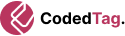
- Conditional Operators
Conditional Assignment Operator in PHP is a shorthand operator that allow developers to assign values to variables based on certain conditions.
In this article, we will explore how the various Conditional Assignment Operators in PHP simplify code and make it more readable.
Let’s begin with the ternary operator.
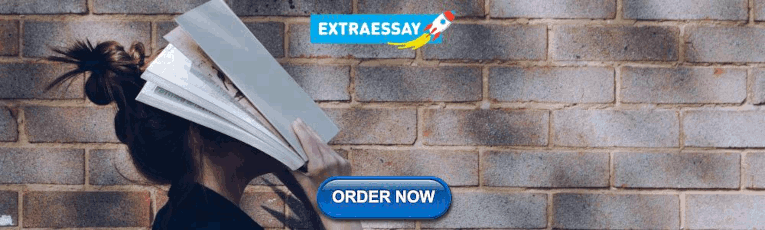
Ternary Operator Syntax
The Conditional Operator in PHP, also known as the Ternary Operator, assigns values to variables based on a certain condition. It takes three operands: the condition, the value to be assigned if the condition is true, and the value to be assigned if the condition is false.
Here’s an example:
In this example, the condition is $score >= 60 . If this condition is true, the value of $result is “Pass”. Otherwise, the value of $result is “Fail”.
To learn more, visit the PHP ternary operator tutorial . Let’s now explore the section below to delve into the Null Coalescing Operator in PHP.
The Null Coalescing Operator (??)
The Null Coalescing Operator, also known as the Null Coalescing Assignment Operator, assigns a default value to a variable if it is null. The operator has two operands: the variable and the default value it assigns if the variable is null. Here’s an example:
So, In this example, if the $_GET['name'] variable is null, the value of $name is “Guest”. Otherwise, the value of $name is the value of $_GET['name'] .
Here’s another pattern utilizing it with the assignment operator. Let’s proceed.
The Null Coalescing Assignment Operator (??=)
The Null Coalescing Operator with Assignment, also known as the Null Coalescing Assignment Operator, assigns a default value to a variable if it is null. The operator has two operands: the variable and the default value it assigns if the variable is null. Here’s an example:
So, the value of $name is null. The Null Coalescing Assignment Operator assigns the value “Guest” to $name. Therefore, the output of the echo statement is “Welcome,”Guest!”.
Moving into the following section, you’ll learn how to use the Elvis operator in PHP.
The Elvis Operator (?:)
In another hand, The Elvis Operator is a shorthand version of the Ternary Operator. Which assigns a default value to a variable if it is null. It takes two operands: the variable and the default value to be assigned if the variable is null. Here’s an example:
In this example, if the $_GET['name'] variable is null, the value“Guest” $name s “Guest”. Otherwise, the value of $name is the value of $_GET['name'] .
Let’s summarize it.
Wrapping Up
The Conditional Assignment Operators in PHP provide developers with powerful tools to simplify code and make it more readable. You can use these operators to assign values to variables based on certain conditions, assign default values to variables if they are null, and perform shorthand versions of conditional statements. By using these operators, developers can write more efficient and elegant code.
Did you find this article helpful?
Sorry about that. How can we improve it ?
- Facebook -->
- Twitter -->
- Linked In -->
- Install PHP
- Hello World
- PHP Constant
- PHP Comments
PHP Functions
- Parameters and Arguments
- Anonymous Functions
- Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
Control Structures
- If-else Block
- Break Statement
PHP Operators
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- PHP String Operators
- Array Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Null Coalescing Operator
Data Format and Types
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
String and Patterns
- Remove the Last Char
Home » PHP Tutorial » PHP if
Summary : in this tutorial, you’ll learn about the PHP if statement and how to use it to execute a code block conditionally.
Introduction to the PHP if statement
The if statement allows you to execute a statement if an expression evaluates to true . The following shows the syntax of the if statement:
In this syntax, PHP evaluates the expression first. If the expression evaluates to true , PHP executes the statement . In case the expression evaluates to false , PHP ignores the statement .
The following flowchart illustrates how the if statement works:
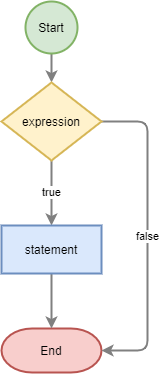
The following example uses the if statement to display a message if the $is_admin variable sets to true :
Since $is_admin is true , the script outputs the following message:
Curly braces
If you want to execute multiple statements in the if block, you can use curly braces to group multiple statements like this:
The following example uses the if statement that executes multiple statements:
In this example, the if statement displays a message and sets the $can_edit variable to true if the $is_admin variable is true .
It’s a good practice to always use curly braces with the if statement even though it has a single statement to execute like this:
In addition, you can use spaces between the expression and curly braces to make the code more readable.
Nesting if statements
It’s possible to nest an if statement inside another if statement as follows:
The following example shows how to nest an if statement in another if statement:
Embed if statement in HTML
To embed an if statement in an HTML document, you can use the above syntax. However, PHP provides a better syntax that allows you to mix the if statement with HTML nicely:
The following example uses the if statement that shows the edit link if the $is_admin is true :
Since the $is_admin is true , the script shows the Edit link. If you change the value of the $is_admin to false , you won’t see the Edit link in the output.
A common mistake with the PHP if statement
A common mistake that you may have is to use the wrong operator in the if statement. For example:
This script shows a message if the $checke d is 'off' . However, the expression in the if statement is an assignment, not a comparison:
This expression assigns the literal string 'off' to the $checked variable and returns that variable. It doesn’t compare the value of the $checked variable with the 'off' value. Therefore, the expression always evaluates to true , which is not correct.
To avoid this error, you can place the value first before the comparison operator and the variable after the comparison operator like this:
If you accidentally use the assignment operator (=), PHP will raise a syntax error instead:
- The if statement executes a statement if a condition evaluates to true .
- Always use curly braces even if you have a single statement to execute in the if statement. It makes the code more obvious.
- Do use the pattern if ( value == $variable_name ) {} to avoid possible mistakes.
Assignment inside a Condition
Share this article
It’s very common in PHP to see code written like this:
Thumbnail credit: sbwoodside
Frequently Asked Questions (FAQs) about Assignment Inside a Condition
What is an assignment inside a condition in programming.
An assignment inside a condition refers to the practice of assigning a value to a variable within a conditional statement such as an ‘if’ statement. This is a common practice in many programming languages including JavaScript, C++, and Python. It allows for more concise code as the assignment and the condition check can be done in a single line. However, it can also lead to confusion and potential bugs if not used carefully, as the assignment operation might be mistaken for a comparison operation.
Why is it considered bad practice to perform assignments in conditional expressions?
Assignments in conditional expressions can lead to confusion and potential bugs. This is because the assignment operator (=) can easily be mistaken for the equality operator (==). As a result, a condition that was meant to be a comparison could inadvertently become an assignment, leading to unexpected behavior in the code. Additionally, assignments in conditions can make the code more difficult to read and understand, particularly for less experienced programmers.
How can I avoid assignments in conditional expressions?
To avoid assignments in conditional expressions, you can separate the assignment and the condition check into two separate lines of code. For example, instead of writing if (x = getValue()) , you could write x = getValue(); if (x) . This makes the code clearer and reduces the risk of confusion or bugs.
Are there any situations where assignments in conditions are acceptable or even beneficial?
While generally discouraged, there are situations where assignments in conditions can be beneficial. For example, in a loop where a value needs to be updated and checked in each iteration, an assignment in the condition can make the code more concise. However, this should be done with caution and the code should be clearly commented to avoid confusion.
What is the difference between the assignment operator and the equality operator?
The assignment operator (=) is used to assign a value to a variable. For example, x = 5 assigns the value 5 to the variable x. On the other hand, the equality operator (==) is used to compare two values. For example, if (x == 5) checks whether the value of x is equal to 5.
How does TypeScript handle assignments in conditions?
TypeScript, like JavaScript, allows assignments in conditions. However, TypeScript has stricter type checking which can help catch potential errors caused by assignments in conditions. For example, if you try to assign a string to a variable that is supposed to be a number inside a condition, TypeScript will give a compile-time error.
What are some common bugs caused by assignments in conditions?
One common bug caused by assignments in conditions is an unintended assignment when a comparison was intended. For example, if (x = 5) will always be true because it assigns 5 to x, rather than checking if x is equal to 5. This can lead to unexpected behavior in the code.
How can I debug issues caused by assignments in conditions?
Debugging issues caused by assignments in conditions can be tricky because the code might not give any errors. One approach is to carefully check all conditional statements to ensure that they are using the correct operators. Using a linter or a static code analysis tool can also help catch these issues.
Can assignments in conditions be used in all programming languages?
Not all programming languages allow assignments in conditions. For example, Python does not allow assignments in conditions and will give a syntax error if you try to do so. Always check the syntax rules of the programming language you are using.
Are there any alternatives to assignments in conditions?
Yes, there are alternatives to assignments in conditions. One common alternative is to use a temporary variable to hold the value that needs to be assigned and checked. This can make the code clearer and easier to understand. Another alternative is to use a function that returns a value and then check that value in the condition.
James is a freelance web developer based in the UK, specialising in JavaScript application development and building accessible websites. With more than a decade's professional experience, he is a published author, a frequent blogger and speaker, and an outspoken advocate of standards-based development.
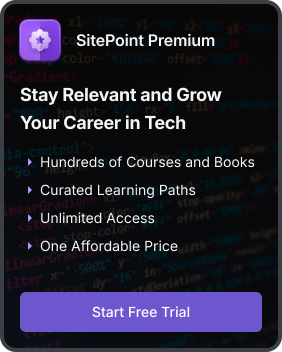
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php if statements.
Conditional statements are used to perform different actions based on different conditions.
PHP Conditional Statements
Very often when you write code, you want to perform different actions for different conditions. You can use conditional statements in your code to do this.
In PHP we have the following conditional statements:
- if statement - executes some code if one condition is true
- if...else statement - executes some code if a condition is true and another code if that condition is false
- if...elseif...else statement - executes different codes for more than two conditions
- switch statement - selects one of many blocks of code to be executed
PHP - The if Statement
The if statement executes some code if one condition is true.
Output "Have a good day!" if 5 is larger than 3:
We can also use variables in the if statement:
Output "Have a good day!" if $t is less than 20:
PHP Exercises
Test yourself with exercises.
Output "Hello World" if $a is greater than $b .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

Buckle up, fellow PHP enthusiast! We're loading up the rocket fuel for your coding adventures...
- Best Practices ,
- PHP: If statement in variable declaration
I'm new to PHP, and I've been trying to improve my programming skills by exploring different ways of using conditional statements. Recently, I came across a concept related to variable declaration within an if statement. I'm a bit confused about how this works and what purpose it serves. Can someone please explain to me what it means to have an if statement within a variable declaration in PHP? How does it work, and what are the benefits of using this approach? Additionally, it would be great if you could provide an example to help clarify things further. I appreciate any help or insights you can provide. Thank you!
All Replies
User 2: Greetings! I've had some experience with utilizing if statements in variable declarations in PHP, and it has proven to be quite handy in certain scenarios. The concept of incorporating an if statement within a variable declaration allows you to determine the value of the variable dynamically based on specific conditions. This can be highly useful when you need to perform different actions based on varying circumstances. Here's an instance that might shed some light on its practical application. Let's say you're developing an e-commerce platform, and you want to calculate the final price of a product based on certain conditions such as discounts, taxes, or shipping costs. By using an if statement in the variable declaration, you can programmatically determine the price. Here's an example: php $isDiscounted = true; $price = ($isDiscounted) ? $originalPrice * 0.8 : $originalPrice; In the above code snippet, the variable `$price` is assigned a discounted value if the condition `$isDiscounted` is true, and the original price otherwise. This allows for flexible pricing calculations without the need for long, repetitive if-else statements throughout your code. The benefit of this approach lies in its conciseness and readability. By incorporating if statements within variable declarations, you can streamline your code and make it more efficient. It helps to improve code maintainability and reduces the chances of duplication or errors. I hope this clarifies the concept for you! If you have any more queries or need further examples, feel free to ask.
User 1: Hey there! I've actually used if statements in variable declarations in PHP before, so I can definitely help you understand how it works. When you use an if statement in a variable declaration, it allows you to conditionally assign a value to the variable based on certain conditions. This can be quite useful when you want to set a variable's value dynamically depending on the outcome of certain conditions. For example, let's say you're building a website and you want to display a different Welcome message based on whether the user is logged in or not. You can use an if statement in the variable declaration to achieve this. Here's an example: php $isLoggedIn = true; $welcomeMessage = ($isLoggedIn) ? 'Welcome back!' : 'Please log in'; In the above code, I'm using the ternary operator (`?`) to check the condition `$isLoggedIn`. If it's `true`, it assigns the value `'Welcome back!'` to the variable `$welcomeMessage`. Otherwise, it assigns `'Please log in'`. This approach is useful because it allows you to easily handle different scenarios and dynamically assign values to variables based on conditions. It helps make your code more concise and readable, avoiding the need for additional if statements later on. I hope this explanation helps! If you have any further questions, feel free to ask.
More Topics Related to PHP
- What are the recommended PHP versions for different operating systems?
- Can I install PHP without root/administrator access?
- How can I verify the integrity of the PHP installation files?
- Are there any specific considerations when installing PHP on a shared hosting environment?
- Is it possible to install PHP alongside other programming languages like Python or Ruby?
More Topics Related to Variable Declaration
- How do I declare a string variable in PHP?
- codeigniter - A PHP Error was encountered Severity: Notice Message: Undefined variable: result
- What is the proper way to declare variables in php?
- javascript - What is the correct way to declare the jquery variable in PHP?
More Topics Related to If Statement
- PHP - If variable is not empty, echo some html code
- javascript - jQuery not recognizing PHP variable under if Statement
- mysql - PHP - if statement - with - if isset - using multiple variables issue
- Using IF statement to check if variable contains ' (pounds) sign in PHP
Popular Tags
- Best Practices
- Web Development
- Documentation
- Implementation

New to LearnPHP.org Community?
- Language Reference
- Control Structures
(PHP 4, PHP 5, PHP 7, PHP 8)
The switch statement is similar to a series of IF statements on the same expression. In many occasions, you may want to compare the same variable (or expression) with many different values, and execute a different piece of code depending on which value it equals to. This is exactly what the switch statement is for.
Note : Note that unlike some other languages, the continue statement applies to switch and acts similar to break . If you have a switch inside a loop and wish to continue to the next iteration of the outer loop, use continue 2 .
Note : Note that switch/case does loose comparison .
Example #1 switch structure
It is important to understand how the switch statement is executed in order to avoid mistakes. The switch statement executes line by line (actually, statement by statement). In the beginning, no code is executed. Only when a case statement is found whose expression evaluates to a value that matches the value of the switch expression does PHP begin to execute the statements. PHP continues to execute the statements until the end of the switch block, or the first time it sees a break statement. If you don't write a break statement at the end of a case's statement list, PHP will go on executing the statements of the following case. For example: <?php switch ( $i ) { case 0 : echo "i equals 0" ; case 1 : echo "i equals 1" ; case 2 : echo "i equals 2" ; } ?>
Here, if $i is equal to 0, PHP would execute all of the echo statements! If $i is equal to 1, PHP would execute the last two echo statements. You would get the expected behavior ('i equals 2' would be displayed) only if $i is equal to 2. Thus, it is important not to forget break statements (even though you may want to avoid supplying them on purpose under certain circumstances).
In a switch statement, the condition is evaluated only once and the result is compared to each case statement. In an elseif statement, the condition is evaluated again. If your condition is more complicated than a simple compare and/or is in a tight loop, a switch may be faster.
The statement list for a case can also be empty, which simply passes control into the statement list for the next case. <?php switch ( $i ) { case 0 : case 1 : case 2 : echo "i is less than 3 but not negative" ; break; case 3 : echo "i is 3" ; } ?>
Note : Multiple default cases will raise a E_COMPILE_ERROR error.
Note : Technically the default case may be listed in any order. It will only be used if no other case matches. However, by convention it is best to place it at the end as the last branch.
If no case branch matches, and there is no default branch, then no code will be executed, just as if no if statement was true.
A case value may be given as an expression. However, that expression will be evaluated on its own and then loosely compared with the switch value. That means it cannot be used for complex evaluations of the switch value. For example: <?php $target = 1 ; $start = 3 ; switch ( $target ) { case $start - 1 : print "A" ; break; case $start - 2 : print "B" ; break; case $start - 3 : print "C" ; break; case $start - 4 : print "D" ; break; } // Prints "B" ?>
For more complex comparisons, the value true may be used as the switch value. Or, alternatively, if - else blocks instead of switch . <?php $offset = 1 ; $start = 3 ; switch ( true ) { case $start - $offset === 1 : print "A" ; break; case $start - $offset === 2 : print "B" ; break; case $start - $offset === 3 : print "C" ; break; case $start - $offset === 4 : print "D" ; break; } // Prints "B" ?>
The alternative syntax for control structures is supported with switches. For more information, see Alternative syntax for control structures . <?php switch ( $i ): case 0 : echo "i equals 0" ; break; case 1 : echo "i equals 1" ; break; case 2 : echo "i equals 2" ; break; default: echo "i is not equal to 0, 1 or 2" ; endswitch; ?>
It's possible to use a semicolon instead of a colon after a case like: <?php switch( $beer ) { case 'tuborg' ; case 'carlsberg' ; case 'stella' ; case 'heineken' ; echo 'Good choice' ; break; default; echo 'Please make a new selection...' ; break; } ?>
Improve This Page
User contributed notes 6 notes.

Conditions in PHP
Hi! Here comes another PHP lesson. Today's topic is one of the most favorite among those who are starting to program. Still, because the conditions in PHP are what allows us to compose various algorithms. Depending on the conditions, the program will behave one way or another. And it is thanks to them that we can get different results with different input data. PHP has several constructs that you can use to implement conditions. All of them are used, and have their advantages in different situations, or, if you like, conditions. There are only conditions around, right? So. After all, no one will argue that in real life, depending on the circumstances, we act differently. In programming, this is no less important, and now we will learn this.
As you should remember from the last lesson, in PHP, depending on the operator, the operands are cast to a certain type. Conditional operators in PHP follow the same rules, and here the operand is always cast to a boolean value. If this value is true , then we consider that the condition is met, and if it is false , then the condition is not met. Depending on whether the condition is met, we can do or not do any actions. And here I propose to consider the first conditional statement - if .
"if" statement
This is the simplest and most commonly used operator. In general, the construction looks like this:
And in real life, the use of the if statement looks like this:
- Hello world
- Reverse Words in a String
- Even numbers
Here we have explicitly passed the value true to the condition. Of course, this is completely pointless. Let's use a condition to define numbers greater than 10. It's quite simple:
And after running we will see the result:
Number greater than 10 Quizzes PHP Quiz for beginners PHP Quiz for advanced MySQL Quiz for beginners All quizzes
"if-else" construct
Is it possible to make it so that when the condition is not met, another code is executed? Yes, you certainly may! To do this, use the else statement along with the if statement. It is written after the curly braces that enclose the code that is executed when the condition is met. And the structure looks like this:
Here again, a message will be displayed on the screen:
However, if we change the input data, and at the very beginning we assign the value 8 to the variable $x , then a message will be displayed:
Number less than or equal to 10
Try it right now.
"if-elseif-else" construct: multiple conditions
In case you need to check several conditions, an elseif statement is added after the if statement. It will check the condition only if the first condition is not met. For example:
In this case, the screen will display:
Number equal to 10
And yes, you can add else after this statement. The code inside it will be executed if none of the conditions are met:
The result of this code, I believe, does not need to be explained. Yes, by the way, a whole list of elseifs is possible. For example, like this:
Cast to boolean
Remember, in the lesson about data types in PHP , we learned how to explicitly cast values to any type. For example:
The result will be true . Working in the same way, only the implicit conversion always happens in the condition. For example, the following condition:
It will succeed because the number 3 will be converted to true . The following values will be cast to false :
- '' (empty string)
- 0 (number 0)
- [] (empty array)
Thus, any non-zero number and non-zero string will be converted to true and the condition will be met. The exception is a string consisting of one zero:
It will also be converted to false .
I covered this topic with casting to boolean in the homework assignment for this tutorial. Be sure to complete it. Now let's move on to the next conditional statement.
"switch" statement
In addition to the if-else construct, there is one more conditional operator. This is switch . This is a very interesting operator that requires memorization of several rules. Let's first see what it looks like in the following example:
At first glance, this operator may seem rather complicated. However, if you understand, then everything becomes clear. An expression is specified in the switch operand. In our case, this is the $x variable, or rather its value is 1 .
In curly braces, we enumerate case statements, after which we indicate the value with which the value of the switch operand is compared. The comparison is not strict, that is, as if we were using the == operator. And if the condition is met, then the code specified after the colon is executed. If none of the conditions is met, then the code from the default section is executed, which, in general, may not exist, and then nothing will be executed. Please note that inside each case section, at the end, we have written a break statement. This is done so that after the code is executed, if the condition is met, the condition check does not continue. That is, if there was no break at the end of the case 1 section, then after the text
The number is 1
would be displayed, the comparison condition with 2 would continue to be fulfilled, and then the code in the default section would also be executed. Don't forget to write break !
switch vs if
In general, this code could also be written using the if-elseif-else construct:
But in the form of a switch-case construct, the code in this particular case looks simpler. And that's why:
- we immediately see what exactly we are comparing (the $x variable) and understand that we are comparing this value in each condition, and not any other;
- it is more convenient for the eye to perceive what we are comparing with - the case 1, case 2 sections are visually perceived easier, the compared value is more noticeable.
And again about switch
And I haven’t said everything about switch yet - you can write several case-s in a row, then the code will be executed provided that at least one of them is executed. For example:
Agree, it can be convenient.
Okay, let's go over the features of the switch statement that you should always keep in mind.
- break breaks a set of conditions, do not forget to specify it;
- default section will be executed if none of the conditions are met. It may be completely absent;
- several _case_s can be written in a row, then the code in the section will be executed if at least one of the conditions is met.
A little practice
Well, remember the conditional operators? Let's put it into practice with more real examples.
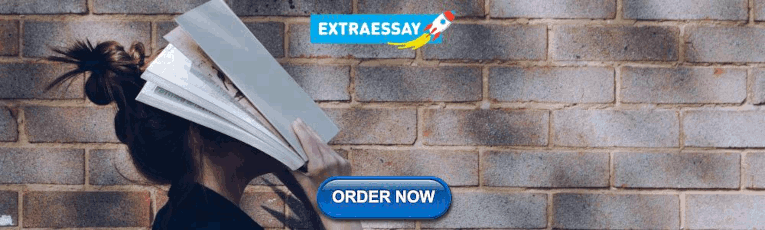
Even or Odd
Here is one example - you need to determine whether a number is even or not. To do this, we need to check that the remainder after dividing by 2 will be 0 . Read more about operators here . Let's do that:
Try changing the value of the $x variable yourself. Cool, yeah? It is working!
The absolute value of a number
Let's now learn how to calculate the modulus of a number. If the number is greater than or equal to zero, then you need to print this number itself, if it is less, change the sign from minus to plus.
Absolute value: 2
As we can see, everything worked out successfully.
Ternary operator
In addition, PHP has another operator, which is a shortened form of the if-else construct. This is a ternary operator. However, it returns different results depending on whether the condition is met or not. In general, its use is as follows:
Or using the same example of finding the absolute value of a number:
Cool, yeah? The ternary operator fits in very elegantly when solving simple problems like this.
And some more practice
Conditions can be placed inside each other and in general, what can you not do with them. For example:
What is the result
Friends, I hope you enjoyed the lesson. If so, I will be glad if you share it on social networks or tell your friends. This is the best support for the project. Thanks to those who do it. If you have any questions or comments - write about it in the comments. And now - we are all quickly doing our homework, there are even more interesting examples with conditions. Bye everyone!
Try the following conditions:
- if ('string') {echo 'Condition met';}
- if (0) {echo 'Condition met';}
- if (null) {echo 'Condition met';}
- if (5) {echo 'Condition met';}
Explain the result.
Use the ternary operator to determine if a number is even or odd and print the result.
- PHP tutorial for beginners
- MySQL tutorial for beginners
- PHP tutorial for advanced
- PHP tutorial for professionals
- PHP Tutorial
- PHP Exercises
- PHP Calendar
- PHP Filesystem
- PHP Programs
- PHP Array Programs
- PHP String Programs
- PHP Interview Questions
- PHP IntlChar
- PHP Image Processing
- PHP Formatter
- Web Technology
What is a Conditional Statement in PHP?
- Conditional Statements in JavaScript
- PHP continue Statement
- PHP goto Statement
- What is the difference between == and === in PHP ?
- Calculator in PHP using If-Else Statement
- What are the Basic Data Types in PHP ?
- What is the use of “=>” symbol in PHP ?
- PHP | XMLWriter startComment() Function
- How to bind an array to an IN() condition in PHP ?
- Difference between try-catch and if-else statements in PHP
- Conditional Statements in Python
- Conditional Statements in COBOL
- Conditional Statements | Shell Script
- MATLAB - Conditional Statements
- Combining Conditional Statements in Golang
- Conditional Statements in Programming | Definition, Types, Best Practices
- Conditional or Ternary Operator (?:) in C
- Conditionals in Rust
- How to use conditional operator in jQuery a template?
- How to execute PHP code using command line ?
- How to pop an alert message box using PHP ?
- How to Insert Form Data into Database using PHP ?
- How to delete an array element based on key in PHP?
- PHP in_array() Function
- How to Upload Image into Database and Display it using PHP ?
- How to check whether an array is empty using PHP?
- PHP | strval() Function
- How to receive JSON POST with PHP ?
- Comparing two dates in PHP
A conditional statement in PHP is a programming construct that allows you to execute different blocks of code based on whether a specified condition evaluates to true or false. It enables you to create dynamic and flexible code logic by controlling the flow of execution based on various conditions.
Conditional statements in PHP include:
PHP conditional statements, like if, else, elseif, and switch, control code execution based on specified conditions, enhancing code flexibility and logic flow.
if statement :
PHP if statement executes a block of code if a specified condition is true.
else statement :
PHP else statement executes a block of code if the condition of the preceding if statement evaluates to false.
else if statement :
PHP else if statement allows you to evaluate multiple conditions sequentially and execute the corresponding block of code if any condition is true.
switch statement :
PHP switch statement provides an alternative to multiple elseif statements by allowing you to test a variable against multiple possible values and execute different blocks of code accordingly.
Please Login to comment...
Similar reads.
- WebTech-FAQs
- Web Technologies
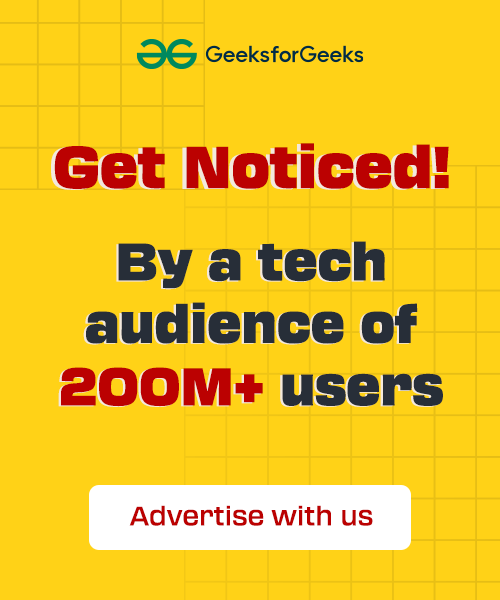
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
PHP Variable Assignment Within If Statement
The usual practice when checking for the return value of functions is to run the function and store the value in a variable, and then test that variable. Here is an example of that process using the strstr() function.
This code will output "bool(false)" as that was the return value of the strstr() function.
There is another way to write the same code within a single if statement. The following example assigns the variable and checks the return value in a single line of code.
This code will output "bool(false)" again as this has the same function as the previous example.
There is one problem with assigning variables in this way, and this is that you can only do one. If you try to assign more than one variable at a time you will find that everything but the final variable will turn out to be the outcome of the boolean comparison. Take the following example, which uses the same $string variable as the other examples.
The output of this code will be as follows:
The first two comparisons come out as true and so $var1 and $var2 are assigned boolean values of true. The final comparison is the only one that is assigned the expected value. This might be a bug in PHP, but as this is a non-standard way of assigning variables I don't think many people have come across it.
Submitted by James Bower on Mon, 09/07/2009 - 21:17
Submitted by Mirek Suk on Tue, 09/28/2010 - 12:08
Name Philip Norton
Submitted by philipnorton42 on Wed, 09/29/2010 - 08:56
Submitted by Jim on Sun, 11/07/2010 - 23:09
This works too: if( !($val = $arg) ) { $val = 'default'; }
Submitted by Frank on Mon, 05/02/2011 - 17:52
Submitted by Vishal on Sun, 05/17/2015 - 09:24
Submitted by Jesus on Tue, 03/20/2018 - 14:55
Submitted by philipnorton42 on Tue, 03/20/2018 - 15:19
A gotcha worth highlighting, assigning a 0 is of course a falsy, so you can work around it as follows where NULL is returned when we really want to exit, and not on 0.
Submitted by Russ on Fri, 05/31/2019 - 18:18
Hi, I need help with below code
How to create variable with if multiple statement in php and print
and want to print as
Submitted by Sam on Fri, 05/15/2020 - 21:30
For fixed payment types, you may be better off to assign an array with the options, with the code as key, and display name as value. That array could be filled from a database query on payment types allowed, which would then pick up when you add a new option, you don't then have to re-code so much.
That has no input validation etc, but might be a start.
Submitted by Peter H on Fri, 06/05/2020 - 12:24
Add new comment
Related content, recreating spotify wrapped in php.
I quite like the end of the year report from Spotify that they call "Wrapped". This is a little application in which they tell you what your favorite artist was and what sort of genres you listened to the most during the year.
Should A Constructor Throw An Exception?
Let's say you had a class that you wanted to use, but there was some sort of error in creating the object. This might be that the wrong parameters were passed, or the third party service (eg. a database) wasn't available at the time of creation.
PHP Question: Variable Reference
What does the following code print out?
Creating An Authentication System With PHP and MariaDB
Using frameworks to handle the authentication of your PHP application is perfectly fine to do, and normally encouraged. They abstract away all of the complexity of managing users and sessions that need to work in order to allow your application to function.
Creating Sparklines In PHP
A sparkline is a very small line graph that is intended to convey some simple information, usually in terms of a numeric value over time. They tend to lack axes or other labels and are added to information readouts in order to expand on numbers in order to give them more context.
PHP:CSI - Improving Bad PHP Logging Code
I read The Daily WTF every now and then and one story about bad logging code in PHP stood out to me. The post looked at some PHP code that was created to log a string to a file, but would have progressively slowed down the application every time a log was generated.
PHP - If Statements and Variables
- Posted on March 14, 2013
GeekThis remains completely ad-free with zero trackers for your convenience and privacy. If you would like to support the site, please consider giving a small contribution at Buy Me a Coffee .
In PHP and other programming languages you don’t want all the code to execute unless a specific value has been reached or is set. To get this functionality we use “If” statements. If statements are a way to check and compare variables to see if they are set to values you want to process code. For instance, if a user is logged into a site, that is when you grab their account information. If the user is not logged into the site, there is no need to grab account information. This is applied to all aspects of the code.
First thing we need to do is create our PHP file again and set some variables. We will add onto this code throughout this tutorial.
This simple PHP file just sets a variable “age” to 20 then shows it on the screen. But what if we only care about if the person is 18 or older, or under 18?
We run two if statements to see if 18 or older, and then to see if they are under 18 and if they are we echo different results. If you don’t understand the format of the “if” statement it goes something like this.
First you specify it to run if() . Inside the parentheses we add our request. You have 2 variables a left and a right, and compare them using the possible operations below.
There is another operation but we will get into that later when working with booleans (true or false). All of these operations also allow for “!” in front of them to mean “NOT”. This lets you check if the operation is NOT true. After the closing parentheses we add a curly bracket opening and closing. Inside the curly brackets we add the code that only executes when the “if” statement is true.
In the code about the age check, the “Over 18” will be printed out since the age is set to 20, which is over 18. If we set it to 17 we will get a different result though. But the above code is bad because we we checking the same variable twice and it only has two possible options in our current case, either 18 or older, or under 18. We should use the “else” command instead.
In this code, if they are not >= 18, “else” will run without having to check any variables. The “else” statement is setup similar to “if” in the sense it has brackets in which only that code will execute if it is true. You don’t have to set up variables to compare since we already did that in the first if statement. Else statements have to be linked to an “if” statement like the one above, right after the “if” closing curly bracket you need to place the “else” statement.
Let us look at another example just to show more “if” statements being used.
We have multiple statement here, and they are nested instead of each other. We first check if they are 18 or older, if they are we then check if they are approved (when approved equals 1, not 0). Each statement has an “else” statement connected to it showing the user a “status” as to why they can’t access the program or site, and then of course if they do have access we tell them. But this code is a little messy and could easily be changed to perform better. They only have access if approved and 18 or older, so let’s make that a single “if” statement, and then have the else handle the “error” messages.
The above code has 2 sets of variables getting compared in the first “if” statement, and if they are both true will make the “if” statement true. We use the symbol “&&” to mean “and”, so both the left and right side of that “if” statement have to be true to work. We could use “||” to mean “or”, so either side has to be true or both for the “if” statement to work. Then on the “else” we check why they are not allowed, and use two separate statements to check if it was because of age and to check if it was because they were approved or not.
You do not always have to show the user exactly why something happened, but if it includes user interaction it is a great idea so they know what they need to fix or wait for. If you just “echo” out “Can’t Access Site” instead of telling them about the age or approved, they won’t know what is wrong, and may just assume the site is not working. But don’t share too much information to the user because it became a privacy concern; but we will get into that in the future.
If I told you to create a site to only allow America and Canada to have access to it. And if a visitor doesn’t have access to it show them the message “Only American and Canadian visitors are allowed”, how will you do it? The visitors country is in a text format of “America”, “Canada”, “Mexico”, etc.
This code will look something similar to this, but do note that if your code doesn’t look exactly the same that is fine, no two codes look alike.
I use a single “if” and then “else” to check. Inside the “if” statement I check if country equals america OR country equals canada. But using string variables for this type of checking isn’t that good of an idea because it uses more space to store information in a database or file. So let us make each country have a number (PHP code will only get the number, not the string)
Now we only need to check if the country is 1 or 2, not a string. This is how most sites will handle this. They will have a database table containing 2 columns, ID and Country. Then users will store the ID of the country they are located in. The string is used only when we need to show the country name dynamically, for instance on a users profile where we display location. Now create a PHP script where instead of strings we use the integers above to check if they are in the USA or Canada.
This is much cleaner and more reliable. There is one more type of “if” statement I want to show before this tutorial ends and we move into part 3, the “else if” statement. It works similar to “else” making it run only when the “if” statement before it isn’t true, but it also allows parameter like in “if” to refine our statements. If I wanted USA and Canada to both see different sites I would consider using if, else if, and then else.
First we check “if” country is 1, if it is none of the other “if”, or “else” statements execute. If it isn’t true, we check “else if” country is 2. And then it keeps going down the “if” statements until it reaches one that is true. If none of them are true it goes to the final “else” or doesn’t execute any code if there is no “else” at the end. You can have unlimited “else if” statements attached together but you can only have 1 “else” and 1 “if” in a series.
For example, the below code has multiple “else if” statements attached to the first “if” statement.
Part 1: PHP Introduction of Variables and Functions
Related Posts
Prevent sending http referer headers from your website.
Learn how to prevent your site from sending HTTP referer headers to external websites that you link to with these three different methods.
Process Incoming Mail with PHP Script with Exim
Learn how to process incoming e-mails to your server with a PHP or other script.
PHP - Check if Production or Sandbox
Use PHP to check of your site is on your development server or uploaded to the production server.
Custom Style RSS Feed
Customize the look and feel of your WordPress RSS feed by adding a stylesheet to your RSS XML feed.

- PHP All Exercises & Assignments
Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database.
Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
Here, you will find a list of PHP programs, along with problem description and solution. These programs can also be used as assignments for PHP students.
Write a program to count 5 to 15 using PHP loop
Description: Write a Program to display count, from 5 to 15 using PHP loop as given below.
Rules & Hint
- You can use “for” or “while” loop
- You can use variable to initialize count
- You can use html tag for line break
View Solution/Program
5 6 7 8 9 10 11 12 13 14 15
Write a program to print “Hello World” using echo
Description: Write a program to print “Hello World” using echo only?
Conditions:
- You can not use any variable.
View Solution /Program
Hello World
Write a program to print “Hello PHP” using variable
Description: Write a program to print “Hello PHP” using php variable?
- You can not use text directly in echo but can use variable.
Write a program to print a string using echo+variable.
Description: Write a program to print “Welcome to the PHP World” using some part of the text in variable & some part directly in echo.
- You have to use a variable that contains string “PHP World”.
Welcome to the PHP World
Write a program to print two variables in single echo
Description: Write a program to print 2 php variables using single echo statement.
- First variable have text “Good Morning.”
- Second variable have text “Have a nice day!”
- Your output should be “Good morning. Have a nice day!”
- You are allowed to use only one echo statement in this program.
Good Morning. Have a nice day!
Write a program to check student grade based on marks
Description:.
Write a program to check student grade based on the marks using if-else statement.
- If marks are 60% or more, grade will be First Division.
- If marks between 45% to 59%, grade will be Second Division.
- If marks between 33% to 44%, grade will be Third Division.
- If marks are less than 33%, student will be Fail.
Click to View Solution/Program
Third Division
Write a program to show day of the week using switch
Write a program to show day of the week (for example: Monday) based on numbers using switch/case statements.
- You can pass 1 to 7 number in switch
- Day 1 will be considered as Monday
- If number is not between 1 to 7, show invalid number in default
It is Friday!
Write a factorial program using for loop in php
Write a program to calculate factorial of a number using for loop in php.
The factorial of 3 is 6
Factorial program in PHP using recursive function
Exercise Description: Write a PHP program to find factorial of a number using recursive function .
What is Recursive Function?
- A recursive function is a function that calls itself.
Write a program to create Chess board in PHP using for loop
Write a PHP program using nested for loop that creates a chess board.
- You can use html table having width=”400px” and take “30px” as cell height and width for check boxes.

Write a Program to create given pattern with * using for loop
Description: Write a Program to create following pattern using for loops:
- You can use for or while loop
- You can use multiple (nested) loop to draw above pattern
View Solution/Program using two for loops
* ** *** **** ***** ****** ******* ********
Simple Tips for PHP Beginners
When a beginner start PHP programming, he often gets some syntax errors. Sometimes these are small errors but takes a lot of time to fix. This happens when we are not familiar with the basic syntax and do small mistakes in programs. These mistakes can be avoided if you practice more and taking care of small things.
I would like to say that it is never a good idea to become smart and start copying. This will save your time but you would not be able to understand PHP syntax. Rather, Type your program and get friendly with PHP code.
Follow Simple Tips for PHP Beginners to avoid errors in Programming
- Start with simple & small programs.
- Type your PHP program code manually. Do not just Copy Paste.
- Always create a new file for new code and keep backup of old files. This will make it easy to find old programs when needed.
- Keep your PHP files in organized folders rather than keeping all files in same folder.
- Use meaningful names for PHP files or folders. Some examples are: “ variable-test.php “, “ loops.php ” etc. Do not just use “ abc.php “, “ 123.php ” or “ sample.php “
- Avoid space between file or folder names. Use hyphens (-) instead.
- Use lower case letters for file or folder names. This will help you make a consistent code
These points are not mandatory but they help you to make consistent and understandable code. Once you practice this for 20 to 30 PHP programs, you can go further with more standards.
The PHP Standard Recommendation (PSR) is a PHP specification published by the PHP Framework Interop Group.
Experiment with Basic PHP Syntax Errors
When you start PHP Programming, you may face some programming errors. These errors stops your program execution. Sometimes you quickly find your solutions while sometimes it may take long time even if there is small mistake. It is important to get familiar with Basic PHP Syntax Errors
Basic Syntax errors occurs when we do not write PHP Code correctly. We cannot avoid all those errors but we can learn from them.
Here is a working PHP Code example to output a simple line.
Output: Hello World!
It is better to experiment with PHP Basic code and see what errors happens.
- Remove semicolon from the end of second line and see what error occurs
- Remove double quote from “Hello World!” what error occurs
- Remove PHP ending statement “?>” error occurs
- Use “
- Try some space between “
Try above changes one at a time and see error. Observe What you did and what error happens.
Take care of the line number mentioned in error message. It will give you hint about the place where there is some mistake in the code.
Read Carefully Error message. Once you will understand the meaning of these basic error messages, you will be able to fix them later on easily.
Note: Most of the time error can be found in previous line instead of actual mentioned line. For example: If your program miss semicolon in line number 6, it will show error in line number 7.
Using phpinfo() – Display PHP Configuration & Modules
phpinfo() is a PHP built-in function used to display information about PHP’s configuration settings and modules.
When we install PHP, there are many additional modules also get installed. Most of them are enabled and some are disabled. These modules or extensions enhance PHP functionality. For example, the date-time extension provides some ready-made function related to date and time formatting. MySQL modules are integrated to deal with PHP Connections.
It is good to take a look on those extensions. Simply use
phpinfo() function as given below.
Example Using phpinfo() function

Write a PHP program to add two numbers
Write a program to perform sum or addition of two numbers in PHP programming. You can use PHP Variables and Operators
PHP Program to add two numbers:
Write a program to calculate electricity bill in php.
You need to write a PHP program to calculate electricity bill using if-else conditions.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements .
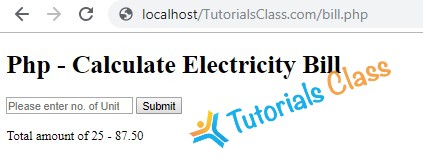
Write a simple calculator program in PHP using switch case
You need to write a simple calculator program in PHP using switch case.
Operations:
- Subtraction
- Multiplication

Remove specific element by value from an array in PHP?
You need to write a program in PHP to remove specific element by value from an array using PHP program.
Instructions:
- Take an array with list of month names.
- Take a variable with the name of value to be deleted.
- You can use PHP array functions or foreach loop.
Solution 1: Using array_search()
With the help of array_search() function, we can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 2: Using foreach()
By using foreach() loop, we can also remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 3: Using array_diff()
With the help of array_diff() function, we also can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [2]=> string(5) “march” [4]=> string(3) “may” }
Write a PHP program to check if a person is eligible to vote
Write a PHP program to check if a person is eligible to vote or not.
- Minimum age required for vote is 18.
- You can use PHP Functions .
- You can use Decision Making Statements .
Click to View Solution/Program.
You Are Eligible For Vote
Write a PHP program to calculate area of rectangle
Write a PHP program to calculate area of rectangle by using PHP Function.
- You must use a PHP Function .
- There should be two arguments i.e. length & width.
View Solution/Program.
Area Of Rectangle with length 2 & width 4 is 8 .
- Next »
- PHP Exercises Categories
- PHP Top Exercises
- PHP Variables
- PHP Decision Making
- PHP Functions
- PHP Operators
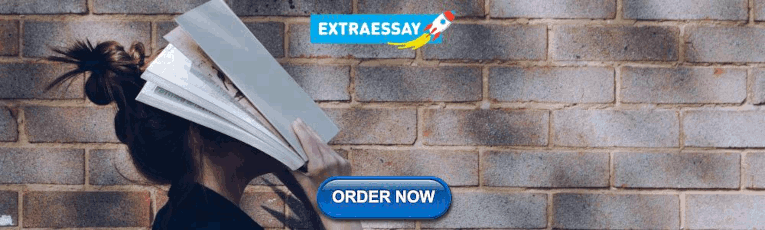
IMAGES
VIDEO
COMMENTS
php variable as conditional assignment. 3. Assign variable whilst checking it. 0. ... Assign and use variable inside of PHP if statement. 2. Assigning a if statement's condition to a variable. Hot Network Questions Companies carrying out private investigations and prosecutions
Conditional Assignment Operator in PHP is a shorthand operator that allow developers to assign values to variables based on certain conditions. In this article, we will explore how the various Conditional Assignment Operators in PHP simplify code and make it more readable. Let's begin with the ternary operator. Ternary Operator Syntax
if. ¶. The if construct is one of the most important features of many languages, PHP included. It allows for conditional execution of code fragments. PHP features an if structure that is similar to that of C: statement. As described in the section about expressions, expression is evaluated to its Boolean value.
The if statement allows you to execute a statement if an expression evaluates to true. The following shows the syntax of the if statement: statement; Code language: HTML, XML (xml) In this syntax, PHP evaluates the expression first. If the expression evaluates to true, PHP executes the statement. In case the expression evaluates to false, PHP ...
Read Assignment inside a Condition and learn with SitePoint. Our web development and design tutorials, courses, and books will teach you HTML, CSS, JavaScript, PHP, Python, and more.
PHP Conditional Statements. Very often when you write code, you want to perform different actions for different conditions. You can use conditional statements in your code to do this. In PHP we have the following conditional statements: if statement - executes some code if one condition is true
This may also have relevance if you need to copy something like a large array inside a tight loop. An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword. ...
Decisions written in code are formed using conditionals: "If x, then y.". Even a button click is a form of condition: "If this button is clicked, go to a certain page.". Conditional statements are part of the logic, decision making, or flow control of a computer program. You can compare a conditional statement to a "Choose Your Own ...
variable assignment inside 'IF' condition. Ask Question Asked 12 years ago. Modified 12 years ago. Viewed 3k times Part of PHP Collective ... php variable assignment inside if conditional. 1. Assignment in conditional, why it's not working? 3. Assign variable whilst checking it. 9.
When you use an if statement in a variable declaration, it allows you to conditionally assign a value to the variable based on certain conditions. This can be quite useful when you want to set a variable's value dynamically depending on the outcome of certain conditions. For example, let's say you're building a website and you want to display a ...
(PHP 4, PHP 5, PHP 7, PHP 8) ... If you have a switch inside a loop and wish to continue to the next iteration of the outer loop, use continue 2. Note: ... statement, the condition is evaluated again. If your condition is more complicated than a simple compare and/or is in a tight loop, a switch may be faster.
PHP Code Quality heresy— assignments inside IF statements. One of the big changes in PHP over the last few years has been that emergence of a relatively common set of code quality standards. So ...
After updating to PHP 8.0, I started using match in my newer projects. The reduction in lines of code and improved readability was evident. The reduction in lines of code and improved readability ...
Parsing conditions in PHP: if-else constructs, multiple if conditions, switch-case statement, and ternary operator. ... I covered this topic with casting to boolean in the homework assignment for this tutorial. Be sure to complete it. Now let's move on to the next conditional statement. ... Conditions can be placed inside each other and in ...
Collectives™ on Stack Overflow - Centralized & trusted content around the technologies you use the most.
A conditional statement in PHP is a programming construct that allows you to execute different blocks of code based on whether a specified condition evaluates to true or false. It enables you to create dynamic and flexible code logic by controlling the flow of execution based on various conditions. Conditional statements in PHP include:
PHP Variable Assignment Within If Statement. Note: This post is over two years old and so the information contained here might be out of date. If you do spot something please leave a comment and we will endeavour to correct. ... This might be a bug in PHP, but as this is a non-standard way of assigning variables I don't think many people have ...
If it is truthy, the code in the conditional executes. You will oftentimes see this sort of assignment/evaluation in the case of looping through database result sets, but generally I would suggest that it should be avoided outside such a common use case for sake of clarity in reading g code.
To get this functionality we use "If" statements. If statements are a way to check and compare variables to see if they are set to values you want to process code. For instance, if a user is logged into a site, that is when you grab their account information. If the user is not logged into the site, there is no need to grab account information.
So in this case, PHP determines that it needs to do the assignment before it can negate the result of the assignment. I think a similar thing is happening here, although you're right that PHP should be able to do the assignment later. Actually, the result might be unpredictable, as is the case with some other expressions:
Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database. Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions. Here, you will find a list of PHP programs, along with problem description and solution.