
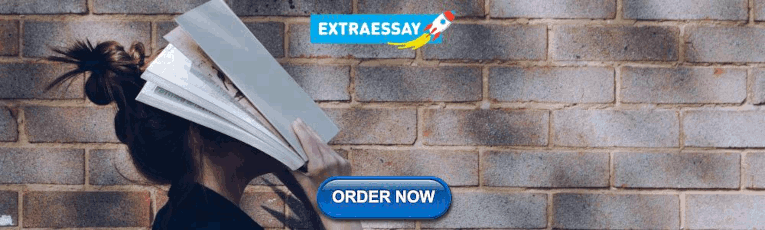
Typeerror: int object does not support item assignment
Today, we will explore the “typeerror: int object does not support item assignment” error message in Python.
If this error keeps bothering you, don’t worry! We’ve got your back.
In this article, we will explain in detail what this error is all about and why it occurs in your Python script.
Aside from that, we’ll hand you the different solutions that will get rid of this “typeerror: ‘int’ object does not support item assignment” error.
What is “typeerror: ‘int’ object does not support item assignment”?
The “typeerror int object does not support item assignment” is an error message in Python that occurs when you try to assign a value to an integer as if it were a list or dictionary, which is not allowed.
It is mainly because integers are immutable in Python, meaning their value cannot be changed after they are created.
In other words, once an integer is created, its value cannot be changed.
For example:
In this example, the code tries to assign the value 1 to the first element of sample , but sample is an integer, not a list or dictionary, and as a result, it throws an error:
This error message indicates that you need to modify your code to avoid attempting to change an integer’s value using item assignment.
What are the root causes of “typeerror: ‘int’ object does not support item assignment”
Here are the most common root causes of “int object does not support item assignment”, which include the following:
- Attempting to modify an integer directly
- Using an integer where a sequence is expected
- Not converting integer objects to mutable data types before modifying them
- Using an integer as a dictionary key
- Passing an integer to a function that expects a mutable object
- Mixing up variables
- Incorrect syntax
- Using the wrong method or function
How to fix “typeerror: int object does not support item assignment”
Here are the following solutions to fix the “typeerror: ‘int’ object does not support item assignment” error message:
Solution 1: Use a list instead of an integer
Since integers are immutable in Python, we cannot modify them.
Therefore, we can use a mutable data type such as a list instead to modify individual elements within a variable.
Solution 2: Convert the integer to a list first
You have to convert the integer first to a string using the str() function.
Then, convert the string to a list using the list() function.
We can also modify individual elements within the list and join it back into a string using the join() function.
Finally, we convert the string back to an integer using the int() function.
Solution 3: Use a dictionary instead of an integer
This solution is similar to using a list. We can use a dictionary data type to modify individual elements within a variable.
In this example code, we modify the value of the ‘b’ key within the dictionary.
Another example:
Solution 4: Use a different method or function
You can define sample as a list and append items to the list using the append() method .
How to avoid “typeerror: int object does not support item assignment”
- You have to use appropriate data types for the task at hand.
- You must convert immutable data types to mutable types before modifying it.
- You have to use the correct operators and functions for specific data types.
- Avoid modifying objects directly if they are not mutable.
Frequently Asked Question (FAQs)
You can easily resolve the error by converting the integer object to a mutable data type or creating a new integer object with the desired value.
The error occurs when you try to modify an integer object, which is not allowed since integer objects are immutable.
By executing the different solutions that this article has already given, you can definitely resolve the “typeerror: int object does not support item assignment” error message in Python.
We are hoping that this article provides you with sufficient solutions.
You could also check out other “ typeerror ” articles that may help you in the future if you encounter them.
- Uncaught typeerror: illegal invocation
- Typeerror slice none none none 0 is an invalid key
- Typeerror: cannot read property ‘getrange’ of null
Thank you very much for reading to the end of this article.

- TypeError: 'str' object does not support item assignment
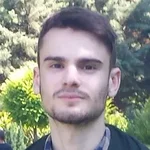
Last updated: Apr 8, 2024 Reading time · 8 min
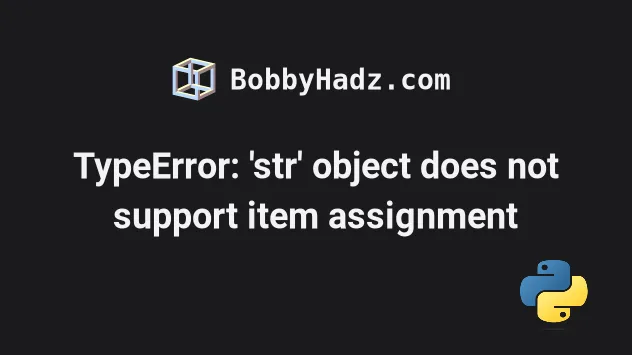
# Table of Contents
- TypeError: 'int' object does not support item assignment
- 'numpy.float64' object does not support item assignment
# TypeError: 'str' object does not support item assignment
The Python "TypeError: 'str' object does not support item assignment" occurs when we try to modify a character in a string.
Strings are immutable in Python, so we have to convert the string to a list, replace the list item and join the list elements into a string.

Here is an example of how the error occurs.
We tried to change a specific character of a string which caused the error.
Strings are immutable, so updating the string in place is not an option.
Instead, we have to create a new, updated string.
# Using str.replace() to get a new, updated string
One way to solve the error is to use the str.replace() method to get a new, updated string.
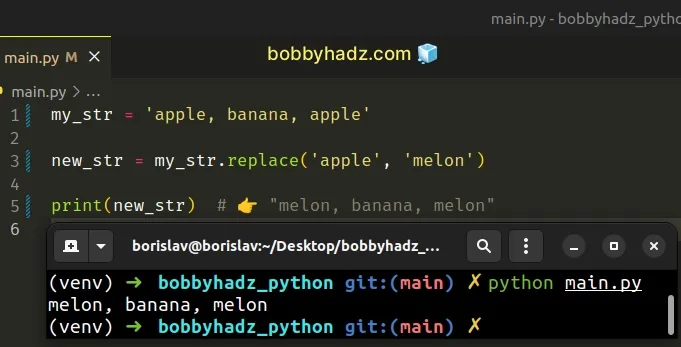
The str.replace() method returns a copy of the string with all occurrences of a substring replaced by the provided replacement.
The method takes the following parameters:
By default, the str.replace() method replaces all occurrences of the substring in the string.
If you only need to replace the first occurrence, set the count argument to 1 .
Setting the count argument to 1 means that only the first occurrence of the substring is replaced.
# Replacing a character with a conversion to list
One way to replace a character at a specific index in a string is to:
- Convert the string to a list.
- Update the list item at the specified index.
- Join the list items into a string.
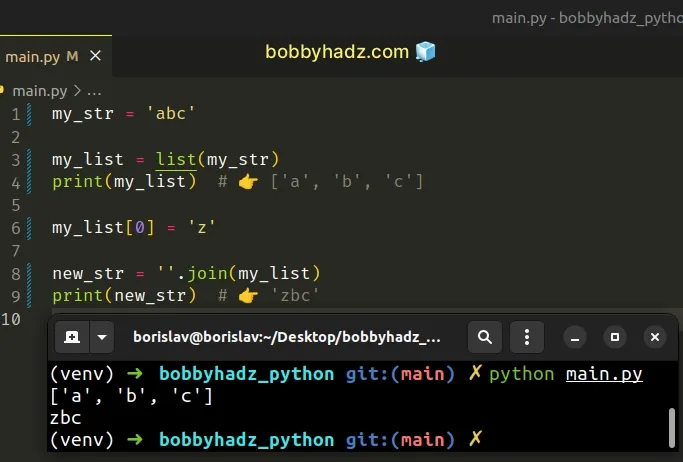
We passed the string to the list() class to get a list containing the string's characters.
The last step is to join the list items into a string with an empty string separator.
The str.join() method takes an iterable as an argument and returns a string which is the concatenation of the strings in the iterable.
Python indexes are zero-based, so the first character in a string has an index of 0 , and the last character has an index of -1 or len(a_string) - 1 .
If you have to do this often, define a reusable function.
The update_str function takes a string, index and new characters as parameters and returns a new string with the character at the specified index updated.
An alternative approach is to use string slicing .
# Reassigning a string variable
If you need to reassign a string variable by adding characters to it, use the += operator.
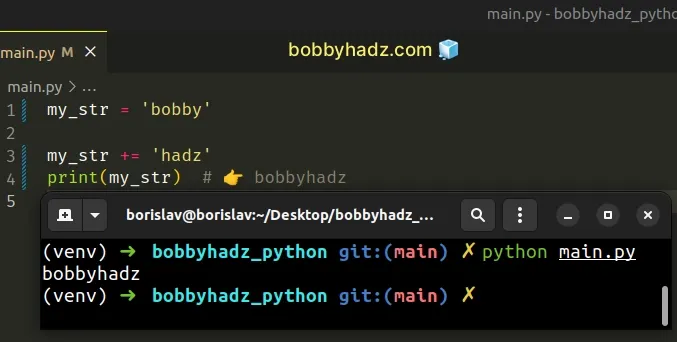
The += operator is a shorthand for my_str = my_str + 'new' .
The code sample achieves the same result as using the longer form syntax.
# Using string slicing to get a new, updated string
Here is an example that replaces an underscore at a specific index with a space.
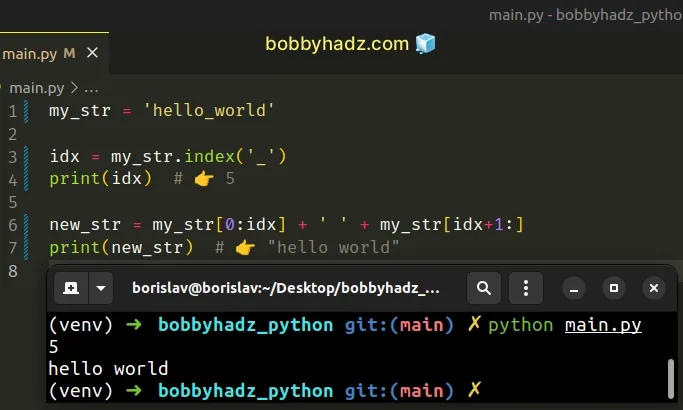
The first piece of the string we need is up to, but not including the character we want to replace.
The syntax for string slicing is a_string[start:stop:step] .
The start index is inclusive, whereas the stop index is exclusive (up to, but not including).
The slice my_str[0:idx] starts at index 0 and goes up to, but not including idx .
The next step is to use the addition + operator to add the replacement string (in our case - a space).
The last step is to concatenate the rest of the string.
Notice that we start the slice at index + 1 because we want to omit the character we are replacing.
We don't specify an end index after the colon, therefore the slice goes to the end of the string.
We simply construct a new string excluding the character at the specified index and providing a replacement string.
If you have to do this often define a reusable function.
The function takes a string, index and a replacement character as parameters and returns a new string with the character at the specified index replaced.
If you need to update multiple characters in the function, use the length of the replacement string when slicing.
The function takes one or more characters and uses the length of the replacement string to determine the start index for the second slice.
If the user passes a replacement string that contains 2 characters, then we omit 2 characters from the original string.
# TypeError: 'int' object does not support item assignment
The Python "TypeError: 'int' object does not support item assignment" occurs when we try to assign a value to an integer using square brackets.
To solve the error, correct the assignment or the accessor, as we can't mutate an integer value.

We tried to change the digit at index 0 of an integer which caused the error.
# Declaring a separate variable with a different name
If you meant to declare another integer, declare a separate variable with a different name.
# Changing an integer value in a list
Primitives like integers, floats and strings are immutable in Python.
If you meant to change an integer value in a list, use square brackets.
Python indexes are zero-based, so the first item in a list has an index of 0 , and the last item has an index of -1 or len(a_list) - 1 .
We used square brackets to change the value of the list element at index 0 .
# Updating a value in a two-dimensional list
If you have two-dimensional lists, you have to access the list item at the correct index when updating it.
We accessed the first nested list (index 0 ) and then updated the value of the first item in the nested list.
# Reassigning a list to an integer by mistake
Make sure you haven't declared a variable with the same name multiple times and you aren't reassigning a list to an integer somewhere by mistake.
We initially declared the variable and set it to a list, however, it later got set to an integer.
Trying to assign a value to an integer causes the error.
To solve the error, track down where the variable got assigned an integer and correct the assignment.
# Getting a new list by running a computation
If you need to get a new list by running a computation on each integer value of the original list, use a list comprehension .
The Python "TypeError: 'int' object does not support item assignment" is caused when we try to mutate the value of an int.
# Checking what type a variable stores
If you aren't sure what type a variable stores, use the built-in type() class.
The type class returns the type of an object.
The isinstance() function returns True if the passed-in object is an instance or a subclass of the passed-in class.
# 'numpy.float64' object does not support item assignment
The Python "TypeError: 'numpy.float64' object does not support item assignment" occurs when we try to assign a value to a NumPy float using square brackets.
To solve the error, correct the assignment or the accessor, as we can't mutate a floating-point number.

We tried to change the digit at index 0 of a NumPy float.
# Declaring multiple floating-point numbers
If you mean to declare another floating-point number, simply declare a separate variable with a different name.
# Floating-point numbers are immutable
Primitives such as floats, integers and strings are immutable in Python.
If you need to update a value in an array of floating-point numbers, use square brackets.
We changed the value of the array element at index 0 .
# Reassigning a variable to a NumPy float by mistake
Make sure you haven't declared a variable with the same name multiple times and you aren't reassigning a list to a float somewhere by mistake.
We initially set the variable to a NumPy array but later reassigned it to a floating-point number.
Trying to update a digit in a float causes the error.
# When working with two-dimensional arrays
If you have a two-dimensional array, access the array element at the correct index when updating it.
We accessed the first nested array (index 0 ) and then updated the value of the first item in the nested array.
The Python "TypeError: 'float' object does not support item assignment" is caused when we try to mutate the value of a float.
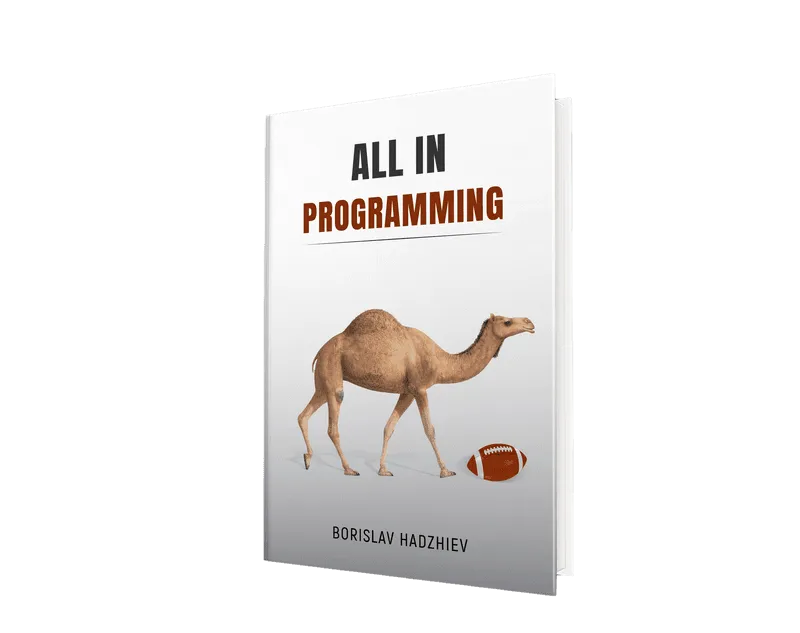
Borislav Hadzhiev
Web Developer
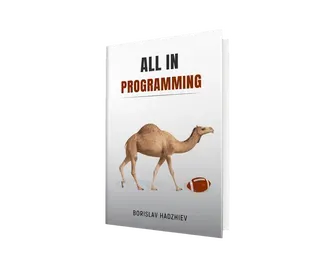
Copyright © 2024 Borislav Hadzhiev
TypeError: builtin_function_or_method object is not subscriptable Python Error [SOLVED]
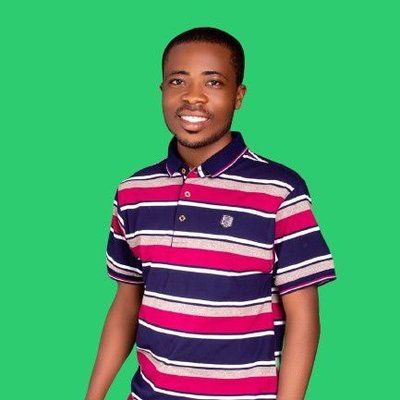
As the name suggests, the error TypeError: builtin_function_or_method object is not subscriptable is a “typeerror” that occurs when you try to call a built-in function the wrong way.
When a "typeerror" occurs, the program is telling you that you’re mixing up types. That means, for example, you might be concatenating a string with an integer.
In this article, I will show you why the TypeError: builtin_function_or_method object is not subscriptable occurs and how you can fix it.
Why The TypeError: builtin_function_or_method object is not subscriptable Occurs
Every built-in function of Python such as print() , append() , sorted() , max() , and others must be called with parenthesis or round brackets ( () ).
If you try to use square brackets, Python won't treat it as a function call. Instead, Python will think you’re trying to access something from a list or string and then throw the error.
For example, the code below throws the error because I was trying to print the value of the variable with square braces in front of the print() function:
And if you surround what you want to print with square brackets even if the item is iterable, you still get the error:
This issue is not particular to the print() function. If you try to call any other built-in function with square brackets, you also get the error.
In the example below, I tried to call max() with square brackets and I got the error:
How to Fix the TypeError: builtin_function_or_method object is not subscriptable Error
To fix this error, all you need to do is make sure you use parenthesis to call the function.
You only have to use square brackets if you want to access an item from iterable data such as string, list, or tuple:
Wrapping Up
This article showed you why the TypeError: builtin_function_or_method object is not subscriptable occurs and how to fix it.
Remember that you only need to use square brackets ( [] ) to access an item from iterable data and you shouldn't use it to call a function.
If you’re getting this error, you should look in your code for any point at which you are calling a built-in function with square brackets and replace it with parenthesis.
Thanks for reading.
Web developer and technical writer focusing on frontend technologies. I also dabble in a lot of other technologies.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
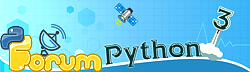
- View Active Threads
- View Today's Posts
- View New Posts
- My Discussions
- Unanswered Posts
- Unread Posts
- Active Threads
- Mark all forums read
- Member List
- Interpreter
'int' object does not support item assignment
- Python Forum
- Python Coding
- General Coding Help
- 0 Vote(s) - 0 Average
- View a Printable Version
User Panel Messages
Announcements.
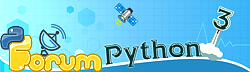
Login to Python Forum
TypeError: 'src' object does not support item assignment
The assignment str[i] = str[j] is working inconsistently. Please refer to the screenshots and let me know if I am missing something.
We are receiving TypeError: ‘src’ object does not support item assignment
Regards, Praveen. Thank you!
Please don’t use screenshots. Show the code and the traceback as text.
Strings are immutable. You can’t modify a string by trying to change a character within.
You can create a new string with the bits before, the bits after, and whatever you want in between.
Yeah, you cannot assign a string to a variable, and then modify the string, but you can use the string to create a new one and assign that result to the same variable. Borrowing some code from @BowlOfRed above, you can do this:
Related Topics
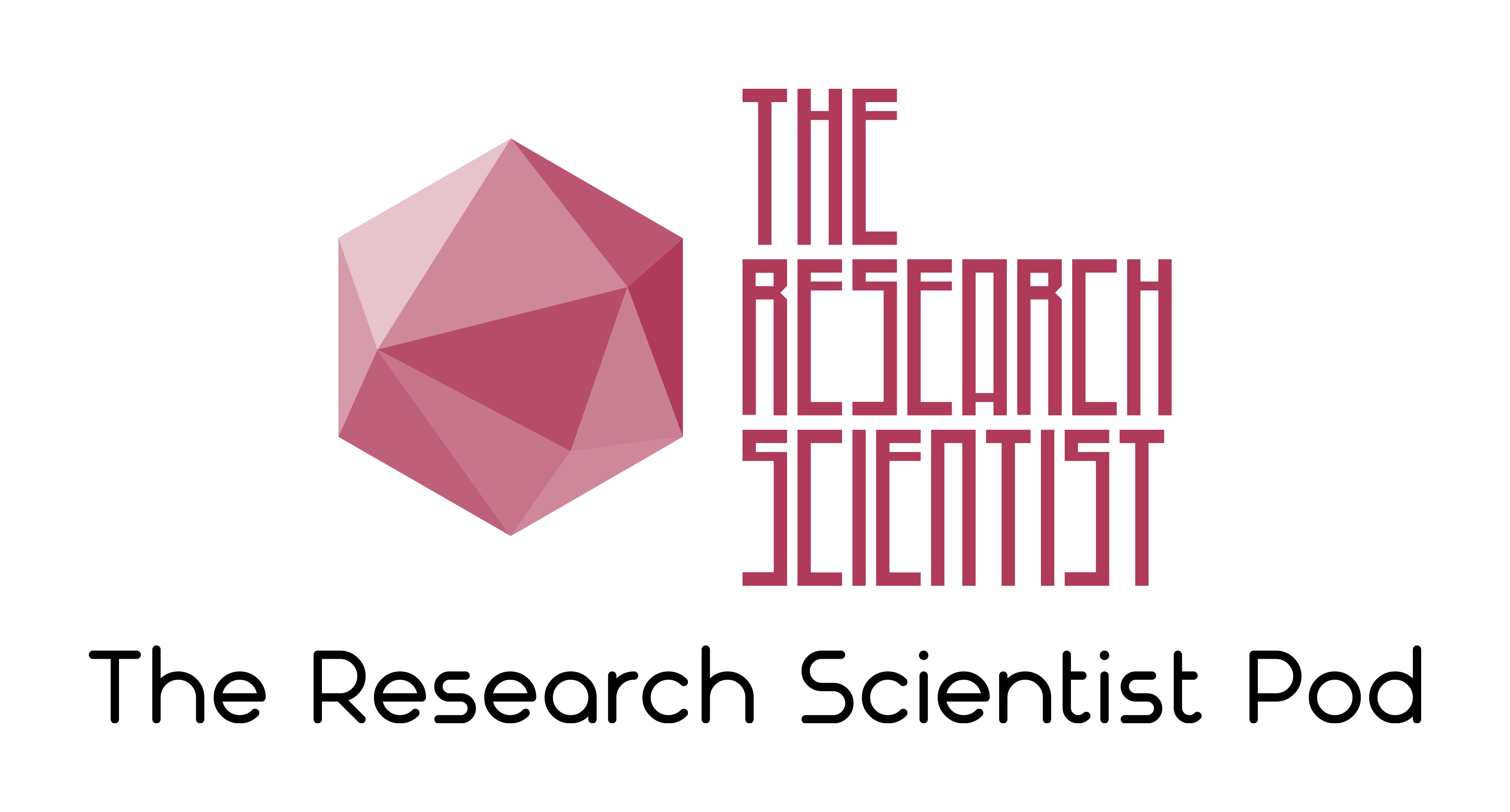
How to Solve Python TypeError: ‘set’ object does not support item assignment
by Suf | Programming , Python , Tips
In Python, you cannot access the elements of sets using indexing. If you try to change a set in place using the indexing operator [], you will raise the TypeError: ‘set’ object does not support item assignment.
This error can occur when incorrectly defining a dictionary without colons separating the keys and values.
If you intend to use a set, you can convert the set to a list, perform an index assignment then convert the list back to a tuple.
This tutorial will go through how to solve this error and solve it with the help of code examples.
Table of contents
Typeerror: ‘set’ object does not support item assignment.
Let’s break up the error message to understand what the error means. TypeError occurs whenever you attempt to use an illegal operation for a specific data type.
The part 'set' object tells us that the error concerns an illegal operation for sets.
The part does not support item assignment tells us that item assignment is the illegal operation we are attempting.
Sets are unordered objects which do not support indexing. You must use indexable container objects like lists to perform item assignment
Example #1: Assigning Items to Set
Let’s look at an example where we have a set of numbers and we want to replace the number 10 with the number 6 in the set using indexing.
Let’s run the code to see the result:
We throw the TypeError because the set object is indexable.
To solve this error, we need to convert the set to a list then perform the item assignment. We will then convert the list back to a set. However, you can leave the object as a list if you do not need a set. Let’s convert the list using the list() method:
The number 10 is the last element in the list. We can access this element using the indexing operator with the index -1 . Let’s look at the item assignment and the conversion back to a set:
Let’s run the code to get the result:
We successfully replaced the number 10 using item assignment.
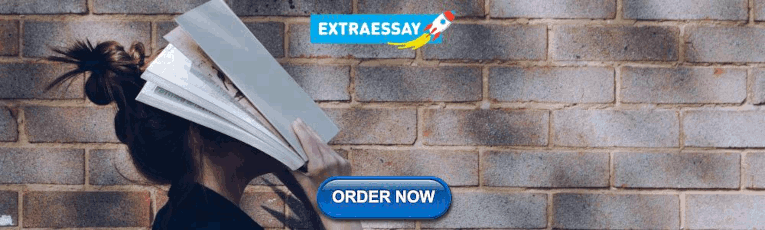
Example #2: Incorrectly Defining a Dictionary
The error can also occur when we try to create a dictionary but fail to use colons between the keys and the values. Let’s look at the difference between a set and a dictionary creation. In this example, want to create a dictionary where the keys are countries and the values are the capital city of each country:
We see that we set the capital of Switzerland set incorrectly to Zurich instead of Geneva . Let’s try to change the value of Switzerland using indexing:
We throw the error because we defined a set and not a dictionary. Let’s print the type of the capitals object:
We cannot index sets and therefore cannot perform item assignments.
To solve this error, we need to define a dictionary instead. The correct way to define a dictionary is to use curly brackets {} with each key-value pair having a colon between them. We will also verify the type of the object using a print statement:
Now we have a dictionary we can perform the item assignment to correct the capital city of Switzerland. Let’s look at the code:
Let’s run the code to see what happens:
We correctly updated the dictionary.
Congratulations on reading to the end of this tutorial. The TypeError: ‘set’ object does not support item assignment occurs when you try to change the elements of a set using indexing. The set data type is not indexable. To perform item assignment you should convert the set to a list, perform the item assignment then convert the list back to a set.
However, if you want to create a dictionary ensure that a colon is between every key and value and that a comma is separating each key-value pair.
For further reading on TypeErrors, go to the articles:
- How to Solve Python TypeError: ‘str’ object does not support item assignment
- How to Solve Python TypeError: ‘tuple’ object does not support item assignment
- How to Solve Python TypeError: ‘int’ object does not support item assignment
To learn more about Python for data science and machine learning, go to the online courses page on Python for the most comprehensive courses available.
Have fun and happy researching!
Share this:
- Click to share on Facebook (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Telegram (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Tumblr (Opens in new window)
Fix Python TypeError: 'str' object does not support item assignment
by Nathan Sebhastian
Posted on Jan 11, 2023
Reading time: 4 minutes
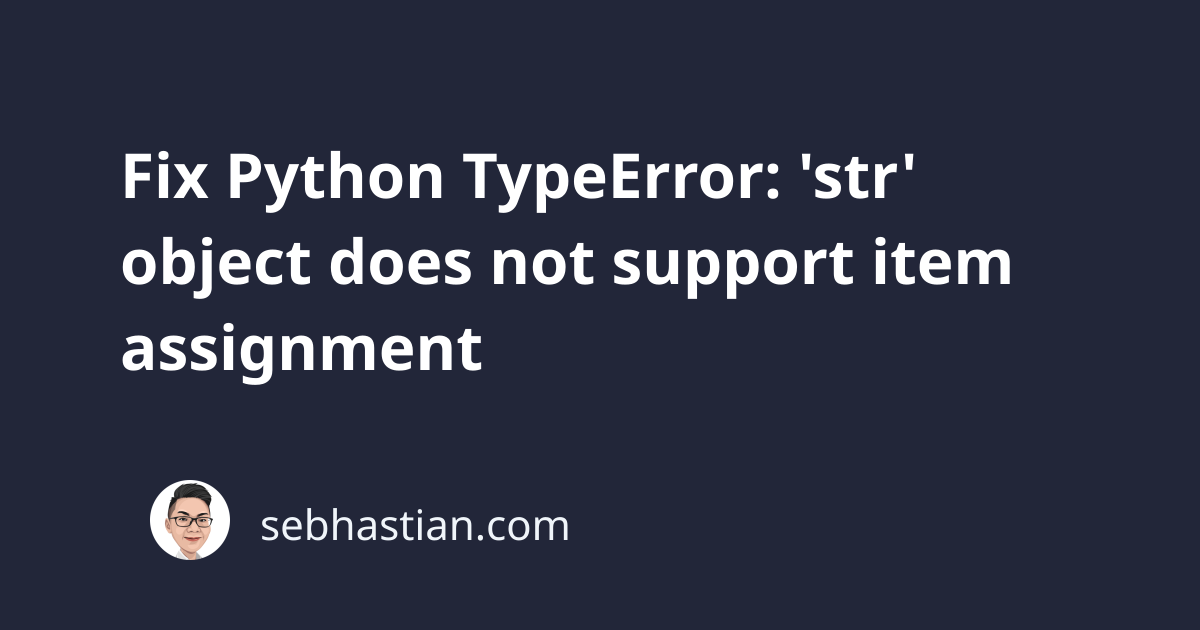
Python shows TypeError: 'str' object does not support item assignment error when you try to access and modify a string object using the square brackets ( [] ) notation.
To solve this error, use the replace() method to modify the string instead.
This error occurs because a string in Python is immutable, meaning you can’t change its value after it has been defined.
For example, suppose you want to replace the first character in your string as follows:
The code above attempts to replace the letter H with J by adding the index operator [0] .
But because assigning a new value to a string is not possible, Python responds with the following error:
To fix this error, you can create a new string with the desired modifications, instead of trying to modify the original string.
This can be done by calling the replace() method from the string. See the example below:
The replace() method allows you to replace all occurrences of a substring in your string.
This method accepts 3 parameters:
- old - the substring you want to replace
- new - the replacement for old value
- count - how many times to replace old (optional)
By default, the replace() method replaces all occurrences of the old string:
You can control how many times the replacement occurs by passing the third count parameter.
The code below replaces only the first occurrence of the old value:
And that’s how you can modify a string using the replace() method.
If you want more control over the modification, you can use a list.
Convert the string to a list first, then access the element you need to change as shown below:
After you modify the list element, merge the list back as a string by using the join() method.
This solution gives you more control as you can select the character you want to replace. You can replace the first, middle, or last occurrence of a specific character.
Another way you can modify a string is to use the string slicing and concatenation method.
Consider the two examples below:
In both examples, the string slicing operator is used to extract substrings of the old_str variable.
In the first example, the slice operator is used to extract the substring starting from index 1 to the end of the string with old_str[1:] and concatenates it with the character ‘J’ .
In the second example, the slice operator is used to extract the substring before index 7 with old_str[:7] and the substring after index 8 with old_str[8:] syntax.
Both substrings are joined together while putting the character x in the middle.
The examples show how you can use slicing to extract substrings and concatenate them to create new strings.
But using slicing and concatenation can be more confusing than using a list, so I would recommend you use a list unless you have a strong reason.
The Python error TypeError: 'str' object does not support item assignment occurs when you try to modify a string object using the subscript or index operator assignment.
This error happens because strings in Python are immutable and can’t be modified.
The solution is to create a new string with the required modifications. There are three ways you can do it:
- Use replace() method
- Convert the string to a list, apply the modifications, merge the list back to a string
- Use string slicing and concatenation to create a new string
Now you’ve learned how to modify a string in Python. Nice work!
Take your skills to the next level ⚡️
I'm sending out an occasional email with the latest tutorials on programming, web development, and statistics. Drop your email in the box below and I'll send new stuff straight into your inbox!
Hello! This website is dedicated to help you learn tech and data science skills with its step-by-step, beginner-friendly tutorials. Learn statistics, JavaScript and other programming languages using clear examples written for people.
Learn more about this website
Connect with me on Twitter
Or LinkedIn
Type the keyword below and hit enter
Click to see all tutorials tagged with:
CodeFatherTech
Learn to Code. Shape Your Future
Tuple Object Does Not Support Item Assignment. Why?
Have you ever seen the error “tuple object does not support item assignment” when working with tuples in Python? In this article we will learn why this error occurs and how to solve it.
The error “tuple object does not support item assignment” is raised in Python when you try to modify an element of a tuple. This error occurs because tuples are immutable data types. It’s possible to avoid this error by converting tuples to lists or by using the tuple slicing operator.
Let’s go through few examples that will show you in which circumstances this error occurs and what to do about it.
Let’s get started!
Explanation of the Error “Tuple Object Does Not Support Item Assignment”
Define a tuple called cities as shown below:
If you had a list you would be able to update any elements in the list .
But, here is what happens if we try to update one element of a tuple:
Tuples are immutable and that’s why we see this error.
There is a workaround to this, we can:
- Convert the tuple into a list.
- Update any elements in the list.
- Convert the final list back to a tuple.
To convert the tuple into a list we will use the list() function :
Now, let’s update the element at index 1 in the same way we have tried to do before with the tuple:
You can see that the second element of the list has been updated.
Finally, let’s convert the list back to a tuple using the tuple() function :
Makes sense?
Avoid the “Tuple Object Does Not Support Item Assignment” Error with Slicing
The slicing operator also allows to avoid this error.
Let’s see how we can use slicing to create a tuple from our original tuple where only one element is updated.
We will use the following tuple and we will update the value of the element at index 2 to ‘Rome’.
Here is the result we want:
We can use slicing and concatenate the first two elements of the original tuple, the new value and the last two elements of the original tuple.
Here is the generic syntax of the slicing operator (in this case applied to a tuple).
This takes a slice of the tuple including the element at index n and excluding the element at index m .
Firstly, let’s see how to print the first two and last two elements of the tuple using slicing…
First two elements
We can also omit the first zero considering that the slice starts from the beginning of the tuple.
Last two elements
Notice that we have omitted index m considering that the slice includes up to the last element of the tuple.
Now we can create the new tuple starting from the original one using the following code:
(‘Rome’,) is a tuple with one element of type string.
Does “Tuple Object Does Not Support Item Assignment” Apply to a List inside a Tuple?
Let’s see what happens when one of the elements of a tuple is a list.
If we try to update the second element of the tuple we get the expected error:
If we try to assign a new list to the third element…
…once again we get back the error “‘ tuple’ object does not support item assignment “.
But if we append another number to the list inside the tuple, here is what happens:
The Python interpreter doesn’t raise any exceptions because the list is a mutable data type.
This concept is important for you to know when you work with data types in Python:
In Python, lists are mutable and tuples are immutable.
How to Solve This Error with a List of Tuples
Do we see this error also with a list of tuples?
Let’s say we have a list of tuples that is used in a game to store name and score for each user:
The user John has gained additional points and I want to update the points associated to his user:
When I try to update his points we get back the same error we have seen before when updating a tuple.
How can we get around this error?
Tuples are immutable but lists are mutable and we could use this concept to assign the new score to a new tuple in the list, at the same position of the original tuple in the list.
So, instead of updating the tuple at index 0 we will assign a new tuple to it.
Let’s see if it works…
It does work! Once again because a list is mutable .
And here is how we can make this code more generic?
Ok, this is a bit more generic because we didn’t have to provide the name of the user when updating his records.
This is just an example to show you how to address this TypeError , but in reality in this scenario I would prefer to use a dictionary instead.
It would allow us to access the details of each user from the name and to update the score without any issues.
Tuple Object Does Not Support Item Assignment Error With Values Returned by a Function
This error can also occur when a function returns multiple values and you try to directly modify the values returned by the function.
I create a function that returns two values: the number of users registered in our application and the number of users who have accessed our application in the last 30 days.
As you can see the two values are returned by the function as a tuple.
So, let’s assume there is a new registered user and because of that I try to update the value returned by the function directly.
I get the following error…
This can happen especially if I know that two values are returned by the function but I’m not aware that they are returned in a tuple.
Why Using Tuples If We Get This Error?
You might be thinking…
What is the point of using tuples if we get this error every time we try to update them?
Wouldn’t be a lot easier to always use lists instead?
We can see the fact that tuples are immutable as an added value for tuples when we have some data in our application that should never be modified.
Let’s say, for example, that our application integrates with an external system and it needs some configuration properties to connect to that system.
The tuple above contains two values: the API endpoint of the system we connect to and the port for their API.
We want to make sure this configuration is not modified by mistake in our application because it would break the integration with the external system.
So, if our code inadvertently updates one of the values, the following happens:
Remember, it’s not always good to have data structures you can update in your code whenever you want.
In this article we have seen when the error “tuple object does not support item assignment” occurs and how to avoid it.
You have learned how differently the tuple and list data types behave in Python and how you can use that in your programs.
If you have any questions feel free to post them in the comment below 🙂
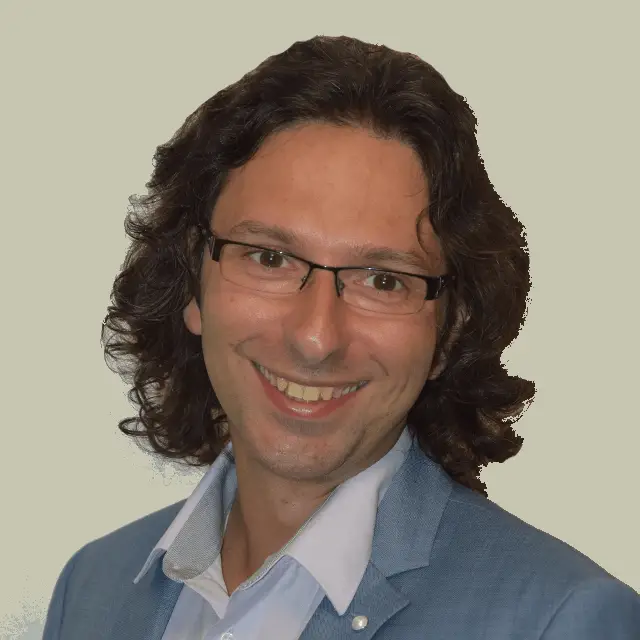
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute .
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
Related posts:
- Python TypeError: int object is not iterable: What To Do To Fix It?
- Python Unexpected EOF While Parsing: The Way To Fix It
- Python AttributeError: Fix This Built-in Exception
- Understand the “str object is not callable” Python Error and Fix It!
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.

- Privacy Overview
- Strictly Necessary Cookies
This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
Strictly Necessary Cookie should be enabled at all times so that we can save your preferences for cookie settings.
If you disable this cookie, we will not be able to save your preferences. This means that every time you visit this website you will need to enable or disable cookies again.
[Solved] TypeError: ‘str’ Object Does Not Support Item Assignment
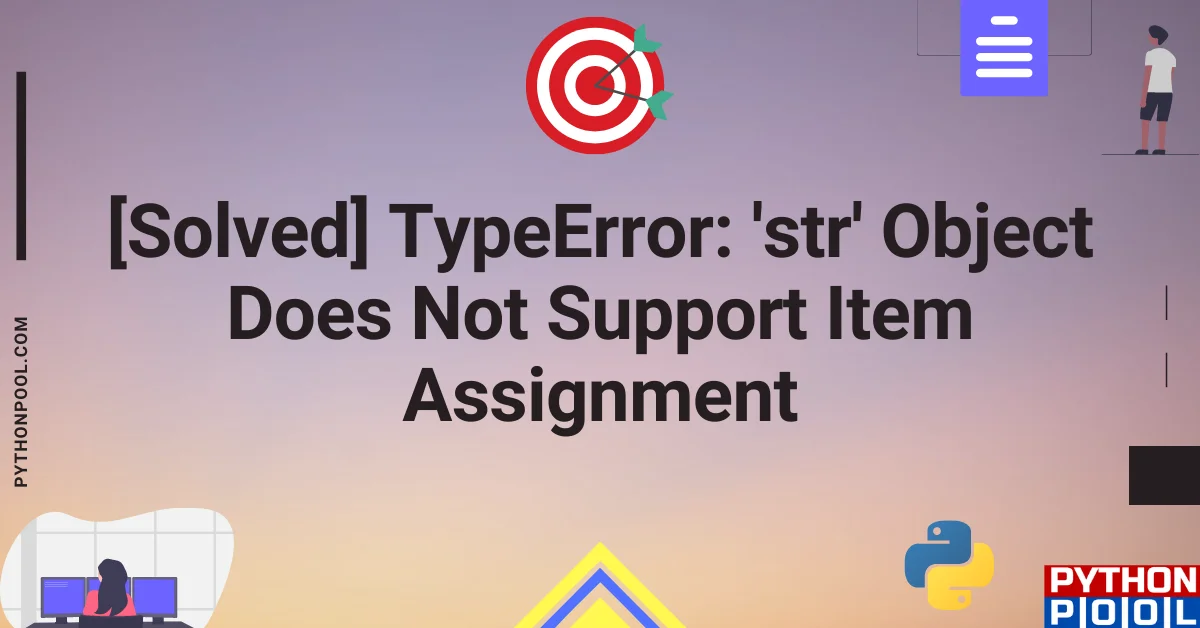
In this article, we will be discussing the TypeError:’str’ Object Does Not Support Item Assignment exception . We will also be going through solutions to this problem with example programs.
Why is This Error Raised?
When you attempt to change a character within a string using the assignment operator, you will receive the Python error TypeError: ‘str’ object does not support item assignment.
As we know, strings are immutable. If you attempt to change the content of a string, you will receive the error TypeError: ‘str’ object does not support item assignment .
There are four other similar variations based on immutable data types :
- TypeError: 'tuple' object does not support item assignment
- TypeError: 'int' object does not support item assignment
- TypeError: 'float' object does not support item assignment
- TypeError: 'bool' object does not support item assignment
Replacing String Characters using Assignment Operators
Replicate these errors yourself online to get a better idea here .
In this code, we will attempt to replace characters in a string.
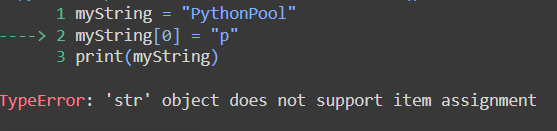
Strings are an immutable data type. However, we can change the memory to a different set of characters like so:
TypeError: ‘str’ Object Does Not Support Item Assignment in JSON
Let’s review the following code, which retrieves data from a JSON file.
In line 5, we are assigning data['sample'] to a string instead of an actual dictionary. This causes the interpreter to believe we are reassigning the value for an immutable string type.
TypeError: ‘str’ Object Does Not Support Item Assignment in PySpark
The following program reads files from a folder in a loop and creates data frames.
This occurs when a PySpark function is overwritten with a string. You can try directly importing the functions like so:
TypeError: ‘str’ Object Does Not Support Item Assignment in PyMongo
The following program writes decoded messages in a MongoDB collection. The decoded message is in a Python Dictionary.
At the 10th visible line, the variable x is converted as a string.
It’s better to use:
Please note that msg are a dictionary and NOT an object of context.
TypeError: ‘str’ Object Does Not Support Item Assignment in Random Shuffle
The below implementation takes an input main and the value is shuffled. The shuffled value is placed into Second .
random.shuffle is being called on a string, which is not supported. Convert the string type into a list and back to a string as an output in Second
TypeError: ‘str’ Object Does Not Support Item Assignment in Pandas Data Frame
The following program attempts to add a new column into the data frame
The iteration statement for dataset in df: loops through all the column names of “sample.csv”. To add an extra column, remove the iteration and simply pass dataset['Column'] = 1 .
![typeerror 'int' object does not support item assignment python dictionary [Solved] runtimeerror: cuda error: invalid device ordinal](https://www.pythonpool.com/wp-content/uploads/2024/01/Solved-runtimeerror-cuda-error-invalid-device-ordinal-300x157.webp)
These are the causes for TypeErrors : – Incompatible operations between 2 operands: – Passing a non-callable identifier – Incorrect list index type – Iterating a non-iterable identifier.
The data types that support item assignment are: – Lists – Dictionaries – and Sets These data types are mutable and support item assignment
As we know, TypeErrors occur due to unsupported operations between operands. To avoid facing such errors, we must: – Learn Proper Python syntax for all Data Types. – Establish the mutable and immutable Data Types. – Figure how list indexing works and other data types that support indexing. – Explore how function calls work in Python and various ways to call a function. – Establish the difference between an iterable and non-iterable identifier. – Learn the properties of Python Data Types.
We have looked at various error cases in TypeError:’str’ Object Does Not Support Item Assignment. Solutions for these cases have been provided. We have also mentioned similar variations of this exception.
Trending Python Articles
![typeerror 'int' object does not support item assignment python dictionary [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
- Python »
- 3.14.0a0 Documentation »
- What’s New in Python »
- What’s New In Python 3.0
- Theme Auto Light Dark |
What’s New In Python 3.0 ¶
Guido van Rossum
This article explains the new features in Python 3.0, compared to 2.6. Python 3.0, also known as “Python 3000” or “Py3K”, is the first ever intentionally backwards incompatible Python release. Python 3.0 was released on December 3, 2008. There are more changes than in a typical release, and more that are important for all Python users. Nevertheless, after digesting the changes, you’ll find that Python really hasn’t changed all that much – by and large, we’re mostly fixing well-known annoyances and warts, and removing a lot of old cruft.
This article doesn’t attempt to provide a complete specification of all new features, but instead tries to give a convenient overview. For full details, you should refer to the documentation for Python 3.0, and/or the many PEPs referenced in the text. If you want to understand the complete implementation and design rationale for a particular feature, PEPs usually have more details than the regular documentation; but note that PEPs usually are not kept up-to-date once a feature has been fully implemented.
Due to time constraints this document is not as complete as it should have been. As always for a new release, the Misc/NEWS file in the source distribution contains a wealth of detailed information about every small thing that was changed.
Common Stumbling Blocks ¶
This section lists those few changes that are most likely to trip you up if you’re used to Python 2.5.
Print Is A Function ¶
The print statement has been replaced with a print() function, with keyword arguments to replace most of the special syntax of the old print statement ( PEP 3105 ). Examples:
You can also customize the separator between items, e.g.:
which produces:
The print() function doesn’t support the “softspace” feature of the old print statement. For example, in Python 2.x, print "A\n", "B" would write "A\nB\n" ; but in Python 3.0, print("A\n", "B") writes "A\n B\n" .
Initially, you’ll be finding yourself typing the old print x a lot in interactive mode. Time to retrain your fingers to type print(x) instead!
When using the 2to3 source-to-source conversion tool, all print statements are automatically converted to print() function calls, so this is mostly a non-issue for larger projects.
Views And Iterators Instead Of Lists ¶
Some well-known APIs no longer return lists:
dict methods dict.keys() , dict.items() and dict.values() return “views” instead of lists. For example, this no longer works: k = d.keys(); k.sort() . Use k = sorted(d) instead (this works in Python 2.5 too and is just as efficient).
Also, the dict.iterkeys() , dict.iteritems() and dict.itervalues() methods are no longer supported.
map() and filter() return iterators. If you really need a list and the input sequences are all of equal length, a quick fix is to wrap map() in list() , e.g. list(map(...)) , but a better fix is often to use a list comprehension (especially when the original code uses lambda ), or rewriting the code so it doesn’t need a list at all. Particularly tricky is map() invoked for the side effects of the function; the correct transformation is to use a regular for loop (since creating a list would just be wasteful).
If the input sequences are not of equal length, map() will stop at the termination of the shortest of the sequences. For full compatibility with map() from Python 2.x, also wrap the sequences in itertools.zip_longest() , e.g. map(func, *sequences) becomes list(map(func, itertools.zip_longest(*sequences))) .
range() now behaves like xrange() used to behave, except it works with values of arbitrary size. The latter no longer exists.
zip() now returns an iterator.
Ordering Comparisons ¶
Python 3.0 has simplified the rules for ordering comparisons:
The ordering comparison operators ( < , <= , >= , > ) raise a TypeError exception when the operands don’t have a meaningful natural ordering. Thus, expressions like 1 < '' , 0 > None or len <= len are no longer valid, and e.g. None < None raises TypeError instead of returning False . A corollary is that sorting a heterogeneous list no longer makes sense – all the elements must be comparable to each other. Note that this does not apply to the == and != operators: objects of different incomparable types always compare unequal to each other.
builtin.sorted() and list.sort() no longer accept the cmp argument providing a comparison function. Use the key argument instead. N.B. the key and reverse arguments are now “keyword-only”.
The cmp() function should be treated as gone, and the __cmp__() special method is no longer supported. Use __lt__() for sorting, __eq__() with __hash__() , and other rich comparisons as needed. (If you really need the cmp() functionality, you could use the expression (a > b) - (a < b) as the equivalent for cmp(a, b) .)
PEP 237 : Essentially, long renamed to int . That is, there is only one built-in integral type, named int ; but it behaves mostly like the old long type.
PEP 238 : An expression like 1/2 returns a float. Use 1//2 to get the truncating behavior. (The latter syntax has existed for years, at least since Python 2.2.)
The sys.maxint constant was removed, since there is no longer a limit to the value of integers. However, sys.maxsize can be used as an integer larger than any practical list or string index. It conforms to the implementation’s “natural” integer size and is typically the same as sys.maxint in previous releases on the same platform (assuming the same build options).
The repr() of a long integer doesn’t include the trailing L anymore, so code that unconditionally strips that character will chop off the last digit instead. (Use str() instead.)
Octal literals are no longer of the form 0720 ; use 0o720 instead.
Text Vs. Data Instead Of Unicode Vs. 8-bit ¶
Everything you thought you knew about binary data and Unicode has changed.
Python 3.0 uses the concepts of text and (binary) data instead of Unicode strings and 8-bit strings. All text is Unicode; however encoded Unicode is represented as binary data. The type used to hold text is str , the type used to hold data is bytes . The biggest difference with the 2.x situation is that any attempt to mix text and data in Python 3.0 raises TypeError , whereas if you were to mix Unicode and 8-bit strings in Python 2.x, it would work if the 8-bit string happened to contain only 7-bit (ASCII) bytes, but you would get UnicodeDecodeError if it contained non-ASCII values. This value-specific behavior has caused numerous sad faces over the years.
As a consequence of this change in philosophy, pretty much all code that uses Unicode, encodings or binary data most likely has to change. The change is for the better, as in the 2.x world there were numerous bugs having to do with mixing encoded and unencoded text. To be prepared in Python 2.x, start using unicode for all unencoded text, and str for binary or encoded data only. Then the 2to3 tool will do most of the work for you.
You can no longer use u"..." literals for Unicode text. However, you must use b"..." literals for binary data.
As the str and bytes types cannot be mixed, you must always explicitly convert between them. Use str.encode() to go from str to bytes , and bytes.decode() to go from bytes to str . You can also use bytes(s, encoding=...) and str(b, encoding=...) , respectively.
Like str , the bytes type is immutable. There is a separate mutable type to hold buffered binary data, bytearray . Nearly all APIs that accept bytes also accept bytearray . The mutable API is based on collections.MutableSequence .
All backslashes in raw string literals are interpreted literally. This means that '\U' and '\u' escapes in raw strings are not treated specially. For example, r'\u20ac' is a string of 6 characters in Python 3.0, whereas in 2.6, ur'\u20ac' was the single “euro” character. (Of course, this change only affects raw string literals; the euro character is '\u20ac' in Python 3.0.)
The built-in basestring abstract type was removed. Use str instead. The str and bytes types don’t have functionality enough in common to warrant a shared base class. The 2to3 tool (see below) replaces every occurrence of basestring with str .
Files opened as text files (still the default mode for open() ) always use an encoding to map between strings (in memory) and bytes (on disk). Binary files (opened with a b in the mode argument) always use bytes in memory. This means that if a file is opened using an incorrect mode or encoding, I/O will likely fail loudly, instead of silently producing incorrect data. It also means that even Unix users will have to specify the correct mode (text or binary) when opening a file. There is a platform-dependent default encoding, which on Unixy platforms can be set with the LANG environment variable (and sometimes also with some other platform-specific locale-related environment variables). In many cases, but not all, the system default is UTF-8; you should never count on this default. Any application reading or writing more than pure ASCII text should probably have a way to override the encoding. There is no longer any need for using the encoding-aware streams in the codecs module.
The initial values of sys.stdin , sys.stdout and sys.stderr are now unicode-only text files (i.e., they are instances of io.TextIOBase ). To read and write bytes data with these streams, you need to use their io.TextIOBase.buffer attribute.
Filenames are passed to and returned from APIs as (Unicode) strings. This can present platform-specific problems because on some platforms filenames are arbitrary byte strings. (On the other hand, on Windows filenames are natively stored as Unicode.) As a work-around, most APIs (e.g. open() and many functions in the os module) that take filenames accept bytes objects as well as strings, and a few APIs have a way to ask for a bytes return value. Thus, os.listdir() returns a list of bytes instances if the argument is a bytes instance, and os.getcwdb() returns the current working directory as a bytes instance. Note that when os.listdir() returns a list of strings, filenames that cannot be decoded properly are omitted rather than raising UnicodeError .
Some system APIs like os.environ and sys.argv can also present problems when the bytes made available by the system is not interpretable using the default encoding. Setting the LANG variable and rerunning the program is probably the best approach.
PEP 3138 : The repr() of a string no longer escapes non-ASCII characters. It still escapes control characters and code points with non-printable status in the Unicode standard, however.
PEP 3120 : The default source encoding is now UTF-8.
PEP 3131 : Non-ASCII letters are now allowed in identifiers. (However, the standard library remains ASCII-only with the exception of contributor names in comments.)
The StringIO and cStringIO modules are gone. Instead, import the io module and use io.StringIO or io.BytesIO for text and data respectively.
See also the Unicode HOWTO , which was updated for Python 3.0.
Overview Of Syntax Changes ¶
This section gives a brief overview of every syntactic change in Python 3.0.
New Syntax ¶
PEP 3107 : Function argument and return value annotations. This provides a standardized way of annotating a function’s parameters and return value. There are no semantics attached to such annotations except that they can be introspected at runtime using the __annotations__ attribute. The intent is to encourage experimentation through metaclasses, decorators or frameworks.
PEP 3102 : Keyword-only arguments. Named parameters occurring after *args in the parameter list must be specified using keyword syntax in the call. You can also use a bare * in the parameter list to indicate that you don’t accept a variable-length argument list, but you do have keyword-only arguments.
Keyword arguments are allowed after the list of base classes in a class definition. This is used by the new convention for specifying a metaclass (see next section), but can be used for other purposes as well, as long as the metaclass supports it.
PEP 3104 : nonlocal statement. Using nonlocal x you can now assign directly to a variable in an outer (but non-global) scope. nonlocal is a new reserved word.
PEP 3132 : Extended Iterable Unpacking. You can now write things like a, b, *rest = some_sequence . And even *rest, a = stuff . The rest object is always a (possibly empty) list; the right-hand side may be any iterable. Example:
This sets a to 0 , b to 4 , and rest to [1, 2, 3] .
Dictionary comprehensions: {k: v for k, v in stuff} means the same thing as dict(stuff) but is more flexible. (This is PEP 274 vindicated. :-)
Set literals, e.g. {1, 2} . Note that {} is an empty dictionary; use set() for an empty set. Set comprehensions are also supported; e.g., {x for x in stuff} means the same thing as set(stuff) but is more flexible.
New octal literals, e.g. 0o720 (already in 2.6). The old octal literals ( 0720 ) are gone.
New binary literals, e.g. 0b1010 (already in 2.6), and there is a new corresponding built-in function, bin() .
Bytes literals are introduced with a leading b or B , and there is a new corresponding built-in function, bytes() .
Changed Syntax ¶
PEP 3109 and PEP 3134 : new raise statement syntax: raise [ expr [from expr ]] . See below.
as and with are now reserved words. (Since 2.6, actually.)
True , False , and None are reserved words. (2.6 partially enforced the restrictions on None already.)
Change from except exc , var to except exc as var . See PEP 3110 .
PEP 3115 : New Metaclass Syntax. Instead of:
you must now use:
The module-global __metaclass__ variable is no longer supported. (It was a crutch to make it easier to default to new-style classes without deriving every class from object .)
List comprehensions no longer support the syntactic form [... for var in item1 , item2 , ...] . Use [... for var in ( item1 , item2 , ...)] instead. Also note that list comprehensions have different semantics: they are closer to syntactic sugar for a generator expression inside a list() constructor, and in particular the loop control variables are no longer leaked into the surrounding scope.
The ellipsis ( ... ) can be used as an atomic expression anywhere. (Previously it was only allowed in slices.) Also, it must now be spelled as ... . (Previously it could also be spelled as . . . , by a mere accident of the grammar.)
Removed Syntax ¶
PEP 3113 : Tuple parameter unpacking removed. You can no longer write def foo(a, (b, c)): ... . Use def foo(a, b_c): b, c = b_c instead.
Removed backticks (use repr() instead).
Removed <> (use != instead).
Removed keyword: exec() is no longer a keyword; it remains as a function. (Fortunately the function syntax was also accepted in 2.x.) Also note that exec() no longer takes a stream argument; instead of exec(f) you can use exec(f.read()) .
Integer literals no longer support a trailing l or L .
String literals no longer support a leading u or U .
The from module import * syntax is only allowed at the module level, no longer inside functions.
The only acceptable syntax for relative imports is from .[ module ] import name . All import forms not starting with . are interpreted as absolute imports. ( PEP 328 )
Classic classes are gone.
Changes Already Present In Python 2.6 ¶
Since many users presumably make the jump straight from Python 2.5 to Python 3.0, this section reminds the reader of new features that were originally designed for Python 3.0 but that were back-ported to Python 2.6. The corresponding sections in What’s New in Python 2.6 should be consulted for longer descriptions.
PEP 343: The ‘with’ statement . The with statement is now a standard feature and no longer needs to be imported from the __future__ . Also check out Writing Context Managers and The contextlib module .
PEP 366: Explicit Relative Imports From a Main Module . This enhances the usefulness of the -m option when the referenced module lives in a package.
PEP 370: Per-user site-packages Directory .
PEP 371: The multiprocessing Package .
PEP 3101: Advanced String Formatting . Note: the 2.6 description mentions the format() method for both 8-bit and Unicode strings. In 3.0, only the str type (text strings with Unicode support) supports this method; the bytes type does not. The plan is to eventually make this the only API for string formatting, and to start deprecating the % operator in Python 3.1.
PEP 3105: print As a Function . This is now a standard feature and no longer needs to be imported from __future__ . More details were given above.
PEP 3110: Exception-Handling Changes . The except exc as var syntax is now standard and except exc , var is no longer supported. (Of course, the as var part is still optional.)
PEP 3112: Byte Literals . The b"..." string literal notation (and its variants like b'...' , b"""...""" , and br"..." ) now produces a literal of type bytes .
PEP 3116: New I/O Library . The io module is now the standard way of doing file I/O. The built-in open() function is now an alias for io.open() and has additional keyword arguments encoding , errors , newline and closefd . Also note that an invalid mode argument now raises ValueError , not IOError . The binary file object underlying a text file object can be accessed as f.buffer (but beware that the text object maintains a buffer of itself in order to speed up the encoding and decoding operations).
PEP 3118: Revised Buffer Protocol . The old builtin buffer() is now really gone; the new builtin memoryview() provides (mostly) similar functionality.
PEP 3119: Abstract Base Classes . The abc module and the ABCs defined in the collections module plays a somewhat more prominent role in the language now, and built-in collection types like dict and list conform to the collections.MutableMapping and collections.MutableSequence ABCs, respectively.
PEP 3127: Integer Literal Support and Syntax . As mentioned above, the new octal literal notation is the only one supported, and binary literals have been added.
PEP 3129: Class Decorators .
PEP 3141: A Type Hierarchy for Numbers . The numbers module is another new use of ABCs, defining Python’s “numeric tower”. Also note the new fractions module which implements numbers.Rational .
Library Changes ¶
Due to time constraints, this document does not exhaustively cover the very extensive changes to the standard library. PEP 3108 is the reference for the major changes to the library. Here’s a capsule review:
Many old modules were removed. Some, like gopherlib (no longer used) and md5 (replaced by hashlib ), were already deprecated by PEP 4 . Others were removed as a result of the removal of support for various platforms such as Irix, BeOS and Mac OS 9 (see PEP 11 ). Some modules were also selected for removal in Python 3.0 due to lack of use or because a better replacement exists. See PEP 3108 for an exhaustive list.
The bsddb3 package was removed because its presence in the core standard library has proved over time to be a particular burden for the core developers due to testing instability and Berkeley DB’s release schedule. However, the package is alive and well, externally maintained at https://www.jcea.es/programacion/pybsddb.htm .
Some modules were renamed because their old name disobeyed PEP 8 , or for various other reasons. Here’s the list:
A common pattern in Python 2.x is to have one version of a module implemented in pure Python, with an optional accelerated version implemented as a C extension; for example, pickle and cPickle . This places the burden of importing the accelerated version and falling back on the pure Python version on each user of these modules. In Python 3.0, the accelerated versions are considered implementation details of the pure Python versions. Users should always import the standard version, which attempts to import the accelerated version and falls back to the pure Python version. The pickle / cPickle pair received this treatment. The profile module is on the list for 3.1. The StringIO module has been turned into a class in the io module.
Some related modules have been grouped into packages, and usually the submodule names have been simplified. The resulting new packages are:
dbm ( anydbm , dbhash , dbm , dumbdbm , gdbm , whichdb ).
html ( HTMLParser , htmlentitydefs ).
http ( httplib , BaseHTTPServer , CGIHTTPServer , SimpleHTTPServer , Cookie , cookielib ).
tkinter (all Tkinter -related modules except turtle ). The target audience of turtle doesn’t really care about tkinter . Also note that as of Python 2.6, the functionality of turtle has been greatly enhanced.
urllib ( urllib , urllib2 , urlparse , robotparse ).
xmlrpc ( xmlrpclib , DocXMLRPCServer , SimpleXMLRPCServer ).
Some other changes to standard library modules, not covered by PEP 3108 :
Killed sets . Use the built-in set() class.
Cleanup of the sys module: removed sys.exitfunc() , sys.exc_clear() , sys.exc_type , sys.exc_value , sys.exc_traceback . (Note that sys.last_type etc. remain.)
Cleanup of the array.array type: the read() and write() methods are gone; use fromfile() and tofile() instead. Also, the 'c' typecode for array is gone – use either 'b' for bytes or 'u' for Unicode characters.
Cleanup of the operator module: removed sequenceIncludes() and isCallable() .
Cleanup of the thread module: acquire_lock() and release_lock() are gone; use acquire() and release() instead.
Cleanup of the random module: removed the jumpahead() API.
The new module is gone.
The functions os.tmpnam() , os.tempnam() and os.tmpfile() have been removed in favor of the tempfile module.
The tokenize module has been changed to work with bytes. The main entry point is now tokenize.tokenize() , instead of generate_tokens.
string.letters and its friends ( string.lowercase and string.uppercase ) are gone. Use string.ascii_letters etc. instead. (The reason for the removal is that string.letters and friends had locale-specific behavior, which is a bad idea for such attractively named global “constants”.)
Renamed module __builtin__ to builtins (removing the underscores, adding an ‘s’). The __builtins__ variable found in most global namespaces is unchanged. To modify a builtin, you should use builtins , not __builtins__ !
PEP 3101 : A New Approach To String Formatting ¶
A new system for built-in string formatting operations replaces the % string formatting operator. (However, the % operator is still supported; it will be deprecated in Python 3.1 and removed from the language at some later time.) Read PEP 3101 for the full scoop.
Changes To Exceptions ¶
The APIs for raising and catching exception have been cleaned up and new powerful features added:
PEP 352 : All exceptions must be derived (directly or indirectly) from BaseException . This is the root of the exception hierarchy. This is not new as a recommendation, but the requirement to inherit from BaseException is new. (Python 2.6 still allowed classic classes to be raised, and placed no restriction on what you can catch.) As a consequence, string exceptions are finally truly and utterly dead.
Almost all exceptions should actually derive from Exception ; BaseException should only be used as a base class for exceptions that should only be handled at the top level, such as SystemExit or KeyboardInterrupt . The recommended idiom for handling all exceptions except for this latter category is to use except Exception .
StandardError was removed.
Exceptions no longer behave as sequences. Use the args attribute instead.
PEP 3109 : Raising exceptions. You must now use raise Exception ( args ) instead of raise Exception , args . Additionally, you can no longer explicitly specify a traceback; instead, if you have to do this, you can assign directly to the __traceback__ attribute (see below).
PEP 3110 : Catching exceptions. You must now use except SomeException as variable instead of except SomeException , variable . Moreover, the variable is explicitly deleted when the except block is left.
PEP 3134 : Exception chaining. There are two cases: implicit chaining and explicit chaining. Implicit chaining happens when an exception is raised in an except or finally handler block. This usually happens due to a bug in the handler block; we call this a secondary exception. In this case, the original exception (that was being handled) is saved as the __context__ attribute of the secondary exception. Explicit chaining is invoked with this syntax:
(where primary_exception is any expression that produces an exception object, probably an exception that was previously caught). In this case, the primary exception is stored on the __cause__ attribute of the secondary exception. The traceback printed when an unhandled exception occurs walks the chain of __cause__ and __context__ attributes and prints a separate traceback for each component of the chain, with the primary exception at the top. (Java users may recognize this behavior.)
PEP 3134 : Exception objects now store their traceback as the __traceback__ attribute. This means that an exception object now contains all the information pertaining to an exception, and there are fewer reasons to use sys.exc_info() (though the latter is not removed).
A few exception messages are improved when Windows fails to load an extension module. For example, error code 193 is now %1 is not a valid Win32 application . Strings now deal with non-English locales.
Miscellaneous Other Changes ¶
Operators and special methods ¶.
!= now returns the opposite of == , unless == returns NotImplemented .
The concept of “unbound methods” has been removed from the language. When referencing a method as a class attribute, you now get a plain function object.
__getslice__() , __setslice__() and __delslice__() were killed. The syntax a[i:j] now translates to a.__getitem__(slice(i, j)) (or __setitem__() or __delitem__() , when used as an assignment or deletion target, respectively).
PEP 3114 : the standard next() method has been renamed to __next__() .
The __oct__() and __hex__() special methods are removed – oct() and hex() use __index__() now to convert the argument to an integer.
Removed support for __members__ and __methods__ .
The function attributes named func_X have been renamed to use the __X__ form, freeing up these names in the function attribute namespace for user-defined attributes. To wit, func_closure , func_code , func_defaults , func_dict , func_doc , func_globals , func_name were renamed to __closure__ , __code__ , __defaults__ , __dict__ , __doc__ , __globals__ , __name__ , respectively.
__nonzero__() is now __bool__() .
PEP 3135 : New super() . You can now invoke super() without arguments and (assuming this is in a regular instance method defined inside a class statement) the right class and instance will automatically be chosen. With arguments, the behavior of super() is unchanged.
PEP 3111 : raw_input() was renamed to input() . That is, the new input() function reads a line from sys.stdin and returns it with the trailing newline stripped. It raises EOFError if the input is terminated prematurely. To get the old behavior of input() , use eval(input()) .
A new built-in function next() was added to call the __next__() method on an object.
The round() function rounding strategy and return type have changed. Exact halfway cases are now rounded to the nearest even result instead of away from zero. (For example, round(2.5) now returns 2 rather than 3 .) round(x[, n]) now delegates to x.__round__([n]) instead of always returning a float. It generally returns an integer when called with a single argument and a value of the same type as x when called with two arguments.
Moved intern() to sys.intern() .
Removed: apply() . Instead of apply(f, args) use f(*args) .
Removed callable() . Instead of callable(f) you can use isinstance(f, collections.Callable) . The operator.isCallable() function is also gone.
Removed coerce() . This function no longer serves a purpose now that classic classes are gone.
Removed execfile() . Instead of execfile(fn) use exec(open(fn).read()) .
Removed the file type. Use open() . There are now several different kinds of streams that open can return in the io module.
Removed reduce() . Use functools.reduce() if you really need it; however, 99 percent of the time an explicit for loop is more readable.
Removed reload() . Use imp.reload() .
Removed. dict.has_key() – use the in operator instead.
Build and C API Changes ¶
Due to time constraints, here is a very incomplete list of changes to the C API.
Support for several platforms was dropped, including but not limited to Mac OS 9, BeOS, RISCOS, Irix, and Tru64.
PEP 3118 : New Buffer API.
PEP 3121 : Extension Module Initialization & Finalization.
PEP 3123 : Making PyObject_HEAD conform to standard C.
No more C API support for restricted execution.
PyNumber_Coerce() , PyNumber_CoerceEx() , PyMember_Get() , and PyMember_Set() C APIs are removed.
New C API PyImport_ImportModuleNoBlock() , works like PyImport_ImportModule() but won’t block on the import lock (returning an error instead).
Renamed the boolean conversion C-level slot and method: nb_nonzero is now nb_bool .
Removed METH_OLDARGS and WITH_CYCLE_GC from the C API.
Performance ¶
The net result of the 3.0 generalizations is that Python 3.0 runs the pystone benchmark around 10% slower than Python 2.5. Most likely the biggest cause is the removal of special-casing for small integers. There’s room for improvement, but it will happen after 3.0 is released!
Porting To Python 3.0 ¶
For porting existing Python 2.5 or 2.6 source code to Python 3.0, the best strategy is the following:
(Prerequisite:) Start with excellent test coverage.
Port to Python 2.6. This should be no more work than the average port from Python 2.x to Python 2.(x+1). Make sure all your tests pass.
(Still using 2.6:) Turn on the -3 command line switch. This enables warnings about features that will be removed (or change) in 3.0. Run your test suite again, and fix code that you get warnings about until there are no warnings left, and all your tests still pass.
Run the 2to3 source-to-source translator over your source code tree. Run the result of the translation under Python 3.0. Manually fix up any remaining issues, fixing problems until all tests pass again.
It is not recommended to try to write source code that runs unchanged under both Python 2.6 and 3.0; you’d have to use a very contorted coding style, e.g. avoiding print statements, metaclasses, and much more. If you are maintaining a library that needs to support both Python 2.6 and Python 3.0, the best approach is to modify step 3 above by editing the 2.6 version of the source code and running the 2to3 translator again, rather than editing the 3.0 version of the source code.
For porting C extensions to Python 3.0, please see Porting Extension Modules to Python 3 .
Table of Contents
- Print Is A Function
- Views And Iterators Instead Of Lists
- Ordering Comparisons
- Text Vs. Data Instead Of Unicode Vs. 8-bit
- Changed Syntax
- Removed Syntax
- Changes Already Present In Python 2.6
- Library Changes
- PEP 3101 : A New Approach To String Formatting
- Changes To Exceptions
- Operators And Special Methods
- Build and C API Changes
- Performance
- Porting To Python 3.0
Previous topic
What’s New In Python 3.1
What’s New in Python 2.7
- Report a Bug
- Show Source
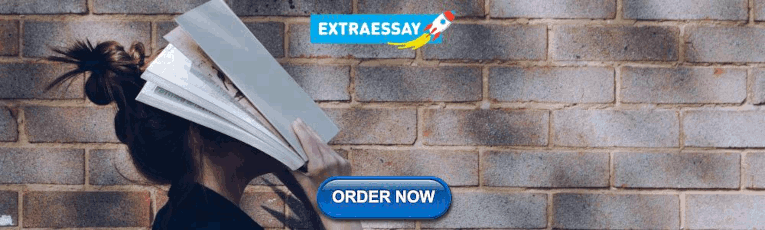
IMAGES
VIDEO
COMMENTS
array[0][0] = 0*0 >> TypeError: 'int' object does not support item assignment Since array[0] is an integer, you can't use the second [0]. There is nothing there to get. So, like Ashalynd said, the array = x*y seems to be the problem. Depending on what you really want to do, there could be many solutions.
However, tuples are immutable, and you cannot perform such an assignment: >>> x[0] = 0 Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: 'tuple' object does not support item assignment You can fix this issue by using a list instead.
How to Solve Python TypeError: 'str' object does not support item assignment; How to Solve Python TypeError: 'tuple' object does not support item assignment; To learn more about Python for data science and machine learning, go to the online courses page on Python for the most comprehensive courses available. Have fun and happy researching!
That's not valid. A dictionary is a collection of unique keys, each of which may have an associated value. What you have shown is a dictionary with non-unique keys (the key 1 is shown multiple times). As your keys are all numeric, you could go with either a nested list or a nested dict, but the data would look a bit different.
#pythonforbeginners Learn how to solve the common Python error "TypeError 'int' object does not support item assignment" with practical examples and step-by-...
Here are the most common root causes of "int object does not support item assignment", which include the following: Attempting to modify an integer directly. Using an integer where a sequence is expected. Not converting integer objects to mutable data types before modifying them. Using an integer as a dictionary key.
In this video, we delve into the common Python error message: 'TypeError: 'int' object does not support item assignment.' Learn why this error occurs, how to...
I have a raster layer of Type Float32, and another raster output of Type Integer created from this raster layer outside the python code. I want to scan every column wise, and pick up the Float raster minimum value location within a 5 neighbourhood of Integer raster value of 1 in every column and assign the value 1 at this minimum value location ...
If the variable stores a None value, we set it to an empty dictionary. # Track down where the variable got assigned a None value You have to figure out where the variable got assigned a None value in your code and correct the assignment to a list or a dictionary.. The most common sources of None values are:. Having a function that doesn't return anything (returns None implicitly).
We accessed the first nested array (index 0) and then updated the value of the first item in the nested array.. Python indexes are zero-based, so the first item in a list has an index of 0, and the last item has an index of -1 or len(a_list) - 1. # Checking what type a variable stores The Python "TypeError: 'float' object does not support item assignment" is caused when we try to mutate the ...
That means, for example, you might be concatenating a string with an integer. In this article, I will show you why the TypeError: builtin_function_or_method object is not subscriptable occurs and how you can fix it. Why The TypeError: builtin_function_or_method object is not subscriptable Occurs
In order to fix it, one need to know what expected output is. Currently that One is just you. You initialized app as an integer in app=0. Later in the code you called app in app [i], which you can only do with list items and dictionaries, not integers. I'm not 100% sure what you were trying to do, but if you want to create a list of numbers ...
Yeah, you cannot assign a string to a variable, and then modify the string, but you can use the string to create a new one and assign that result to the same variable. Borrowing some code from @BowlOfRed above, you can do this: s = "foobar". s = s[:3] + "j" + s[4:] print(s)
The TypeError: 'set' object does not support item assignment occurs when you try to change the elements of a set using indexing. The set data type is not indexable. To perform item assignment you should convert the set to a list, perform the item assignment then convert the list back to a set.
greet[0] = 'J'. TypeError: 'str' object does not support item assignment. To fix this error, you can create a new string with the desired modifications, instead of trying to modify the original string. This can be done by calling the replace() method from the string. See the example below: old_str = 'Hello, world!'.
>>> ext_system_config[0] = 'incorrect_value' Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: 'tuple' object does not support item assignment . Remember, it's not always good to have data structures you can update in your code whenever you want. Conclusion
Lot of issues here, I'll try to go through them one by one. The data structure dict = {} Not only is this overwriting python's dict, (see mgilson's comment) but this is the wrong data structure for the project.You should use a list instead (or a set if you have unique unordered values)
A module object has a namespace implemented by a dictionary object (this is the dictionary referenced by the __globals__ attribute of functions defined in the module). Attribute references are translated to lookups in this dictionary, e.g., m.x is equivalent to m.__dict__["x"]. A module object does not contain the code object used to initialize ...
This is the line that's causing the error, at any rate. dict is a type. You have to create a dictionary before you set keys on it, you can't just set keys on the type's class. Don't use "dict" as var_name. Then you can use it.
read_dict (dictionary, source = '<dict>') ¶ Load configuration from any object that provides a dict-like items() method. Keys are section names, values are dictionaries with keys and values that should be present in the section. If the used dictionary type preserves order, sections and their keys will be added in order.
Check in your code for assignment to dictionary. The variable dictionary isn't a dictionary, but a string, so somewhere in your code will be something like: dictionary = " evaluates to string "
TypeError: 'str' object does not support item assignment Solution. The iteration statement for dataset in df: loops through all the column names of "sample.csv". To add an extra column, remove the iteration and simply pass dataset['Column'] = 1.
5. Strings in Python are immutable (you cannot change them inplace). What you are trying to do can be done in many ways: Copy the string: foo = 'Hello'. bar = foo. Create a new string by joining all characters of the old string: new_string = ''.join(c for c in oldstring) Slice and copy:
Note: The print() function doesn't support the "softspace" feature of the old print statement. For example, in Python 2.x, print "A\n", "B" would write "A\nB\n"; but in Python 3.0, print("A\n", "B") writes "A\n B\n". Initially, you'll be finding yourself typing the old print x a lot in interactive mode. Time to retrain your fingers to type print(x) instead!