How to Use Functions in C - Explained With Examples
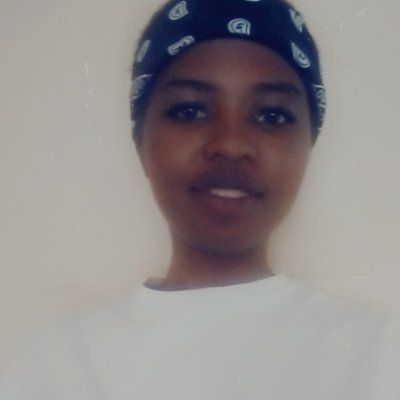
Functions are an essential component of the C programming language. They help you divide bigger problems into smaller, more manageable chunks of code, making it simpler to create and run programs.
We'll look at functions in C, their syntax, and how to use them successfully in this article.
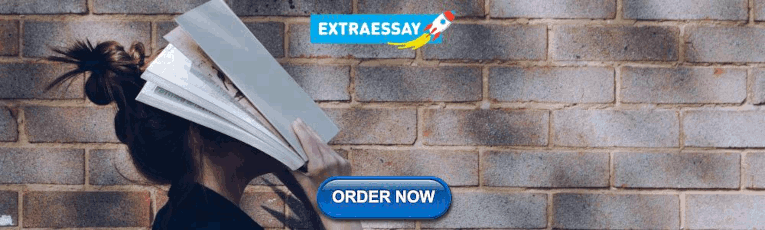
What is a Function in C?
A function is a block of code that executes a particular task in programing. It is a standalone piece of code that can be called from anywhere in the program.
A function can take in parameters, run computations, and output a value. A function in C can be created using this syntax:
The return_type specifies the type of value that the function will return. If the function does not return anything, the return_type will be void .
The function_name is the name of the function, and the parameter list specifies the parameters that the function will take in.
How to Declare a Function in C
Declaring a function in C informs the compiler about the presence of a function without giving implementation details. This enables the function to be called by other sections of the software before it is specified or implemented.
A function declaration usually contains the function name , return type , and the parameter types. The following is the syntax for defining a function in C:
Here, return_type is the data type of the value that the function returns. function_name is the name of the function, and parameter_list is the list of parameters that the function takes as input.
For example, suppose we have a function called add that takes two integers as input and returns their sum. We can declare the function as follows:
This tells the compiler that there is a function called add that takes two integers as input and returns an integer as output.
It's worth noting that function declarations do not include the function body, which includes the actual code that runs when the function is invoked.
The body of the function is defined independently of the function statement, usually in a separate block of code called the function definition.
Here's an example:
In this example, the add function is declared with a function statement at the top of the file, which specifies its name, return type ( int ), and parameters ( a and b , both int s).
The actual code for the add function is defined in the function definition. Here, the function simply adds its two parameters and returns the result.
The main function calls the add function with arguments 2 and 3 , and stores the result in the result variable. Finally, it prints the result using the printf function.
How to Use a Function in Multiple Source Files
If you want to use a function in numerous source files, you must include a function declaration (also known as a function prototype) in the header file and the definition in one source file.
when you build, you first compile the source files to object files, and then you link the object files into the final executable.
Let's create a header file called myfunctions.h :
In this header file, we declare a function add using a function statement.
Next, let's create a source file called myfunctions.c , which defines the add function:
In this file, we include the myfunctions.h header file using quotes, and we define the add function.
Finally, let's create a source file called main.c , which uses the add function:
In this file, we include both the stdio.h header file and our myfunctions.h header file using angle brackets and quotes, respectively. We then call the add function, passing in values a and b and storing the result in sum . Finally, we print the result using printf .
The way you create it is heavily influenced by your environment. If you are using an IDE (such as Visual Studio), you must position all files in the proper locations in the project.
If you are creating from the command line e.g Linux. To compile this program, you would need to compile both myfunctions.c and main.c and link them together as shown below:
The -c option instructs the compiler to create an object file with the same name as the source file but with a .o suffix. The final instruction joins the two object files to create the final executable, which is named program (the -o option specifies the name of the output file).
What Happens if You Call a Function Before Its Declaration in C?
In this instance, the computer believes the usual return type is an integer. If the function gives a different data type, it throws an error.
If the return type is also an integer, it will function properly. But some cautions may be generated:
In this code, the function function() is called before it is declared. This returns an error:

How to Define a Function in C
Assuming you want to create a code that accepts two integers and returns their sum, you can define a function that does that this way:
In this example, the function sum takes in two integer parameters – num1 and num2 . The function calculates their sum and returns the result. The return type of the function is int .
Where Should a Function Be Defined?
In C, a function can be defined anywhere in the program, as long as it is defined before it is used. But it is a good practice to define functions at the beginning of the file or in a separate file to make the code more readable and organized.
Here's an example code showing how to define a function in C:
In this example, the function add() is defined after its declaration (or prototype) within the same file.
Another approach is to define the function in a separate header file, which is then included in the main file using the #include directive. For example:
In this approach, the function declaration (or prototype) is included in the header file math.h , which is then included in the main file main.c using the #include directive. The function implementation is defined in a separate file math.c .
This approach allows for better code organization and modularity, as the function implementation can be separated from the main program code.
How to Call a Function in C
We can call a function from anywhere in the program once we've defined it. We use the function name followed by the argument list in parentheses to call a function. For example, we can use the following code to call the sum function that we defined earlier:
In this code, we are calling the sum function with a and b as its parameters. The function returns the sum of a and b , which is then stored in the variable c .
How to Pass Parameters to a Function
There are two methods of passing parameters (also called arguments) to a function in C: by value and by reference.
When we pass a parameter by value, the method receives a copy of the parameter's value. Changes to the parameter within the code have no effect on the initial variable outside the function.
When we pass a parameter by reference, the method receives a link to the parameter's memory location. Any modifications to the parameter within the code will have an impact on the initial variable outside the function.
Consider the following examples of passing parameters by value and by reference. Assuming we want to create a function that accepts an integer and multiplies it by two, the function can be defined as follows:
In this example, the function doubleValue takes in an integer parameter num by value. It doubles the value of num and assigns it back to num . However, this change will not affect the original value of num outside the function.
Here's another example that shows how you can pass a single parameter by value:
In this example, we define a function called square that takes an integer parameter num by value. Inside the function, we calculate the square of num and print the result. We then call the function with the argument 5 .
Now, let's look at an example of passing a parameter by reference:
In this example, we define a function square that takes an integer pointer parameter num by reference. Inside the function, we reference the pointer and calculate the square of the value pointed to by num .
We then call the function with the address of the integer variable x . After calling the function, the value of x is modified to be the square of its original value, which we then print in the main function.
In conclusion, functions are an essential component of C programming. You can use them to divide large problems into smaller, more manageable pieces of code.
You can declare and define functions in C, and pass parameters either by value or by reference. It's a good practice to declare all functions before using them, and to define them at the beginning of the file or in a separate file for better code organization and modularity.
By using functions effectively, you can write cleaner, more readable code that is easier to debug and maintain.
A curious full-stack web developer. I love solving problems using software development and representing Data in a meaningful way. I like pushing myself and taking up new challenges.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
C Recursion
- C Storage Class
C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
- Check Prime or Armstrong Number Using User-defined Function
C Control Flow Examples
- Check Whether a Number can be Expressed as Sum of Two Prime Numbers
- Convert Binary Number to Octal and vice-versa
- Find Factorial of a Number Using Recursion
String Examples in C Programming
A function is a block of code that performs a specific task.
You will find examples related to functions in this article. To understand examples in this page, you should have the knowledge of the following topics:
- User-Defined Function
- Types of User-defined functions
- Scope of a local variable
Sorry about that.
Related Tutorials
C Struct Examples

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Scope Rules
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Recursion
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable or an expression. The value to be assigned forms the right hand operand, whereas the variable to be assigned should be the operand to the left of = symbol, which is defined as a simple assignment operator in C. In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple assignment operator (=)
The = operator is the most frequently used operator in C. As per ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed. You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented assignment operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression a+=b has the same effect of performing a+b first and then assigning the result back to the variable a.
Similarly, the expression a<<=b has the same effect of performing a<<b first and then assigning the result back to the variable a.
Here is a C program that demonstrates the use of assignment operators in C:
When you compile and execute the above program, it produces the following result −
cppreference.com
Copy assignment operator.
A copy assignment operator is a non-template non-static member function with the name operator = that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
For the formal copy assignment operator syntax, see function declaration . The syntax list below only demonstrates a subset of all valid copy assignment operator syntaxes.
[ edit ] Explanation
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) .
Due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++14) . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types, the operator performs member-wise copy assignment of the object's direct bases and non-static data members, in their initialization order, using built-in assignment for the scalars, memberwise copy-assignment for arrays, and copy assignment operator for class types (called non-virtually).
[ edit ] Deleted copy assignment operator
An implicitly-declared or explicitly-defaulted (since C++11) copy assignment operator for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a non-static data member of a const-qualified non-class type (or possibly multi-dimensional array thereof).
- T has a non-static data member of a reference type.
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that the overload resolution as applied to find M 's copy assignment operator
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
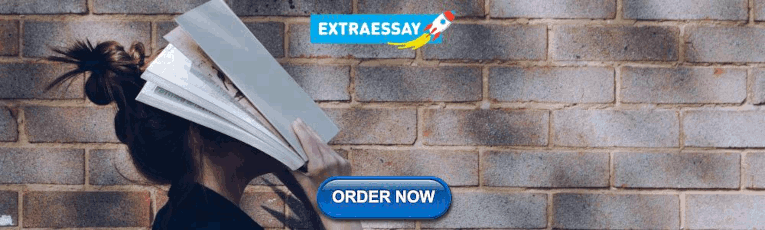
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial.
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Eligible copy assignment operator
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type .
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy constructor
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 15:13.
- This page has been accessed 1,333,785 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- <cassert> (assert.h)
- <cctype> (ctype.h)
- <cerrno> (errno.h)
- C++11 <cfenv> (fenv.h)
- <cfloat> (float.h)
- C++11 <cinttypes> (inttypes.h)
- <ciso646> (iso646.h)
- <climits> (limits.h)
- <clocale> (locale.h)
- <cmath> (math.h)
- <csetjmp> (setjmp.h)
- <csignal> (signal.h)
- <cstdarg> (stdarg.h)
- C++11 <cstdbool> (stdbool.h)
- <cstddef> (stddef.h)
- C++11 <cstdint> (stdint.h)
- <cstdio> (stdio.h)
- <cstdlib> (stdlib.h)
- <cstring> (string.h)
- C++11 <ctgmath> (tgmath.h)
- <ctime> (time.h)
- C++11 <cuchar> (uchar.h)
- <cwchar> (wchar.h)
- <cwctype> (wctype.h)
Containers:
- C++11 <array>
- <deque>
- C++11 <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- C++11 <unordered_map>
- C++11 <unordered_set>
- <vector>
Input/Output:
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
Multi-threading:
- C++11 <atomic>
- C++11 <condition_variable>
- C++11 <future>
- C++11 <mutex>
- C++11 <thread>
- <algorithm>
- <bitset>
- C++11 <chrono>
- C++11 <codecvt>
- <complex>
- <exception>
- <functional>
- C++11 <initializer_list>
- <iterator>
- <limits>
- <locale>
- <memory>
- <new>
- <numeric>
- C++11 <random>
- C++11 <ratio>
- C++11 <regex>
- <stdexcept>
- <string>
- C++11 <system_error>
- C++11 <tuple>
- C++11 <type_traits>
- C++11 <typeindex>
- <typeinfo>
- <utility>
- <valarray>
- C++11 mem_fn
wrapper classes
- binary_negate
- C++11 function
- C++11 reference_wrapper
- unary_negate
operator classes
- C++11 bit_and
- C++11 bit_or
- C++11 bit_xor
- greater_equal
- logical_and
- logical_not
- not_equal_to
other classes
- C++11 bad_function_call
- C++11 is_bind_expression
- C++11 is_placeholder
- C++11 placeholders
- binary_function
- const_mem_fun_ref_t
- const_mem_fun_t
- const_mem_fun1_ref_t
- const_mem_fun1_t
- mem_fun_ref
- mem_fun_ref_t
- mem_fun1_ref_t
- pointer_to_binary_function
- pointer_to_unary_function
- unary_function
- C++11 function::~function
- C++11 function::function
member functions
- C++11 function::assign
- C++11 function::operator bool
- C++11 function::operator()
- C++11 function::operator=
- C++11 function::swap
- C++11 function::target
- C++11 function::target_type
non-member overloads
- C++11 relational operators (function)
- C++11 swap (function)
std:: function ::operator=
Return value, exception safety.
C Functions
C structures.
A function is a block of code which only runs when it is called.
You can pass data, known as parameters, into a function.
Functions are used to perform certain actions, and they are important for reusing code: Define the code once, and use it many times.
Predefined Functions
So it turns out you already know what a function is. You have been using it the whole time while studying this tutorial!
For example, main() is a function, which is used to execute code, and printf() is a function; used to output/print text to the screen:
Create a Function
To create (often referred to as declare ) your own function, specify the name of the function, followed by parentheses () and curly brackets {} :
Example Explained
- myFunction() is the name of the function
- void means that the function does not have a return value. You will learn more about return values later in the next chapter
- Inside the function (the body), add code that defines what the function should do
Call a Function
Declared functions are not executed immediately. They are "saved for later use", and will be executed when they are called.
To call a function, write the function's name followed by two parentheses () and a semicolon ;
In the following example, myFunction() is used to print a text (the action), when it is called:
Inside main , call myFunction() :
A function can be called multiple times:
C Exercises
Test yourself with exercises.
Create a method named myFunction and call it inside main() .
Start the Exercise

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Immediate Functions in C++
- Passing a Function as a Parameter in C++
- Creating a C++ reusable Header File and its Implementation Files
- Difference between std::numeric_limits<T> min, max, and lowest in C++
- C++ Keywords
- std::fstream::close() in C++
- real() function in C++
- Why it is important to write "using namespace std" in C++ program?
- Reason of runtime error in C/C++
- How to create the boilerplate code in VS Code?
- Memory Limit Exceeded Error
- MakeFile in C++ and its applications
- imag() function in C++
- Reciprocating from a function statement
- Encrypt and decrypt text file using C++
- Line control directive
- Recursive lambda expressions in C++
- How does generic find() function works in C++ STL?
- Object Delegation in C++
Assigning function to a variable in C++
In C++ , assigning a function to a variable and using that variable for calling the function as many times as the user wants, increases the code reusability. Below is the syntax for the same:
Program 1: Below is the C++ program to implement a function assigned to a variable:
Program 2: Below is the C++ program to implement a parameterized function assigned to a variable:
Program 3: Below is the C++ program to implement a function assigned to a variable that returns a value:
Please Login to comment...
- CPP-Functions
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
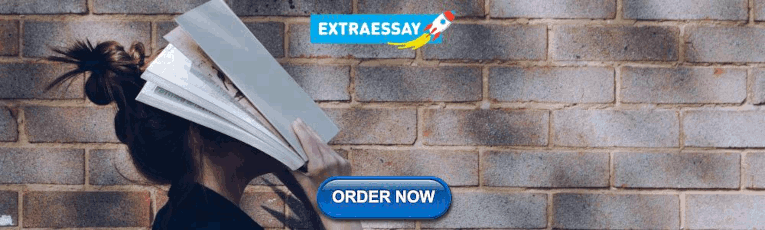
IMAGES
VIDEO
COMMENTS
With foo changed to a function pointer type foo = baz; is perfectly legal. The name of a function in an expression is converted to a pointer-to-function type (except where it is the argument to unary & or sizeof). -
The target type of the function pointer must be upward compatible with the type of the function (see Compatible Types).. There is no need for '&' in front of double_add.Using a function name such as double_add as an expression automatically converts it to the function's address, with the appropriate function pointer type. However, it is ok to use '&' if you feel that is clearer:
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: 2. "+=": This operator is combination of '+' and '=' operators.
For example, we can use the following code to call the sum function that we defined earlier: int a = 5; int b = 10; int c = sum(a, b); In this code, we are calling the sum function with a and b as its parameters. The function returns the sum of a and b, which is then stored in the variable c.
A function in C is a set of statements that when called perform some specific task.It is the basic building block of a C program that provides modularity and code reusability. The programming statements of a function are enclosed within { } braces, having certain meanings and performing certain operations.They are also called subroutines or procedures in other languages.
The declaration of a function pointer variable (or structure field) looks almost like a function declaration, except it has an additional ' * ' just before the variable name. Proper nesting requires a pair of parentheses around the two of them. For instance, int (*a) (); says, "Declare a as a pointer such that *a is an int -returning ...
In C, we can use function pointers to avoid code redundancy. For example a simple qsort () function can be used to sort arrays in ascending order or descending or by any other order in case of array of structures. Not only this, with function pointers and void pointers, it is possible to use qsort for any data type.
An assignment in C is an expression because it has a value; we call it an assignment expression. A simple assignment looks like. lvalue = value-to-store. We say it assigns the value of the expression value-to-store to the location lvalue, or that it stores value-to-store there. You can think of the "l" in "lvalue" as standing for ...
C Function Examples. Display all prime numbers between two Intervals. Check prime and Armstrong number by making functions. Check whether a number can be expressed as the sum of two prime numbers. Find the sum of natural numbers using recursion. Calculate the factorial of a number using recursion. Find G.C.D using recursion.
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Parameters fn Either a function object of the same type, or some function, function pointer, pointer to member, or function object, as forwarded to function's constructor. Return value none Data races The object is modified (including both its target and its allocator). If fn is an rvalue reference, the function may modify fn. Exception safety If fn is a function pointer or a reference_wrapper ...
Equivalent to function (std:: allocator_arg, alloc, std:: forward < F > (f)). swap (* this);. Contents. 1 Parameters; 2 Return value; 3 Exceptions; 4 See also Parameters. f - callable function to initialize the target with alloc - allocator to use to allocate memory for the internal data structures
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
(4) clearing assignment The object becomes an empty function object. Parameters rhs A function object of the same type (with the same signature, as described by its template parameters) whose target is either copied or moved. If rhs is an empty function object, the object becomes an empty function. fn Either a function, a function pointer, a pointer to member, or any kind of function object (i ...
C Functions. C. Functions. A function is a block of code which only runs when it is called. You can pass data, known as parameters, into a function. Functions are used to perform certain actions, and they are important for reusing code: Define the code once, and use it many times.
Few Points to Note regarding functions in C: 1) main() in C program is also a function. 2) Each C program must have at least one function, which is main(). 3) There is no limit on number of functions; A C program can have any number of functions. 4) A function can call itself and it is known as "Recursion". I have written a separate guide ...
Assigning function to a variable in C++. In C++, assigning a function to a variable and using that variable for calling the function as many times as the user wants, increases the code reusability. Below is the syntax for the same: Syntax: C++. auto fun = [&]() {. cout << "inside function".
So assigning function to one another generally means assigning the address of the function to a variable which can hold an address, i.e. a function pointer. void f(){} typedef void(*pF)(); //typedef for easy use. pf foo; //create a function pointer object. foo = &f; //assign it the address of the function. Share.
The reason why is ill formed, is that the member functions of the class std::vector could call the assignment operator of its template argument, but in this case it's a constant type parameter "const int" which means it doesn't have an assignment operator ( it's none sense to assign to a const variable!!). the same behavior is observed with a ...