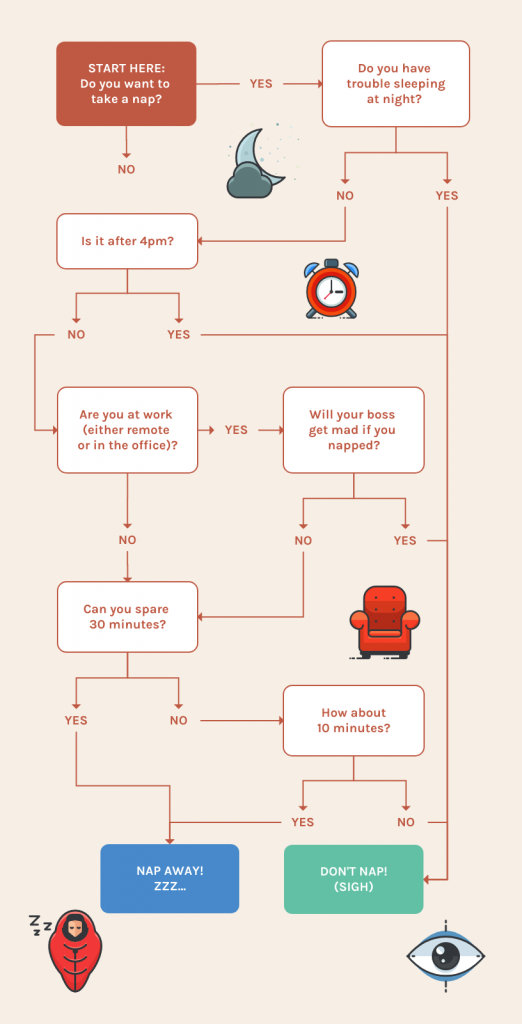
Designing effective problem-solving flowcharts involves careful consideration of various factors to ensure clarity, accuracy and usability. Here are some best practices to create efficient and useful problem-solving flowcharts:
- Understand the problem first & clearly define it
- Keep it simple
- Use standard & recognizable symbols
- Ensure that the flowchart follows a logical and sequential order
- Clearly label each decision point, action and outcome
- Verify the flowchart’s accuracy by testing it
- Clearly state the decision criteria that lead to different branches
- Provide context when the flowchart is part of a larger process or system
- Review and revise the flowchart
Creating problem-solving flowchart on Venngage is incredibly simple. All you have to do is:
- Start by Signing Up and Creating an Account with Venngage
- Choose a flowchart template that best suits your needs from our library.
- Start editing your flowchart by choosing the desired shapes, labels and colors.
- You can also enhance your flowchart by incorporating icons, illustrations or backgrounds all of which are readily available in our library.
- Once done, you will have 2 options to choose from, either sharing it online for free or downloading your flowchart to your desktop by subscribing to the Premium or Business Plan.
Is flowchart the representation of problem solutions?
Flowcharts are not the representation of problem solutions per se; rather, they are a visual representation of processes, decision-making steps and actions taken to arrive at a solution to problem.
What are the 3 basic structures of flowcharts?
3 Basic Structures of Flowcharts are:
- Sequence: Simplify Complexity
- Selection (Decision): Embrace Choices
- Repetition (Loop): Emphasize Iteration
What are the elements of a good flowchart?
A good flowchart should exhibit clarity and simplicity, using consistent symbols and labels to depict a logical sequence of steps. It should be readable, with appropriate white space to avoid clutter while eliminating ambiguity through well-defined decision criteria and paths.
Can flowcharts be used for both simple and complex problem-solving?
Yes, flowcharts can be used for both simple and complex problem-solving scenarios. Flowcharts are versatile visual tools that can effectively represent various processes, decision-making steps and problem-solving approaches regardless of their complexity.
In both cases, flowcharts offer a systematic and visual means of organizing information, identifying potential problems and facilitating collaboration among team members.
Can problem-solving flowcharts be used in any industry or domain?
Problem-solving flowcharts can be used in virtually any industry or domain. The versatility and effectiveness of flowcharts make them applicable to a wide range of fields such as Business and Management, Software Development and IT, Healthcare, Education, Finance, Marketing & Sales and a lot more other industries.
Final thoughts
Problem-solving flowcharts are a valuable and versatile tool that empowers individuals and teams to tackle complex problems with clarity and efficiency.
By visually representing the step-by-step process of identifying, analyzing and resolving issues, flowcharts serve as navigational guides simplifying intricate challenges into digestible parts.
With the aid of modern tools like Venngage’s Flowchart Maker and Venngage’s Flowchart Templates , designing impactful flowcharts becomes accessible to all while revolutionizing the way problems are approached and solved.
- Machine Learning Decision Tree – Solved Problem (ID3 algorithm)
- Poisson Distribution | Probability and Stochastic Process
- Conditional Probability | Joint Probability
- Solved assignment problems in communicaion |online Request
- while Loop in C++
EngineersTutor
Problem solving and algorithms, problem analysis.
Problem analysis can be defined as studying a problem to arrive at a satisfactory solution. To solve a problem successfully, the first step is to understand the problem i.e, problem must be understood clearly. Also the problem must be stated clearly and accurately without any confuse. A well-defined problem and clear description of input and output are important for an effective and efficient solution. Study the outputs to be generated so that input can be specified. Design the steps, which will produce the desired result after supplying the input.
If the problem is very complex, split the problem into multiple sub-problems. Solve each sub-problem and combine the solutions of all the sub-problems to at arrive at overall solution to a large problem. This is called divide and conquer technique.
Problem solving steps
- Understand the problem and plan its logic
- Construction of the List of Variables
- Develop an algorithm
- Refine the algorithm. Refining means making changes
- Program Development
- Testing the Program (solution)
- Validating the Program (solution)
An algorithm is defined as sequence of steps to solve a problem (task) . The steps must be finite, well defined and unambiguous. Writing algorithm requires some thinking. Algorithm can also be defined as a plan to solve a problem and represents its logic. Note that an algorithm is of no use if it does not help us arrive at the desired solution
Algorithm characteristics
- It should have finite number of steps . No one can be expected to execute infinite number of steps.
- The steps must be in order and simple
- Each step should be defined clearly stated e. without un-ambiguity
- Must include all required information
- Should exhibit at least one output
For accomplishing a particular task, different algorithms can be written. Different algorithms can differ in their requirements of time and space. Programmer selects the best suited algorithm for the given task to be solved.
Algorithm for preparing two cups of tea
- Add 1.5 cups of water to the vessel
- Add 2 tea spoons of tea leaves
- Add half cup of milk
- Add some sugar
Statement 5 is an example of an ambiguous (unclear) statement. This statement doesn’t clearly state the amount of sugar to be added.
Algorithm design
- Design an algorithm that is easy to understand code and debug. Debugging is the process finding and fixing errors in a program
- Design an algorithm that makes use of resource such as space (memory) and time efficiently
- ← Merge Sort Algorithm with example
- Flowchart and Algorithm →
Gopal Krishna
Hey Engineers, welcome to the award-winning blog,Engineers Tutor. I'm Gopal Krishna. a professional engineer & blogger from Andhra Pradesh, India. Notes and Video Materials for Engineering in Electronics, Communications and Computer Science subjects are added. "A blog to support Electronics, Electrical communication and computer students".
You May Also Like
Flowchart and algorithm, examples of algorithms and flowcharts in c, solved assignment problems – algorithms and flowcharts, leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
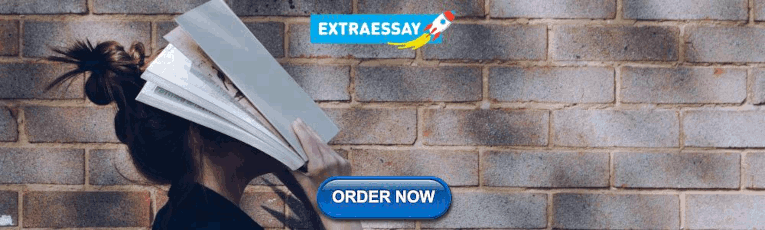
IMAGES
VIDEO
COMMENTS
This tutorial is for beginners.This tutorial helps the user understand how to write pseudocode and flowchart for a simple algorithm of determining the total ...
The algorithm and flowchart are classified into three types of control structures. Sequence; Branching(Selection) ... This is why flowchart is often considered as a blueprint of a design used for solving a specific problem. A flowchart is defined as a symbolic or a graphical representation of an algorithm that uses different standard symbols ...
Explain the role of each. 1.3: Activity 3 - Using pseudo-codes and flowcharts to represent algorithms is shared under a CC BY-SA license and was authored, remixed, and/or curated by LibreTexts. The student will learn how to design an algorithm using either a pseudo code or flowchart. Pseudo code is a mixture of English like statements, some ...
Overview, Objectives, and Key Terms¶. In this lesson, we'll dive right into the basic logic needed to plan one's program, significantly extending the process identified in Lesson 2.We'll examine algorithms for several applications and illustrate solutions using flowcharts and pseudocode.Along the way, we'll see for the first time the three principal structures in programming logic ...
In the New Diagram window, select Flowchart and click Next. You can start from an empty diagram or start from a flowchart template or flowchart example provided. Let's start from a blank diagram. Select Blank and click Next. Enter the name of the flowchart and click OK. Let's start by creating a Start symbol.
ICT1101 Program Logic Formulation Chapter 4: The Sequential Logic Structure f Objective - Solve the problem of sequential logic structure using the seven program development tools 2 f Introduction • Commonly used and the simplest logic structure. • All the problems use this logic structure.
The learners will particularly learn what is an algorithm, the process of developing a solution for a given task, and finally examples of application of the algorithms are given. 1.2: Activity 2 - The characteristics of an algorithm. This section introduces the learners to the characteristics of algorithms.
Algorithms. 1. Introduction. In this tutorial, we'll study how to represent relevant programming structures into a flowchart, thus exploring how to map an algorithm to a flowchart and vice-versa. First, we'll understand why using pseudocode and flowcharts to design an algorithm before actually implementing it with a specific programming ...
Problem Solving with the Sequential Logic Structure Chapter 5. Review • Recall that the: • Algorithm: set of instructions telling the computer how to process a module in a solution. • A flowchart: visual illustration of an algorithm. • In this chapter you will learn how to: • Write an algorithm for each module in a program.
Sequential structure. Is the simplest and most commonly used logic structure. Used by all problems and used in conjunction with one or more other logic structures. Sequential structure used when. Programmers use sequential to tell computer to process a set of instructions in sequence from top to bottom of an algorithm.
An algorithm is made up of three basic building blocks: sequencing, selection, and iteration. Sequencing: An algorithm is a step-by-step process, and the order of those steps are crucial to ensuring the correctness of an algorithm. Here's an algorithm for translating a word into Pig Latin, like from "pig" to "ig-pay": 1.
An algorithm flowchart is a diagrammatic representation that depicts the step-by-step procedure of an algorithm. Each step is symbolized by a specific shape, with arrows guiding the order of operations. These flowcharts simplify complex algorithms into visually understandable forms, enabling programmers, project managers, and even non-technical ...
Examples of flowcharts in programming. 1. Add two numbers entered by the user. Flowchart to add two numbers. 2. Find the largest among three different numbers entered by the user. Flowchart to find the largest among three numbers. 3. Find all the roots of a quadratic equation ax2+bx+c=0.
Rules For Creating Flowchart : A flowchart is a graphical representation of an algorithm.it should follow some rules while creating a flowchart. Rule 1: Flowchart opening statement must be 'start' keyword. Rule 2: Flowchart ending statement must be 'end' keyword. Rule 3: All symbols in the flowchart must be connected with an arrow line.
By using an example, describe how the concept of algorithms can be well presented to a group of students being introduced to it. 1.1: Activity 1 - Introduction to Algorithms and Problem Solving is shared under a CC BY-SA license and was authored, remixed, and/or curated by LibreTexts. In this learning activity section, the learner will be ...
To perform a cause-and-effect analysis, follow these steps. 1. Start with a problem statement. The problem statement is usually placed in a box or another shape at the far right of your page. Draw a horizontal line, called a "spine" or "backbone," along the center of the page pointing to your problem statement. 2.
A flowchart is the graphical or pictorial representation of an algorithm with the help of different symbols, shapes, and arrows to demonstrate a process or a program. With algorithms, we can easily understand a program. The main purpose of using a flowchart is to analyze different methods. Several standard symbols are applied in a flowchart:
A flowchart is a diagram used to illustrate the steps of an algorithm. Flowcharts are made up of symbols, each containing a single step of the algorithm. The shape of the symbol represents the type of process that the symbol contains. Arrows are used to show the flow of execution, meaning that flowcharts can represent all the core concepts of ...
Problem-Solving Flowcharts is a graphical representation used to break down problem or process into smaller, manageable parts, identify the root causes and outline a step-by-step solution. It helps in visually organizing information and showing the relationships between various parts of the problem. This type of flowcharts consists of different ...
An algorithm is defined as sequence of steps to solve a problem (task). The steps must be finite, well defined and unambiguous. Writing algorithm requires some thinking. Algorithm can also be defined as a plan to solve a problem and represents its logic. Note that an algorithm is of no use if it does not help us arrive at the desired solution.
Flowcharts support novice programmers to keep track of where they are and give guidance to what they need to do next, similar to a road-map. High-level flowcharts play a key role in this approach ...
Chapter 2 Beginning Problem-Solving Concepts for the Computer. Constants and Variables; Data Types; ... Chapter 5 Problem Solving with the Sequential Logic Structure. Algorithm Instructions, Flowchart Symbols; ... Algorithms and Flowcharts to Add, Delete, and Access Data in a Linked List; Summary; New Terms; Questions;