- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
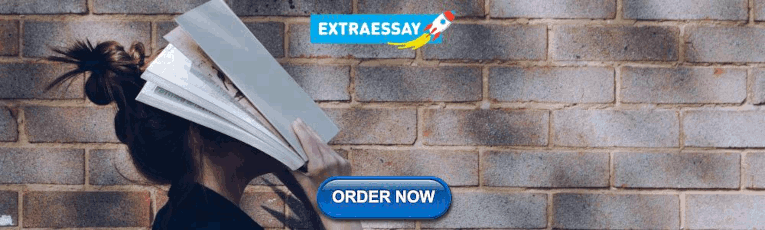
UnboundLocalError Local variable Referenced Before Assignment in Python
Handling errors is an integral part of writing robust and reliable Python code. One common stumbling block that developers often encounter is the “UnboundLocalError” raised within a try-except block. This error can be perplexing for those unfamiliar with its nuances but fear not â in this article, we will delve into the intricacies of the UnboundLocalError and provide a comprehensive guide on how to effectively use try-except statements to resolve it.
What is UnboundLocalError Local variable Referenced Before Assignment in Python?
The UnboundLocalError occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Why does UnboundLocalError: Local variable Referenced Before Assignment Occur?
below, are the reasons of occurring “Unboundlocalerror: Try Except Statements” in Python :
Variable Assignment Inside Try Block
Reassigning a global variable inside except block.
- Accessing a Variable Defined Inside an If Block
In the below code, example_function attempts to execute some_operation within a try-except block. If an exception occurs, it prints an error message. However, if no exception occurs, it prints the value of the variable result outside the try block, leading to an UnboundLocalError since result might not be defined if an exception was caught.
In below code , modify_global function attempts to increment the global variable global_var within a try block, but it raises an UnboundLocalError. This error occurs because the function treats global_var as a local variable due to the assignment operation within the try block.
Solution for UnboundLocalError Local variable Referenced Before Assignment
Below, are the approaches to solve “Unboundlocalerror: Try Except Statements”.
Initialize Variables Outside the Try Block
Avoid reassignment of global variables.
In modification to the example_function is correct. Initializing the variable result before the try block ensures that it exists even if an exception occurs within the try block. This helps prevent UnboundLocalError when trying to access result in the print statement outside the try block.
Below, code calculates a new value ( local_var ) based on the global variable and then prints both the local and global variables separately. It demonstrates that the global variable is accessed directly without being reassigned within the function.
In conclusion , To fix “UnboundLocalError” related to try-except statements, ensure that variables used within the try block are initialized before the try block starts. This can be achieved by declaring the variables with default values or assigning them None outside the try block. Additionally, when modifying global variables within a try block, use the `global` keyword to explicitly declare them.
Please Login to comment...
Similar reads.
- Python Errors
- Python Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Local variable referenced before assignment in Python
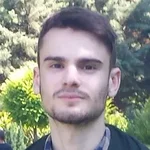
Last updated: Apr 8, 2024 Reading time ¡ 4 min
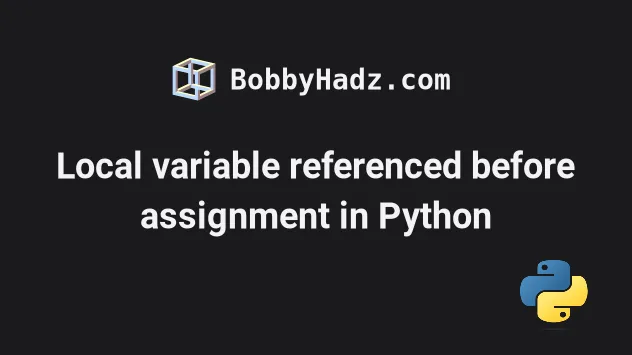
# Local variable referenced before assignment in Python
The Python "UnboundLocalError: Local variable referenced before assignment" occurs when we reference a local variable before assigning a value to it in a function.
To solve the error, mark the variable as global in the function definition, e.g. global my_var .
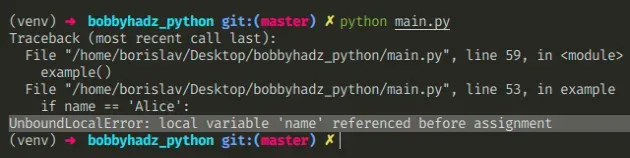
Here is an example of how the error occurs.
We assign a value to the name variable in the function.
# Mark the variable as global to solve the error
To solve the error, mark the variable as global in your function definition.
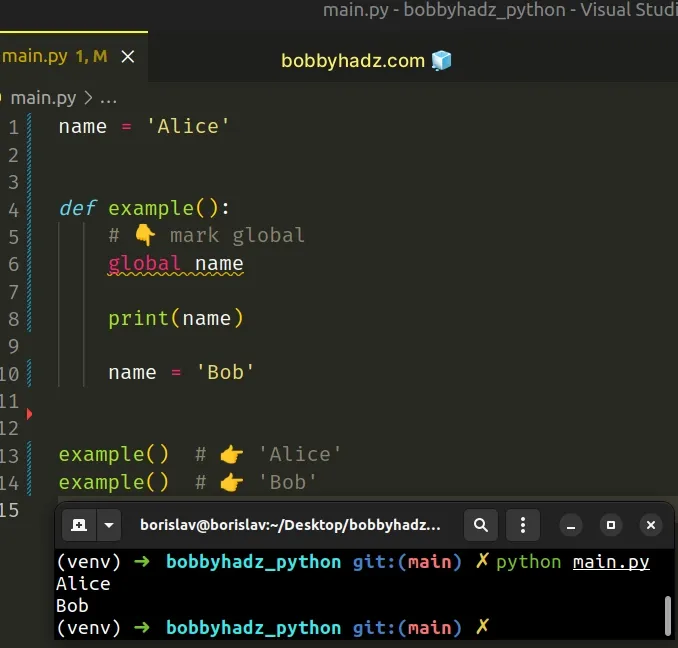
If a variable is assigned a value in a function's body, it is a local variable unless explicitly declared as global .
# Local variables shadow global ones with the same name
You could reference the global name variable from inside the function but if you assign a value to the variable in the function's body, the local variable shadows the global one.
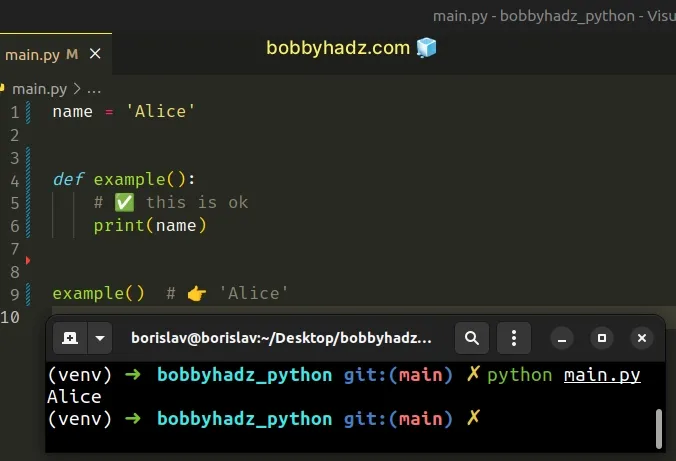
Accessing the name variable in the function is perfectly fine.
On the other hand, variables declared in a function cannot be accessed from the global scope.
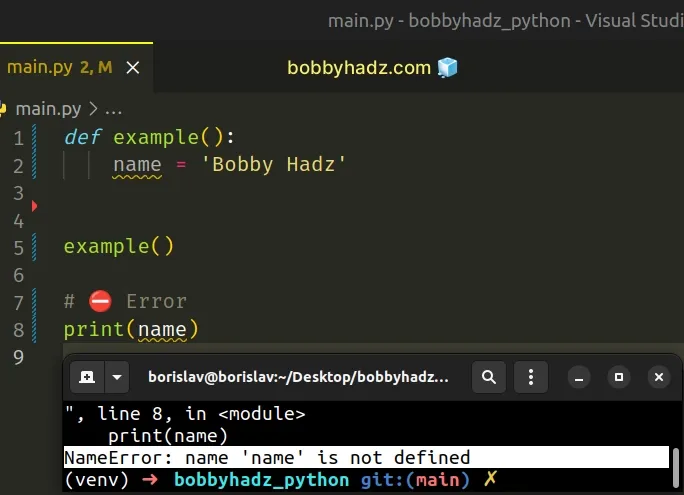
The name variable is declared in the function, so trying to access it from outside causes an error.
Make sure you don't try to access the variable before using the global keyword, otherwise, you'd get the SyntaxError: name 'X' is used prior to global declaration error.
# Returning a value from the function instead
An alternative solution to using the global keyword is to return a value from the function and use the value to reassign the global variable.
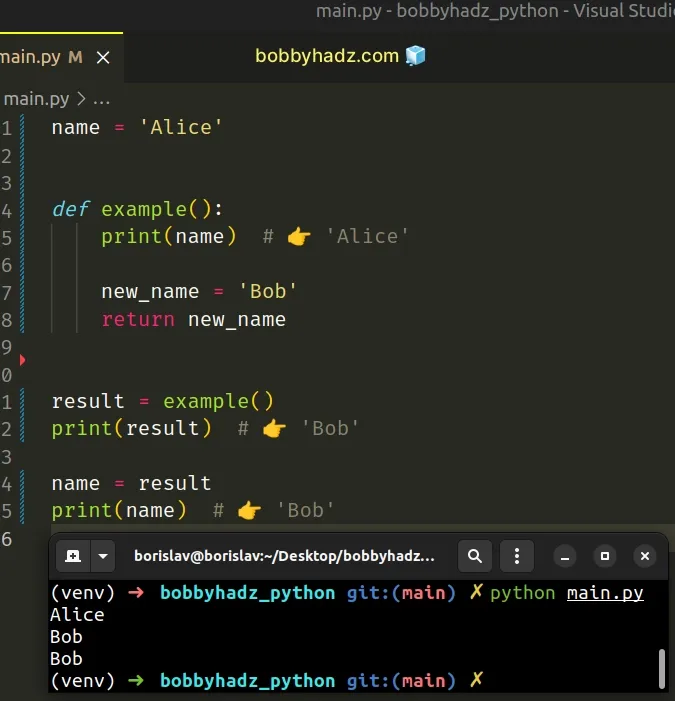
We simply return the value that we eventually use to assign to the name global variable.
# Passing the global variable as an argument to the function
You should also consider passing the global variable as an argument to the function.
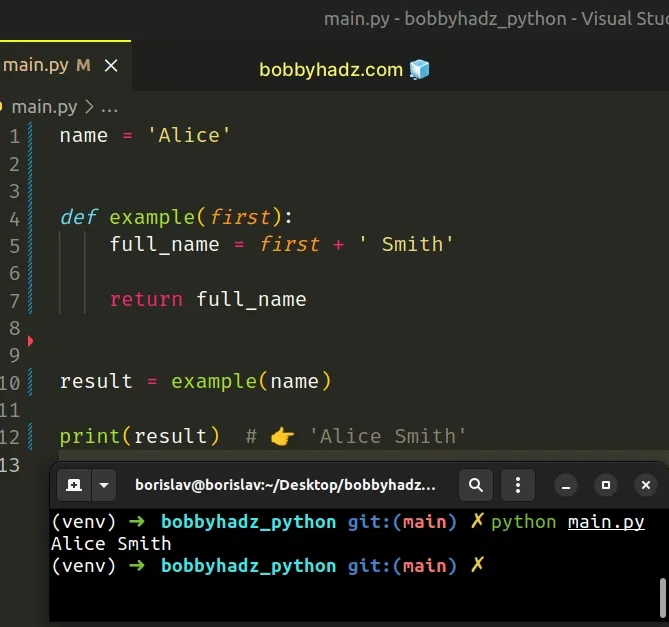
We passed the name global variable as an argument to the function.
If we assign a value to a variable in a function, the variable is assumed to be local unless explicitly declared as global .
# Assigning a value to a local variable from an outer scope
If you have a nested function and are trying to assign a value to the local variables from the outer function, use the nonlocal keyword.
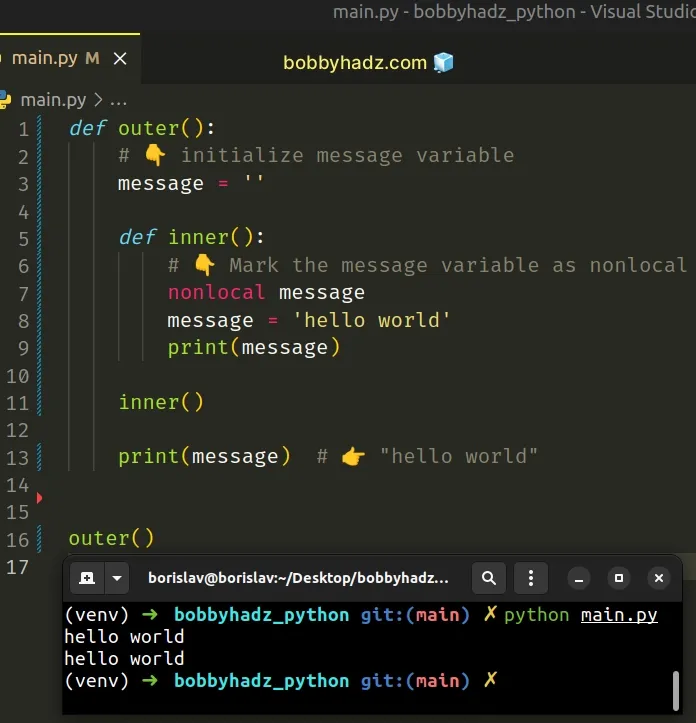
The nonlocal keyword allows us to work with the local variables of enclosing functions.
Had we not used the nonlocal statement, the call to the print() function would have returned an empty string.
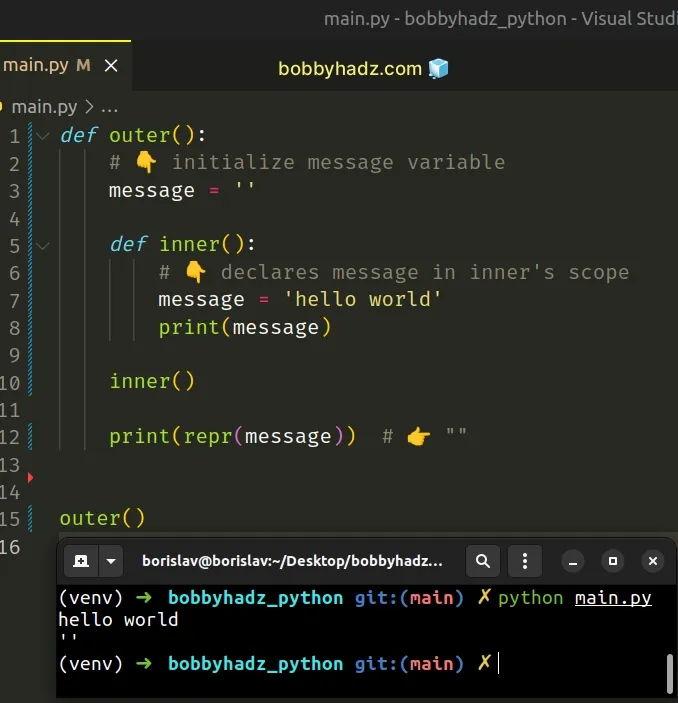
Printing the message variable on the last line of the function shows an empty string because the inner() function has its own scope.
Changing the value of the variable in the inner scope is not possible unless we use the nonlocal keyword.
Instead, the message variable in the inner function simply shadows the variable with the same name from the outer scope.
# Discussion
As shown in this section of the documentation, when you assign a value to a variable inside a function, the variable:
- Becomes local to the scope.
- Shadows any variables from the outer scope that have the same name.
The last line in the example function assigns a value to the name variable, marking it as a local variable and shadowing the name variable from the outer scope.
At the time the print(name) line runs, the name variable is not yet initialized, which causes the error.
The most intuitive way to solve the error is to use the global keyword.
The global keyword is used to indicate to Python that we are actually modifying the value of the name variable from the outer scope.
- If a variable is only referenced inside a function, it is implicitly global.
- If a variable is assigned a value inside a function's body, it is assumed to be local, unless explicitly marked as global .
If you want to read more about why this error occurs, check out [this section] ( this section ) of the docs.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: name 'X' is used prior to global declaration
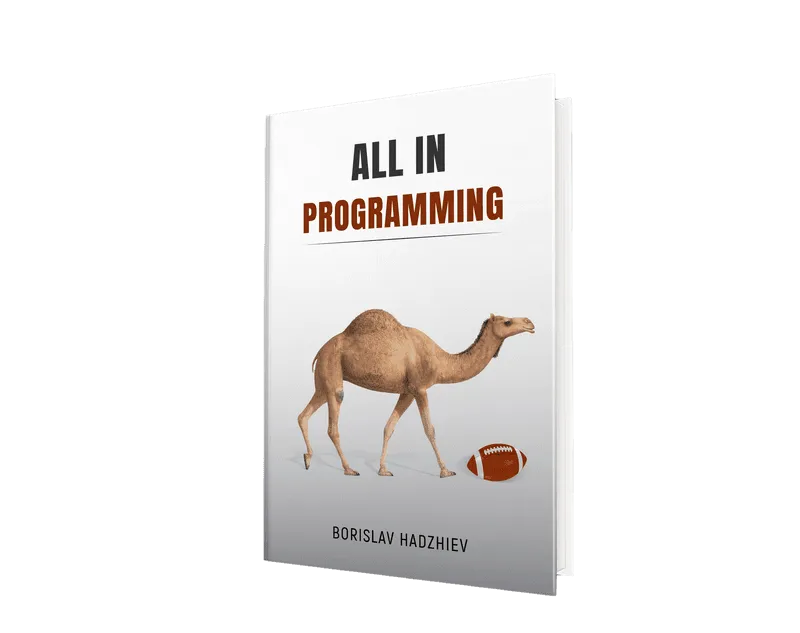
Borislav Hadzhiev
Web Developer
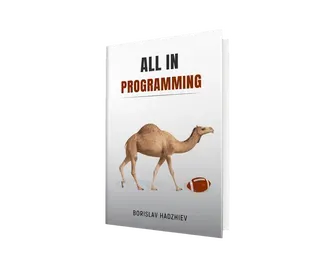
Copyright Š 2024 Borislav Hadzhiev
How to fix UnboundLocalError: local variable 'x' referenced before assignment in Python
You could also see this error when you forget to pass the variable as an argument to your function.
How to reproduce this error
How to fix this error.
I hope this tutorial is useful. See you in other tutorials.
Take your skills to the next level âĄď¸
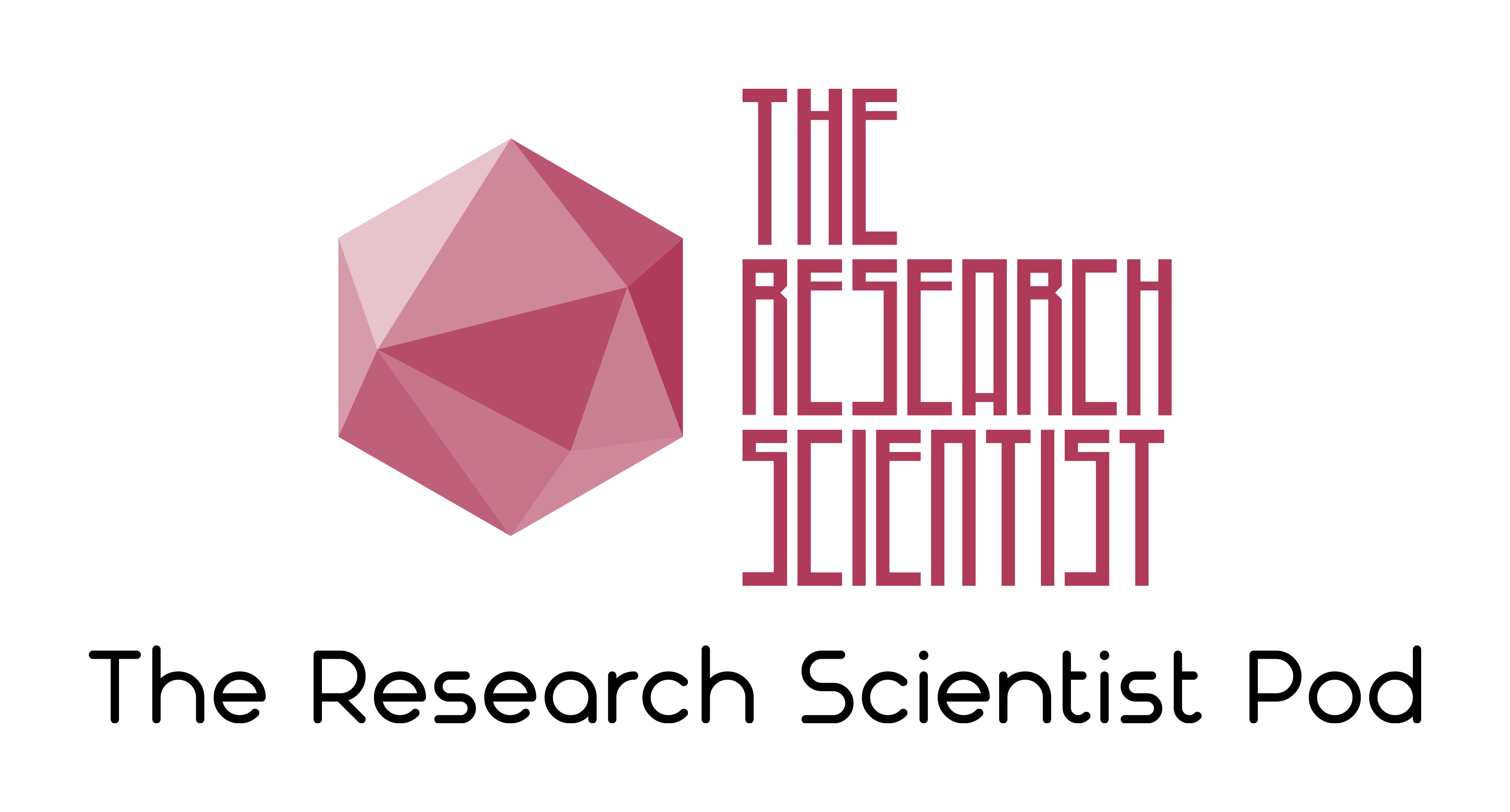
Python UnboundLocalError: local variable referenced before assignment
by Suf | Programming , Python , Tips
If you try to reference a local variable before assigning a value to it within the body of a function, you will encounter the UnboundLocalError: local variable referenced before assignment.
The preferable way to solve this error is to pass parameters to your function, for example:
Alternatively, you can declare the variable as global to access it while inside a function. For example,
This tutorial will go through the error in detail and how to solve it with code examples .
Table of contents
What is scope in python, unboundlocalerror: local variable referenced before assignment, solution #1: passing parameters to the function, solution #2: use global keyword, solution #1: include else statement, solution #2: use global keyword.
Scope refers to a variable being only available inside the region where it was created. A variable created inside a function belongs to the local scope of that function, and we can only use that variable inside that function.
A variable created in the main body of the Python code is a global variable and belongs to the global scope. Global variables are available within any scope, global and local.
UnboundLocalError occurs when we try to modify a variable defined as local before creating it. If we only need to read a variable within a function, we can do so without using the global keyword. Consider the following example that demonstrates a variable var created with global scope and accessed from test_func :
If we try to assign a value to var within test_func , the Python interpreter will raise the UnboundLocalError:
This error occurs because when we make an assignment to a variable in a scope, that variable becomes local to that scope and overrides any variable with the same name in the global or outer scope.
var +=1 is similar to var = var + 1 , therefore the Python interpreter should first read var , perform the addition and assign the value back to var .
var is a variable local to test_func , so the variable is read or referenced before we have assigned it. As a result, the Python interpreter raises the UnboundLocalError.
Example #1: Accessing a Local Variable
Let’s look at an example where we define a global variable number. We will use the increment_func to increase the numerical value of number by 1.
Let’s run the code to see what happens:
The error occurs because we tried to read a local variable before assigning a value to it.
We can solve this error by passing a parameter to increment_func . This solution is the preferred approach. Typically Python developers avoid declaring global variables unless they are necessary. Let’s look at the revised code:
We have assigned a value to number and passed it to the increment_func , which will resolve the UnboundLocalError. Let’s run the code to see the result:
We successfully printed the value to the console.
We also can solve this error by using the global keyword. The global statement tells the Python interpreter that inside increment_func , the variable number is a global variable even if we assign to it in increment_func . Let’s look at the revised code:
Let’s run the code to see the result:
Example #2: Function with if-elif statements
Let’s look at an example where we collect a score from a player of a game to rank their level of expertise. The variable we will use is called score and the calculate_level function takes in score as a parameter and returns a string containing the player’s level .
In the above code, we have a series of if-elif statements for assigning a string to the level variable. Let’s run the code to see what happens:
The error occurs because we input a score equal to 40 . The conditional statements in the function do not account for a value below 55 , therefore when we call the calculate_level function, Python will attempt to return level without any value assigned to it.
We can solve this error by completing the set of conditions with an else statement. The else statement will provide an assignment to level for all scores lower than 55 . Let’s look at the revised code:
In the above code, all scores below 55 are given the beginner level. Let’s run the code to see what happens:
We can also create a global variable level and then use the global keyword inside calculate_level . Using the global keyword will ensure that the variable is available in the local scope of the calculate_level function. Let’s look at the revised code.
In the above code, we put the global statement inside the function and at the beginning. Note that the “default” value of level is beginner and we do not include the else statement in the function. Let’s run the code to see the result:
Congratulations on reading to the end of this tutorial! The UnboundLocalError: local variable referenced before assignment occurs when you try to reference a local variable before assigning a value to it. Preferably, you can solve this error by passing parameters to your function. Alternatively, you can use the global keyword.
If you have if-elif statements in your code where you assign a value to a local variable and do not account for all outcomes, you may encounter this error. In which case, you must include an else statement to account for the missing outcome.
For further reading on Python code blocks and structure, go to the article: How to Solve Python IndentationError: unindent does not match any outer indentation level .
Go to the online courses page on Python to learn more about Python for data science and machine learning.
Have fun and happy researching!
Share this:
- Click to share on Facebook (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Telegram (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Tumblr (Opens in new window)
[SOLVED] Local Variable Referenced Before Assignment
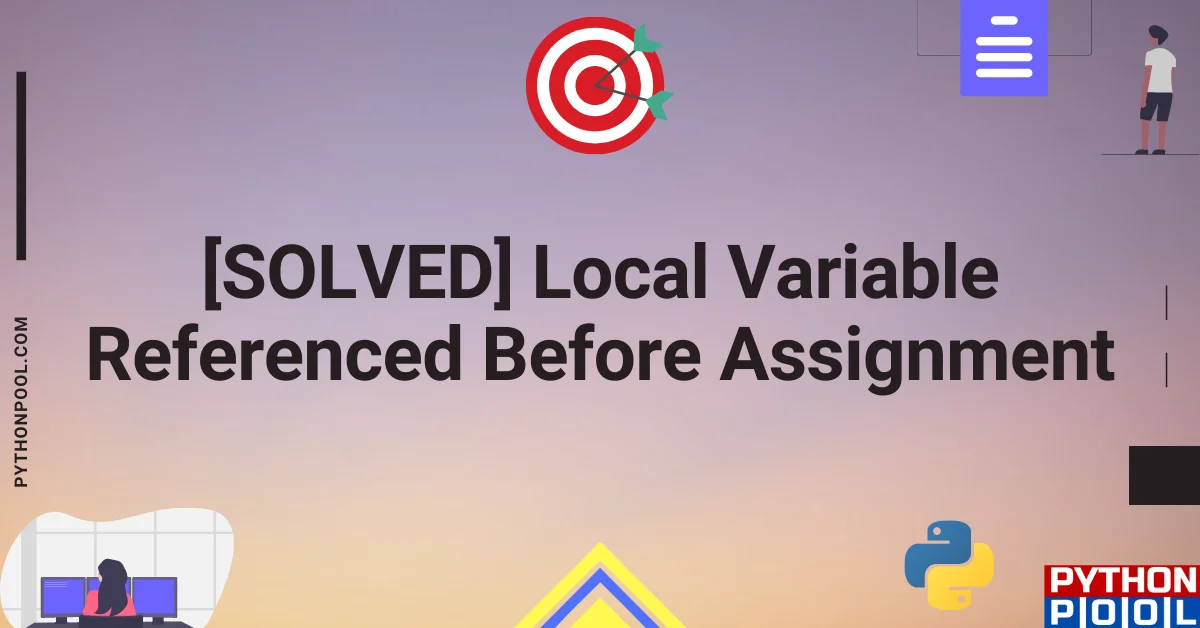
Python treats variables referenced only inside a function as global variables. Any variable assigned to a function’s body is assumed to be a local variable unless explicitly declared as global.
Why Does This Error Occur?
Unboundlocalerror: local variable referenced before assignment occurs when a variable is used before its created. Python does not have the concept of variable declarations. Hence it searches for the variable whenever used. When not found, it throws the error.
Before we hop into the solutions, let’s have a look at what is the global and local variables.
Local Variable Declarations vs. Global Variable Declarations
Local Variables | Global Variables |
---|---|
A variable is declared primarily within a Python function. | Global variables are in the global scope, outside a function. |
A local variable is created when the function is called and destroyed when the execution is finished. | A Variable is created upon execution and exists in memory till the program stops. |
Local Variables can only be accessed within their own function. | All functions of the program can access global variables. |
Local variables are immune to changes in the global scope. Thereby being more secure. | Global Variables are less safer from manipulation as they are accessible in the global scope. |
![unboundlocalerror local variable 'xxx' referenced before assignment [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
Local Variable Referenced Before Assignment Error with Explanation
Try these examples yourself using our Online Compiler.
Let’s look at the following function:

Explanation
The variable myVar has been assigned a value twice. Once before the declaration of myFunction and within myFunction itself.
Using Global Variables
Passing the variable as global allows the function to recognize the variable outside the function.
Create Functions that Take in Parameters
Instead of initializing myVar as a global or local variable, it can be passed to the function as a parameter. This removes the need to create a variable in memory.
UnboundLocalError: local variable ‘DISTRO_NAME’
This error may occur when trying to launch the Anaconda Navigator in Linux Systems.
Upon launching Anaconda Navigator, the opening screen freezes and doesn’t proceed to load.
Try and update your Anaconda Navigator with the following command.
If solution one doesn’t work, you have to edit a file located at
After finding and opening the Python file, make the following changes:
In the function on line 159, simply add the line:
DISTRO_NAME = None
Save the file and re-launch Anaconda Navigator.
DJANGO – Local Variable Referenced Before Assignment [Form]
The program takes information from a form filled out by a user. Accordingly, an email is sent using the information.
Upon running you get the following error:
We have created a class myForm that creates instances of Django forms. It extracts the user’s name, email, and message to be sent.
A function GetContact is created to use the information from the Django form and produce an email. It takes one request parameter. Prior to sending the email, the function verifies the validity of the form. Upon True , .get() function is passed to fetch the name, email, and message. Finally, the email sent via the send_mail function
Why does the error occur?
We are initializing form under the if request.method == “POST” condition statement. Using the GET request, our variable form doesn’t get defined.
Local variable Referenced before assignment but it is global
This is a common error that happens when we don’t provide a value to a variable and reference it. This can happen with local variables. Global variables can’t be assigned.
This error message is raised when a variable is referenced before it has been assigned a value within the local scope of a function, even though it is a global variable.
Here’s an example to help illustrate the problem:
In this example, x is a global variable that is defined outside of the function my_func(). However, when we try to print the value of x inside the function, we get a UnboundLocalError with the message “local variable ‘x’ referenced before assignment”.
This is because the += operator implicitly creates a local variable within the function’s scope, which shadows the global variable of the same name. Since we’re trying to access the value of x before it’s been assigned a value within the local scope, the interpreter raises an error.
To fix this, you can use the global keyword to explicitly refer to the global variable within the function’s scope:
However, in the above example, the global keyword tells Python that we want to modify the value of the global variable x, rather than creating a new local variable. This allows us to access and modify the global variable within the function’s scope, without causing any errors.
Local variable ‘version’ referenced before assignment ubuntu-drivers
This error occurs with Ubuntu version drivers. To solve this error, you can re-specify the version information and give a split as 2 –
Here, p_name means package name.
With the help of the threading module, you can avoid using global variables in multi-threading. Make sure you lock and release your threads correctly to avoid the race condition.
When a variable that is created locally is called before assigning, it results in Unbound Local Error in Python. The interpreter can’t track the variable.
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
Trending Python Articles
![unboundlocalerror local variable 'xxx' referenced before assignment [Fixed] nameerror: name Unicode is not defined](https://www.pythonpool.com/wp-content/uploads/2024/01/Fixed-nameerror-name-Unicode-is-not-defined-300x157.webp)
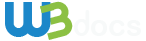
- Password Generator
- HTML Editor
- HTML Encoder
- JSON Beautifier
- CSS Beautifier
- Markdown Convertor
- Find the Closest Tailwind CSS Color
- Phrase encrypt / decrypt
- Browser Feature Detection
- Number convertor
- CSS Maker text shadow
- CSS Maker Text Rotation
- CSS Maker Out Line
- CSS Maker RGB Shadow
- CSS Maker Transform
- CSS Maker Font Face
- Color Picker
- Colors CMYK
- Color mixer
- Color Converter
- Color Contrast Analyzer
- Color Gradient
- String Length Calculator
- MD5 Hash Generator
- Sha256 Hash Generator
- String Reverse
- URL Encoder
- URL Decoder
- Base 64 Encoder
- Base 64 Decoder
- Extra Spaces Remover
- String to Lowercase
- String to Uppercase
- Word Count Calculator
- Empty Lines Remover
- HTML Tags Remover
- Binary to Hex
- Hex to Binary
- Rot13 Transform on a String
- String to Binary
- Duplicate Lines Remover
Python 3: UnboundLocalError: local variable referenced before assignment
This error occurs when you are trying to access a variable before it has been assigned a value. Here is an example of a code snippet that would raise this error:
Watch a video course Python - The Practical Guide
The error message will be:
In this example, the variable x is being accessed before it is assigned a value, which is causing the error. To fix this, you can either move the assignment of the variable x before the print statement, or give it an initial value before the print statement.
Both will work without any error.
Related Resources
- Using global variables in a function
- "Least Astonishment" and the Mutable Default Argument
- Why is "1000000000000000 in range(1000000000000001)" so fast in Python 3?
- HTML Basics
- Javascript Basics
- TypeScript Basics
- React Basics
- Angular Basics
- Sass Basics
- Vue.js Basics
- Python Basics
- Java Basics
- NodeJS Basics
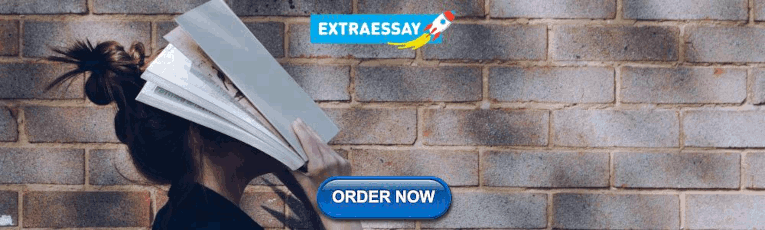
UnboundLocalError: local variable 'values' referenced before assignment in lr_scheduler
This my code:

how to solve this error?
Could you post a code snippet to reproduce this issue, please? This dummy example runs fine:
Need any other snippet code? Iâm not sure how much complete code you need
A minimal and executable code snippet would be great. Could you try to remove unnecessary functions and use some random inputs, so that we can reproduce this issue locally?
Iâm sorry for my slow responseăThis code might suit your needsă

You can see that the first call to scheduler. Step should have been fault-free because it printed the following print statement, as well as eval for the model. But an error should have occurred on the second call
I forgot the code that came out and this is the following code
Now it turns out that if you use annotated code, you get an error, while unannotated code works fine
I really donât know why, is it the data problem that caused the error
Thanks for the code so far. Could you also post the code you are using to initialize the model, optimizer, and scheduler? Also, could you try to run the code on the CPU only and check, if you see the same error? If not, could you rerun the GPU code using CUDA_LAUNCH_BLOCKING=1 python script.py args and post the stack trace again?
This is my code:
It was very embarrassing that I could not debug with CPU because of the machine, but if I used GPU to debug, the error result did not change
Could you call scheudler.get_lr() before the error is thrown and check the return value, please?
I am very sorry for replying to you a few days later, because I am a sophomore student in university and I have a lot of things to do recently, so I didnât deal with this problem for a few days. As you requested, I added this line of code. The problem is that it returned the value successfully without any problems during the first epoch. But after the second epoch, it reported an error
Are you recreating or manipulating the scheduler or optimizer in each epoch somehow?
29/5000 Thank you for answering my question so patiently. My code should not have this problemă If I use following code,the error will not appear( it will appear when using the annotating code):
Here is my complete training code(When you put scheduler. Step () into each batch iteration,error will apear)ďź
I had the same error that I think has been fixed.
In the end it seems like the number of epochs you had mentioned in your scheduler was less than the number of epochs you tried training for. I went into %debug in the notebook and tried calling self.get_lr() as suggested. I got this message: *** ValueError: Tried to step 3752 times. The specified number of total steps is 3750
Then with some basic math and a lot of code search I realised that I had specified 5 epochs in my scheduler but called for 10 epochs in my fit function.
Hope this helps.
There is an error with total_steps.i am also getting same error but i rectified it
Python has lexical scoping by default, which means that although an enclosed scope can access values in its enclosing scope, it cannot modify them (unless theyâre declared global with the global keyword). A closure binds values in the enclosing environment to names in the local environment. The local environment can then use the bound value, and even reassign that name to something else, but it canât modify the binding in the enclosing environment. UnboundLocalError happend because when python sees an assignment inside a function then it considers that variable as local variable and will not fetch its value from enclosing or global scope when we execute the function. To modify a global variable inside a function, you must use the global keyword.
Hello,I had the same error that I canât solve it. I want to ask you some question about it.Thank you. What is the âThe specified number of total steps is 3750â? How to change the number of steps? Thank you.
Hi make sure that your dataloader and the scheduler have the same number of iterations. If I remember correctly I got this error when using the OneCycle LR scheduler which needs you to specify the max number of steps as init parameter. Hope this helps! If this isnât the error you have, then please provide code and try to see what your scheduler.get_lr() method returns.
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
UnboundLocalError: local variable referenced before assignment
I have following simple function to get percent values for different cover types from a raster. It gives me following error: UnboundLocalError: local variable 'a' referenced before assignment
which isn't clear to me. Any suggestions?
- arcgis-10.1
- unboundlocalerror
- 1 Because if row.getValue("Value") == 1 might be false and so a never gets assigned. – Nathan W Commented May 20, 2013 at 2:39
- It has value and do gets assigned. I checked it in arcmap interactive python window but can't get it to work in a stand alone script. – Ibe Commented May 20, 2013 at 2:44
- 1 your loop will also only give you the values of the last loop iteration as you are returning out of the loop and not doing anything with each value. – Nathan W Commented May 20, 2013 at 2:49
- You could use 3 x elif and an else to see if any values other than 1-4 are encountered. – PolyGeo ♦ Commented May 20, 2013 at 3:44
- I tried that way as well but still hung up with error. – Ibe Commented May 20, 2013 at 4:15
This error is pretty much explained here and it helped me to get assignments and return values for all variables.
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking âPost Your Answerâ, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged arcpy arcgis-10.1 unboundlocalerror or ask your own question .
- The Overflow Blog
- How to build open source apps in a highly regulated industry
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests â hereâs how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- What are these courtesy names and given names? - confusion in translation
- lme4 Inconsistency
- Why is a game's minor update (e.g., New World) ~15 GB to download?
- Are there examples of triple entendres in English?
- Do Christians believe that Jews and Muslims go to hell?
- Are there conditions for an elliptic Fq-curve to have a quadratic Fq-cover of the line without ramification Fq-points?
- Is there a drawback to using Heart's blood rote repeatedly?
- Is it correct to say "glide my fingernail on the adhesive tape to feel its rim"?
- Inversion naming conventions
- Equivariant Smith normal form?
- Geometry question about a six-pack of beer
- Create a phone-book using LaTeX
- 224.0.0.0/4 In IPv4 Routing Table
- Why is Uranus colder than Neptune?
- Book that I read around 1975, where the main character is a retired space pilot hired to steal an object from a lab called Menlo Park
- What does a letter "R" means in a helipad?
- E flat tuning - adjust bass?
- Help identifying hollow spring / inverted header sockets
- Position where last x halfmoves are determined
- How to NDSolve a PDE that contains the integral of the solution?
- Unsorted Intersection
- Is anything implied by the author using the (Greek) phrase translated "work with your hands" in 1 Thessalonians 4:11?
- Dagesh on final letter kaf
- Boundary Conditions on the Inlet and Outlet in a Discontinuous Galerkin framework
Get the Reddit app
Subreddit for posting questions and asking for general advice about your python code.
having a problem with error code: UnboundLocalError: local variable 'player' referenced before assignment
hi hoping someone here can actually help me. I asked this question on stack overflow and they closed it and redirected to similar previously asked questions. none of which actually helped me to understand how to correct the error code.
I am following a video tutorial series for learning to code with python/pygame. and i have checked my coding aginst the source material and they are the same, because i typed it as was told to in the video.
here's the full tracebackback including the error:
File "/home/dev/PycharmProjects/game7_meteor_game/game7_meteor_game.py", line 160, in <module>
File "/home/dev/PycharmProjects/game7_meteor_game/game7_meteor_game.py", line 152, in main
GameInterface(num_player=1, screen=screen)
File "/home/dev/PycharmProjects/game7_meteor_game/game7_meteor_game.py", line 82, in GameInterface
if player.cooling_time > 0:
UnboundLocalError: local variable 'player' referenced before assignment.
here's the actual code:
if player.cooling_time > 0: player.cooling_time -= 1
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . Weâll occasionally send you account related emails.
Already on GitHub? Sign in to your account
UnboundLocalError: local variable 'boxprops' referenced before assignment #3647
catskillsresearch commented Mar 3, 2024
On Python 3.9.7, with a fresh pip install seaborn, at line 1373 of , with this call: I get this error: |
The text was updated successfully, but these errors were encountered: |
mwaskom commented Mar 3, 2024
Please provide a reproducible example, thank! |
Sorry, something went wrong.
catskillsresearch commented Mar 3, 2024 • edited Loading
There is a for loop in the routine in categorical.py. The variable boxprops is set for various cases inside the for loop. It is undefined before the loop and used afterwards. It just doesn't imagine the case where there is no data and the for loop has 0 iterations. Setting boxprops = None before the start of the loop cures the problem. |
A reproducible example is code that can be copy-pasted in toto to demonstrate the problem. Thanks! |
|
catskillsresearch commented Mar 4, 2024
It is hard to reproduce the exact context. If you add on line 630 of categorical.py, the problem I experienced will not recur. If for any reason self.iter_vars is empty for a given application, this problem will be experienced by other users, without the addition of that line, for the reasons I described above. |
mwaskom commented Mar 4, 2024
I donât understand why it is so hard to just show the actual code you are using. Even if your suggested change is correct, we will still want to add a test for the edge case, and that requires understanding the circumstances where it arises. |
- đ 1 reaction
jhncls commented Mar 7, 2024
: The external file you link to has too many dependencies to try to run it here. And even then, we don't know which input has been used. It would help if you'd inspect the values and datatypes of , , and , and based on those try to create a stand-alone example. Can you verify whether my tests below coincide with your case? : Trying to reproduce, I can only see the crash with .boxplot(data=[None, None], palette=['r', 'b'])This gives a warning: without assigning is deprecated and will be removed in v0.14.0. Assign the variable to and set for the same effect.and then an error UnboundLocalError: cannot access local variable 'boxprops' where it is not associated with a value Doing the same without gives another error: .boxplot(data=[None, None]) values must have same the lengthReplacing with empty lists or an empty dataframe doesn't give an error (just an empty plot, as I would expect). I've tested with Seaborn 0.13.2 and with the latest dev version. Both behave the same. Maybe, instead of adding an extra , the function should exit earlier? Adding might just postpone the crash. Anyway, this doesn't look like a high-priority issue. |
mwaskom commented Mar 7, 2024
Thanks for looking into it ! It would also be very helpful to know what is in the original report... |
Testing with different guesses for didn't make a difference. Hopefully OP can shed a light here. Testing with other functions, it seems only crashes on . Other functions just give empty plots. When also is set, there is a warning about not being set. But they do take the palette into account with e.g. (without complaining about the missing hue). By the way, gives a "correct" plot. I am really amazed by the wide range of input types seaborn is handling. A limited list of extravagant inputs that crash (and only with ) ) crashes with values must have same the length" |
nicholasdehnen commented Mar 27, 2024 • edited Loading
Not sure if exactly the same, but I ran into this issue while trying to adhere to the generated by this code: numpy as np, pandas as pd, seaborn as sns cat_color = ['Black', 'Brown', 'Orange'] mu, sigma = [1.0, 2.5, 4.0], [0.5, 0.75, 0.5] cat_silliness = np.random.normal(mu, sigma, (100, 3)) df = pd.DataFrame(columns=cat_color, data=cat_silliness) melted = df.melt(var_name='CatColor', value_name='Silliness') sns.boxplot(x='CatColor', y='Silliness', data=melted, showfliers=False, palette='tab10', order=cat_color[::-1], legend=False) without assigning is deprecated and will be removed in v0.14.0. Assign the variable to and set for the same effect.Simply replacing with raises the exact same error as posted by the OP: This is technically a user error since I failed to replace with , but that wasn't immediately obvious to me. Maybe should be ignored in case no value is passed? |
MischaelR commented Apr 18, 2024
I was stuck with this error for a long time but I am not sure if it is the same cause. In essence, this error popped up when listing an invalid order. See For this particular example, I think a more descriptive error message would be beneficial.
|
EkaterinaAbramova commented May 24, 2024
Hi there, I can't seem to run this function: Im getting an error: Ive tried to use GPT4 to help resolve it and tried many things but it's not working. Could you please advise what to do? |
jhncls commented May 25, 2024
Hi Ekaterina, |
EkaterinaAbramova commented May 25, 2024
I am confused as to why you say my post is unrelated to this thread. The thread title and my error are exactly the same: "UnboundLocalError: local variable 'boxprops' referenced before assignment". The % issue you are referring to is a warning not an error. If you add boxprops = None on line 630 of categorical.py, the problem I experienced will not recur as per above comment, however it still doesn't plot anything. I am expecting plots as per this post: . Could you help? The data I have are attached here. |
nicholasdehnen commented May 25, 2024
. Could you help? The data I have are attached here. If you take a look at your stack trace, you should see that the (initial) call that caused the problem was in . Assuming you didn't modify that library, you should probably report an issue in the corresponding repository, since your error arises from the way seaborn is called by pyfolio. That's nothing can change, and the reason why your post is unrelated. However, if you look right above your first comment here, you will see a mention of this issue in a pyfolio pull request ( ). That pull request addresses your exact problem, so updating your pyfolio to version 0.9.7 might help. |
EkaterinaAbramova commented Jun 4, 2024
Thank you for helping me, updating to 0.9.7 solved my problem! Much appreciated |
- đ 1 reaction
rheagr commented Jun 24, 2024
I've tried to update pyfolio, however I get: |
No branches or pull requests
UnboundLocalError in IMDiffusion: Handling Local Variable References
Abstract: In this article, we will discuss how to handle UnboundLocalError exceptions in IMDiffusion, a Python-based diffusion model.
UnboundLocalError: Handling Local Variable References in Python
In Python, a UnboundLocalError is a type of exception that is raised when a local variable is referenced before it is assigned. This error typically occurs when a variable is used within a function before it has been given a value.
Understanding Local Variables
In Python, a variable is considered local to a function if it is assigned a value within the function. Local variables are created when the function is called and are destroyed when the function returns. This means that local variables are only accessible within the function where they are defined.
For example, consider the following code:
In this example, the variable result is a local variable because it is assigned a value within the function. It is only accessible within the add\_numbers function and cannot be accessed from outside the function.
The UnboundLocalError Exception
An UnboundLocalError exception is raised when a local variable is referenced before it is assigned. This can occur if a variable is used within a function before it has been given a value.
In this example, the variable result is used in the print statement before it is assigned a value. This will result in an UnboundLocalError exception being raised:
Handling the UnboundLocalError Exception
The UnboundLocalError exception can be handled using a try-except block. For example:
In this example, the try-except block is used to handle the UnboundLocalError exception. If the exception is raised, the code within the except block will be executed.
Avoiding the UnboundLocalError Exception
The best way to avoid the UnboundLocalError exception is to ensure that local variables are only used after they have been assigned a value. This can be done by assigning a value to the variable at the beginning of the function or by using a default value for the variable.
For example:
In this example, the variable result is assigned a default value of 0 at the beginning of the function. This ensures that the variable is always defined before it is used.
Python: Errors and Exceptions
Python: Try-Except
Code Example
In this example, the function compute\_one\_subset\_one\_strategy takes four arguments: dataset\_name , subset\_name , compute\_sum , and compute\_abs . The function uses a for loop to iterate over the files in the specified directory and calculates the residuals for each file. The residuals are then returned as a list.
The function uses a default value of 0 for the residual variable, which ensures that the variable is always defined before it is used. This helps to avoid the UnboundLocalError exception.
The function also uses a try-except block to handle any exceptions that may be raised. If an exception is raised, the function will return a message indicating that an error has occurred.
Learn how to properly assign and handle local variables in IMDiffusion to avoid UnboundLocalError exceptions.
Understanding undefined behavior in c++: directly removing elements under range filter view.
This article explores undefined behavior in C++ and its impact on directly removing elements under a range filter view using a given code snippet.
Troubleshooting Site with Hosted Web3 Storage on Cloudflare
This article provides solutions for issues encountered when using Hosted Web3 Storage on Cloudflare.
Importing CSV File to PostgreSQL Database using Talend Open Studio 8.0.1
Learn how to import a CSV file into a PostgreSQL database using Talend Open Studio 8.0.1.
Tags: : Python Error Handling IMDiffusion
Latest news
- Updating Excel DataTable: Entering Value Input Box using Row Name and Column Header Input Value Table
- File Upload Functionality in Firefox Not Working Properly on Kubuntu 22.04.4 LTS
- Unexpected Amazon Bot Activity: Mass-Downloading Web Imagery
- Understanding Enum Name Validation in Pydantic
- Error Parsing Lever API Response: Unfinished CDATA Section
- Resolving CORS Error when Calling JAX-RS Endpoint with AngularJS
- Customizing CORS Headers in Azure Functions: Attachment-Name Response
- Configuring Kafka GCS Sink Connector Flush Size with JDBC Source Connector
- Resolving Unknown Key 'home_lamlng_Arch:key' in Yay Package Manager on Arch Linux
- Compiling COBOL Code: Understanding WHITE LINES and Required Components
- Making Tags Break Like Buttons in WordPress using Elementor
- Bootstrap Model Estimation: Understanding the Differences in Heritability Estimates
- Filter Handle: Input Character Removal in Software Development
- GoneException with AWSLambdaAPIGatewayWebSockets in Bedrock/Claudestream Mobile App
- Finding the Biggest Number in C++: A Simple Example
- HitBox Item Synchronization Issue with OpenTK.WinForms: Rectangle Drawing
- Misunderstanding Singly Linked List Error Checks: The 'x != nullptr' Mystery
- Importing 'functions.ts' in TypeScript: Not Finding 'global type Promise'
- Deleting Text Array DB Entries with Prepared Statements in PostgreSQL
- Using MESON build system with a simple C++ project
- Imputing Missing Values with Amelia: Handling IV Summary Reports and Coordinate Data in R
- GitHub Action: Triggering Workflows with Path Updates and Tag Pushed
- Troubleshooting Docker Build Caches: A Case Study with Next.js and React App
- Sorting Steam Player Counts for a List of Games: An Idea and Steam API Support
- Three JSPortal Implementation Artifacts: Stencil Buffer in Three.js
- Amending Commits Quickly with Git: Rebase Dependencies
- Merging Documents in MongoDB: Aggregation and Existing Collection
- Handling IOException in BufferedWriter: Using try-catch
- Effective Use of Function Pointer Parameters with C++17 Packing, Unpacking, and Storing Parameter Packs
- Adding CSS Class Element with PHP: Timed Day
- Using pip install Git Pull Request: Handling Unmerged Pull Requests
- Geneva Action Extension Package Upload Fails: Resolving the Issue
- Adding a new class to HTML table cells: td specific number conditions
- Automating Jira Support Tickets: A Solution for Multiple Projects and Clients
- Understanding End-to-End Processes with Multiprocessing in Python
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives⢠on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Seaborn Error: local variable 'boxprops' referenced before assignment [closed]
I am trying to plot using seaborn boxplot, however, I get the following error:
These codes were executing fine last week. I have tried updating seaborn and pyfolio, yet I still get the same error. Does anyone know how to fix this?
**Attempted Solutions: **
- Updating seaborn and pyfolio to the latest versions.
Does anyone have suggestions on how to resolve this issue?

- Can you test the code as shown? Does it still generate the error? Do you get other warnings? – JohanC Commented Jun 24 at 13:58
- @JohanC It works ! thank you! Do you know what the exact problem was? – Rhea Groenenberg Commented Jun 24 at 14:05
- I think there was something wrong with the input data. Maybe the problem is "solved" in this test, but not with your real data. In that case, you could try to print out information about filtered_df and create test data similar to that one. Or maybe your update of pyfolio finally had effect (I suppose pyfolio changed something to the input they are sending to seaborn). – JohanC Commented Jun 24 at 14:10
- I'm voting to close this issue as not reproducible. – Trenton McKinney Commented Jun 25 at 1:05
Browse other questions tagged python matplotlib seaborn pyfolio or ask your own question .
- The Overflow Blog
- How to build open source apps in a highly regulated industry
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests â hereâs how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- Book that I read around 1975, where the main character is a retired space pilot hired to steal an object from a lab called Menlo Park
- How to clean up interrupted edge loops using geometry nodes and fill holes with quad faces?
- Are there examples of triple entendres in English?
- Use of Compile[] to optimize the code to run faster
- Why should I meet my advisor even if I have nothing to report?
- Old animated film with flying creatures born from a pod
- Every simple topological group is either discrete or connected
- Dagesh on final letter kaf
- Can you help me to identify the aircraft in a 1920s photograph?
- Travel to Mexico from India, do I need to pay a fee?
- Could someone translate & explain the Mesorah?
- Is non-temperature related Symmetry Breaking possible?
- Is it prohibited to consume things that unclean animals produce?
- How to handle a missing author on an ECCV paper submission after the deadline?
- Is arxiv strictly for new stuff?
- Turning Misty step into a reaction to dodge spells/attacks
- Equivariant Smith normal form?
- Is it correct to say "glide my fingernail on the adhesive tape to feel its rim"?
- Are there conditions for an elliptic Fq-curve to have a quadratic Fq-cover of the line without ramification Fq-points?
- Proposition 8 Corollary 1, Section 5.7 of Hungerford’s Algebra
- Ideal diode in parallel with resistor and voltage source
- Phantom points in QGIS do not dissapear
- Did the BBC censor a non-binary character in Transformers: EarthSpark?
- What's the point of Dream Chaser?
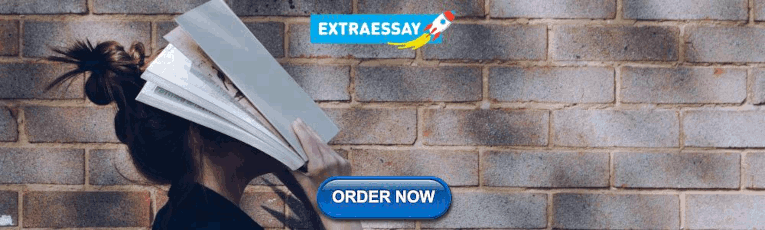
IMAGES
VIDEO
COMMENTS
File "weird.py", line 5, in main. print f(3) UnboundLocalError: local variable 'f' referenced before assignment. Python sees the f is used as a local variable in [f for f in [1, 2, 3]], and decides that it is also a local variable in f(3). You could add a global f statement: def f(x): return x. def main():
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
Traceback (most recent call last): File "identify_northsouth_point.py", line 22, in <module> findPoints(geometry, results) File "identify_northsouth_point.py", line 8, in findPoints results['north'] = (x,y) UnboundLocalError: local variable 'x' referenced before assignment I have tried global and nonlocal, but it does not work.
Avoid Reassignment of Global Variables. Below, code calculates a new value (local_var) based on the global variable and then prints both the local and global variables separately.It demonstrates that the global variable is accessed directly without being reassigned within the function.
The Python "UnboundLocalError: Local variable referenced before assignment" occurs when we reference a local variable before assigning a value to it in a function. To solve the error, mark the variable as global in the function definition, e.g. global my_var .
The UnboundLocalError: local variable 'x' referenced before assignment occurs when you reference a variable inside a function before declaring that variable. To resolve this error, you need to use a different variable name when referencing the existing variable, or you can also specify a parameter for the function. I hope this tutorial is useful.
UnboundLocalError: local variable referenced before assignment. Example #1: Accessing a Local Variable. Solution #1: Passing Parameters to the Function. Solution #2: Use Global Keyword. Example #2: Function with if-elif statements. Solution #1: Include else statement. Solution #2: Use global keyword. Summary.
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
To fix this, you can either move the assignment of the variable x before the print statement, or give it an initial value before the print statement. def example (): x = 5 print (x) example()
UnboundLocalError: local variable referenced before assignment. 1. ... Error: local variable referenced before assignment in ArcPy. 1. python - psycopg2.errors.RaiseException find_srid() - could not find the corresponding SRID. 4. Using ogr2ogr in Python scripts. Hot Network Questions Psychology Today Culture Fair IQ test question
UnboundLocalError: local variable 'a' referenced before assignment The text was updated successfully, but these errors were encountered: đ 17 callensm, bendesign55, nulladdict, rohanbanerjee, balovbohdan, drewszurko, hellotuitu, rribani, jeromesteve202, egmaziero, and 7 more reacted with thumbs up emoji
UnboundLocalError: local variable 'count_all' referenced before assignment The detail path of debug trace is in below: Traceback (most recent call last): File "finetune.py", line 186, in test preds...
UnboundLocalError: local variable 's' referenced before assignment #1016. NikolaiVChr opened this issue Jun 29, 2024 ¡ 1 comment Comments. Copy link NikolaiVChr commented Jun 29, 2024 ⢠... s UnboundLocalError: local variable 's' referenced before assignment ...
A closure binds values in the enclosing environment to names in the local environment. The local environment can then use the bound value, and even reassign that name to something else, but it can't modify the binding in the enclosing environment.
In order for you to modify test1 while inside a function you will need to do define test1 as a global variable, for example: test1 = 0. def test_func(): global test1. test1 += 1. test_func() However, if you only need to read the global variable you can print it without using the keyword global, like so: test1 = 0.
I have following simple function to get percent values for different cover types from a raster. It gives me following error: UnboundLocalError: local variable 'a' referenced before assignment whic...
UnboundLocalError: local variable 'player' referenced before assignment. here's the actual code: if player.cooling_time > 0: player.cooling_time -= 1 Share Sort by: Best. Open comment sort options ... UnboundLocalError: local variable 'player' referenced before assignment Reply reply
UnboundLocalError: local variable 'ru_blade' referenced before assignment Unless I am missing something else, this doesn't make sense since I don't have duplicate global variables. Here is the code.
@catskillsresearch: The external file you link to has too many dependencies to try to run it here.And even then, we don't know which input has been used. It would help if you'd inspect the values and datatypes of is_returns, is_weekly, is_monthly and kwargs, and based on those try to create a stand-alone example.Can you verify whether my tests below coincide with your case?
Understanding Local Variables. In Python, a variable is considered local to a function if it is assigned a value within the function. Local variables are created when the function is called and are destroyed when the function returns. This means that local variables are only accessible within the function where they are defined.
UnBoundLocalError: local variable referenced before assignment (Python) 1. ... "UnboundLocalError: local variable referenced before assignment" when calling a function. 0. global variable and reference before assignment. 2. UnboundLocalError: local variable <var> referenced before assignment. 1.
UnboundLocalError: local variable 'labels' referenced before assignment IMDiffusion. Ask Question ... local variable 'labels' referenced before assignment run:python compute_score.py --dataset_name SMD There are errors in the lines I've marked with line numbers. I want to know why they are wrong and how to fix them. ... local variable ...
I think there was something wrong with the input data. Maybe the problem is "solved" in this test, but not with your real data. In that case, you could try to print out information about filtered_df and create test data similar to that one. Or maybe your update of pyfolio finally had effect (I suppose pyfolio changed something to the input they are sending to seaborn).