Forgot password? New user? Sign up
Existing user? Log in
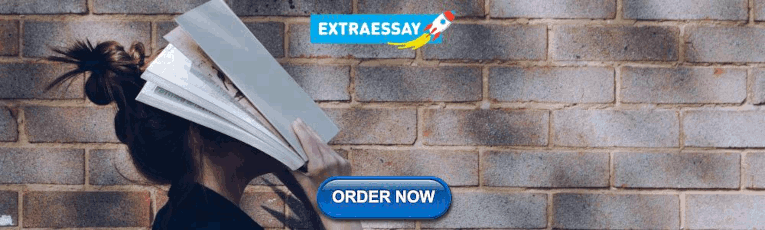
Hungarian Maximum Matching Algorithm
Already have an account? Log in here.
The Hungarian matching algorithm , also called the Kuhn-Munkres algorithm, is a \(O\big(|V|^3\big)\) algorithm that can be used to find maximum-weight matchings in bipartite graphs , which is sometimes called the assignment problem . A bipartite graph can easily be represented by an adjacency matrix , where the weights of edges are the entries. Thinking about the graph in terms of an adjacency matrix is useful for the Hungarian algorithm.
A matching corresponds to a choice of 1s in the adjacency matrix, with at most one 1 in each row and in each column.
The Hungarian algorithm solves the following problem:
In a complete bipartite graph \(G\), find the maximum-weight matching. (Recall that a maximum-weight matching is also a perfect matching.)
This can also be adapted to find the minimum-weight matching.
Say you are having a party and you want a musician to perform, a chef to prepare food, and a cleaning service to help clean up after the party. There are three companies that provide each of these three services, but one company can only provide one service at a time (i.e. Company B cannot provide both the cleaners and the chef). You are deciding which company you should purchase each service from in order to minimize the cost of the party. You realize that is an example of the assignment problem, and set out to make a graph out of the following information: \(\quad\) Company\(\quad\) \(\quad\) Cost for Musician\(\quad\) \(\quad\) Cost for Chef\(\quad\) \(\quad\) Cost for Cleaners\(\quad\) \(\quad\) Company A\(\quad\) \(\quad\) $108\(\quad\) \(\quad\) $125\(\quad\) \(\quad\) $150\(\quad\) \(\quad\) Company B\(\quad\) \(\quad\) $150\(\quad\) \(\quad\) $135\(\quad\) \(\quad\) $175\(\quad\) \(\quad\) Company C\(\quad\) \(\quad\) $122\(\quad\) \(\quad\) $148\(\quad\) \(\quad\) $250\(\quad\) Can you model this table as a graph? What are the nodes? What are the edges? Show Answer The nodes are the companies and the services. The edges are weighted by the price.
What are some ways to solve the problem above? Since the table above can be thought of as a \(3 \times 3\) matrix, one could certainly solve this problem using brute force, checking every combination and seeing what yields the lowest price. However, there are \(n!\) combinations to check, and for large \(n\), this method becomes very inefficient very quickly.
The Hungarian Algorithm Using an Adjacency Matrix
The hungarian algorithm using a graph.
With the cost matrix from the example above in mind, the Hungarian algorithm operates on this key idea: if a number is added to or subtracted from all of the entries of any one row or column of a cost matrix, then an optimal assignment for the resulting cost matrix is also an optimal assignment for the original cost matrix.
The Hungarian Method [1] Subtract the smallest entry in each row from all the other entries in the row. This will make the smallest entry in the row now equal to 0. Subtract the smallest entry in each column from all the other entries in the column. This will make the smallest entry in the column now equal to 0. Draw lines through the row and columns that have the 0 entries such that the fewest lines possible are drawn. If there are \(n\) lines drawn, an optimal assignment of zeros is possible and the algorithm is finished. If the number of lines is less than \(n\), then the optimal number of zeroes is not yet reached. Go to the next step. Find the smallest entry not covered by any line. Subtract this entry from each row that isn’t crossed out, and then add it to each column that is crossed out. Then, go back to Step 3.
Solve for the optimal solution for the example in the introduction using the Hungarian algorithm described above. Here is the initial adjacency matrix: Subtract the smallest value in each row from the other values in the row: Now, subtract the smallest value in each column from all other values in the column: Draw lines through the row and columns that have the 0 entries such that the fewest possible lines are drawn: There are 2 lines drawn, and 2 is less than 3, so there is not yet the optimal number of zeroes. Find the smallest entry not covered by any line. Subtract this entry from each row that isn’t crossed out, and then add it to each column that is crossed out. Then, go back to Step 3. 2 is the smallest entry. First, subtract from the uncovered rows: Now add to the covered columns: Now go back to step 3, drawing lines through the rows and columns that have 0 entries: There are 3 lines (which is \(n\)), so we are done. The assignment will be where the 0's are in the matrix such that only one 0 per row and column is part of the assignment. Replace the original values: The Hungarian algorithm tells us that it is cheapest to go with the musician from company C, the chef from company B, and the cleaners from company A. We can verify this by brute force. 108 + 135 + 250 = 493 108 + 148 + 175 = 431 150 + 125 + 250 = 525 150 + 148 + 150 = 448 122 + 125 + 175 = 422 122 + 135 + 150 = 407. We can see that 407 is the lowest price and matches the assignment the Hungarian algorithm determined. \(_\square\)
The Hungarian algorithm can also be executed by manipulating the weights of the bipartite graph in order to find a stable, maximum (or minimum) weight matching. This can be done by finding a feasible labeling of a graph that is perfectly matched, where a perfect matching is denoted as every vertex having exactly one edge of the matching.
How do we know that this creates a maximum-weight matching?
A feasible labeling on a perfect match returns a maximum-weighted matching. Suppose each edge \(e\) in the graph \(G\) connects two vertices, and every vertex \(v\) is covered exactly once. With this, we have the following inequality: \[w(M’) = \sum_{e\ \epsilon\ E} w(e) \leq \sum_{e\ \epsilon\ E } \big(l(e_x) + l(e_y)\big) = \sum_{v\ \epsilon\ V} l(v),\] where \(M’\) is any perfect matching in \(G\) created by a random assignment of vertices, and \(l(x)\) is a numeric label to node \(x\). This means that \(\sum_{v\ \epsilon\ V}\ l(v)\) is an upper bound on the cost of any perfect matching. Now let \(M\) be a perfect match in \(G\), then \[w(M) = \sum_{e\ \epsilon\ E} w(e) = \sum_{v\ \epsilon\ V}\ l(v).\] So \(w(M’) \leq w(M)\) and \(M\) is optimal. \(_\square\)
Start the algorithm by assigning any weight to each individual node in order to form a feasible labeling of the graph \(G\). This labeling will be improved upon by finding augmenting paths for the assignment until the optimal one is found.
A feasible labeling is a labeling such that
\(l(x) + l(y) \geq w(x,y)\ \forall x \in X, y \in Y\), where \(X\) is the set of nodes on one side of the bipartite graph, \(Y\) is the other set of nodes, \(l(x)\) is the label of \(x\), etc., and \(w(x,y)\) is the weight of the edge between \(x\) and \(y\).
A simple feasible labeling is just to label a node with the number of the largest weight from an edge going into the node. This is certain to be a feasible labeling because if \(A\) is a node connected to \(B\), the label of \(A\) plus the label of \(B\) is greater than or equal to the weight \(w(x,y)\) for all \(y\) and \(x\).
A feasible labeling of nodes, where labels are in red [2] .
Imagine there are four soccer players and each can play a few positions in the field. The team manager has quantified their skill level playing each position to make assignments easier.
How can players be assigned to positions in order to maximize the amount of skill points they provide?
The algorithm starts by labeling all nodes on one side of the graph with the maximum weight. This can be done by finding the maximum-weighted edge and labeling the adjacent node with it. Additionally, match the graph with those edges. If a node has two maximum edges, don’t connect them.
Although Eva is the best suited to play defense, she can't play defense and mid at the same time!
If the matching is perfect, the algorithm is done as there is a perfect matching of maximum weights. Otherwise, there will be two nodes that are not connected to any other node, like Tom and Defense. If this is the case, begin iterating.
Improve the labeling by finding the non-zero label vertex without a match, and try to find the best assignment for it. Formally, the Hungarian matching algorithm can be executed as defined below:
The Hungarian Algorithm for Graphs [3] Given: the labeling \(l\), an equality graph \(G_l = (V, E_l)\), an initial matching \(M\) in \(G_l\), and an unmatched vertex \(u \in V\) and \(u \notin M\) Augmenting the matching A path is augmenting for \(M\) in \(G_l\) if it alternates between edges in the matching and edges not in the matching, and the first and last vertices are free vertices , or unmatched, in \(M\). We will keep track of a candidate augmenting path starting at the vertex \(u\). If the algorithm finds an unmatched vertex \(v\), add on to the existing augmenting path \(p\) by adding the \(u\) to \(v\) segment. Flip the matching by replacing the edges in \(M\) with the edges in the augmenting path that are not in \(M\) \((\)in other words, the edges in \(E_l - M).\) Improving the labeling \(S \subseteq X\) and \(T \subseteq Y,\) where \(S\) and \(T\) represent the candidate augmenting alternating path between the matching and the edges not in the matching. Let \(N_l(S)\) be the neighbors to each node that is in \(S\) along edges in \(E_l\) such that \(N_l(S) = \{v|\forall u \in S: (u,v) \in E_l\}\). If \(N_l(S) = T\), then we cannot increase the size of the alternating path (and therefore can't further augment), so we need to improve the labeling. Let \(\delta_l\) be the minimum of \(l(u) + l(v) - w(u,v)\) over all of the \(u \in S\) and \(v \notin T\). Improve the labeling \(l\) to \(l'\): If \(r \in S,\) then \(l'(r) = l(r) - \delta_l,\) If \(r \in T,\) then \(l'(r) = l(r) + \delta_l.\) If \(r \notin S\) and \(r \notin T,\) then \(l'(r) = l(r).\) \(l'\) is a valid labeling and \(E_l \subset E_{l'}.\) Putting it all together: The Hungarian Algorithm Start with some matching \(M\), a valid labeling \(l\), where \(l\) is defined as the labelling \(\forall x \in X, y \in Y| l(y) = 0, l(x) = \text{ max}_{y \in Y}(w\big(x, y)\big)\). Do these steps until a perfect matching is found \((\)when \(M\) is perfect\():\) (a) Look for an augmenting path in \(M.\) (b) If an augmenting path does not exist, improve the labeling and then go back to step (a).
Each step will increase the size of the matching \(M\) or it will increase the size of the set of labeled edges, \(E_l\). This means that the process will eventually terminate since there are only so many edges in the graph \(G\). [4]
When the process terminates, \(M\) will be a perfect matching. By the Kuhn-Munkres theorem , this means that the matching is a maximum-weight matching.
The algorithm defined above can be implemented in the soccer scenario. First, the conflicting node is identified, implying that there is an alternating tree that must be reconfigured.
There is an alternating path between defense, Eva, mid, and Tom.
To find the best appropriate node, find the minimum \(\delta_l\), as defined in step 4 above, where \(l_u\) is the label for player \(u,\) \(l_v\) is the label for position \(v,\) and \(w_{u, v}\) is the weight on that edge.
The \(\delta_l\) of each unmatched node is computed, where the minimum is found to be a value of 2, between Tom playing mid \((8 + 0 – 6 = 2).\)
The labels are then augmented and the new edges are graphed in the example. Notice that defense and mid went down by 2 points, whereas Eva’s skillset got back two points. However, this is expected as Eva can't play in both positions at once.
Augmenting path leads to relabeling of nodes, which gives rise to the maximum-weighted path.
These new edges complete the perfect matching of the graph, which implies that a maximum-weighted graph has been found and the algorithm can terminate.
The complexity of the algorithm will be analyzed using the graph-based technique as a reference, yet the result is the same as for the matrix-based one.
Algorithm analysis [3] At each \(a\) or \(b\) step, the algorithm adds one edge to the matching and this happens \(O\big(|V|\big)\) times. It takes \(O\big(|V|\big)\) time to find the right vertex for the augmenting (if there is one at all), and it is \(O\big(|V|\big)\) time to flip the matching. Improving the labeling takes \(O\big(|V|\big)\) time to find \(\delta_l\) and to update the labelling accordingly. We might have to improve the labeling up to \(O\big(|V|\big)\) times if there is no augmenting path. This makes for a total of \(O\big(|V|^2\big)\) time. In all, there are \(O\big(|V|\big)\) iterations each taking \(O\big(|V|\big)\) work, leading to a total running time of \(O\big(|V|^3\big)\).
- Matching Algorithms
- Bruff, D. The Assignment Problem and the Hungarian Method . Retrieved June 26, 2016, from http://www.math.harvard.edu/archive/20_spring_05/handouts/assignment_overheads.pdf
- Golin, M. Bipartite Matching & the Hungarian Method . Retrieved Retrieved June 26th, 2016, from http://www.cse.ust.hk/~golin/COMP572/Notes/Matching.pdf
- Grinman, A. The Hungarian Algorithm for Weighted Bipartite Graphs . Retrieved June 26, 2016, from http://math.mit.edu/~rpeng/18434/hungarianAlgorithm.pdf
- Golin, M. Bipartite Matching & the Hungarian Method . Retrieved June 26, 2016, from http://www.cse.ust.hk/~golin/COMP572/Notes/Matching.pdf
Problem Loading...
Note Loading...
Set Loading...
- WolframAlpha.com
- WolframCloud.com
- All Sites & Public Resources...
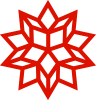
- Wolfram|One
- Mathematica
- Wolfram|Alpha Notebook Edition
- Finance Platform
- System Modeler
- Wolfram Player
- Wolfram Engine
- WolframScript
- Enterprise Private Cloud
- Application Server
- Enterprise Mathematica
- Wolfram|Alpha Appliance
- Corporate Consulting
- Technical Consulting
- Wolfram|Alpha Business Solutions
- Data Repository
- Neural Net Repository
- Function Repository
- Wolfram|Alpha Pro
- Problem Generator
- Products for Education
- Wolfram Cloud App
- Wolfram|Alpha for Mobile
- Wolfram|Alpha-Powered Apps
- Paid Project Support
- Summer Programs
- All Products & Services »
- Wolfram Language Revolutionary knowledge-based programming language. Wolfram Cloud Central infrastructure for Wolfram's cloud products & services. Wolfram Science Technology-enabling science of the computational universe. Wolfram Notebooks The preeminent environment for any technical workflows. Wolfram Engine Software engine implementing the Wolfram Language. Wolfram Natural Language Understanding System Knowledge-based broadly deployed natural language. Wolfram Data Framework Semantic framework for real-world data. Wolfram Universal Deployment System Instant deployment across cloud, desktop, mobile, and more. Wolfram Knowledgebase Curated computable knowledge powering Wolfram|Alpha.
- All Technologies »
- Aerospace & Defense
- Chemical Engineering
- Control Systems
- Electrical Engineering
- Image Processing
- Industrial Engineering
- Mechanical Engineering
- Operations Research
- Actuarial Sciences
- Bioinformatics
- Data Science
- Econometrics
- Financial Risk Management
- All Solutions for Education
- Machine Learning
- Multiparadigm Data Science
- High-Performance Computing
- Quantum Computation Framework
- Software Development
- Authoring & Publishing
- Interface Development
- Web Development
- All Solutions »
- Wolfram Language Documentation
- Fast Introduction for Programmers
- Videos & Screencasts
- Wolfram Language Introductory Book
- Webinars & Training
- Support FAQ
- Wolfram Community
- Contact Support
- All Learning & Support »
- Company Background
- Wolfram Blog
- Careers at Wolfram
- Internships
- Other Wolfram Language Jobs
- Wolfram Foundation
- Computer-Based Math
- A New Kind of Science
- Wolfram Technology for Hackathons
- Student Ambassador Program
- Wolfram for Startups
- Demonstrations Project
- Wolfram Innovator Awards
- Wolfram + Raspberry Pi
- All Company »
Wolfram Language ™
Optimal assignment problem.
Find the amount of electricity a company must send from its four power plants to five cities so as to maximize profit and minimize cost while meeting the cities' peak demands.
This example demonstrates how LinearFractionalOptimization may be used to minimize the ratio of cost to profit within given constraints. Use of a matrix-valued variable makes the modeling relatively simple.
As an example, here is the cost of transporting one million kilowatt hours (kWh) of electricity from four plants to five cities.
The profit that each power plant generates by selling 1 million kWh to each city is shown here.
The cities have a peak demand of 45, 20, 30, 30 and 40 million kWh, respectively, and a minimum demand of 5 million kWh.
The power plants can supply a minimum of 35, 50, 40 and 40 million kWh of electricity, respectively.
The optimal amount of electricity to send each city by each plant can be found by minimizing the ratio of cost to profit.
The breakdown of electricity supplied is shown.
Related Examples

- Wolfram|Alpha Notebook Edition
- Mobile Apps
- Wolfram Workbench
- Volume & Site Licensing
- View all...
- For Customers
- Online Store
- Product Registration
- Product Downloads
- Service Plans Benefits
- User Portal
- Your Account
- Customer Service
- Get Started with Wolfram
- Fast Introduction for Math Students
- Public Resources
- Wolfram|Alpha
- Resource System
- Connected Devices Project
- Wolfram Data Drop
- Wolfram Science
- Computational Thinking
- About Wolfram
- Legal & Privacy Policy


www.springer.com The European Mathematical Society
- StatProb Collection
- Recent changes
- Current events
- Random page
- Project talk
- Request account
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information
- View source
Assignment problem
The problem of optimally assigning $ m $ individuals to $ m $ jobs. It can be formulated as a linear programming problem that is a special case of the transport problem :
maximize $ \sum _ {i,j } c _ {ij } x _ {ij } $
$$ \sum _ { j } x _ {ij } = a _ {i} , i = 1 \dots m $$
(origins or supply),
$$ \sum _ { i } x _ {ij } = b _ {j} , j = 1 \dots n $$
(destinations or demand), where $ x _ {ij } \geq 0 $ and $ \sum a _ {i} = \sum b _ {j} $, which is called the balance condition. The assignment problem arises when $ m = n $ and all $ a _ {i} $ and $ b _ {j} $ are $ 1 $.
If all $ a _ {i} $ and $ b _ {j} $ in the transposed problem are integers, then there is an optimal solution for which all $ x _ {ij } $ are integers (Dantzig's theorem on integral solutions of the transport problem).
In the assignment problem, for such a solution $ x _ {ij } $ is either zero or one; $ x _ {ij } = 1 $ means that person $ i $ is assigned to job $ j $; the weight $ c _ {ij } $ is the utility of person $ i $ assigned to job $ j $.
The special structure of the transport problem and the assignment problem makes it possible to use algorithms that are more efficient than the simplex method . Some of these use the Hungarian method (see, e.g., [a5] , [a1] , Chapt. 7), which is based on the König–Egervary theorem (see König theorem ), the method of potentials (see [a1] , [a2] ), the out-of-kilter algorithm (see, e.g., [a3] ) or the transportation simplex method.
In turn, the transportation problem is a special case of the network optimization problem.
A totally different assignment problem is the pole assignment problem in control theory.
- This page was last edited on 5 April 2020, at 18:48.
- Privacy policy
- About Encyclopedia of Mathematics
- Disclaimers
- Impressum-Legal
- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
- Hungarian Algorithm for Assignment Problem | Set 2 (Implementation)
- Count of nodes with maximum connection in an undirected graph
- Erdos Renyl Model (for generating Random Graphs)
- Types of Graphs with Examples
- Clustering Coefficient in Graph Theory
- Maximum number of edges in Bipartite graph
- Find node having maximum number of common nodes with a given node K
- Convert the undirected graph into directed graph such that there is no path of length greater than 1
- Count of Disjoint Groups by grouping points that are at most K distance apart
- Maximize count of nodes disconnected from all other nodes in a Graph
- Program to find the number of region in Planar Graph
- Cost of painting n * m grid
- Ways to Remove Edges from a Complete Graph to make Odd Edges
- Number of Simple Graph with N Vertices and M Edges
- Balance pans using given weights that are powers of a number
- Chiliagon Number
- Sum of all the numbers in the Nth parenthesis
- Shortest path in a graph from a source S to destination D with exactly K edges for multiple Queries
- Find if two given Quadratic equations have common roots or not
Hungarian Algorithm for Assignment Problem | Set 1 (Introduction)
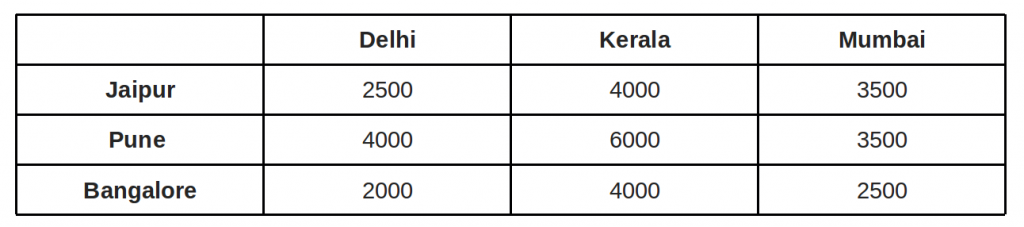
- For each row of the matrix, find the smallest element and subtract it from every element in its row.
- Do the same (as step 1) for all columns.
- Cover all zeros in the matrix using minimum number of horizontal and vertical lines.
- Test for Optimality: If the minimum number of covering lines is n, an optimal assignment is possible and we are finished. Else if lines are lesser than n, we haven’t found the optimal assignment, and must proceed to step 5.
- Determine the smallest entry not covered by any line. Subtract this entry from each uncovered row, and then add it to each covered column. Return to step 3.
Try it before moving to see the solution
Explanation for above simple example:
An example that doesn’t lead to optimal value in first attempt: In the above example, the first check for optimality did give us solution. What if we the number covering lines is less than n.
Time complexity : O(n^3), where n is the number of workers and jobs. This is because the algorithm implements the Hungarian algorithm, which is known to have a time complexity of O(n^3).
Space complexity : O(n^2), where n is the number of workers and jobs. This is because the algorithm uses a 2D cost matrix of size n x n to store the costs of assigning each worker to a job, and additional arrays of size n to store the labels, matches, and auxiliary information needed for the algorithm.
In the next post, we will be discussing implementation of the above algorithm. The implementation requires more steps as we need to find minimum number of lines to cover all 0’s using a program. References: http://www.math.harvard.edu/archive/20_spring_05/handouts/assignment_overheads.pdf https://www.youtube.com/watch?v=dQDZNHwuuOY
Please Login to comment...
- Mathematical
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Quadratic assignment problem
Author: Thomas Kueny, Eric Miller, Natasha Rice, Joseph Szczerba, David Wittmann (SysEn 5800 Fall 2020)
- 1 Introduction
- 2.1 Koopmans-Beckman Mathematical Formulation
- 2.2.1 Parameters
- 2.3.1 Optimization Problem
- 2.4 Computational Complexity
- 2.5 Algorithmic Discussions
- 2.6 Branch and Bound Procedures
- 2.7 Linearizations
- 3.1 QAP with 3 Facilities
- 4.1 Inter-plant Transportation Problem
- 4.2 The Backboard Wiring Problem
- 4.3 Hospital Layout
- 4.4 Exam Scheduling System
- 5 Conclusion
- 6 References
Introduction
The Quadratic Assignment Problem (QAP), discovered by Koopmans and Beckmann in 1957 [1] , is a mathematical optimization module created to describe the location of invisible economic activities. An NP-Complete problem, this model can be applied to many other optimization problems outside of the field of economics. It has been used to optimize backboards, inter-plant transportation, hospital transportation, exam scheduling, along with many other applications not described within this page.
Theory, Methodology, and/or Algorithmic Discussions
Koopmans-beckman mathematical formulation.
Economists Koopmans and Beckman began their investigation of the QAP to ascertain the optimal method of locating important economic resources in a given area. The Koopmans-Beckman formulation of the QAP aims to achieve the objective of assigning facilities to locations in order to minimize the overall cost. Below is the Koopmans-Beckman formulation of the QAP as described by neos-guide.org.
Quadratic Assignment Problem Formulation
Inner Product
Note: The true objective cost function only requires summing entries above the diagonal in the matrix comprised of elements
Since this matrix is symmetric with zeroes on the diagonal, dividing by 2 removes the double count of each element to give the correct cost value. See the Numerical Example section for an example of this note.
Optimization Problem
With all of this information, the QAP can be summarized as:
Computational Complexity
QAP belongs to the classification of problems known as NP-complete, thus being a computationally complex problem. QAP’s NP-completeness was proven by Sahni and Gonzalez in 1976, who states that of all combinatorial optimization problems, QAP is the “hardest of the hard”. [2]
Algorithmic Discussions
While an algorithm that can solve QAP in polynomial time is unlikely to exist, there are three primary methods for acquiring the optimal solution to a QAP problem:
- Dynamic Program
- Cutting Plane
Branch and Bound Procedures
The third method has been proven to be the most effective in solving QAP, although when n > 15, QAP begins to become virtually unsolvable.
The Branch and Bound method was first proposed by Ailsa Land and Alison Doig in 1960 and is the most commonly used tool for solving NP-hard optimization problems.
A branch-and-bound algorithm consists of a systematic enumeration of candidate solutions by means of state space search: the set of candidate solutions is thought of as forming a rooted tree with the full set at the root. The algorithm explores branches of this tree, which represent subsets of the solution set. Before one lists all of the candidate solutions of a branch, the branch is checked against upper and lower estimated bounds on the optimal solution, and the branch is eliminated if it cannot produce a better solution than the best one found so far by the algorithm.
Linearizations
The first attempts to solve the QAP eliminated the quadratic term in the objective function of
in order to transform the problem into a (mixed) 0-1 linear program. The objective function is usually linearized by introducing new variables and new linear (and binary) constraints. Then existing methods for (mixed) linear integer programming (MILP) can be applied. The very large number of new variables and constraints, however, usually poses an obstacle for efficiently solving the resulting linear integer programs. MILP formulations provide LP relaxations of the problem which can be used to compute lower bounds.
Numerical Example
Qap with 3 facilities.
Applications
Inter-plant transportation problem.
The QAP was first introduced by Koopmans and Beckmann to address how economic decisions could be made to optimize the transportation costs of goods between both manufacturing plants and locations. [1] Factoring in the location of each of the manufacturing plants as well as the volume of goods between locations to maximize revenue is what distinguishes this from other linear programming assignment problems like the Knapsack Problem.
The Backboard Wiring Problem
As the QAP is focused on minimizing the cost of traveling from one location to another, it is an ideal approach to determining the placement of components in many modern electronics. Leon Steinberg proposed a QAP solution to optimize the layout of elements on a blackboard by minimizing the total amount of wiring required. [4]
When defining the problem Steinberg states that we have a set of n elements
as well as a set of r points
In his paper he derives the below formula:
In his paper Steinberg a backboard with a 9 by 4 array, allowing for 36 potential positions for the 34 components that needed to be placed on the backboard. For the calculation, he selected a random initial placement of s1 and chose a random family of 25 unconnected sets.
The initial placement of components is shown below:
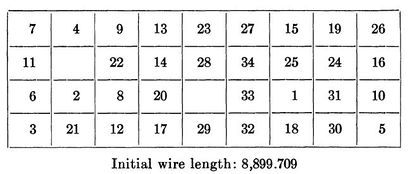
After the initial placement of elements, it took an additional 35 iterations to get us to our final optimized backboard layout. Leading to a total of 59 iterations and a final wire length of 4,969.440.
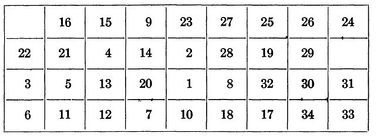
Hospital Layout
Building new hospitals was a common event in 1977 when Alealid N Elshafei wrote his paper on "Hospital Layouts as a Quadratic Assignment Problem". [5] With the high initial cost to construct the hospital and to staff it, it is important to ensure that it is operating as efficiently as possible. Elshafei's paper was commissioned to create an optimization formula to locate clinics within a building in such a way that minimizes the total distance that a patient travels within the hospital throughout the year. When doing a study of a major hospital in Cairo he determined that the Outpatient ward was acting as a bottleneck in the hospital and focused his efforts on optimizing the 17 departments there.
Elshafei identified the following QAP to determine where clinics should be placed:
For the Cairo hospital with 17 clinics, and one receiving and recording room bringing us to a total of 18 facilities. By running the above optimization Elshafei was able to get the total distance per year down to 11,281,887 from a distance of 13,973,298 based on the original hospital layout.
Exam Scheduling System
The scheduling system uses matrices for Exams, Time Slots, and Rooms with the goal of reducing the rate of schedule conflicts. To accomplish this goal, the “examination with the highest cross faculty student is been prioritized in the schedule after which the examination with the highest number of cross-program is considered and finally with the highest number of repeating student, at each stage group with the highest number of student are prioritized.” [6]
- ↑ 1.0 1.1 1.2 Koopmans, T., & Beckmann, M. (1957). Assignment Problems and the Location of Economic Activities. Econometrica, 25(1), 53-76. doi:10.2307/1907742
- ↑ 2.0 2.1 Quadratic Assignment Problem. (2020). Retrieved December 14, 2020, from https://neos-guide.org/content/quadratic-assignment-problem
- ↑ 3.0 3.1 3.2 Burkard, R. E., Çela, E., Pardalos, P. M., & Pitsoulis, L. S. (2013). The Quadratic Assignment Problem. https://www.opt.math.tugraz.at/~cela/papers/qap_bericht.pdf .
- ↑ 4.0 4.1 Leon Steinberg. The Backboard Wiring Problem: A Placement Algorithm. SIAM Review . 1961;3(1):37.
- ↑ 5.0 5.1 Alwalid N. Elshafei. Hospital Layout as a Quadratic Assignment Problem. Operational Research Quarterly (1970-1977) . 1977;28(1):167. doi:10.2307/300878
- ↑ 6.0 6.1 Muktar, D., & Ahmad, Z.M. (2014). Examination Scheduling System Based On Quadratic Assignment.
Navigation menu
Linear Assignment Problems in Combinatorial Optimization
- First Online: 07 December 2017
Cite this chapter
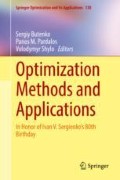
- Boris Goldengorin 6 , 7 &
- Dmitry Krushinsky 8
Part of the book series: Springer Optimization and Its Applications ((SOIA,volume 130))
1286 Accesses
1 Citations
In this chapter we introduce the notion of a “pattern” in the Linear Assignment Problem and show that patterns may be useful to create new insights and approaches for many combinatorial optimization problems defined on a rectangular input matrix. We define a pattern as a specific collection of cells in the rectangular matrix reflecting the structure of an optimal solution to the original combinatorial optimization problem (COP). We illustrate the notion of pattern by means of some well-known problems in combinatorial optimization, including the variations of the Linear Ordering Problem, the Cell Formation Problem, and some others. Then, we give a detailed consideration to pattern-based solution approaches for the two mentioned problems.
- Linear Assignment Problem (LAP)
- Linear Ordering Problem (LOP)
- Cell Formation Problem (CFP)
- Feasible Patterns
- Multicut Problem
These keywords were added by machine and not by the authors. This process is experimental and the keywords may be updated as the learning algorithm improves.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
Institutional subscriptions
See http://www.iwr.uni-heidelberg.de/iwr/comopt/soft/LOLIB/LOLIB.html .
Ballakur, A., Steudel, H.J.: A within cell utilization based heuristic for designing cellular manufacturing systems. Int. J. Prod. Res. 25 , 639–655 (1987)
Article Google Scholar
Bekker, H., Braad, E.P., Goldengorin, B.: Using bipartite and multidimensional matching to select the roots of a system of polynomial equations. Lect. Notes Comput. Sci. 3483 , 397–406 (2003)
Garey, M.R., Johnson, D.S.: Computers and Intractability: A Guide to the Theory of NP-Completeness. W.H. Freeman, New York (1979)
MATH Google Scholar
Goldengorin, B., Krushinsky, D., Slomp, J.: Flexible PMP approach for large-size cell formation. Oper. Res. 60 (5), 1157–1166 (2012)
Article MathSciNet MATH Google Scholar
Goldengorin, B., Krushinsky, D., Pardalos, P.: Cell Formation in Industrial Engineering. Springer, New York (2013)
Book MATH Google Scholar
Goncalves, J.F., Resende, M.C.G.: An evolutionary algorithm for manufacturing cell formation. Comput. Ind. Eng. 47 , 247–273 (2004)
Jünger, M.: Polyhedral Combinatorics and the Acyclic Subdigraph Problem. Heldermann Verlag, Berlin (1985)
Krishnamoorthy, M.S.: An NP-hard problem in bipartite graphs. SIGACT News 7 (1), 26 (1975)
Kuhn, H.W.: The Hungarian method for the assignment problem. Nav. Res. Logist. Q. 2 , 83–97 (1955)
McCormick, W.T., Schweitzer, P.J., White, T.W.: Problem decomposition and data reorganization by a clustering technique. Oper. Res. 20 (5), 993–1009 (1972)
Article MATH Google Scholar
Nering, E.D., Tucker, A.W.: Linear Programs and related Problems. Academic, San Diego (1993)
Google Scholar
Reinelt, G.: The Linear Ordering Problem. Heldermann Verlag, Berlin (1985)
Waghodekar, P.H., Sahu, S.: Machine-component cell formation in group technology: MACE. Int. J. Prod. Res. 22 (6), 937–948 (1984)
Download references
Author information
Authors and affiliations.
Ohio University, Athens, OH, USA
Boris Goldengorin
University of Florida, Gainesville, FL, USA
Wageningen University and Research, Wageningen, The Netherlands
Dmitry Krushinsky
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to Dmitry Krushinsky .
Editor information
Editors and affiliations.
Industrial & Systems Engineering, Texas A&M University, College Station, Texas, USA
Sergiy Butenko
Department of Industrial and Systems Engineering, University of Florida, Gainesville, Florida, USA
Panos M. Pardalos
Methods of Discrete Optimization, V.M. Glushkov Institute of Cybernetics, Kyiv, Ukraine
Volodymyr Shylo
Rights and permissions
Reprints and permissions
Copyright information
© 2017 Springer International Publishing AG
About this chapter
Goldengorin, B., Krushinsky, D. (2017). Linear Assignment Problems in Combinatorial Optimization. In: Butenko, S., Pardalos, P., Shylo, V. (eds) Optimization Methods and Applications . Springer Optimization and Its Applications, vol 130. Springer, Cham. https://doi.org/10.1007/978-3-319-68640-0_9
Download citation
DOI : https://doi.org/10.1007/978-3-319-68640-0_9
Published : 07 December 2017
Publisher Name : Springer, Cham
Print ISBN : 978-3-319-68639-4
Online ISBN : 978-3-319-68640-0
eBook Packages : Mathematics and Statistics Mathematics and Statistics (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research

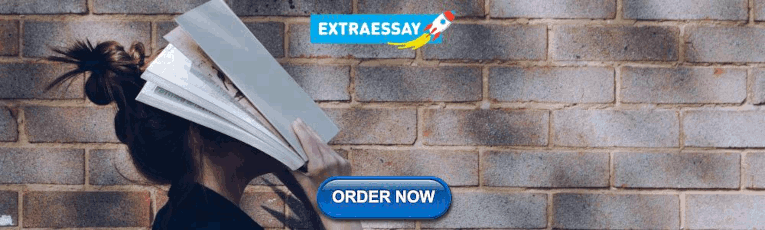
How to Solve the Assignment Problem: A Complete Guide
Table of Contents
Assignment problem is a special type of linear programming problem that deals with assigning a number of resources to an equal number of tasks in the most efficient way. The goal is to minimize the total cost of assignments while ensuring that each task is assigned to only one resource and each resource is assigned to only one task. In this blog, we will discuss the solution of the assignment problem using the Hungarian method, which is a popular algorithm for solving the problem.
Understanding the Assignment Problem
Before we dive into the solution, it is important to understand the problem itself. In the assignment problem, we have a matrix of costs, where each row represents a resource and each column represents a task. The objective is to assign each resource to a task in such a way that the total cost of assignments is minimized. However, there are certain constraints that need to be satisfied – each resource can be assigned to only one task and each task can be assigned to only one resource.
Solving the Assignment Problem
There are various methods for solving the assignment problem, including the Hungarian method, the brute force method, and the auction algorithm. Here, we will focus on the steps involved in solving the assignment problem using the Hungarian method, which is the most commonly used and efficient method.
Step 1: Set up the cost matrix
The first step in solving the assignment problem is to set up the cost matrix, which represents the cost of assigning a task to an agent. The matrix should be square and have the same number of rows and columns as the number of tasks and agents, respectively.
Step 2: Subtract the smallest element from each row and column
To simplify the calculations, we need to reduce the size of the cost matrix by subtracting the smallest element from each row and column. This step is called matrix reduction.
Step 3: Cover all zeros with the minimum number of lines
The next step is to cover all zeros in the matrix with the minimum number of horizontal and vertical lines. This step is called matrix covering.
Step 4: Test for optimality and adjust the matrix
To test for optimality, we need to calculate the minimum number of lines required to cover all zeros in the matrix. If the number of lines equals the number of rows or columns, the solution is optimal. If not, we need to adjust the matrix and repeat steps 3 and 4 until we get an optimal solution.
Step 5: Assign the tasks to the agents
The final step is to assign the tasks to the agents based on the optimal solution obtained in step 4. This will give us the most cost-effective or profit-maximizing assignment.
Solution of the Assignment Problem using the Hungarian Method
The Hungarian method is an algorithm that uses a step-by-step approach to find the optimal assignment. The algorithm consists of the following steps:
- Subtract the smallest entry in each row from all the entries of the row.
- Subtract the smallest entry in each column from all the entries of the column.
- Draw the minimum number of lines to cover all zeros in the matrix. If the number of lines drawn is equal to the number of rows, we have an optimal solution. If not, go to step 4.
- Determine the smallest entry not covered by any line. Subtract it from all uncovered entries and add it to all entries covered by two lines. Go to step 3.
The above steps are repeated until an optimal solution is obtained. The optimal solution will have all zeros covered by the minimum number of lines. The assignments can be made by selecting the rows and columns with a single zero in the final matrix.
Applications of the Assignment Problem
The assignment problem has various applications in different fields, including computer science, economics, logistics, and management. In this section, we will provide some examples of how the assignment problem is used in real-life situations.
Applications in Computer Science
The assignment problem can be used in computer science to allocate resources to different tasks, such as allocating memory to processes or assigning threads to processors.
Applications in Economics
The assignment problem can be used in economics to allocate resources to different agents, such as allocating workers to jobs or assigning projects to contractors.
Applications in Logistics
The assignment problem can be used in logistics to allocate resources to different activities, such as allocating vehicles to routes or assigning warehouses to customers.
Applications in Management
The assignment problem can be used in management to allocate resources to different projects, such as allocating employees to tasks or assigning budgets to departments.
Let’s consider the following scenario: a manager needs to assign three employees to three different tasks. Each employee has different skills, and each task requires specific skills. The manager wants to minimize the total time it takes to complete all the tasks. The skills and the time required for each task are given in the table below:
The assignment problem is to determine which employee should be assigned to which task to minimize the total time required. To solve this problem, we can use the Hungarian method, which we discussed in the previous blog.
Using the Hungarian method, we first subtract the smallest entry in each row from all the entries of the row:
Next, we subtract the smallest entry in each column from all the entries of the column:
We draw the minimum number of lines to cover all the zeros in the matrix, which in this case is three:
Since the number of lines is equal to the number of rows, we have an optimal solution. The assignments can be made by selecting the rows and columns with a single zero in the final matrix. In this case, the optimal assignments are:
- Emp 1 to Task 3
- Emp 2 to Task 2
- Emp 3 to Task 1
This assignment results in a total time of 9 units.
I hope this example helps you better understand the assignment problem and how to solve it using the Hungarian method.
Solving the assignment problem may seem daunting, but with the right approach, it can be a straightforward process. By following the steps outlined in this guide, you can confidently tackle any assignment problem that comes your way.
How useful was this post?
Click on a star to rate it!
Average rating 0 / 5. Vote count: 0
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you! 😔
Let us improve this post!
Tell us how we can improve this post?
Operations Research
1 Operations Research-An Overview
- History of O.R.
- Approach, Techniques and Tools
- Phases and Processes of O.R. Study
- Typical Applications of O.R
- Limitations of Operations Research
- Models in Operations Research
- O.R. in real world
2 Linear Programming: Formulation and Graphical Method
- General formulation of Linear Programming Problem
- Optimisation Models
- Basics of Graphic Method
- Important steps to draw graph
- Multiple, Unbounded Solution and Infeasible Problems
- Solving Linear Programming Graphically Using Computer
- Application of Linear Programming in Business and Industry
3 Linear Programming-Simplex Method
- Principle of Simplex Method
- Computational aspect of Simplex Method
- Simplex Method with several Decision Variables
- Two Phase and M-method
- Multiple Solution, Unbounded Solution and Infeasible Problem
- Sensitivity Analysis
- Dual Linear Programming Problem
4 Transportation Problem
- Basic Feasible Solution of a Transportation Problem
- Modified Distribution Method
- Stepping Stone Method
- Unbalanced Transportation Problem
- Degenerate Transportation Problem
- Transhipment Problem
- Maximisation in a Transportation Problem
5 Assignment Problem
- Solution of the Assignment Problem
- Unbalanced Assignment Problem
- Problem with some Infeasible Assignments
- Maximisation in an Assignment Problem
- Crew Assignment Problem
6 Application of Excel Solver to Solve LPP
- Building Excel model for solving LP: An Illustrative Example
7 Goal Programming
- Concepts of goal programming
- Goal programming model formulation
- Graphical method of goal programming
- The simplex method of goal programming
- Using Excel Solver to Solve Goal Programming Models
- Application areas of goal programming
8 Integer Programming
- Some Integer Programming Formulation Techniques
- Binary Representation of General Integer Variables
- Unimodularity
- Cutting Plane Method
- Branch and Bound Method
- Solver Solution
9 Dynamic Programming
- Dynamic Programming Methodology: An Example
- Definitions and Notations
- Dynamic Programming Applications
10 Non-Linear Programming
- Solution of a Non-linear Programming Problem
- Convex and Concave Functions
- Kuhn-Tucker Conditions for Constrained Optimisation
- Quadratic Programming
- Separable Programming
- NLP Models with Solver
11 Introduction to game theory and its Applications
- Important terms in Game Theory
- Saddle points
- Mixed strategies: Games without saddle points
- 2 x n games
- Exploiting an opponent’s mistakes
12 Monte Carlo Simulation
- Reasons for using simulation
- Monte Carlo simulation
- Limitations of simulation
- Steps in the simulation process
- Some practical applications of simulation
- Two typical examples of hand-computed simulation
- Computer simulation
13 Queueing Models
- Characteristics of a queueing model
- Notations and Symbols
- Statistical methods in queueing
- The M/M/I System
- The M/M/C System
- The M/Ek/I System
- Decision problems in queueing
OPERATIONS RESEARCH
Lesson 9. solution of assignment problem.
Current course
Procedure, Example Solved Problem | Operations Research - Solution of assignment problems (Hungarian Method) | 12th Business Maths and Statistics : Chapter 10 : Operations Research
Chapter: 12th business maths and statistics : chapter 10 : operations research.
Solution of assignment problems (Hungarian Method)
First check whether the number of rows is equal to the numbers of columns, if it is so, the assignment problem is said to be balanced.
Step :1 Choose the least element in each row and subtract it from all the elements of that row.
Step :2 Choose the least element in each column and subtract it from all the elements of that column. Step 2 has to be performed from the table obtained in step 1.
Step:3 Check whether there is atleast one zero in each row and each column and make an assignment as follows.

Step :4 If each row and each column contains exactly one assignment, then the solution is optimal.
Example 10.7
Solve the following assignment problem. Cell values represent cost of assigning job A, B, C and D to the machines I, II, III and IV.
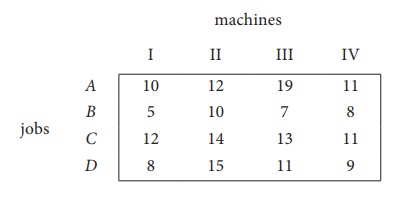
Here the number of rows and columns are equal.
∴ The given assignment problem is balanced. Now let us find the solution.
Step 1: Select a smallest element in each row and subtract this from all the elements in its row.
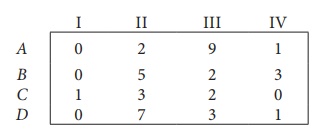
Look for atleast one zero in each row and each column.Otherwise go to step 2.
Step 2: Select the smallest element in each column and subtract this from all the elements in its column.
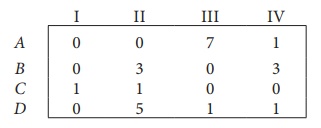
Since each row and column contains atleast one zero, assignments can be made.
Step 3 (Assignment):
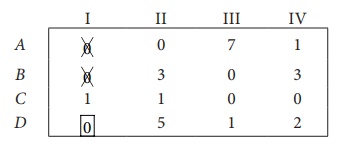
Thus all the four assignments have been made. The optimal assignment schedule and total cost is
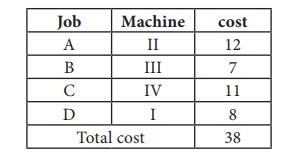
The optimal assignment (minimum) cost
Example 10.8
Consider the problem of assigning five jobs to five persons. The assignment costs are given as follows. Determine the optimum assignment schedule.
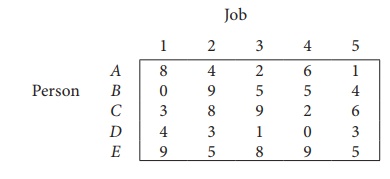
∴ The given assignment problem is balanced.
Now let us find the solution.
The cost matrix of the given assignment problem is
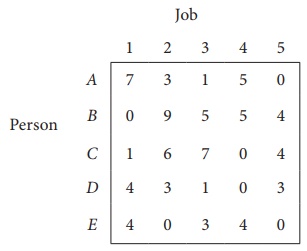
Column 3 contains no zero. Go to Step 2.
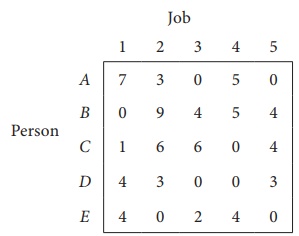
Thus all the five assignments have been made. The Optimal assignment schedule and total cost is
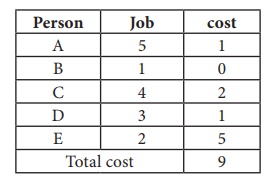
The optimal assignment (minimum) cost = ` 9
Example 10.9
Solve the following assignment problem.
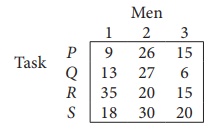
Since the number of columns is less than the number of rows, given assignment problem is unbalanced one. To balance it , introduce a dummy column with all the entries zero. The revised assignment problem is
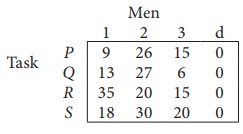
Here only 3 tasks can be assigned to 3 men.
Step 1: is not necessary, since each row contains zero entry. Go to Step 2.
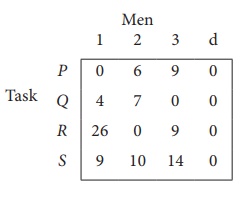
Step 3 (Assignment) :
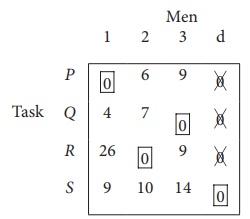
Since each row and each columncontains exactly one assignment,all the three men have been assigned a task. But task S is not assigned to any Man. The optimal assignment schedule and total cost is
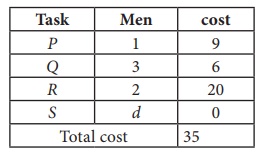
The optimal assignment (minimum) cost = ₹ 35
Related Topics
Privacy Policy , Terms and Conditions , DMCA Policy and Compliant
Copyright © 2018-2024 BrainKart.com; All Rights Reserved. Developed by Therithal info, Chennai.
Hungarian Algorithm Calculator Online
The Hungarian Algorithm Calculator is a powerful tool used to solve optimization problems known as the assignment problem. It finds the optimal assignment of tasks to resources, minimizing the total cost or maximizing the total profit. This calculator employs the Hungarian algorithm, a method that efficiently solves assignment problems by iteratively reducing the problem to a series of steps until an optimal assignment is achieved.
Formula of Hungarian Algorithm Calculator
The Hungarian Algorithm Calculator follows these steps:
Step1: Subtract the minimum value in each row from all the values in that row.
Step2: Subtract the minimum value in each column from all the values in that column.
Step3: Cover all zeros with the minimum number of lines.
Step : Test for optimality. If the number of lines drawn is equal to the number of rows or columns, an optimal assignment is found. If not, proceed to step 5.
Step5: Determine the smallest uncovered value (let it be k) and subtract it from all uncovered values. Then add it to all the values intersected by the lines. Return to step 3.
Step6: The optimal assignment is obtained from the resulting matrix.
You'll need to represent your problem as a matrix of costs or distances, and then apply the Hungarian algorithm steps iteratively until an optimal assignment is found.
General Terms Table
This table provides general terms related to the Hungarian Algorithm Calculator, helping users understand key concepts without needing to calculate each time .
Example of Hungarian Algorithm Calculator
Suppose we have a scenario where three workers (W1, W2, W3) are assign to three tasks (T1, T2, T3). The cost matrix representing the cost of assigning each worker to each task is as follows:
Using the Hungarian Algorithm Calculator, we can find the optimal assignment of workers to tasks. After calculation, the optimal assignment would be:
W1 -> T3 W2 -> T2 W3 -> T1
Most Common FAQs
The Algorithm Calculator is use to find the optimal assignment of tasks to resources, minimizing costs or maximizing profits.
The algorithm works by iteratively reducing the assignment problem to a series of steps until an optimal assignment is find. It involves subtracting row and column minima, covering zeros, and testing for optimality.
Yes, the calculator is applicable to various real-life scenarios such as workforce scheduling, job assignment, and resource allocation.
The calculator provides accurate results based on the input cost matrix and follows the rigorous steps of the algorithm to find the optimal assignment.
Yes, the calculator is capable of handling large datasets efficiently, making it suitable for complex optimization problems.
🚀 Upgrade Your Calculations with AI-Powered Precision!
Solve any problem in a snap with Calculatorshub Ai Calculator.
Related Calculators
Converting Azimuth to Bearing Calculator Online
Complex Division Calculator Online
Commutative Property Calculator Online
Cofactors Calculator Online
Clockwise Rotation Calculator Online
BODMAS Calculator Online
Babylonian Numeral Calculator Online
Average Slope Calculator Online
Angles of Polygons Calculator Online
Angle Addition Postulate Calculator Online
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Help Center Help Center
- Help Center
- Trial Software
- Product Updates
- Documentation
Introduction to Assignment Methods in Tracking Systems
In a multiple target tracking (MTT) system, one or more sensors generate multiple detections from multiple targets in a scan. To track these targets, one essential step is to assign these detections correctly to the targets or tracks maintained in the tracker so that these detections can be used to update these tracks. If the number of targets or detections is large, or there are conflicts between different assignment hypotheses, assigning detections is challenging.
Depending on the dimension of the assignment, assignment problems can be categorized into:
2-D assignment problem – assigns n targets to m observations. For example, assign 5 tracks to 6 detections generated from one sensor at one time step.
S-D assignment problem – assigns n targets to a set ( m 1 , m 2 , m 3 , …) of observations. For example, assign 5 tracks to 6 detections from one sensor, and 4 detections from another sensor at the same time. This example is a typical 3-D assignment problem.
To illustrate the basic idea of an assignment problem, consider a simple 2-D assignment example. One company tries to assign 3 jobs to 3 workers. Because of the different experience levels of the workers, not all workers are able to complete each job with the same effectiveness. The cost (in hours) of each worker to finish each job is given by the cost matrix shown in the table. An assignment rule is that each worker can only take one job, and one job can only be taken by one worker. To guarantee efficiency, the object of this assignment is to minimize the total cost.
Since the numbers of workers and jobs are both small in this example, all the possible assignments can be obtained by enumeration, and the minimal cost solution is highlighted in the table with assignment pairs (1, 3), (2, 2) and (3, 1). In practice, as the size of the assignment becomes larger, the optimal solution is difficult to obtain for 2-D assignment. For an S-D assignment problem, the optimal solution may not be obtainable in practice.
2-D Assignment in Multiple Target Tracking
In the 2-D MTT assignment problem, a tracker tries to assign multiple tracks to multiple detections. Other than the dimensionality challenge mentioned above, a few other factors can significantly change the complexity of the assignment:
Target or detection distribution — If targets are sparsely distributed, associating a target to its corresponding detection is relatively easy. However, if targets or detections are densely distributed, assignments become ambiguous because assigning a target to a detection or another nearby detection rarely makes any differences on the cost.
Probability of detection ( P d ) of the sensor — P d describes the probability that a target is detected by the sensor if the target is within the field of view of the sensor. If the P d of a sensor is small, then the true target may not give rise to any detection during a sensor scan. As a result, the track represented by the true target may steal detections from other tracks.
Sensor resolution — Sensor resolution determines the sensor’s ability to distinguish the detections from two targets. If the sensor resolution is low, then two targets in proximity may only give rise to one detection. This violates the common assumption that each detection can only be assigned to one track and results in unresolvable assignment conflicts between tracks.
Clutter or false alarm rate of the sensor — False alarms introduce additional possible assignments and therefore increase the complexity of data assignment.
The complexity of the assignment task can determine which assignment methods to apply. In Sensor Fusion and Tracking Toolbox™ toolbox, three 2-D assignment approaches are employed corresponding to three different trackers:
trackerGNN — adopts a global nearest data assignment approach
trackerJPDA — adopts a joint probability data association approach
trackerTOMHT — adopts a tracker-oriented multiple hypothesis tracking approach
Note that each tracker processes the data from sensors sequentially, meaning that each tracker only deals with the assignment problem with the detections of one sensor at a time. Even with this treatment, there may still be too many assignment pairs. To reduce the number of track and detection pairs considered for assignment, the gating technique is frequently used.
Gating is a screening mechanism to determine which observations are valid candidates to update existing tracks and eliminate unlikely detection-to-track pairs using the distribution information of the predicted tracks. To establish the validation gate for a track at the current scan, the estimated track for the current step is predicted from the previous step.
For example, a tracker confirms a track at time t k and receives several detections at time t k +1 . To form a validation gate at time t k +1 , the tracker first needs to obtain the predicted measurement as:
y ^ k + 1 = h ( x ^ k + 1 | k )
where x ^ k + 1 | k is the track estimate predicted from time t k and h ( x ^ k + 1 | k ) is the measurement model that outputs the expected measurement given the track state. The observation residual vector is
y ˜ = y k + 1 − y ^ k + 1
where y k +1 is the actual measurement. To establish the boundary of the gate, the detection residual covariance S is used to form an ellipsoidal validation gate. The ellipsoidal gate that establishes a spatial ellipsoidal region in the measurement space is defined in Mahalanobis distance as:
d 2 ( y k + 1 ) = y ˜ T S − 1 y ˜ ≤ G
where G is the gating threshold which you can specify based on the assignment requirement. Increasing the threshold can incorporate more detections into the gate.
After the assignment gate is established for each track, the gating status of each detection y i ( i = 1,…, n ) can be determined by comparing its Mahalanobis distance d 2 ( y i ) with the gating threshold G . If d 2 ( y i ) < G , then detection y i is inside the gate of the track and will be considered for association. Otherwise, the possibility of the detection associated with the track is removed. In Figure 1, T 1 represents a predicted track estimate, and O 1 – O 6 are six detections. Based on the gating result, O 1 , O 2 , and O 3 are within the validation gate in the figure.

Global Nearest Neighbor (GNN) Method
The GNN method is a single hypothesis assignment method. For each new data set, the goal is to assign the global nearest observations to existing tracks and to create new track hypotheses for unassigned detections.
The GNN assignment problem can be easily solved if there are no conflicts of association between tracks. The tracker only needs to assign a track to its nearest neighbor. However, conflict situations (see Figure 2) occur when there is more than one observation within a track’s validation gate or an observation is in the gates of more than one track. To resolve these conflicts, the tracker must evaluate a cost matrix.

The elements of a cost matrix for the GNN method includes the distance from tracks to detections and other factors you might want to consider. For example, one approach is to define a generalized statistical distance between observation j to track i as:
C i j = d i j + ln ( | S i j | )
where d ij is the Mahalanobis distance and ln(| S ij |), the logarithm of the determinant of the residual covariance matrix, is used to penalize tracks with greater prediction uncertainty.
For the assignment problem given in Figure 2, the following table shows a hypothetical cost matrix. The nonallowed assignments, which failed the gating test, are denoted by X. (In practice, the costs of nonallowed assignments can be denoted by large values, such as 1000.)
For this problem, the highlighted optimal solution can be found by enumeration. Detection O 3 is unassigned, so the tracker will use it to create a new tentative track. For more complicated GNN assignment problems, more accurate formulations and more efficient algorithms to obtain the optimal or suboptimal solution are required.
A general 2-D assignment problem can be formed as following. Given the cost matrix element C ij , find an assignment Z = { z ij } that minimizes
J = ∑ i = 0 n ∑ j = 0 m C i j z i j
subject to two constraints:
∑ i = 0 m z i j = 1 , ∀ j ∑ j = 0 n z i j = 1 , ∀ i
If track i is assigned to observation j , then z ij = 1. Otherwise, z ij = 0. z i 0 = 1 represents the hypothesis that track i is not assigned to any detection. Similarly, z 0 j = 1 represents the hypothesis that observation j is not assigned to any track. The first constraint means each detection can be assigned to no more than one track. The second constraint means each track can be assigned to no more than one detection.
Sensor Fusion and Tracking Toolbox provides multiple functions to solve 2-D GNN assignment problems:
assignmunkres – Uses the Munkres algorithm, which guarantees an optimal solution but may require more calculation operations.
assignauction – Uses the auction algorithm, which requires fewer operations but can possibly converge on an optimal or suboptimal solution.
assignjv – Uses the Jonker-Volgenant algorithm, which also converges on an optimal or suboptimal solution but usually with a faster converging speed.
In trackerGNN , you can select the assignment algorithm by specifying the Assignment property.
K Best Solutions to the 2-D Assignment Problem
Because of the uncertainty nature of assignment problems, only obtaining a solution (optimal or suboptimal) may not be sufficient. To account for multiple hypotheses about the assignment between tracks and detections, multiple suboptimal solutions are required. These suboptimal solutions are called K best solutions to the assignment problem.
The K best solutions are usually obtained by varying the solution obtained by any of the previously mentioned assignment functions. Then, at the next step, the K best solution algorithm removes one track-to-detection pair in the original solution and finds the next best solution. For example, for this cost matrix:
[ 10 5 8 9 7 × 20 × × × 21 1 5 × 17 × × × × 1 6 22 ]
each row represents the cost associated with a track, and each column represents the cost associated with a detection. As highlighted, the optimal solution is (7,15,16, 9) with a cost of 47. In the next step, remove the first pair (corresponding to 7), and the next best solution is (10,15, 20, 22) with a cost of 67. After that, remove the second pair (corresponding to 15), and the next best solution is (7, 5,16, 9) with a cost of 51. After a few steps, the five best solutions are:
See the Find Five Best Solutions Using Assignkbest example, which uses the assignkbest function to find the K best solutions.
Joint Probability Data Association (JPDA) Method
While the GNN method makes a rigid assignment of a detection to a track, the JPDA method applies a soft assignment so that detections within the validation gate of a track can all make weighted contributions to the track based on their probability of association.
For example, for the gating results shown in Figure 1, a JPDA tracker calculates the possibility of association between track T 1 and observations O 1 , O 2 , and O 3 . Assume the association probability of these three observations are p 11 , p 12 , and p 13 , and their residuals relative to track T 1 are y ˜ 11 , y ˜ 12 , and y ˜ 13 , respectively. Then the weighted sum of the residuals associated with track T 1 is:
y ˜ 1 = ∑ j = 1 3 p 1 j y ˜ 1 j
In the tracker, the weighted residual is used to update track T 1 in the correction step of the tracking filter. In the filter, the probability of unassignment, p 10 , is also required to update track T 1 . For more details, see JPDA Correction Algorithm for Discrete Extended Kalman Filter .
The JPDA method requires one more step when there are conflicts between assignments in different tracks. For example, in the following figure, track T 2 conflicts with T 1 on the assignment of observation O 3 . Therefore, to calculate the association probability p 13 , the joint probability that T 2 is not assigned to O 3 (that is T 2 is assigned to O 6 or unassigned) must be accounted for.

Track-Oriented Multiple Hypothesis Tracking (TOMHT) Method
Unlike the JPDA method, which combines all detections within the validation gate using a weighted sum, the TOMHT method generates multiple hypotheses or branches of the tracks based on the detections within the gate and propagates high-likelihood branches between scan steps. After propagation, these hypotheses can be tested and pruned based on the new set of detections.
For example, for the gating scenario shown in Figure 1, a TOMHT tracker considers the following four hypotheses:
Assign no detection to T 1 resulting in hypothesis T 10
Assign O 1 to T 1 resulting in hypothesis T 11
Assign O 2 to T 1 resulting in hypothesis T 12
Assign O 3 to T 1 resulting in hypothesis T 13
Given the assignment threshold, the tracker will calculate the possibility of each hypothesis and discard hypotheses with probability lower than the threshold. Hypothetically, if only p 10 and p 11 are larger than the threshold, then only T 10 and T 11 are propagated to the next step for detection update.
S-D Assignment in Multiple Target Tracking
In an S-D assignment problem, the dimension of assignment S is larger than 2. Note that all three trackers ( trackerGNN , trackerJPDA , and trackerTOMHT ) process detections from each sensor sequentially, which results in a 2-D assignment problem. However, some applications require a tracker that processes simultaneous observations from multiple sensor scans all at once, which requires solving an S-D assignment problem. Meanwhile, the S-D assignment is widely used in tracking applications such as static data fusion, which preprocesses the detection data before fed to a tracker.
An S-D assignment problem for static data fusion has S scans of a surveillance region from multiple sensors simultaneously, and each scan consists of multiple detections. The detection sources can be real targets or false alarms. The object is to detect an unknown number of targets and estimate their states. For example, as shown in the Figure 4, three sensor scans produce six detections. The detections in the same color belong to the same scan. Since each scan generates two detections, there are probably two targets in the region of surveillance. To choose between different assignment or association possibilities, evaluate the cost matrix.

The calculation of the cost can depend on many factors, such as the distance between detections and the covariance distribution of each detection. To illustrate the basic concept, the assignment costs for a few hypotheses are hypothetically given in the table [1] .
In the table, 0 denotes a track is associated with no detection in that scan. Assume the hypotheses not shown in the table are truncated by gating or neglected because of high costs. To concisely represent each track, use c ijk to represent the cost for association of observation i in scan 1, j in scan 2, and k in scan 3. For example, for the assignment hypothesis 1, c 011 = -10.2. Several track hypotheses conflict with other in the table. For instance, the two most likely assignments, c 111 and c 121 are incompatible because they share the same observation in scans 1 and 3.
The goal of solving an S-D assignment problem is to find the most likely compatible assignment hypothesis accounting for all the detections. When S ≥ 3, however, the problem is known to scale with the number of tracks and detections at an exponential rate (NP-hard). The Lagrangian relaxation method is commonly used to obtain the optimal or sub-optimal solution for an S-D assignment problem efficiently.
Brief Introduce to the Lagrangian Relaxation Method for 3-D Assignment
Three scans of data have a number of M 1 , M 2 , and M 3 observations, respectively. Denote an observation of scan 1, 2, and 3 as i , j , and k , respectively. For example, i = 1, 2, …, M 1 . Use z ijk to represent the track formation hypothesis of O 1 i , O 2 j , and O 3 k . If the hypothesis is valid, then z ijk = 1; otherwise, z ijk = 0. As mentioned, c ijk is used to represent the cost of z ijk association. c ijk is 0 for false alarms and negative for possible associations. The S-D optimization problem can be formulated as:
J ( z ) = min i , j , k ∑ i = 0 M 1 ∑ j = 0 M 2 ∑ k = 0 M 3 c i j k z i j k
subject to three constraints:
∑ i = 0 M 1 ∑ j = 0 M 2 z i j k = 1 , ∀ k = 1 , 2 , … , M 3 ∑ i = 0 M 1 ∑ k = 0 M 3 z i j k = 1 , ∀ j = 1 , 2 , … , M 2 ∑ j = 0 M 2 ∑ k = 0 M 3 z i j k = 1 , ∀ i = 1 , 2 , … , M 1
The optimization function chooses associations to minimize the total cost. The three constraints ensure that each detection is accounted for (either included in an assignment or treated as false alarm).
The Lagrangian relaxation method approaches this 3-D assignment problem by relaxing the first constraint using Lagrange multipliers. Define a new function L ( λ ) :
L ( λ ) = ∑ k = 0 M 3 λ k [ ∑ i = 0 M 1 ∑ j = 0 M 2 z i j k − 1 ]
where λ k , k = 1, 2, …, M 3 are Lagrange multipliers. Subtract L from the original object function J ( z ) to get a new object function, and the first constraint in k is relaxed. Therefore, the 3-D assignment problem reduces to a 2-D assignment problem, which can be solved by any of the 2-D assignment method. For more details, see [1] .
The Lagrangian relaxation method allows the first constraint to be mildly violated, and therefore can only guarantee a suboptimal solution. For most applications, however, this is sufficient. To specify the solution accuracy, the method uses the solution gap, which defines the difference between the current solution and the potentially optimistic solution. The gap is nonnegative, and a smaller solution gap corresponds to a solution closer to the optimal solution.
Sensor Fusion and Tracking Toolbox provides assignsd to solve for S-D assignment using the Lagrangian relaxation method. Similar to the K best 2-D assignment solver assignkbest , the toolbox also provides a K best S-D assignment solver, assignkbestsd , which is used to provide multiple suboptimal solutions for an S-D assignment problem.
See Tracking Using Distributed Synchronous Passive Sensors for the application of S-D assignment in static detection fusion.
assignTOMHT | assignauction | assignjv | assignkbest | assignkbestsd | assignmunkres | assignsd | trackerGNN | trackerJPDA | trackerTOMHT
[1] Blackman, S., and R. Popoli. Design and Analysis of Modern Tracking Systems. Artech House Radar Library, Boston, 1999.
[2] Musicki, D., and R. Evans. "Joint Integrated Probabilistic Data Association: JIPDA." IEEE Transactions on Aerospace and Electronic Systems. Vol. 40, Number 3, 2004, pp 1093 –1099.
MATLAB Command
You clicked a link that corresponds to this MATLAB command:
Run the command by entering it in the MATLAB Command Window. Web browsers do not support MATLAB commands.
Select a Web Site
Choose a web site to get translated content where available and see local events and offers. Based on your location, we recommend that you select: .
- Switzerland (English)
- Switzerland (Deutsch)
- Switzerland (Français)
- 中国 (English)
You can also select a web site from the following list:
How to Get Best Site Performance
Select the China site (in Chinese or English) for best site performance. Other MathWorks country sites are not optimized for visits from your location.
- América Latina (Español)
- Canada (English)
- United States (English)
- Belgium (English)
- Denmark (English)
- Deutschland (Deutsch)
- España (Español)
- Finland (English)
- France (Français)
- Ireland (English)
- Italia (Italiano)
- Luxembourg (English)
- Netherlands (English)
- Norway (English)
- Österreich (Deutsch)
- Portugal (English)
- Sweden (English)
- United Kingdom (English)
Asia Pacific
- Australia (English)
- India (English)
- New Zealand (English)
Contact your local office
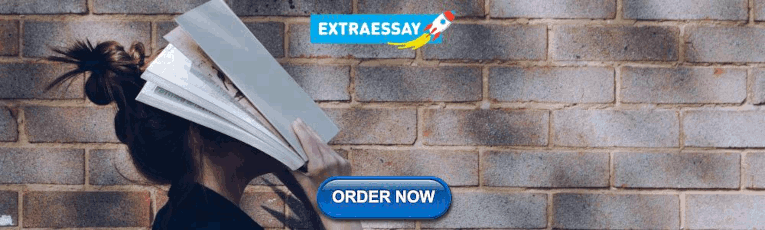
IMAGES
VIDEO
COMMENTS
The assignment problem is a fundamental combinatorial optimization problem. In its most general form, the problem is as follows: ... Usually the weight function is viewed as a square real-valued matrix C, ... Fortunately, there are many algorithms for finding the optimal assignment in time polynomial in n.
Kuhn gave the following algorithm for solving the optimal assignment problem in 1954. He called it the Hungarian method since it was inspired by Egerv ary's proof of Theorem 6.9. Suppose N is a network obtained from Km;m by giving each edge e an integer weight w(e). The algorithm iteratively constructs a
zero entries of the cost matrix are covered and the minimum number of such lines is used. Step 4. Test for Optimality: (i) If the minimum number of covering lines is n, an optimal assignment of zeros is possible and we are finished. (ii) If the minimum number of covering lines is less than n, an optimal assignment of zeros is not yet possible.
The Hungarian matching algorithm, also called the Kuhn-Munkres algorithm, is a \(O\big(|V|^3\big)\) algorithm that can be used to find maximum-weight matchings in bipartite graphs, which is sometimes called the assignment problem.A bipartite graph can easily be represented by an adjacency matrix, where the weights of edges are the entries.Thinking about the graph in terms of an adjacency ...
row and each column and obtain a new cost matrix C . By the rst observation, the optimal assignment for C is the same as the optimal assignment for C. Now, we use the maximum cardinality matching algorithm to check if there is a perfect matching that uses only edges of cost 0.
the optimal assignment problem as well as a novel method of projecting matrices into the doubly stochastic polytope while preserving the optimal assignment. The optimal assignment problem is a classical combinatorial optimisation problem that has fuelled extensive research in the last century.
The primal-dual algorithm described in [34] provides an optimal integer solution for the Assignment Problem. Besides this optimal assignment with the corresponding lower bound LB on the original problem, a reduced cost matrix c ¯ is obtained. Each c ¯ i j estimates the additional cost to be added to LB if variable next i takes the value j ...
Optimal Assignment Problem. Find the amount of electricity a company must send from its four power plants to five cities so as to maximize profit and minimize cost while meeting the cities' peak demands. ... Total[m, 2] gives the total of all elements of a matrix . The total profit made by the power company is , ...
The assignment problem is one of the fundamental combinatorial optimization problems in the branch of optimization or operations research in mathematics. In an ... Cost matrix C = [c ij] where c ij = cost of man i working on job j Variable x ij = 0 or 1. x ij = 1 if man i ...
The assignment problem arises when $ m = n $ and all $ a _ {i} $ and $ b _ {j} $ are $ 1 $. If all $ a _ {i} $ and $ b _ {j} $ in the transposed problem are integers, then there is an optimal solution for which all $ x _ {ij } $ are integers (Dantzig's theorem on integral solutions of the transport problem). In the assignment problem, for such ...
The Hungarian algorithm, aka Munkres assignment algorithm, utilizes the following theorem for polynomial runtime complexity (worst case O(n 3)) and guaranteed optimality: If a number is added to or subtracted from all of the entries of any one row or column of a cost matrix, then an optimal assignment for the resulting cost matrix is also an ...
The Hungarian method is a combinatorial optimization algorithm that solves the assignment problem in polynomial time and which anticipated later primal-dual methods.It was developed and published in 1955 by Harold Kuhn, who gave it the name "Hungarian method" because the algorithm was largely based on the earlier works of two Hungarian mathematicians, Dénes Kőnig and Jenő Egerváry.
The problem in this work will consider the case n=k. Mathematically stated the assignment problem is as follows: Let R be an n x n matrix with positive integer entries. The optimal assignment problem consists of choosing n entries, so that no two chosen entries come from the same row or column and such that the sum of the entries is maximized.
For the optimal solution = (), the calculation is as follows: = [] [] [] = [], (), = [] Looking at the figure of the optimal solution, the flow distance values are repeated in this matrix which is why the sum of all elements must be divided by 2. This gives , = so the minimum cost is 86.5.. Optimal Solution for the example with facility/location pairings listed.
The optimal assignment problem of network flow theory can be stated as follows: given an n × n matrix A = (a 0 of real numbers, find the location of n matrix elements with minimum sum under the proviso that no two elements are in the same row or column. More formally this can be expressed as finding the ...
Abstract. In this chapter we introduce the notion of a "pattern" in the Linear Assignment Problem and show that patterns may be useful to create new insights and approaches for many combinatorial optimization problems defined on a rectangular input matrix. We define a pattern as a specific collection of cells in the rectangular matrix ...
Step 1: Set up the cost matrix. The first step in solving the assignment problem is to set up the cost matrix, which represents the cost of assigning a task to an agent. The matrix should be square and have the same number of rows and columns as the number of tasks and agents, respectively.
optimal assignment problems. This parallel preprocessing allows one to delete entries which do not be-long to optimal permutations, leading to a reduced instance which becomes solvable with limited memory requirements. Keywords: large scale optimal assignment problem; entropy maximization; matrix diagonal scaling; par-
The assignment problem can be solved by the following four methods: a) Complete enumeration method. b) Simplex Method. c) Transportation method. d) Hungarian method. 9.2.1 Complete enumeration method. In this method, a list of all possible assignments among the given resources and activities is prepared.
The optimal assignment schedule and total cost is. The optimal assignment (minimum) cost = ₹ 38. Example 10.8. Consider the problem of assigning five jobs to five persons. The assignment costs are given as follows. ... The cost matrix of the given assignment problem is. Column 3 contains no zero. Go to Step 2.
The Hungarian Algorithm Calculator is a powerful tool used to solve optimization problems known as the assignment problem. It finds the optimal assignment of tasks to resources, minimizing the total cost or maximizing the total profit. ... A step-by-step procedure for solving a problem. Matrix: A rectangular array of numbers arranged in rows ...
Unbalanced assignment problem, Hungarian method, Optimal solution. Introduction The assignment problem is a combinatorial optimization problem in the ... Such problems can be termed as unbalanced assignment problem. The effectiveness matrix in this case will not be a square matrix. In such problems, dummy row(s) or dummy column(s) are added in ...
For an S-D assignment problem, the optimal solution may not be obtainable in practice. 2-D Assignment in Multiple Target Tracking. In the 2-D MTT assignment problem, a tracker tries to assign multiple tracks to multiple detections. ... Given the cost matrix element C ij, find an assignment Z = {z ij} that minimizes . J = ∑ i = 0 n ∑ j = 0 m ...