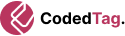
- Variable Function
PHP variable function is a variable that is used to assign a function name with parentheses “()”. However, the PHP interpreter checks if it contains a callback or a function which should exist in the PHP project. If it doesn’t exist, the interpreter will produce an error.
Let’s see how to do that.
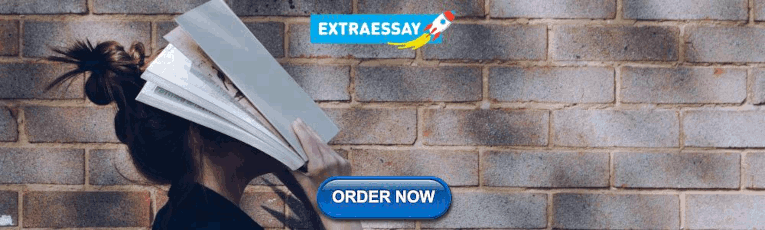
How to Assign a Function to Variable using PHP
According to this concept, PHP allow us to write a string inside a variable which can be called later as a function callback. Here is an example.
In this example, I assigned a string value to the variable “$func”, which contains the function name “codedtag”. Then, I defined the function with the same name as this string.
In the following line, I called the variable as a function “$func()”. However, when the interpreter reads it, it will connect the parentheses “()” with the string inside the variable and consider it as a callback.
Additionally, the PHP interpreter will consider this as a variable function, so if the function is not found during execution, the interpreter will stop executing the code immediately and show you an error message.
Here’s an example to demonstrate this scenario.
In this example, I called an undefined function, which is “calling_func”. That means PHP has to execute this function, “calling_func()”. But it is not defined yet in the document. So, it will show you the following error.
Anyway, let’s focus on another aspect of PHP variable functions, which involves the functions inside a class.
Assign a PHP Function of Class as a Variable
In PHP, you can also use the methods of a class as variables with parentheses to call them once an instance of the class is created.
Here is an example:
In this example, we can see that the “$group” variable stores the name of the method, which can be called by using parentheses.
But, what if the method inside the class contains a static property? Let’s see how it works with the example below.
One way to call the function using the variable name as a function name is by using the scope resolution operator like this: $obj::$group() .
Moreover, there is another method that works on PHP 5.4 and above. It allows us to use a complex callback. For example:
Let’s see how it works with the static method.
In both of these examples, the array acts as a function by implementing the class object, with the function being callable once it finds that the main variable has parentheses.
You learned how to assign a function to a PHP variable, but how to pass arguments within? Let’s focus on this in the following section.
Variable Function and Arguments
You can follow the same approach and pass the arguments into the parentheses of the variable function.
In the above example, the $func variable contains the string name “count”. When invoked with parentheses, PHP looks for the count() function, which requires a countable element as an argument.
However, some PHP predefined callbacks, such as language constructs (e.g., require, empty, include, isset, print, unset, and echo), will not work with variables.
This will show you a fetal error in PHP like the below.
Anyway, Let’s summarize it.
Wrapping Up
During this tutorial, you learned what variable functions are and how to use them with a class, static methods, and more. Here is the explanation in a few points:
- The variable functions in PHP lets you use a variable as a function name. You assign the name of a function to a variable. And then, you call it with parentheses.
- Assign "codedtag" to a variable $func . Define a function codedtag() . Call $func() to execute codedtag() .
- If the assigned function doesn’t exist, PHP stops. It shows a fatal error. This means the function is undefined.
- You can assign methods from a class to variables. Call these methods through the variable. Use the scope resolution operator ( :: ) for static methods.
- Variable functions can take arguments. Include them within the calling parentheses.
- Some PHP constructs like require and empty can’t be variable functions. Trying this results in a fatal error.
Thank you for reading. Happy Coding!
Did you find this article helpful?
Sorry about that. How can we improve it ?
- Facebook -->
- Twitter -->
- Linked In -->
- Install PHP
- Hello World
- PHP Constant
- PHP Comments
PHP Functions
- Parameters and Arguments
- Anonymous Functions
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
Control Structures
- If-else Block
- Break Statement
PHP Operators
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- PHP String Operators
- Array Operators
- Conditional Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Null Coalescing Operator
Data Format and Types
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
String and Patterns
- Remove the Last Char

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP Variable functions
Introduction.
If name of a variable has parentheses (with or without parameters in it) in front of it, PHP parser tries to find a function whose name corresponds to value of the variable and executes it. Such a function is called variable function. This feature is useful in implementing callbacks, function tables etc.
Variable functions can not be built eith language constructs such as include, require, echo etc. One can find a workaround though, using function wrappers.
Variable function example
In following example, value of a variable matches with function of name. The function is thus called by putting parentheses in front of variable
Live Demo
This will produce following result. −
Here is another example of variable function with arguments
In following example, name of function to called is input by user
Variable method example
Concept of variable function can be extended to method in a class
A static method can be also called by variable method technique
This will now throw exception as follows −

Related Articles
- PHP Anonymous functions
- PHP Variable Basics
- PHP Variable Scope
- PHP variable Variables
- PHP User-defined functions
- Uniform variable syntax in PHP 7
- PHP How to cast variable to array?
- Functions that accept variable length key value pair as arguments
- How to declare a global variable in PHP?
- How to assign a PHP variable to JavaScript?
- What do we mean by hash functions in PHP?
- Explain include(),require(),include_once() and require_once() functions in PHP.
- PHP Casting Variable as Object type in foreach Loop
- Convert PHP variable “11:00 AM” to MySQL time format?
- Which is faster? Constants, Variables or Variable Arrays in PHP?
Kickstart Your Career
Get certified by completing the course
Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php variables.
Variables are "containers" for storing information.
Creating (Declaring) PHP Variables
In PHP, a variable starts with the $ sign, followed by the name of the variable:
In the example above, the variable $x will hold the value 5 , and the variable $y will hold the value "John" .
Note: When you assign a text value to a variable, put quotes around the value.
Note: Unlike other programming languages, PHP has no command for declaring a variable. It is created the moment you first assign a value to it.
Think of variables as containers for storing data.
A variable can have a short name (like $x and $y ) or a more descriptive name ( $age , $carname , $total_volume ).
Rules for PHP variables:
- A variable starts with the $ sign, followed by the name of the variable
- A variable name must start with a letter or the underscore character
- A variable name cannot start with a number
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- Variable names are case-sensitive ( $age and $AGE are two different variables)
Remember that PHP variable names are case-sensitive!
Advertisement
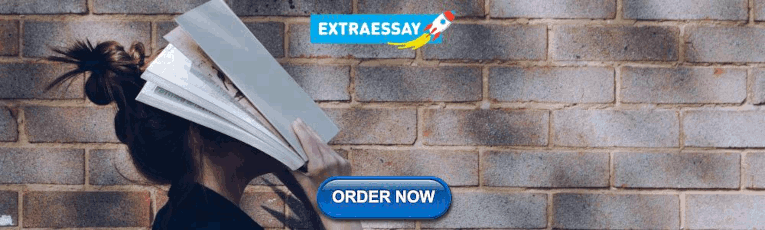
Output Variables
The PHP echo statement is often used to output data to the screen.
The following example will show how to output text and a variable:
The following example will produce the same output as the example above:
The following example will output the sum of two variables:
Note: You will learn more about the echo statement and how to output data to the screen in the PHP Echo/Print chapter .
PHP is a Loosely Typed Language
In the example above, notice that we did not have to tell PHP which data type the variable is.
PHP automatically associates a data type to the variable, depending on its value. Since the data types are not set in a strict sense, you can do things like adding a string to an integer without causing an error.
In PHP 7, type declarations were added. This gives an option to specify the data type expected when declaring a function, and by enabling the strict requirement, it will throw a "Fatal Error" on a type mismatch.
You will learn more about strict and non-strict requirements, and data type declarations in the PHP Functions chapter.
Variable Types
PHP has no command for declaring a variable, and the data type depends on the value of the variable.
PHP supports the following data types:
- Float (floating point numbers - also called double)
Get the Type
To get the data type of a variable, use the var_dump() function.
The var_dump() function returns the data type and the value:
See what var_dump() returns for other data types:
Assign String to a Variable
Assigning a string to a variable is done with the variable name followed by an equal sign and the string:
String variables can be declared either by using double or single quotes, but you should be aware of the differences. Learn more about the differences in the PHP Strings chapter .
Assign Multiple Values
You can assign the same value to multiple variables in one line:
All three variables get the value "Fruit":

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- Sign up Free
Code has been added to clipboard!
PHP Variable Explained: Learn How to Use It
After you learn PHP basics, you will see dollar signs not only in your dreams but also on your screen, as this symbol ($) is used to mark variables. A PHP variable is similar to a little container that is used to store information (value). A value usually consists of letters or numbers, but a variable can also exist without storing any value.
You are not required to declare a variable before adding a value to it. The first time you assign a value to a particular variable name, you create a variable. The value is assigned by using the equal sign, also called the assignment operator (=). Repetition of the process with the same name results in a change of value.
PHP will also automatically understand the type of the variable according to the value you have assigned to it. The context in which a particular PHP variable works is called PHP variable scope.
- 1. PHP Variable: Main Tips
- 2. What a Variable Is
- 3. Way to Output
- 4. The Scope
- 5. PHP Variable: Summary
PHP Variable: Main Tips
- PHP variables start with a $ sign.
- PHP variables are used to store information (value).
- The name of a variable can begin with a letter or an underscore character (_), but never a number. However, it may contain numbers.
- Variables are case-sensitive .
What a Variable Is
A PHP variable may have a name as long or short as you wish. It can consist of one letter (x, y, z, and so on) or a whole bunch of them (tree, household, thelongestwordinthewholewideworld).
The example below shows that the variable $text is a container to the value of Hey coders! and both $x and $y variables hold their respective values of 9 and 1.
Note: In order to assign text to a variable correctly, quotes are used. They are not needed for numerical values.
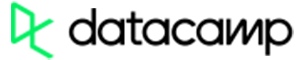
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
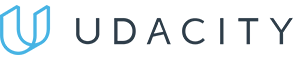
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
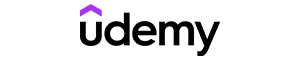
- Easy to navigate
- No technical issues
- Seems to care about its users
- Huge variety of courses
- 30-day refund policy
Way to Output
If you wish to output the information to the screen, it's easy to do by using an echo statement. In the example below, you can see a PHP variable being used in the echo statement. The result will be the output of both statement text and the value that has been assigned to the $website variable.
The user can declare variables anywhere in the PHP script, outside of a function or within one. The exact part in which a certain variable can be used is called PHP variable scope . There are three main scopes:
Local vs. Global
By expressing a PHP variable outside the function, you make it a PHP Global variable. This means that a certain variable can be used anywhere outside the function.
If you express a variable inside the function, it gives it a Local variable scope. As the name suggests, this PHP variable will be only usable locally: inside that particular function.
Note: Different variables with local PHP variable scopes can have identical names and still be executed correctly if they are used within different functions.
If you wish to use a PHP global variable inside a certain function, you should use the keyword global in front of the variable. In the example below you can see how PHP variables $x and $y are used inside a function called learnTest() .
Global variables are stored in a $GLOBALS[index] array. These variables can be accessed and updated without leaving the function. This example illustrates how it works in the learnTest() function:
A local PHP variable scope also indicates that after a certain function is done, the variables inside are deleted. Sometimes, we might prefer to keep them longer.
For a local variable to stay after the function is executed, a static keyword must be used when declaring it. You can see an example of how it is applied on the PHP variable $x below.
The variable will keep both its local scope and the data it held before. It will not be deleted, no matter how many times you will repeat the function.
PHP Variable: Summary
- You can recognize a PHP variable from a first glance, as it always starts with a $ . While it's name can contain numbers, it cannot start with one: the first symbol must be either a letter or an underscore (_).
- Information stored in a certain PHP variable is called value.
- Unlike functions, variables are case-sensitive .
PHP Cheatsheets of Functions
Php and mysql, php and xml, best-rated moocs to learn programming:.
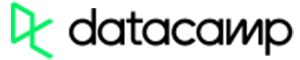
Related Posts
What is php find out its purpose and the main features.
Find out what is PHP and what is PHP used for: learn php basics and get main tips. Read this guide, understand what is PHP and start learning it!
Master PHP Functions: How to Use Inbuilt Functions and Create Your Own
Learn about PHP function: find out how to use PHP functions in your projects and what PHP function argument to use with this PHP function tutorial.
Guide on PHP Form Validation: How, Why and What Functions to Use
Find tips and tricks on PHP form validation: learn the proper way of using php_self function in your work. PHP form validation example included.
Related Code Examples
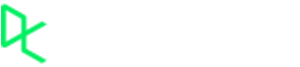
EXCLUSIVE OFFER: GET 25% OFF
- Language Reference
Variables in PHP are represented by a dollar sign followed by the name of the variable. The variable name is case-sensitive.
Variable names follow the same rules as other labels in PHP. A valid variable name starts with a letter or underscore, followed by any number of letters, numbers, or underscores. As a regular expression, it would be expressed thus: ^[a-zA-Z_\x80-\xff][a-zA-Z0-9_\x80-\xff]*$
Note : For our purposes here, a letter is a-z, A-Z, and the bytes from 128 through 255 ( 0x80-0xff ).
Note : $this is a special variable that can't be assigned. Prior to PHP 7.1.0, indirect assignment (e.g. by using variable variables ) was possible.
See also the Userland Naming Guide .
For information on variable related functions, see the Variable Functions Reference .
<?php $var = 'Bob' ; $Var = 'Joe' ; echo " $var , $Var " ; // outputs "Bob, Joe" $ 4site = 'not yet' ; // invalid; starts with a number $_4site = 'not yet' ; // valid; starts with an underscore $täyte = 'mansikka' ; // valid; 'ä' is (Extended) ASCII 228. ?>
By default, variables are always assigned by value. That is to say, when you assign an expression to a variable, the entire value of the original expression is copied into the destination variable. This means, for instance, that after assigning one variable's value to another, changing one of those variables will have no effect on the other. For more information on this kind of assignment, see the chapter on Expressions .
PHP also offers another way to assign values to variables: assign by reference . This means that the new variable simply references (in other words, "becomes an alias for" or "points to") the original variable. Changes to the new variable affect the original, and vice versa.
To assign by reference, simply prepend an ampersand (&) to the beginning of the variable which is being assigned (the source variable). For instance, the following code snippet outputs ' My name is Bob ' twice: <?php $foo = 'Bob' ; // Assign the value 'Bob' to $foo $bar = & $foo ; // Reference $foo via $bar. $bar = "My name is $bar " ; // Alter $bar... echo $bar ; echo $foo ; // $foo is altered too. ?>
One important thing to note is that only named variables may be assigned by reference. <?php $foo = 25 ; $bar = & $foo ; // This is a valid assignment. $bar = &( 24 * 7 ); // Invalid; references an unnamed expression. function test () { return 25 ; } $bar = & test (); // Invalid. ?>
It is not necessary to initialize variables in PHP however it is a very good practice. Uninitialized variables have a default value of their type depending on the context in which they are used - booleans default to false , integers and floats default to zero, strings (e.g. used in echo ) are set as an empty string and arrays become to an empty array.
Example #1 Default values of uninitialized variables
Relying on the default value of an uninitialized variable is problematic in the case of including one file into another which uses the same variable name. E_WARNING (prior to PHP 8.0.0, E_NOTICE ) level error is issued in case of working with uninitialized variables, however not in the case of appending elements to the uninitialized array. isset() language construct can be used to detect if a variable has been already initialized.
User Contributed Notes 2 notes

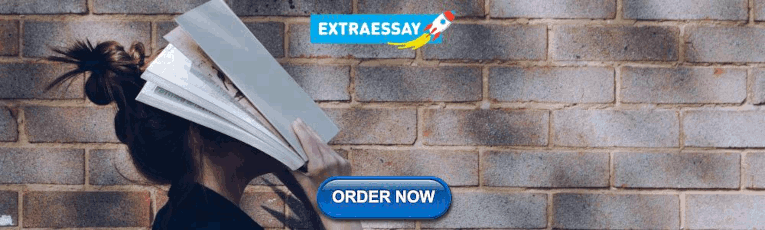
IMAGES
VIDEO
COMMENTS
I too had to work with a codebase that had function calls similar to this. Luckily, I had access to developers that wrote the code. Here is what I learned.
Variable functions. PHP supports the concept of variable functions. This means that if a variable name has parentheses appended to it, PHP will look for a function with the same name as whatever the variable evaluates to, and will attempt to execute it. ... //Assignment using ternary operator.}} abstract class MBR_ATTR {//A class full of ...
php is an interpreted language. what php compiler are you using, hiphop? can you provide some additional context? also, I think you should reconsider checking for !empty on the find method you are calling with an injected value. If you were unit testing this it would be a mess. break out your functionality into reuseable units. -
The arguments are evaluated from left to right, before the function is actually called ( eager evaluation). PHP supports passing arguments by value (the default), passing by reference, and default argument values. Variable-length argument lists and Named Arguments are also supported. Example #1 Passing arrays to functions.
Summary: in this tutorial, you will learn about the PHP variable functions and how to use them to call a function, a method of an object, and a class's static method. Introduction to PHP variable functions. Variable functions allow you to use a variable like a function.
Function Reference Affecting PHP's Behaviour Audio Formats Manipulation ... Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference.
Assigning functions to variables in PHP is a powerful technique for creating dynamic and flexible code. It allows developers to pass functions as arguments, store them in arrays or objects, and create more reusable and maintainable code. Overall, the ability to assign functions to variables is a useful tool to have in any PHP developer's toolbox.
This is a short guide on how to assign an anonymous function to a variable in PHP and then call it. Note that these kind of functions are sometimes referred to as lambda functions. Let's start off with a simple example with no parameters: //Create an anonymous function and assign it to a PHP variable.
PHP User Defined Functions. Besides the built-in PHP functions, it is possible to create your own functions. A function is a block of statements that can be used repeatedly in a program. A function will not execute automatically when a page loads. A function will be executed by a call to the function.
In PHP, the reference assignment operator (=&) is used to create a new variable as an alias to an existing spot in memory. In other words, the reference assignment operator (=&) creates two variable names which point to the same value. So, changes to one variable will affect the other, without having to copy the existing data.
In PHP global variables must be declared global inside a function if they are going to be used in that function. The global ... I had to add the following code to my safeinclude function (before variables are used or file is included) ... When you assign some variable value by reference you in fact write address of source variable to recepient ...
Introduction. If name of a variable has parentheses (with or without parameters in it) in front of it, PHP parser tries to find a function whose name corresponds to value of the variable and executes it. Such a function is called variable function. This feature is useful in implementing callbacks, function tables etc.
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
Closures can also be used as the values of variables; PHP automatically converts such expressions into instances of the Closure internal class. Assigning a closure to a variable uses the same syntax as any other assignment, including the trailing semicolon: ... Since a property can be also an anonymous function as of PHP 5.3.0, an oddity arises ...
Rules for PHP variables: A variable starts with the $ sign, followed by the name of the variable. A variable name must start with a letter or the underscore character. A variable name cannot start with a number. A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
Variable variables are a powerful feature of PHP that allows you to use the value of a variable as the name of another variable. Learn how to use them, what are the benefits and pitfalls, and how they differ from normal variables. This manual page also provides examples and links to related topics.
The first time you assign a value to a particular variable name, you create a variable. The value is assigned by using the equal sign, also called the assignment operator (=). ... By expressing a PHP variable outside the function, you make it a PHP Global variable. This means that a certain variable can be used anywhere outside the function ...
Basics. ¶. Variables in PHP are represented by a dollar sign followed by the name of the variable. The variable name is case-sensitive. Variable names follow the same rules as other labels in PHP. A valid variable name starts with a letter or underscore, followed by any number of letters, numbers, or underscores.
That's simply not a real question and there is no point in trying to answer it directly, especially if you aren't familiar with PHP syntax.. It's the OP's premises have to be questioned, not the code he's posted.. This code will plainly output the result of getClientAccount method (unless it's 0 or empty array).. So, the OP have to check what "was in the code actually" once more or verify the ...