previous episode
Octave programming, making choices, next episode.
Overview Teaching: 30 min Exercises: 0 min Questions How can programs do different things for different data values? Objectives Construct a conditional statement using if, elseif, and else Test for equality within a conditional statement Combine conditional tests using AND and OR Build a nested loop
Our previous lessons have shown us how to manipulate data and repeat things. However, the programs we have written so far always do the same things, regardless of what data they’re given. We want programs to make choices based on the values they are manipulating.
The tool that Octave gives us for doing this is called a conditional statement , and it looks like this:
The second line of this code uses the keyword if to tell Octave that we want to make a choice. If the test that follows is true, the body of the if (i.e., the lines between if and else ) are executed. If the test is false, the body of the else (i.e., the lines between else and end ) are executed instead. Only one or the other is ever executed.
Conditional statements don’t have to have an else block. If there isn’t one, Octave simply doesn’t do anything if the test is false:
We can also chain several tests together using elseif . This makes it simple to write a script that gives the sign of a number:
One important thing to notice in the code above is that we use a double equals sign == to test for equality rather than a single equals sign. This is because the latter is used to mean assignment. In our test, we want to check for the equality of num and 0 , not assign 0 to num . This convention was inherited from C, and it does take a bit of getting used to…
We can also combine tests, using && (and) and || (or). && is true if both tests are true:
|| is true if either test is true:
In this case, “either” means “either or both”, not “either one or the other but not both”.
True and False Statements 1 and 0 aren’t the only values in Octave that are true or false. In fact, any value can be used in an if or elseif . After reading and running the code below, explain what the rule is for which values that are considered true and which are considered false. a. if '' disp('empty string is true') end b. if 'foo' disp('non empty string is true') end c. if [] disp ('empty array is true') end d. if [22.5, 1.0] disp ('non empty array is true') end e. if [0, 0] disp ('array of zeros is true') end f. if true disp('true is true') end
Close Enough Write a script called near that performs a test on two variables, and displays 1 when the first variable is within 10% of the other and 0 otherwise. Compare your implementation with your partner’s: do you get the same answer for all possible pairs of numbers?
Another thing to realize is that if statements can be also combined with loops. For example, if we want to sum the positive numbers in a list, we can write this:
With a little extra effort, we can calculate the positive and negative sums in a loop:
We can even put one loop inside another:
Nesting Will changing the order of nesting in the above loop change the output? Why? Write down the output you might expect from changing the order of the loops, then rewrite the code to test your hypothesis. Octave (and most other languges in the C family) provides in-place operators that work like this: x = 1; x += 1; x *= 3; Rewrite the code that sums the positive and negative values in an array using these in-place operators. Do you think that the result is more or less readable than the original?
Currently, our script analyze.m reads in data, analyzes it, and saves plots of the results. If we would rather display the plots interactively, we would have to remove (or comment out ) the following code:
And, we’d also have to change this line of code, from:
This is not a lot of code to change every time, but it’s still work that’s easily avoided using conditionals. Here’s our script re-written to use conditionals to switch between saving plots as images and plotting them interactively:
Key Points Use if and else to make choices based on values in your program.
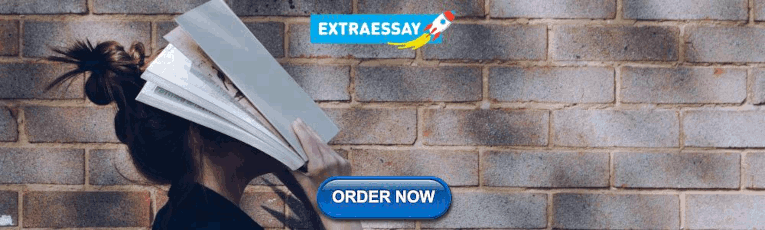
10.1 The if Statement
The if statement is Octave’s decision-making statement. There are three basic forms of an if statement. In its simplest form, it looks like this:
condition is an expression that controls what the rest of the statement will do. The then-body is executed only if condition is true.
The condition in an if statement is considered true if its value is nonzero, and false if its value is zero. If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if it is non-empty and all of the elements are nonzero. The conceptually equivalent code when condition is a matrix is shown below.
The second form of an if statement looks like this:
If condition is true, then-body is executed; otherwise, else-body is executed.
Here is an example:
In this example, if the expression rem (x, 2) == 0 is true (that is, the value of x is divisible by 2), then the first printf statement is evaluated, otherwise the second printf statement is evaluated.
The third and most general form of the if statement allows multiple decisions to be combined in a single statement. It looks like this:
Any number of elseif clauses may appear. Each condition is tested in turn, and if one is found to be true, its corresponding body is executed. If none of the conditions are true and the else clause is present, its body is executed. Only one else clause may appear, and it must be the last part of the statement.
In the following example, if the first condition is true (that is, the value of x is divisible by 2), then the first printf statement is executed. If it is false, then the second condition is tested, and if it is true (that is, the value of x is divisible by 3), then the second printf statement is executed. Otherwise, the third printf statement is performed.
Note that the elseif keyword must not be spelled else if , as is allowed in Fortran. If it is, the space between the else and if will tell Octave to treat this as a new if statement within another if statement’s else clause. For example, if you write
Octave will expect additional input to complete the first if statement. If you are using Octave interactively, it will continue to prompt you for additional input. If Octave is reading this input from a file, it may complain about missing or mismatched end statements, or, if you have not used the more specific end statements ( endif , endfor , etc.), it may simply produce incorrect results, without producing any warning messages.
It is much easier to see the error if we rewrite the statements above like this,
using the indentation to show how Octave groups the statements. See Functions and Scripts .
© 1996–2018 John W. Eaton Permission is granted to make and distribute verbatim copies of this manual provided the copyright notice and this permission notice are preserved on all copies. Permission is granted to copy and distribute modified versions of this manual under the conditions for verbatim copying, provided that the entire resulting derived work is distributed under the terms of a permission notice identical to this one.Permission is granted to copy and distribute translations of this manual into another language, under the above conditions for modified versions. https://octave.org/doc/interpreter/The-if-Statement.html
Technology eXplorer
- Octave:2> Conditional Execution
- April 19, 2020
Purnendu Kumar
To control the flow operations in an algorithm, one must be able to verify some conditions and act accordingly. Other-times it might be required to perform same task repeatedly until certain conditions are met. Conditional execution are the backbone of any algorithm. In this article we will look through common conditional and looping expressions. We encourage readers planning to learn Octave/Matlab to try the exercises marked as “ Ex “ along with the examples used in this article.
if Statement
“ if ” statement is the most common conditional execution block in any algorithm. It executes the part of block only the boolean expression provided after “ if ” is true. Boolean expression after “ if “is known as expression. To end the loop, “ end ” statement is used.
“ if ” statement can also be used with an “ else ” statement and thus some action can be performed in both cases either the condition is true or false.
It is often the case algorithm night require to verify more than one condition and then do accordingly. in such case “ elseif ” condition can be used as many time required.
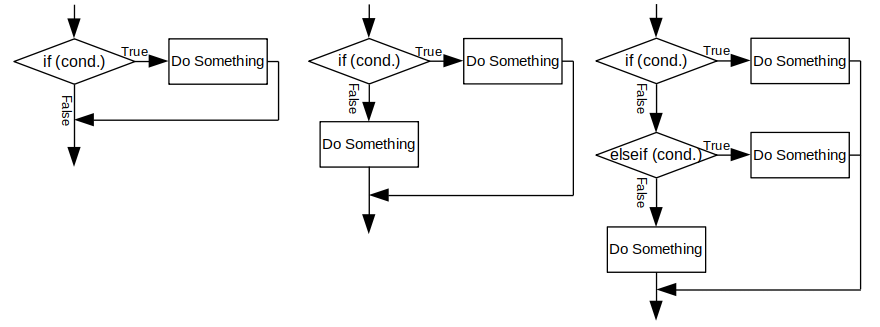
switch Statement
A “ switch ” is a series of checks on a variable. outcome of this statement is comparable to “ if .. elseif .. else “, but execution is not. In “ switch case ” execution happens as per variable, multiple condition checks are not done.
while Statement
“ while ” is a looping statement with boolean condition. “ while ” loop keeps on executing the same block of code until the condition is true. while loop is preferable when the number of iteration is not known.
Loop can be terminated in mid also using “ break ” statement.

for Statement
When number of iteration is known and steps are equidistant, for loop is preferable for algorithm to work efficiently.
Colon operator is preferred choice in for loop, but not the only way to use a for loop.
After learning the conditional execution along with scripting, try to create a solution for any known problem and verify your script. keep learning, keep growing. Let us know your query and questions in comment section.

- GNU Octave – Scientific Programming Language
- Octave:1> Getting Started
- Octave:1> Variables and Constants
- Octave:1> Expressions and operators
- Octave:1> Built-In Functions, Commands, and Package
- Octave:2> Vectors and Matrices Basics
- Octave:2> Scripts and Algorithm
- Octave:2> Functions
- Octave:3> File Operations
- Octave:3> Data Visualization
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Facebook (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to email a link to a friend (Opens in new window)
- Click to print (Opens in new window)
- Click to share on Tumblr (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Pocket (Opens in new window)
- Click to share on Telegram (Opens in new window)
Tags: GNU Octave Matlab Conditional Execution Loop if while switch
Purnendu is currently working as Senior Project Engineer at QuNu Labs, Indias only Quantum security company. He has submitted his thesis for Masters (MS by research 2014-17) in electrical engineering at IIT Madras for doing his research on “constant fraction discriminator” and “amplitude and rise-time compensated discriminator” for precise time stamping of Resistive Plate Chamber detector signals. In collaboration with India Based Neutrino observatory project, he has participated in design and upgrade of FPGA-based data acquisition system, test-jig development, and discrete front-end design. After completion of his bachelors in Electrical Engineering (Power and Electronics), he was awarded Junior Research Fellowship at Department of Physics and Astro-physics, University of Delhi under same (INO) project. His current interest is in high-speed circuit design, embedded systems, IoT, FPGA implementation and optimization of complex algorithms, experimental high-energy physics, and quantum mechanics.
You may also like...

DIY two-channel 120W DC power supply

Checklist for error-free optimized PCB layout

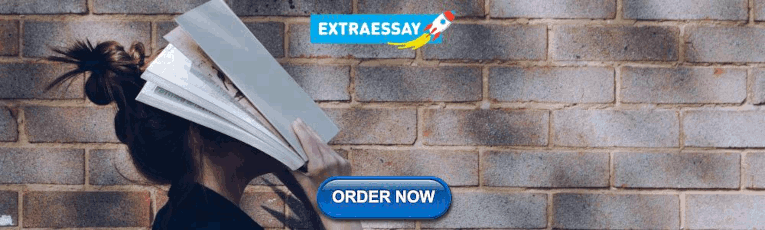
Introduction to diode
- Next Octave:3> File Operations
- Previous Octave:2> Scripts and Algorithm
- Git- The ultimate version control (1)
- Image Profiler (1)
- DIY Electronics (1)
- GNU Octave (11)
- PCB Design (10)

- February 2020
- October 2019
- February 2018
Next: Functions and Scripts , Previous: Evaluation [ Contents ][ Index ]
10 Statements ¶
Statements may be a simple constant expression or a complicated list of nested loops and conditional statements.
Control statements such as if , while , and so on control the flow of execution in Octave programs. All the control statements start with special keywords such as if and while , to distinguish them from simple expressions. Many control statements contain other statements; for example, the if statement contains another statement which may or may not be executed.
Each control statement has a corresponding end statement that marks the end of the control statement. For example, the keyword endif marks the end of an if statement, and endwhile marks the end of a while statement. You can use the keyword end anywhere a more specific end keyword is expected, but using the more specific keywords is preferred because if you use them, Octave is able to provide better diagnostics for mismatched or missing end tokens.
The list of statements contained between keywords like if or while and the corresponding end statement is called the body of a control statement.
- The if Statement
- The switch Statement
- The while Statement
- The do-until Statement
- The for Statement
- The break Statement
- The continue Statement
- The unwind_protect Statement
- The try Statement
- Continuation Lines
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to do multivariable assignment in octave?
I want to do multivariable assignment. I can do [a,b] = min([1 2 3]) but I can't do [a,b] = [1,2] . Why? Is there any workaround?
- Examples in this PAQ stackoverflow.com/questions/9908398/… – Cobusve Commented Aug 8, 2015 at 14:22
- Please consider removing the Matlab tag since the accepted answer won't work there. My answer using deal would do the job in Octave and Matlab. – Matt Commented Aug 8, 2015 at 14:30
3 Answers 3
The [1,2] on the right hand side of the assignment is interpreted as array with the two elements 1 and 2 .
If you want to do the multi-variable-assignment in one line, you can use deal in Matlab. This should work in Octave as well according to the documentation here .
The advantage of using deal is that it works in Matlab as well, where the solution with [a b] = {1 2}{:} won't.
Octave basics: How to assign variables from a vector
To adapt Cobusve's answer to Matlab, two lines are required:

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged matlab syntax octave or ask your own question .
- The Overflow Blog
- Masked self-attention: How LLMs learn relationships between tokens
- Deedy Das: from coding at Meta, to search at Google, to investing with Anthropic
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Feedback Requested: How do you use the tagged questions page?
Hot Network Questions
- Does history possess the epistemological tools to establish the occurrence of an anomaly in the past that defies current scientific models?
- What happened with the 31.5 ft giant in Jubbulpore district (now Jabalpur), India, August 8, 1934?
- Choosing MCU for motor control
- Is there an error in the dissipation calculation of a mosfet?
- Shock absorber rusted from being outside
- How can fideism's pursuit of truth through faith be considered a sound epistemology when mutually exclusive religious beliefs are accepted on faith?
- Do pilots have to produce their pilot license to police when asked?
- Java class subset of C++ std::list with efficient std::list::sort()
- What happens if parents refuse to name their newborn child?
- How can I make a 2D FTL-lane map on a galaxy-wide scale?
- How do you measure exactly 31 minutes by burning the ropes?
- If Voyager is still an active NASA spacecraft, does it have a flight director? Is that a part time job?
- World's smallest Sudoku!
- Where is this NPC's voice coming from?
- How can I draw the intersection of a plane with a dome tent?
- Taking out the film from the roll can it still work?
- Does copying files from one drive to another also copy previously deleted data from the drive one?
- Is a 1500w inverter suitable for a 10a portable band saw?
- How to do automated content publishing post deployment in XMCloud?
- Looking for the source of a drasha
- Can I use named pipes to achieve temporal uncoupling?
- All combinations of ascending/descending digits
- Book where the humans discover tachyon technology and use this against a race of liquid metal beings
- God the Father punished the Son as sin-bearer: how does that prove God’s righteousness?
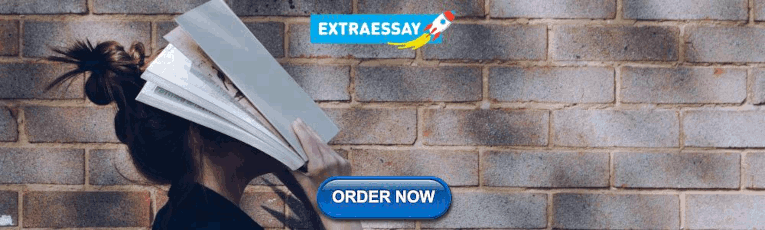
IMAGES
VIDEO
COMMENTS
However, using conditionals with matrices only seems to let you assign a scalar to some elements, not a full matrix. I tried using conditionals to determine which elements are iterated, but using conditionals changes the dimensions of the matrix to a one dimensional array. Zn = Zn.^2+Z; Iter(Iter==0 & (abs(Zn)>10))=m; Here's my full program.
condition is an expression that controls what the rest of the statement will do. The then-body is executed only if condition is true.. The condition in an if statement is considered true if its value is nonzero, and false if its value is zero. If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if it is non-empty and all of the ...
The functions any and all are useful for determining whether any or all of the elements of a matrix satisfy some condition. The find function is also useful in determining which elements of a matrix meet a specified condition. : tf = any (x) ¶. : tf = any (x, dim) ¶. For a vector argument, return true (logical 1) if any element of the vector ...
The tool that Octave gives us for doing this is called a conditional statement, and it looks like this: ... Conditional statements don't have to have an else block. If there isn't one, Octave simply doesn't do anything if the test is false: ... This is because the latter is used to mean assignment.
If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if all of the elements are non-zero. Octave's while ... The assignment expression in the for statement works a bit differently than Octave's normal assignment statement. Instead of assigning the complete result of the ...
Matlab/Octave tutorial for conditional statements for absolute beginners.Please feel free to make any comments, and subscribe and thumbs up if you like the v...
<CONDITION> is just an <EXPRESSION> inside of parentheses. (The expression needs to have a boolean value, but that's not a syntactic restriction; any expression is syntactically valid.) Creating grammars for expressions is tedious and requires a detailed knowledge of the expression syntax (obviously), but they are generally pretty similar, so you should be able to find any number of examples ...
Octave Replace elements in a vector under certain circumstances. 0. Vectorizing logic to check if index matches. 0. Using vectorization to reduce for loops, how to use conditional if? 1. How to optimize conditional statement in for loop over image? 1. Octave how to compare matrix elements efficiently.
In this tutorial if elseif and else conditional statements are explained in GNU Octave run in a Windows 11 Pro environment.
Note that in conditional contexts (like the test clause of if and while statements) Octave treats the test as if you had typed all (all (condition)). The functions isinf, finite, and isnan return 1 if their arguments are infinite, finite, or not a number, respectively, and return 0 otherwise. For matrix values, they all work on an element by ...
This page titled 7.5: Working with Conditions is shared under a CC BY-NC-SA 4.0 license and was authored, remixed, and/or curated by Allen B. Downey (Green Tea Press) via source content that was edited to the style and standards of the LibreTexts platform. Shows how to use conditions to test for valid variable values.
the value of the variable b is incremented even if the variable a is zero.. This behavior is necessary for the boolean operators to work as described for matrix-valued operands. Built-in Function: z = and (x, y) Built-in Function: z = and (x1, x2, …) Return the logical AND of x and y.. This function is equivalent to the operator syntax x & y.If more than two arguments are given, the logical ...
Expressions are the basic building block of statements in Octave. An expression evaluates to a value, which you can print, test, store in a variable, pass to a function, or assign a new value to a variable with an assignment operator. An expression can serve as a statement on its own.
If you have mathematical questions given to you with multiple conditions, use this video in order to know how to use the conditions in octave programming.
Expressions are the basic building block of statements in Octave. It can be created using values, variables that have already been created, operators, functions, and parentheses. An expression evaluates to value and can serve as a statement on its own. Generally, statements contain one or more expressions that specify data to be operated on.
The if statement is Octave's decision-making statement. There are three basic forms of an if statement. ... If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if it is non-empty and all of the elements are nonzero. The conceptually equivalent code when condition is a matrix is ...
An assignment is an expression that stores a new value into a variable. For example, the following expression assigns the value 1 to the variable z: z = 1. After this expression is executed, the variable z has the value 1. Whatever old value z had before the assignment is forgotten. The ' = ' sign is called an assignment operator.
This entry is part 8 of 11 in the series GNU Octave. To control the flow operations in an algorithm, one must be able to verify some conditions and act accordingly. Other-times it might be required to perform same task repeatedly until certain conditions are met. Conditional execution are the backbone of any algorithm.
10 Statements ¶. Statements may be a simple constant expression or a complicated list of nested loops and conditional statements. Control statements such as if, while, and so on control the flow of execution in Octave programs.All the control statements start with special keywords such as if and while, to distinguish them from simple expressions.Many control statements contain other ...
The [1,2] on the right hand side of the assignment is interpreted as array with the two elements 1 and 2. If you want to do the multi-variable-assignment in one line, you can use deal in Matlab. This should work in Octave as well according to the documentation here. >> [a,b] = deal(1,2) a =. 1.